Mastering JavaScript: A Beginner's Guide to the Language Powering the Web
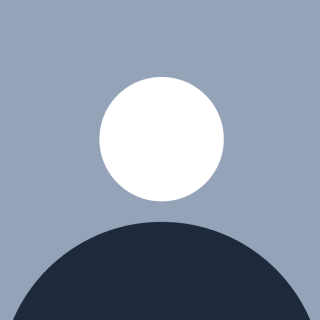
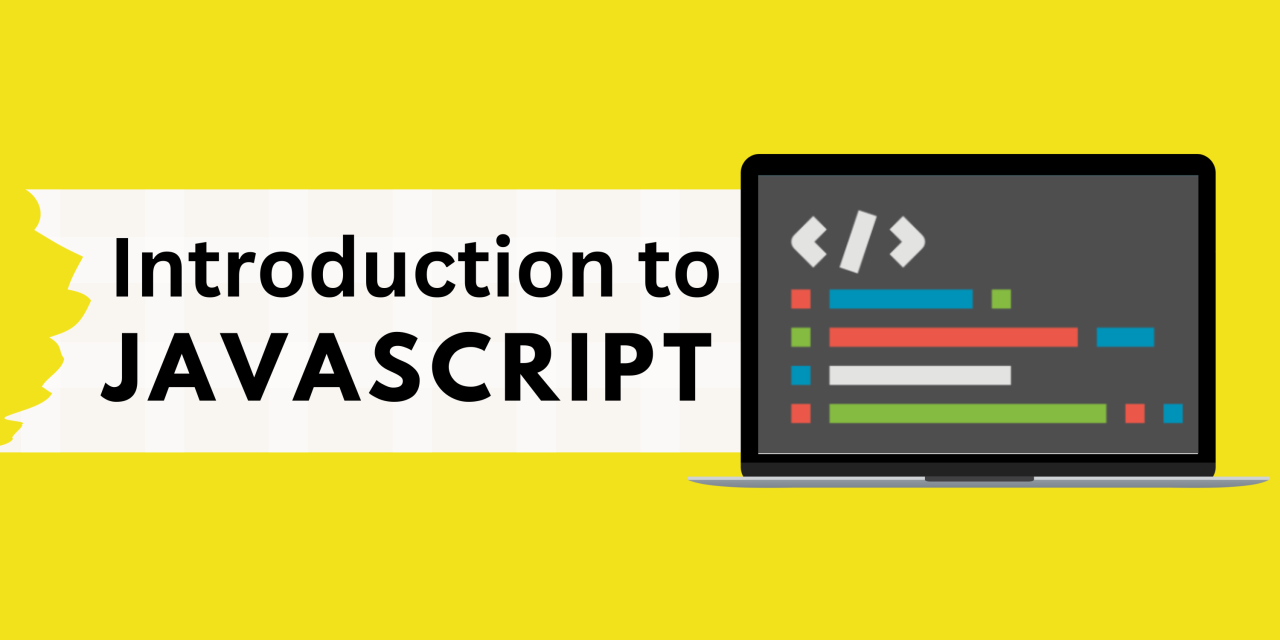
So you know the web pages that have buttons, links and other styles on it but how does other functionality works
How is the page changing when we click on a button
Why it’s loading 2nd image before 1st image
how it’s deciding size of various sections of a web page dynamically
The magic behind these dynamic changes is JavaScript – the programming language that brings web pages to life.
Initially, JavaScript was created to add interactive elements to web pages. However, over time, developers realized its potential beyond the front-end. Today, JavaScript is a full-fledged language used for both front-end and back-end development.
But the fun part is that javascript does not support any synchronous or asynchronous nature natively
then how those dynamic loading occurs ?
It’s because of Javascript Engine ( V8 , SpiderMonkey, etc) or say browsers and Node.js APi as with help of these Javascript runs asynchronous tasks
Fun Fact : Javascript variables can store any thing that could be stored in memory so you can do anything by using javascript for sure
And also javascript is a Object Oriented Programming language (OOPL)
OOPL meaning is that we can easily define any real life entity in this programming language we will discuss more about it in upcoming article as whole javascript moves around objects
Now let’s start learning Javascript from scratch to build some thing crazy will be done via blogs on blog.unknownbug.tech
Javascript Variables
Now if you want to process any thing you must need to store that thing somewhere right ? or else how could you process it
So for storing those things ( here Data ) we use variables
In lame word variables are just few boxes and data is like balls inside those boxes nothing more then that
But as we discussed above in this article javascript can store anything in it like literally any anything let it be some text, images, videos or some address of your memory. As in javascript we do not have to specify the datatype of any thing before storing any variable we store all the variables here by simply writing 3 keywords let, const , var
However there is naming convention for different type of variables such as
Datatype | Examples or Explaination |
Number | 2 , -10 , 0 , 0.3 , -0.4 ( Numbers with decimals are also called float ) |
String | “Hello”,”h” |
boolean | true,false |
null | it like explicitly(in no values stored and it’s possible that value may never be stored in it ( let’s say a variable that stores details about their Netflix account but it’s possible that a person exist who does not have the netflix account so variable will be empty for ever ) saying that variable is empty |
undefined | it means variable is empty implicitly ( means we declared a variable and will store some data in it soon ) |
Object | Contains object in it |
Array | Contains Array |
Example for object
{
name:"Shivam", // object has attribute storing a string
age:22, // object has attribute storing a number
billingDetails:null // object has attribute storing a null or storing nothing
}
Example for Array
let arr = ["apple",22,"B",true] // can stor diffrent types apart from null or undefined
Datatypes such that : String, Number, Boolean , Null, Undefined are called primitive Datatype
Datatypes such that : array and object are called non primitive Datatype
There is also methods assigned to every datatype using which you can perform many crucial and complex actions with ease in optimal way I already discussed about those methods here and for objects we will discuss it later
Also already wrote articles on how to make our custom methods in this article
now let’s dive into more easy topics yet crucial and important for programming in javascript
Operators in Javascript
Like mathematics it does have some operators to perform mathematical computation like
Arithmetic Operators
Operator | Task |
+ | To Add |
- | To subtract |
/ | To divide |
* | To multiply |
% | to get remainder (eg : 3%2 = 1 and 2%3 == 2) |
Comparison Operators
Operator | Task |
\== | Checks is there value is equal ( returns true for “2” == 2 ) |
\=== | Strictly Checks if both values are equal ( returns false for “2” === 2 ) |
!= | Checks Not equal to |
!== | Strictly checks not equal to |
> | More then |
< | less then |
>= | More then equal to |
<= | less then equal to |
Logical Operators
And Operator
Represented by &&
for x && y (x and y are two expression )
x | x | output |
false | false | false |
false | true | false |
true | false | false |
true | true | true |
Or Operator
Represented by ||
for x && y (x and y are two expression )
x | y | output |
false | true | false |
false | true | true |
true | false | true |
true | true | true |
Not Operator
For X = true → !X returns false
For X = false → !X returns true
Var , Let and Const
Let’s Start with Let
but before that
if(){ // block scope starts here
} // block scopes ends here
function(){ // function or block scope starts here
} // function or block scope ends here
Let
it’s the most commonly used variable in javascript
It can not be re declared in same scope no matter if it is a function scope or Block Scope
It can be defined without giving any value
- will have
undefined
as it’s value if not given initially
- will have
It can be reassigned
If declare in a scope then it can only be accessed in that scope it self
Const
it’s second most commonly used variable in javascript
It can not be re declared in same scope no matter if it is a function scope or Block Scope
It can not be defined without giving any value
It can not be reassigned
Var
It’s rarely used now days as it is very confusing how ever will try to explain it too you
It can be re declared in same scope no matter if it is a function scope or Block Scope
It can be defined without giving any value
- will have
undefined
as it’s value if not given initially
- will have
It can be reassigned
Now the confusing part
If declared in function scope then it’s not accessible outside the scope
If it’s declared inside a block scope then it’s accessible in the code
Hoisting and Temporal Dead Zone (TDZ) in JavaScript
Hoisting is JavaScript’s behavior of moving variable and function declarations to the top of their scope before code execution.
However, there’s a key difference between var
, let
, and const
regarding hoisting:
var
is hoisted and initialized asundefined
.let
andconst
are hoisted but not initialized. means if you are writing a variable at line number 12 using let ann const then you can’t use it at line number 11 or earlier linesFunctions (declared with
function
) are fully hoisted (both declaration and definition).
Now let’s talk about Temporal Dead Zone no such thing exists for var but for let and const it’s important concept
Line 100 : console.log(myName); // Cannot access 'myName' before initialization
Line 101 : let myName = "Vineet"
Line 102 : console.log(myName); //'Vineet'
here from line 0 to 100 is the TDZ for variable myName
but for var at line number 100 it will print undefined
Line 100 : console.log(myName); //undefined
Line 101 : let myName = "Vineet"
Line 102 : console.log(myName); //'Vineet'
Subscribe to my newsletter
Read articles from Vineet Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by