Building a HIPAA and DPDPA-Compliant Healthcare MVP
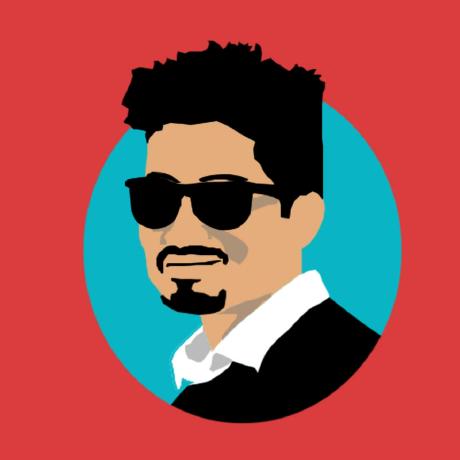

The healthcare industry is one of the most regulated industries globally. Building a healthcare MVP (Minimum Viable Product) that processes patient data requires more than just strong software architecture—it demands rigorous security controls, data privacy protection, and strict compliance with government regulations.
Healthcare data is highly sensitive and valuable, containing not only personal details like names and addresses but also medical histories, lab results, imaging files, and insurance information. A data breach in a healthcare system is catastrophic, often leading to:
Legal penalties and fines (up to $1.5 million per violation for HIPAA breaches)
Criminal charges (in cases of intentional HIPAA violations)
Reputational damage
Loss of business contracts and customer trust
Two key regulations apply when building healthcare platforms:
HIPAA (Health Insurance Portability and Accountability Act) – Governs patient data privacy and security in the United States.
DPDPA (Digital Personal Data Protection Act) – Regulates personal and healthcare data in India.
To avoid compliance failures and build a secure, scalable, and future-proof product, healthcare startups must address both security and compliance at every level of the architecture—from data encryption and secure transmission to role-based access and audit trails.
This guide provides a professional, technical roadmap for building a HIPAA and DPDPA-compliant healthcare MVP using:
✅ Python Django (Backend)
✅ React (Frontend)
✅ PostgreSQL (Database)
✅ Docker (Containerization)
✅ Kubernetes (Scaling)
✅ AWS (Infrastructure)
✅ BigQuery (Data Analysis)
✅ GitLab (CI/CD)
By the end of this guide, you will have a detailed blueprint for launching a compliant and production-ready healthcare MVP.
Understanding HIPAA and DPDPA
🔎 What is HIPAA?
The Health Insurance Portability and Accountability Act (HIPAA) is a US federal law enacted in 1996 to protect sensitive patient data (Protected Health Information – PHI). It regulates how healthcare organizations and their business partners store, transmit, and manage patient data.
✅ Types of PHI Covered Under HIPAA
HIPAA protects 18 types of patient data:
Patient name
Date of birth
Address
Phone number
Email
Medical history
Lab results
Insurance details
Payment information
Biometric data
Genetic information
Healthcare plan participation
🛡️ Key Components of HIPAA
Component | Description | Example |
Privacy Rule | Defines the use and disclosure of PHI | Consent for sharing data |
Security Rule | Protects PHI integrity, confidentiality, and availability | Encryption and access control |
Breach Notification Rule | Requires reporting breaches within 60 days | Email to affected patients |
Enforcement Rule | Defines penalties for violations | Fines and corrective action |
Omnibus Rule | Expands liability to business associates | Data processors and vendors |
🔎 What is DPDPA?
The Digital Personal Data Protection Act (DPDPA) 2023 governs personal data protection in India, including healthcare data.
✅ Types of Data Covered Under DPDPA
Personal data (name, email, phone)
Financial data
Health records (diagnosis, treatment)
Genetic and biometric data
Sexual orientation and lifestyle
🛡️ Key Components of DPDPA
Component | Description | Example |
Consent | Explicit patient consent for data collection and sharing | Consent form at signup |
Data Localization | Health data must be stored on Indian servers | AWS Mumbai region |
Right to Access | Patients must be able to access their data | User dashboard for health data |
Data Retention | Data can only be retained for the period necessary | Automatic cleanup policy |
Breach Notification | Data breaches must be reported within 72 hours | Email + SMS alert |
High-Level Architecture Overview
A HIPAA and DPDPA-compliant healthcare MVP must address both data security and infrastructure scalability:
1. Infrastructure Layers
Layer | Technology | Role |
Client Layer | React, React Native | User-facing app |
Application Layer | Django + Gunicorn | Business logic |
API Layer | Django REST Framework (DRF) | API exposure |
Database Layer | PostgreSQL | Secure data storage |
Storage Layer | AWS S3 + KMS | Secure file handling |
Queue Layer | Celery + Redis | Background processing |
Monitoring Layer | CloudWatch, Prometheus | System health and logging |
2. Data Flow Between Layers
Client --> Load Balancer;
Load Balancer --> Web Server;
Web Server --> Application Server;
Application Server --> API Layer;
API Layer --> Database;
Application Server --> Storage;
Storage --> Backup;
Monitoring --> Logs;
3. Secure Data Handling Process
Patient Data Collection: Collected through secure React forms.
Data Transmission: Sent to the backend over HTTPS using TLS 1.2+.
API Layer: Data is authenticated and validated using JWT.
Data Storage: Sensitive fields are encrypted at rest using
pgcrypto
.Data Retrieval: Access is controlled via Django’s Role-Based Access Control (RBAC).
Audit Trails: All data modifications are logged and stored securely.
✅ Backend Design
🔐 1. Authentication and Access Control
Use Django’s built-in User
model for granular permissions.
JWT authentication for secure login
Multi-factor authentication (MFA)
Example:
from rest_framework_simplejwt.tokens import RefreshToken
def get_tokens_for_user(user):
refresh = RefreshToken.for_user(user)
return {
'refresh': str(refresh),
'access': str(refresh.access_token)
}
🔐 2. Data Encryption
At Rest:
- Use PostgreSQL
pgcrypto
for field-level encryption
In Transit:
- Use TLS 1.2+ for all communications
🔐 3. Database Partitioning and Data Segmentation
Handling Protected Health Information (PHI) at scale requires careful database design to:
✅ Reduce query time for large datasets
✅ Minimize the impact of data breaches
✅ Improve scalability and retrieval performance
✅ Comply with legal requirements for PHI separation
Instead of storing all patient data in a single table or database, partition the data into:
PHI Partition: Data containing sensitive patient information (e.g., names, diagnoses, lab results)
Non-PHI Partition: Metadata and operational data (e.g., appointment IDs, timestamps, doctor names)
This allows for faster retrieval of non-sensitive data while securing sensitive data separately.
Example: PostgreSQL Partitioning
Partitioning sensitive and non-sensitive data in PostgreSQL:
CREATE TABLE patient_data (
id serial PRIMARY KEY,
name text NOT NULL,
dob date NOT NULL,
diagnosis text NOT NULL
) PARTITION BY RANGE (id);
CREATE TABLE patient_data_physical PARTITION OF patient_data
FOR VALUES FROM (1) TO (100000);
4. Row-Level Encryption
Use PostgreSQL's pgcrypto
to encrypt individual columns at the row level.
INSERT INTO patient_data (name, dob, diagnosis)
VALUES (
pgp_sym_encrypt('John Doe', 'encryption_key'),
pgp_sym_encrypt('1992-07-12', 'encryption_key'),
pgp_sym_encrypt('Anxiety', 'encryption_key')
);
5. Row-Level Security (RLS)
Row-Level Security allows you to control access to specific rows in a table based on the user’s role or group.
Example: Restrict doctors to only their patients' records:
ALTER TABLE patient_data ENABLE ROW LEVEL SECURITY;
CREATE POLICY patient_policy
ON patient_data
USING (current_user = user_id);
This ensures that a doctor accessing patient records can only retrieve data for patients they are authorized to treat.
🔐 6. Role-Based Access Control (RBAC)
Use Django's permissions
module to define user roles and granular permissions.
Example: Custom User Model in Django
from django.contrib.auth.models import AbstractUser
class CustomUser(AbstractUser):
is_doctor = models.BooleanField(default=False)
is_patient = models.BooleanField(default=False)
is_admin = models.BooleanField(default=False)
✅ Example Role-Based Permissions:
Role | Permissions | Example |
Doctor | Read and write patient data | View diagnosis, add notes |
Patient | Read only own data | View own diagnosis |
Receptionist | Modify appointment data | Schedule appointments |
Admin | Full permissions | Add or remove doctors |
7. Audit Logging
HIPAA requires that all data access and modification actions be logged for audit purposes.
Every login, logout, and data access event should be logged.
Audit logs should be tamper-proof and secured using hashing and encryption.
Example using Django's built-in logging:
import logging
logger = logging.getLogger(__name__)
def log_patient_access(patient_id, user):
logger.info(f"{user} accessed patient {patient_id}")
You can store logs in AWS CloudWatch or ELK Stack (Elasticsearch, Logstash, Kibana) for real-time monitoring.
8. Background Processing Using Celery
Heavy data processing should be offloaded to background workers to keep the main application responsive.
Appointment reminders
Generating reports
Processing payment notifications
Example Celery Task for Appointment Reminders:
from celery import shared_task
@shared_task
def send_appointment_reminder(appointment_id):
appointment = Appointment.objects.get(id=appointment_id)
# Send reminder via SMS or email
Add Celery to the Django settings.py
:
CELERY_BROKER_URL = 'redis://localhost:6379/0'
You can also use AWS SQS or RabbitMQ for more robust queuing in production.
Frontend Design
While most HIPAA and DPDPA requirements apply to the backend, the frontend also needs to be secure to prevent data leaks and client-side attacks.
✅ 1. Secure State Management
Use React’s useContext
and useState
for state management.
Avoid storing sensitive information (like tokens) in
localStorage
orsessionStorage
.Use
HttpOnly
andSecure
cookies for session storage.
Example using HttpOnly
cookies in Django:
SESSION_COOKIE_SECURE = True
SESSION_COOKIE_HTTPONLY = True
✅ 2. Prevent XSS (Cross-Site Scripting)
XSS attacks can expose sensitive patient data. Avoid using dangerouslySetInnerHTML
in React.
❌ Bad Example:
<div dangerouslySetInnerHTML={{ __html: userInput }} />
✅ Good Example:
import DOMPurify from 'dompurify';
<div>{DOMPurify.sanitize(userInput)}</div>
✅ 3. Prevent CSRF (Cross-Site Request Forgery)
Django includes built-in CSRF protection.
Backend (Django):
@csrf_exempt
def appointment(request):
if request.method == 'POST':
# Process request
Frontend (React):
const csrfToken = document.cookie.match(/csrftoken=([^;]*)/)[1];
fetch('/api/appointment/', {
method: 'POST',
headers: {
'X-CSRFToken': csrfToken
}
});
✅ 4. Secure API Calls
Use HTTPS for all API requests and include Authorization headers:
const response = await fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Authorization': `Bearer ${token}`
}
});
API Layer
The API layer acts as the interface between the frontend and the backend.
✅ 1. JWT Authentication
Use django-rest-framework-jwt
for secure token-based authentication.
from rest_framework_simplejwt.tokens import RefreshToken
def get_tokens_for_user(user):
refresh = RefreshToken.for_user(user)
return {
'refresh': str(refresh),
'access': str(refresh.access_token)
}
✅ 2. Rate Limiting
Use Django’s ratelimit
decorator to prevent DDoS and brute-force attacks:
from django_ratelimit.decorators import ratelimit
@ratelimit(key='ip', rate='5/m')
def appointment(request):
# Handle request
✅ 3. Pagination
Use Django’s built-in pagination to avoid large payloads in API responses:
from rest_framework.pagination import PageNumberPagination
class AppointmentPagination(PageNumberPagination):
page_size = 10
Storage Layer
Secure storage is critical when dealing with patient files (e.g., prescriptions, lab results).
✅ 1. Encrypt Files at Rest
Use AWS KMS (Key Management System) to encrypt files:
aws s3 cp myfile.txt s3://mybucket --sse aws:kms
✅ 2. Signed URLs for Secure File Access
Generate time-limited signed URLs:
import boto3
s3 = boto3.client('s3')
url = s3.generate_presigned_url(
'get_object',
Params={'Bucket': 'mybucket', 'Key': 'file.txt'},
ExpiresIn=3600
)
Monitoring and Compliance Reporting
Monitoring is critical for HIPAA and DPDPA compliance since both regulations require you to log and monitor data access, changes, and potential breaches. Effective monitoring helps you quickly identify and respond to security threats and ensures that you maintain an audit trail for future compliance audits.
✅ 1. Centralized Logging with AWS CloudWatch + ELK (Elasticsearch, Logstash, Kibana)
Centralized logging allows you to capture and analyze logs from multiple services in one place.
Use AWS CloudWatch to collect system and application logs.
Use the ELK Stack to store, query, and visualize logs.
Encrypt logs at rest and in transit using TLS.
Example AWS CloudWatch Configuration:
- Create a CloudWatch log group:
aws logs create-log-group --log-group-name /myapp
- Send logs to CloudWatch from Django:
import logging
import watchtower
logger = logging.getLogger(__name__)
logger.addHandler(watchtower.CloudWatchLogHandler(log_group="myapp"))
✅ 2. Compliance Audits and Data Access Reports
HIPAA and DPDPA require:
Regular security audits
Patient data access reports
User activity tracking
Breach detection reports
Example Django Admin-Based Reporting:
from django.contrib import admin
from .models import PatientAccessLog
class PatientAccessLogAdmin(admin.ModelAdmin):
list_display = ('user', 'patient', 'access_time')
list_filter = ('access_time', 'user')
Generate monthly reports using a management command:
from django.core.management.base import BaseCommand
from .models import PatientAccessLog
class Command(BaseCommand):
def handle(self, *args, **kwargs):
logs = PatientAccessLog.objects.all()
for log in logs:
print(f"{log.user} accessed {log.patient} at {log.access_time}")
✅ 3. Anomaly Detection and Intrusion Prevention
Use AWS GuardDuty and CloudWatch for real-time anomaly detection:
Unusual login attempts
Suspicious API activity
Failed login rates above the norm
Unauthorized access attempts
Example AWS GuardDuty Setup:
- Enable GuardDuty for your AWS account:
aws guardduty create-detector
- Set up a CloudWatch alert:
aws cloudwatch put-metric-alarm --alarm-name "Unusual Login Activity"
✅ 4. Real-Time Breach Response
HIPAA and DPDPA require breaches to be reported within specific timeframes:
HIPAA: Within 60 days
DPDPA: Within 72 hours
Use AWS SNS (Simple Notification Service) to automate breach alerts:
import boto3
sns = boto3.client('sns')
response = sns.publish(
TopicArn='arn:aws:sns:region:account-id:topic-name',
Message='Data breach detected',
Subject='Breach Notification'
)
You can also integrate Slack, PagerDuty, and email notifications for real-time breach response.
Deployment and Scaling
To build a production-grade healthcare MVP, you need a deployment pipeline that ensures:
✅ Rapid updates and bug fixes
✅ High availability under load
✅ Zero-downtime releases
✅ 1. Docker-Based Containerization
Use Docker to containerize the app for consistent deployment across environments:
Dockerfile:
FROM python:3.10-slim
WORKDIR /app
COPY . /app
RUN pip install -r requirements.txt
CMD ["gunicorn", "myapp.wsgi:application", "--bind", "0.0.0.0:8000"]
✅ 2. Kubernetes for Orchestration
Use Kubernetes to scale and manage the containerized app in production:
Example Kubernetes Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:latest
ports:
- containerPort: 8000
Horizontal Pod Autoscaler (HPA) – Automatically scale up or down based on CPU and memory usage.
Pod Disruption Budget – Ensure that at least one pod is always running during updates.
✅ 3. CI/CD Pipeline with GitLab or GitHub Actions
Set up a CI/CD pipeline to automate testing, security checks, and deployment.
GitLab .gitlab-ci.yml
Configuration:
stages:
- build
- test
- deploy
build:
script:
- docker build -t myapp .
- docker push registry.example.com/myapp
test:
script:
- pytest
deploy:
script:
- kubectl apply -f k8s/deployment.yaml
This pipeline will:
Build the Docker image
Run unit tests and integration tests
Deploy to Kubernetes if tests pass
Challenges and Solutions
1. Handling Sensitive Data at Scale
Problem: High volume of sensitive patient data increases the risk of breaches.
✅ Solution:
Partition PHI and non-PHI data
Encrypt data at rest and in transit
Enable row-level encryption
2. Data Retention and Deletion
Problem: HIPAA and DPDPA require you to delete patient data upon request.
✅ Solution:
Add an automated cleanup script
Use AWS lifecycle policies to delete backups automatically
Example Cleanup Script:
from django.utils.timezone import now
from .models import PatientData
def cleanup_patient_data():
expired_data = PatientData.objects.filter(last_accessed__lt=now() - timedelta(days=365))
expired_data.delete()
3. Cross-Border Data Storage
Problem: HIPAA requires data to remain in the US; DPDPA requires data to remain in India.
✅ Solution:
Store US patient data in AWS US regions
Store Indian patient data in AWS Mumbai region
Example AWS Region Configuration:
AWS_REGION = 'us-east-1' # US Data
AWS_REGION_INDIA = 'ap-south-1' # India Data
4. Multi-Tenant Access Control
Problem: A single application serving multiple clinics must isolate patient data.
✅ Solution:
Use Django’s
TenantModel
for multi-tenancyDefine a tenant at the database level
Example TenantModel:
from django_tenants.models import TenantMixin
class Clinic(TenantMixin):
name = models.CharField(max_length=100)
created_on = models.DateTimeField(auto_now_add=True)
Best Practices for Real-World Compliance
Best Practice | Implementation | Benefit |
Encrypt data at rest and in transit | PostgreSQL pgcrypto + TLS | Data security |
Enable Row-Level Security | Django's RLS module | Fine-grained access control |
Use MFA for Authentication | AWS Cognito or Django MFA | Increased login security |
Use Signed URLs for File Access | AWS S3 Signed URLs | Temporary secure access |
Set API Rate Limits | Django Ratelimit | Prevent DDoS attacks |
Enable Centralized Logging | AWS CloudWatch + ELK | Centralized audit trail |
Partition PHI and non-PHI Data | PostgreSQL Partitioning | Better query performance |
Data Localization | AWS Mumbai for Indian data | DPDPA compliance |
Conclusion
Building a HIPAA and DPDPA-compliant healthcare MVP is complex but achievable with the right architecture and security measures. By implementing encryption, secure data partitioning, role-based access control, logging, and monitoring, you can create a product that meets regulatory requirements while maintaining scalability and performance.
Subscribe to my newsletter
Read articles from Ahmad W Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
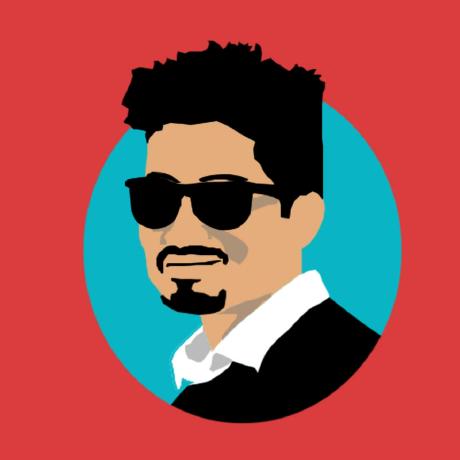