Getting Started with Numpy

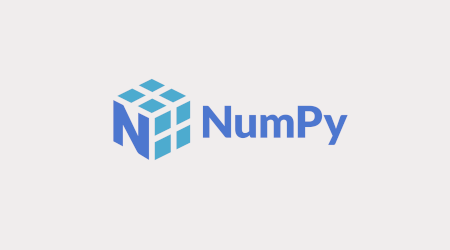
Numpy (Numerical Python) is a powerful Python library used for numerical computing. It provides fast, flexible and efficient operations on large multi-dimensional arrays and matrices, along with mathematical functions to operate on these data structures.
As always, I will answer the question: Why? Why should we learn NumPy in our machine learning journey instead of simply using Python lists?
Think of Python lists as a notebook where you manually perform calculations—it's possible, but slow and inefficient. NumPy, on the other hand, is like a supercomputer—it makes everything faster, easier, and more memory-efficient. It’s your best friend in this ML journey!
Numpy arrays are way faster than Python lists because they use contiguous memory storage and are implemented in C(which is super fast).
Python lists store each element separately as an object, which means that every number in a list comes with additional metadata(like type info, references,etc.)This extra information takes up extra memory.Numpy arrays on the other hand, store data in a single contiguous block of memory ,making it more compact and efficient.
With Python lists, if we want to multiply every element by 2, we need a loop. Numpy does it in one line! Numpy does operations on entire arrays at once(vectorization), making our code faster and cleaner.Also Numpy can do complex math easily.
Before we dive into the more advanced features like broadcasting, let’s get comfortable with some basic NumPy operations.
Now that we have explored the basics, let’s move on to one of NumPy’s most powerful features—broadcasting.
Broadcasting allows NumPy to perform element-wise operations on arrays of different shapes without creating unnecessary copies of data. This makes operations faster and memory-efficient.
Imagine you want to add a single number to every element in an array. Traditionally, you would need a loop to iterate through each element and apply the operation. However, NumPy eliminates that extra effort! Broadcasting allows NumPy to handle operations between arrays of different shapes effortlessly, automatically expanding the smaller array to match the larger one.
It has some basic rules:
If two arrays have the same shape,element-wise operations happen normally.
If shapes are different, NumPy “stretches“ the smaller array so they match.
Dimension size of 1 can be stretched to match the other array.
Let’s take a look at how broadcasting works in action.
Now that we understand broadcasting, let’s talk about something equally important—indexing and slicing in NumPy arrays.
Just like with Python lists, we can access specific elements in a NumPy array using indexing. However, NumPy takes it a step further by allowing more advanced slicing techniques that make it easier to work with large datasets.
With slicing, we can extract specific portions of an array without writing complex loops. Whether it’s selecting a single value, a range of elements, or even modifying specific parts of an array, NumPy makes it incredibly efficient.
Let’s see how indexing and slicing work in NumPy!
Now that we know how to access and manipulate specific elements of a NumPy array, let’s move on to something even more exciting—arithmetic operations!
Now that we’ve explored arithmetic operations, let’s dive into another essential aspect of NumPy—aggregation functions and basic statistical operations.
When working with large datasets, we often need to quickly compute values like the sum, mean, maximum, or minimum. NumPy provides built-in functions that make these calculations fast and efficient, eliminating the need for manual loops.
With just a single function call, we can find the sum of all elements, the average, the standard deviation, and more.
These operations are crucial in data analysis and machine learning, where summarizing data efficiently is key.
Let’s take a look at how NumPy handles aggregation and statistical operations!
Now that we’ve seen how NumPy handles aggregation and statistical functions, let’s explore another powerful feature—element-wise operations.
We’ve covered a lot about NumPy’s capabilities, but before we wrap up, let’s explore one of its most powerful features—matrix operations and linear algebra functions.
NumPy provides a dedicated submodule called linalg
that makes working with matrices and performing complex linear algebra calculations incredibly easy. Whether it’s matrix multiplication, finding the determinant, computing eigenvalues, or solving linear equations, NumPy does it all efficiently.
These operations are widely used in machine learning, data science, and scientific computing, making them essential tools for anyone working with numerical data.
Let’s check out some of the built-in linear algebra functions in NumPy
And that’s it! This blog was just me sharing what I’ve learned about NumPy so far, and I hope it helped you in some way. I’m definitely not a pro, just someone exploring and trying to understand things better.
If you have any feedback or suggestions, or if I got something wrong, I’d love to hear from you! Learning is always more fun when it’s a two-way conversation. So feel free to share your thoughts!
Let’s keep learning together.
Subscribe to my newsletter
Read articles from Sruti Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sruti Mishra
Sruti Mishra
Tech enthusiast on a journey to master AI/ML|MCA student| Documenting my learnings, challenges, and breakthroughs—one line of code at a time!