Day 15: JavaScript Fetch API - Handling HTTP Requests with Ease ✨

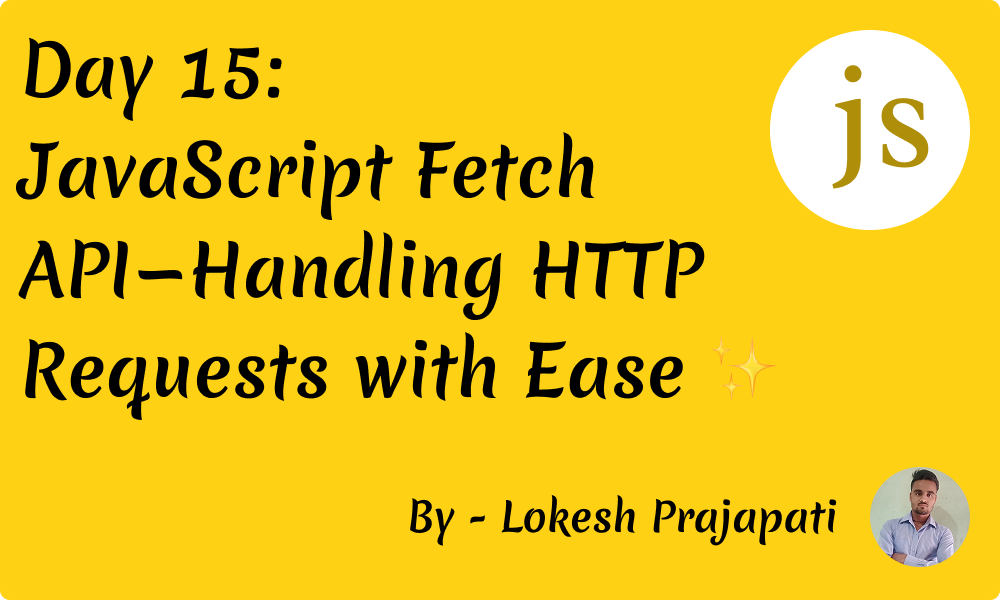
The Fetch API is a powerful and modern way to make HTTP requests in JavaScript. Whether you’re fetching data from a public API, submitting form data, or handling RESTful endpoints, the Fetch API makes these tasks straightforward. 🌐
In this post, we’ll explore the Fetch API in detail, including: ✅ Basic Syntax ✅ GET & POST Requests ✅ Error Handling ✅ Practical Examples with Real-World Code
Let’s dive in! 🚀
1. What is the Fetch API? 🤔
The Fetch API is a modern interface that allows you to make network requests in JavaScript. It is based on Promises, making it cleaner and easier to manage asynchronous data fetching compared to older methods like XMLHttpRequest
.
Basic Syntax:
fetch(url, options)
.then(response => response.json()) // Parsing JSON data
.then(data => console.log(data)) // Handling the data
.catch(error => console.error('Error:', error));
2. Performing a GET Request 🔎
A GET request is used to fetch data from an API endpoint.
Example: Fetching User Data
fetch('https://jsonplaceholder.typicode.com/users/1')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP Error! Status: ${response.status}`);
}
return response.json();
})
.then(data => console.log('User Data:', data))
.catch(error => console.error('Error fetching data:', error));
✅ response.ok
ensures successful response handling.
✅ Always use .catch()
to handle errors gracefully. 🔥
3. Performing a POST Request 📤
A POST request is used to send data to an API endpoint.
Example: Sending Data with POST
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
title: 'JavaScript Fetch API',
body: 'Learning fetch is amazing! 🚀',
userId: 1
})
})
.then(response => response.json())
.then(data => console.log('New Post:', data))
.catch(error => console.error('Error posting data:', error));
✅ Include headers like Content-Type
to define the data format.
✅ Always use .json()
to parse the JSON response. 💪
4. Handling Errors Gracefully ⚠️
Proper error handling is crucial for a smooth user experience.
Example with Error Handling:
async function fetchData() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/users/1000');
if (!response.ok) {
throw new Error(`Network Error: ${response.status}`);
}
const data = await response.json();
console.log('User Data:', data);
} catch (error) {
console.error('Error fetching data:', error.message);
}
}
fetchData();
✅ try...catch
helps you manage errors effectively. ✅ Display meaningful error messages for better debugging. 🐞
5. Using Async/Await for Cleaner Code ✨
async/await
provides a cleaner and more readable syntax for handling asynchronous tasks with Fetch API.
Example: Fetching Data with Async/Await
async function getPosts() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const posts = await response.json();
console.log('Posts:', posts);
} catch (error) {
console.error('Failed to fetch posts:', error);
}
}
getPosts();
✅ Cleaner syntax with async/await
makes code easier to maintain.
✅ Less nesting compared to .then()
chaining. 😎
6. Real-Life Example: Fetching Weather Data 🌦️
Let’s combine everything to create a practical example of fetching weather data from an API.
Example: Weather App
async function getWeather(city) {
const apiKey = 'your_api_key_here';
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`;
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error('City not found!');
}
const data = await response.json();
console.log(`Weather in ${city}:`, data.weather[0].description);
} catch (error) {
console.error('Error:', error.message);
}
}
getWeather('London');
✅ Fetch live weather data dynamically.
✅ Perfect example of integrating real APIs into your projects. 🌍
7. Best Practices for Using Fetch API 🧠
✅ Always handle network errors using .catch()
or try...catch
.
✅ Use async/await
for better readability in complex logic.
✅ Validate data before displaying it to users.
✅ Implement timeout logic to avoid indefinite loading states.
✅ Use meaningful error messages for improved debugging. ⚙️
Conclusion 🎯
The Fetch API is a powerful tool for handling HTTP requests in JavaScript. With its clean syntax, promise-based structure, and compatibility with async/await
, it's the go-to choice for modern web developers.
Mastering the Fetch API is crucial for building interactive web applications that communicate with servers efficiently. 🚀
Have you faced challenges while using the Fetch API? Share your thoughts and experiences in the comments below! 😊
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode