Testing Before Production: The Smart Path to Bug-Free GraphQL APIs
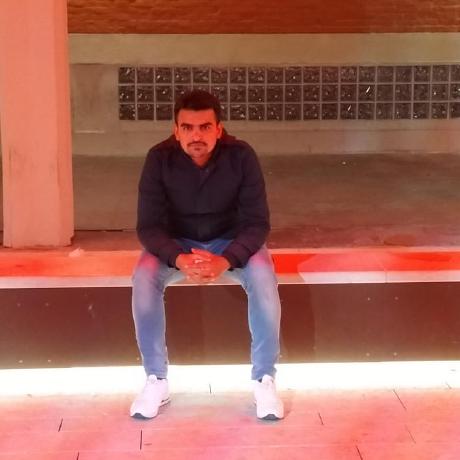

Testing is a fundamental aspect of software development, ensuring that your GraphQL API works as intended and preventing regression bugs. In this tutorial, we’ll walk through the process of writing tests for a GraphQL API built with Go. We’ll cover everything from setting up a test environment to verifying query and mutation responses. By the end, you should have a strong understanding of how to test your GraphQL APIs effectively.
Prerequisites
Before diving into writing tests, ensure you have the following:
Golang installed on your system. Download Go if you haven’t already.
A GraphQL API built using a library such as graphql-go or gqlgen.
Familiarity with GraphQL and Go testing practices.
Step 1: Setting Up the Test Environment
First, create a dedicated folder for your tests, such as api_test
. Make sure your test files end with _test.go
as required by Go’s testing tools.
Next, import the necessary packages:
package api_test
import (
"bytes"
"net/http"
"net/http/httptest"
"testing"
"encoding/json"
"github.com/stretchr/testify/assert"
"your_project_path/handler" // Replace with your handler package
)
Here’s a breakdown:
net/http/httptest
is used to create a mock HTTP server for testing.github.com/stretchr/testify/assert
simplifies assertions in tests.Replace
your_project_path/handler
with the path to your GraphQL handler.
Step 2: Writing a Basic Test for Queries
Test Setup
Let’s assume you have a GraphQL query for fetching a user:
query {
user(id: "1") {
id
name
}
}
To test this query, write the following test:
func TestUserQuery(t *testing.T) {
// Define the query
query := `{"query": "query { user(id: \"1\") { id name } }"}`
// Create a request
req, err := http.NewRequest("POST", "/graphql", bytes.NewBufferString(query))
if err != nil {
t.Fatalf("Failed to create request: %v", err)
}
req.Header.Set("Content-Type", "application/json")
// Mock server response
rr := httptest.NewRecorder()
handler := handler.GraphQLHandler() // Replace with your GraphQL handler function
handler.ServeHTTP(rr, req)
// Validate response
assert.Equal(t, http.StatusOK, rr.Code, "Expected status code to be 200")
var response map[string]interface{}
if err := json.Unmarshal(rr.Body.Bytes(), &response); err != nil {
t.Fatalf("Failed to parse response: %v", err)
}
expected := map[string]interface{}{
"data": map[string]interface{}{
"user": map[string]interface{}{
"id": "1",
"name": "Ahmed Dev",
},
},
}
assert.Equal(t, expected, response)
}
Step 3: Writing Tests for Mutations
Let’s test a mutation for creating a user:
mutation {
createUser(input: {name: "Ahmed"}) {
id
name
}
}
The corresponding test:
func TestCreateUserMutation(t *testing.T) {
// Define the mutation
mutation := `{"query": "mutation { createUser(input: {name: \"Ahmed\"}) { id name } }"}`
// Create a request
req, err := http.NewRequest("POST", "/graphql", bytes.NewBufferString(mutation))
if err != nil {
t.Fatalf("Failed to create request: %v", err)
}
req.Header.Set("Content-Type", "application/json")
// Mock server response
rr := httptest.NewRecorder()
handler := handler.GraphQLHandler()
handler.ServeHTTP(rr, req)
// Validate response
assert.Equal(t, http.StatusOK, rr.Code)
var response map[string]interface{}
if err := json.Unmarshal(rr.Body.Bytes(), &response); err != nil {
t.Fatalf("Failed to parse response: %v", err)
}
data := response["data"].(map[string]interface{})
createdUser := data["createUser"].(map[string]interface{})
assert.Equal(t, "Ahmed", createdUser["name"])
assert.NotEmpty(t, createdUser["id"])
}
Step 4: Mocking Dependencies
In most cases, your GraphQL API will interact with databases or external APIs. Use mocking libraries like gomock to isolate and test your logic.
For example, to mock a database call in a resolver:
Define an interface for the dependency.
Use
gomock
to generate a mock implementation.Inject the mock into your resolver during testing.
Step 5: Running Your Tests
Run your tests using:
go test ./...
This command recursively runs all test files in your project.
Best Practices for Testing GraphQL APIs
Test All Scenarios: Cover edge cases, error responses, and null values.
Use Fixtures: Store example responses to simplify assertions.
Automate: Integrate your tests into CI/CD pipelines for continuous validation.
Documentation and Further Reading
By following these steps and best practices, you can confidently write and maintain robust tests for your GraphQL APIs in Go.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
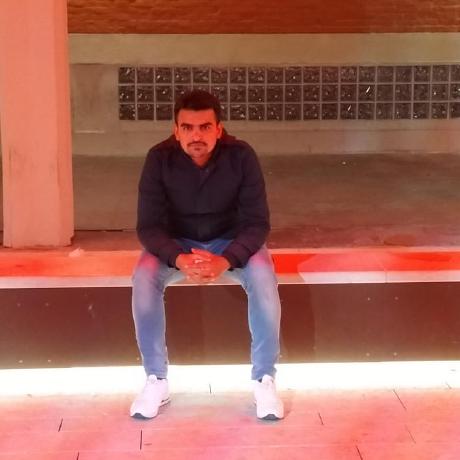
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.