JavaScript Date() and Commonly Used Methods

Table of contents
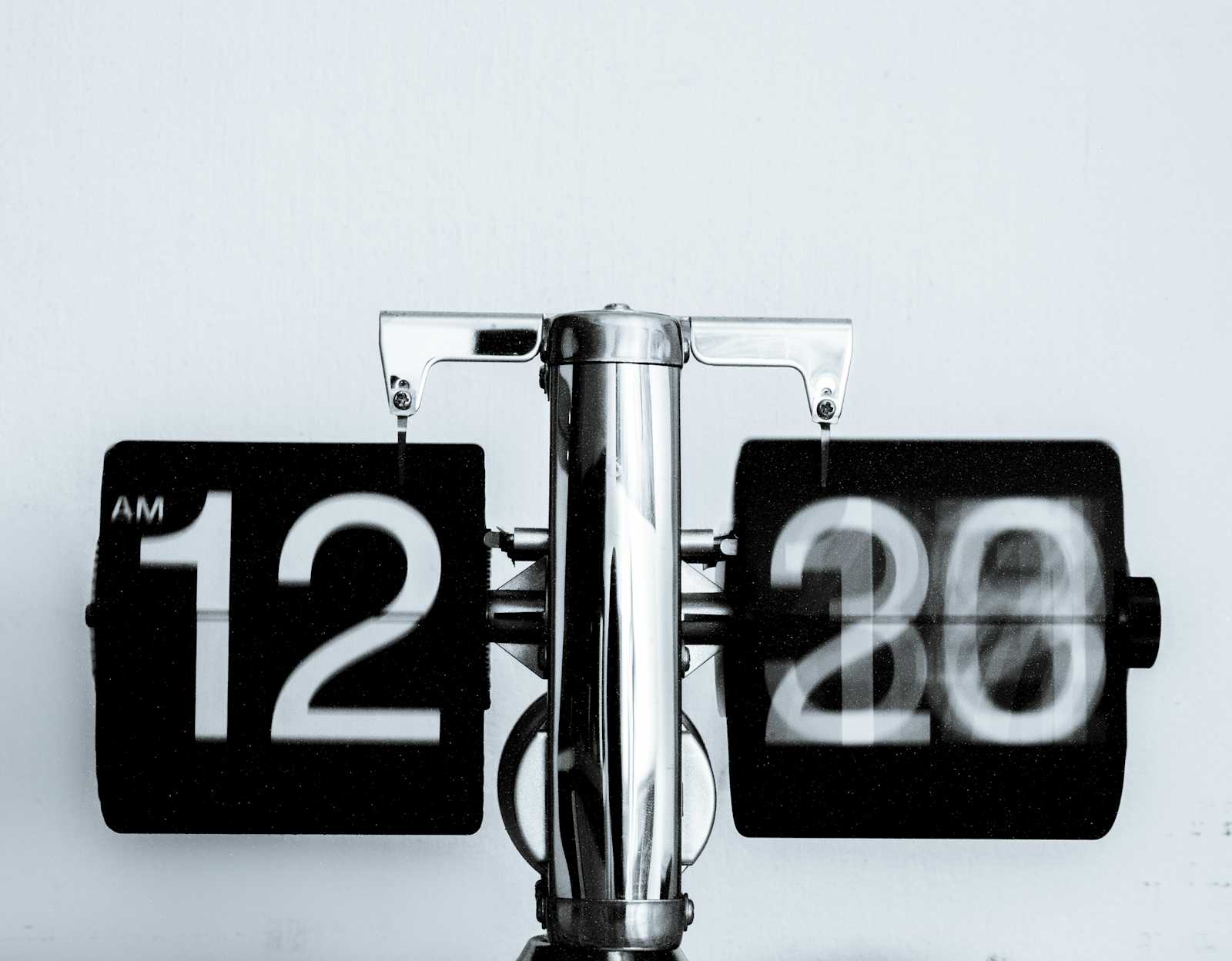
JavaScript provides the Date
object to work with dates and times. You can create a new date using:
let currentDate = new Date();
console.log(currentDate);
This gives the current date and time.
Common Date
Methods Used in Production
Getting Date and Time
getFullYear()
: Returns the year.getMonth()
: Returns the month (0-11, where 0 is January).getDate()
: Returns the day of the month (1-31).getDay()
: Returns the day of the week (0-6, where 0 is Sunday).getHours()
,getMinutes()
,getSeconds()
: Get the respective time values.
let today = new Date();
console.log(today.getFullYear()); // Example: 2025
console.log(today.getMonth()); // Example: 2 (March)
console.log(today.getDate()); // Example: 11
Formatting Dates
toDateString()
: Returns a readable date string.toISOString()
: Returns a date inYYYY-MM-DDTHH:mm:ss.sssZ
format (useful in APIs).toLocaleDateString()
: Formats date based on the user's locale.
console.log(today.toDateString()); // Example: "Tue Mar 11 2025"
console.log(today.toISOString()); // Example: "2025-03-11T14:30:00.000Z"
console.log(today.toLocaleDateString()); // Example: "3/11/2025" (based on locale)
Setting Date and Time
setFullYear(year)
,setMonth(month)
,setDate(day)
: Set date values.
let customDate = new Date();
customDate.setFullYear(2026);
customDate.setMonth(5); // June (0-based)
console.log(customDate);
Time Difference (Timestamps)
getTime()
: Returns milliseconds since January 1, 1970 (useful for time calculations).
let timestamp = today.getTime();
console.log(timestamp); // Example: 1741557600000
Conclusion
JavaScript Date()
is powerful for handling dates and times in web applications. The above methods help in fetching, formatting, and manipulating dates effectively.
Subscribe to my newsletter
Read articles from Dikshant Koriwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dikshant Koriwar
Dikshant Koriwar
Hi, I'm Dikshant – a passionate developer with a knack for transforming ideas into robust, scalable applications. I thrive on crafting clean, efficient code and solving challenging problems with innovative solutions. Whether I'm diving into the latest frameworks, optimizing performance, or contributing to open source, I'm constantly pushing the boundaries of what's possible with technology. On Hashnode, I share my journey, insights, and the occasional coding hack—all fueled by curiosity and a love for continuous learning. When I'm not immersed in code, you'll likely find me exploring new tech trends, tinkering with side projects, or simply enjoying a great cup of coffee. Let's connect, collaborate, and build something amazing—one line of code at a time!