How To Efficiently Scrape CoinMarketCap Data

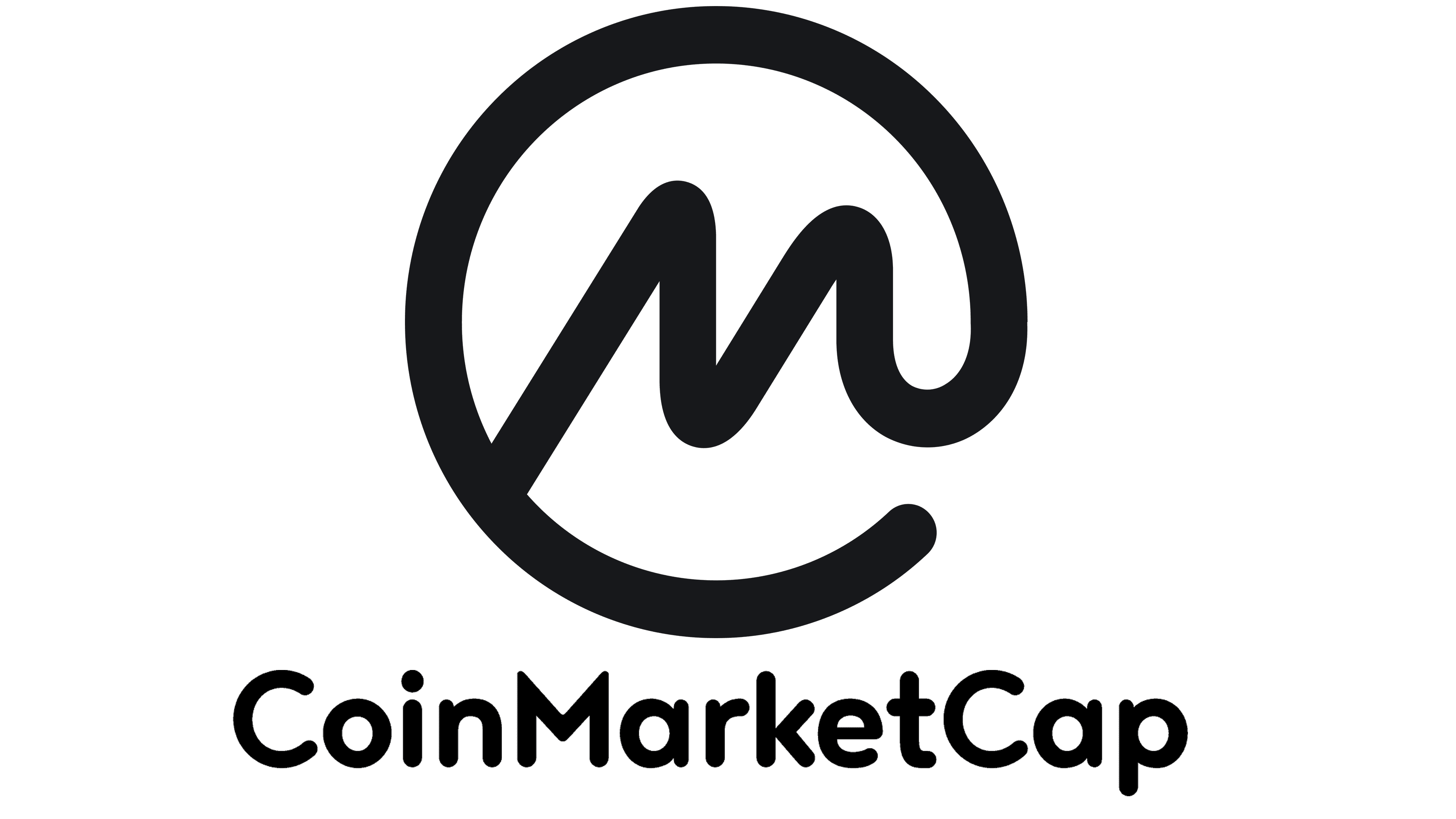
CoinMarketCap is one of the largest cryptocurrency data aggregators, which gives real-time price data, historical data, market capitalization, and other essential information for investors and traders. If you wish to scrape data from CoinMarketCap with efficiency, one of the most suitable methods is web scraping.
In this tutorial, we're going to take you through various methods of scraping CoinMarketCap data with Python, APIs, and web scraping libraries while maintaining compatibility with ethical and legal guidelines.
Why Scrape CoinMarketCap Data?
Before diving into the technical details, let’s explore why scraping CoinMarketCap data can be useful:
Market Analysis – Track cryptocurrency trends and market movements.
Price Monitoring – Get real-time price updates for trading strategies.
Data Visualization – Use the extracted data for charts, dashboards, and reports.
Historical Data Collection – Backtest trading strategies using historical price data.
Portfolio Management – Automate portfolio tracking based on real-time data.
Method 1: Using the CoinMarketCap API (Recommended)
The simplest and most effective means of extracting CoinMarketCap information is through the use of their official CoinMarketCap API. This provides for safe and orderly access to crypto data without breach of terms of service.
Step 1: Get an API Key
Sign up at CoinMarketCap Developers Portal.
Choose a pricing plan (they offer free and paid options).
Copy your API Key after registration.
Step 2: Install Required Libraries
To interact with the API using Python, install the requests
library if you haven't already:
pip install requests
Step 3: Fetch Data Using Python
Use the following Python script to fetch real-time cryptocurrency data:
import requests
API_KEY = "your_api_key_here"
url = "https://pro-api.coinmarketcap.com/v1/cryptocurrency/listings/latest"
headers = {
"Accepts": "application/json",
"X-CMC_PRO_API_KEY": API_KEY,
}
response = requests.get(url, headers=headers)
data = response.json()
# Print the top 5 cryptocurrencies
for coin in data["data"][:5]:
print(f"{coin['name']} ({coin['symbol']}): ${coin['quote']['USD']['price']:.2f}")
Method 2: Web Scraping CoinMarketCap (Alternative Approach)
If you prefer web scraping instead of using the API, you can extract data directly from the CoinMarketCap website using BeautifulSoup and Selenium.
Step 1: Install Required Libraries
pip install requests beautifulsoup4 selenium
Step 2: Scrape CoinMarketCap with BeautifulSoup
import requests
from bs4 import BeautifulSoup
url = "https://coinmarketcap.com/"
headers = {"User-Agent": "Mozilla/5.0"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract cryptocurrency names and prices
table = soup.find_all('tr')[1:6] # First 5 rows
for row in table:
columns = row.find_all('td')
name = columns[2].text.strip()
price = columns[3].text.strip()
print(f"{name}: {price}")
Step 3: Handling JavaScript-Rendered Content
Since CoinMarketCap uses JavaScript to load data dynamically, you may need Selenium to extract complete data:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
from webdriver_manager.chrome import ChromeDriverManager
options = Options()
options.add_argument("--headless")
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
driver.get("https://coinmarketcap.com/")
page_source = driver.page_source
driver.quit()
soup = BeautifulSoup(page_source, 'html.parser')
print(soup.prettify()) # View the extracted HTML content
Ethical Considerations and Best Practices
While web scraping can be useful, always follow best practices to avoid legal and ethical issues:
Use APIs whenever possible – The CoinMarketCap API is the best method for structured data extraction.
Respect robots.txt
– Check CoinMarketCap’s robots.txt file to see scraping permissions.
Avoid Overloading Servers – Use delays between requests to prevent overwhelming the website.
Comply with Terms of Service – Always ensure that your scraping activities comply with the website’s terms.
Conclusion
Web scraping CoinMarketCap data can be very useful for developers, analysts, and traders. The ideal method is to utilize the CoinMarketCap API for structured and reliable access to data. Nevertheless, if you want to use web scraping, BeautifulSoup and Selenium can be utilized to scrape data from the website directly.
By adhering to the best practices in this guide, you can effectively collect cryptocurrency data for market analysis, portfolio management, or trading strategies.
Do you have any questions or need more advice? Leave a comment below or share this guide with others in the crypto space!
Happy scraping!
Know More >> https://scrapelead.io/crypto-and-stock/coinmarketcap-web-data-scraper/
Subscribe to my newsletter
Read articles from ScrapeLead directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ScrapeLead
ScrapeLead
Scrape Any Website and Connect With Your Popular Apps It’s easy to connect your data to thousands of apps, including Google Sheets and Airtable. You can utilize Zapier, http://scrapelead.io’s API, and more for smooth data sharing and integration across multiple platforms.