Functions in JAVASCRIPT

Table of contents
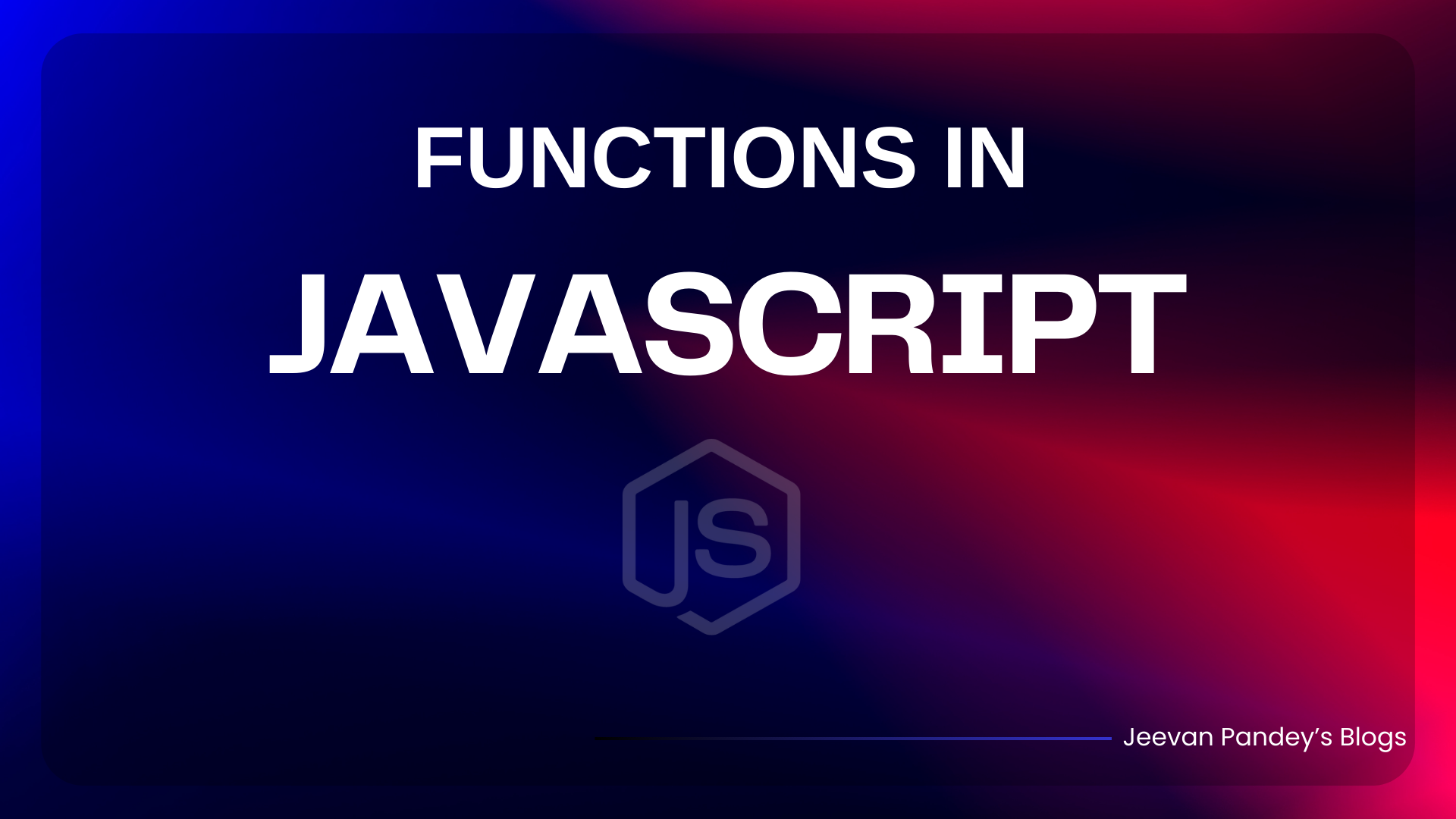
Introduction
Well Functions… As its name Suggests, it helps in Performing Various Tasks in JavaScript. It used as a block of code to Perform any Specific Task.
→ Eg - Doing Some Calculations, Validating some forms before submitting it and many more…
So, In this Blog we will explore Functions , Types of Functions and understand their usage with the help of some code samples.
Functions
Functions are known as one of the fundamental building block of JavaScript.
As it allows us to reuse our same code multiple times by reducing code duplication.
They helps in breakdown the complex programs into smaller units, which makes it easier to understand the written code.
They also create their own Scope.
Fun Fact : Functions are known as the First-class citizens in JavaScript .
Have u wonder why? Let’s Understand :
They often termed as the first-class citizens in JavaScript as :
They can be Assigned to variables
Returned from other Functions
Can be stored in Data Structures
Types of Functions
Function Declaration
The most common way which widely used among geeks is Function Declaration. It has a very simple syntax, lets take a look —
function greet(name) { // In this we have defined a function named 'greet' which accepts a parameter 'name' in this case.
console.log(`Hello ${name}!`);
} // This function is using a Template literal to log a greeting to the console.
greet('Jeevan Pandey')
// In order to call the function(which named 'greet') we will be needed to give a argument for the parameter 'name'.
Output —
Hello Jeevan Pandey!
—> Let’s Understand the above code
Inside function it starts with the ‘ function ’ keyword which followed by ‘ name ‘ , followed by ( )
( ) → these parentheses includes the Parameters which can be Separated by commas
And, the code which will be executed is Placed inside the { } Curly braces.
And this function will be executed when we invokes or ( Calls ) function.
‘ Return ‘ → it actually worked as a break in a code when the Code reaches return statement, the function stops executing.
function Sum(Num1 , Num2){ return Num1 + Num2; //return statement } console.log(Sum(4,5));
Output —
9 //Output
Function Expression
Just like Function Declaration, Function Expression is another way to create a function in JavaScript , where the function is assigned to a Variable which eventually used to call the function.
Let’s take a look to the Syntax —
const myfunction = function(parameters){
// function body
return value;
}
—> Let’s Understand the above code
Here, we have assigned an anonymous function to a variable named ‘ myfunction ‘ .
It accepts some parameters and returns value .
And, to call this function, we can provide any argument to ‘ myfunction ‘ .
const Sum = function(Num1 , Num2){
return Num1 + Num2; //return statement
}
console.log(Sum(4,5));
//It's very similar to function declaration but the difference is here the functions assigned to the variable.
Output —
9 //Output
Difference Between Function Declaration and Function Expression
Here You Might think - Okay, So Is this the Only difference between Function Declaration and Function Expression?
→ The Simple Answer is No.
Function Expressions Cannot be Used before the Initialisation which means they are not Hoisted.
let’s understand with example —
→ Let’s see an example using Function Declaration —
//Function Declaration
const result = sum(20, 50)
console.log(result)
function sum(num1, num2) {
return num1 + num2
}
Output —
70 //Output
What happens here is Hoisting as we have called the function before initialising the function and the code is running properly.
→ Let’s see an example using Function Expression —
//Function Expression
const result = sum(20, 50)
console.log(result)
const sum = function(num1, num2) {
return num1 + num2
}
Output —
ReferenceError: Cannot access 'sum' before initialization
//Output Reference Error
In the case of Expression function we got the error it means they are not Hoisted.
Arrow Function
Arrow function first Introduced in ES6. They are used to write shorter function as it provides a more concise syntax.
They are very much similar to function expression but here we use ‘ => ‘ symbol to separate the function parameters and the function body.
Let’s have a look how we write Arrow Function —
const name = (parameters) => {function body}
const greetingUser = (userName) => {
console.log(`Hello ${userName}!, How are you?`)
}
greetingUser("Jeevan pandey")
Output —
Hello Jeevan pandey!, How are you? //Output
—> Let’s Understand the above code
In the above example, we have defined an arrow function called ‘ greetingUser ‘ that accepts parameter ‘ userName ‘ .
To call above function, we simply provide an argument for parameter and log it to the console.
Some more example —
const add = (a,b) => {
return a + b;
}
console.log(add(5,4));
Output —
9 //Output
Immediately Invoked Function Expression (IIFE)
Immediately Invoked Function Expression (IIFE) is a type of function which runs as soon as it is defined.
It also very much similar to Function Expression as it also assigned to a Variable.
→ We need IIFE to avoid pollutions for Global namespace as it creates their own new scope.
Let’s have a look at how to we write IIFE —
(function() {
// Function body
console.log("Chai aur Code");
})();
—> Let’s Understand the above code
The above written code is basically divided in to two sets -
(function() { Function Body... })
- This part defines the function expression. () → Parentheses are very important as they are telling the JavaScript to treat the code as expression not declaration.
()
- This part helps in Immediately Invocation of the code.
Let’s write some code for the same —
(function add(a,b) {
console.log(a+b);
})(4,5)
Output —
9 //Output
Callback Function
Callback function passed as an argument to another function. It allows us to execute code once a particular operation has been done.
Let’s take a look how we use callback functions —
function mainFunction(parameter) {
// Some code inside mainFunction
// ...
parameter(); // Execute the parameter function
}
function callbackFunction() {
// Code to be executed later
console.log("Callback function executed!");
}
mainFunction(callbackFunction); // Passing callbackFunction as an argument
—> Let’s Understand the above code
In the above syntax we have declared a function named mainFunction that recieves the parameter called ‘ parameter ‘.
This parameter is like a placeholder for the function named ‘ callbackFunction ‘ .
Inside the mainFunction , parameter() is used to execute the function.
Now, the callbackFunction is the function that will be executed later.
Inside the callbackFunction we have logged some code to the console. at the end we can see the function has been called.
Here is one more example —
function greet(userName) {
console.log(`Hello! I am ${userName}`);
}
function greetingNow(callback) {
const userName = "Jeevan Pandey"
callback(userName);
}
greetingNow(greet);
Output —
Hello! I am Jeevan Pandey //Output
Conclusion
Here, In this Blog We have Learned about JavaScript Functions, How they can be written and what are the powers they actually carry. we also learned Different types of Functions which we use . I hope you guys will be taking some notes from the article.
—> In the Next Article on Functions We will take some more deep dives and will learn about Generator Functions and Async/Await Functions……
Want to Learn More?
If Yes…. You Can checkout my other works on https://jeevanpandey-dev.hashnode.dev/ and can connect with me on — Twitter / X
Subscribe to my newsletter
Read articles from Jeevan Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
