The Ultimate Guide to Web Application Security: Types of Attacks, Prevention Techniques, and Best Practices

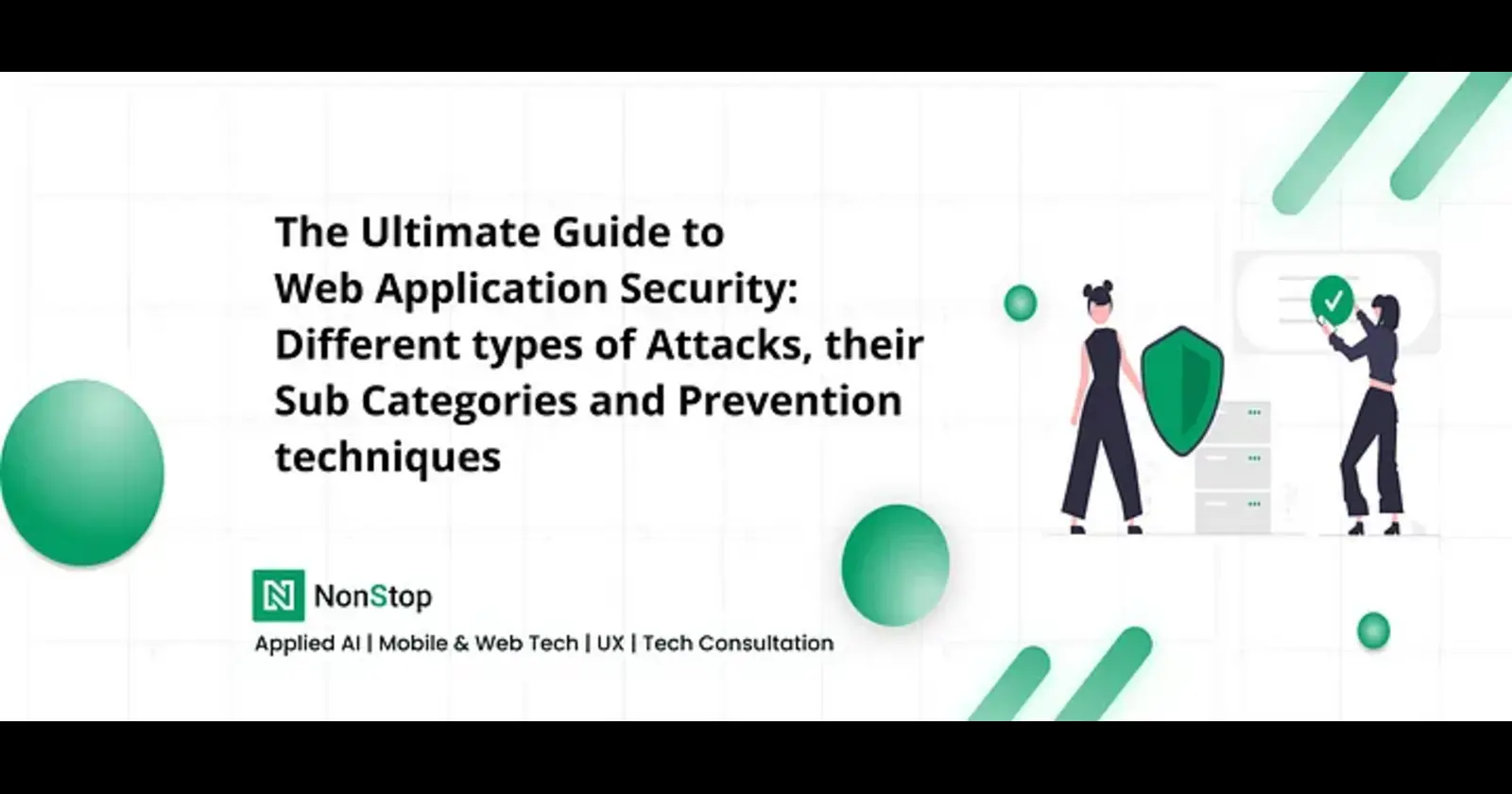
In today’s digital world, everything from your finances to your medical history is online. Large servers hold this data and it is easily accessible to anyone with ill intentions to exploit it unless you have secured it well.
So as a software engineer, how can you ensure the website you are working on will not be the culprit of losing such critical data? Curious? Keep reading on..
To properly safeguard data linked to our web applications, we need to first understand what the 8 different types of web attacks are and different ways we can adopt to protect our apps from them, starting from:
Different types of Web Security Attacks possible on your Website
1. Input Validation and Injection Attacks
These attacks exploit how a web application handles untrusted input, aiming to manipulate queries or execute malicious commands. It has 3 sub-types:
a. SQL Injection Attack
b. Command Injection Attack
c. No-SQL Injection Attack
a. SQL Injection Attack: Occurs when an attacker manipulates an application’s SQL query to execute unintended commands. Say, in
SELECT * FROM users WHERE email = 'email-input' AND password = 'pass-input';
the user puts email-input = ‘ OR ‘1’=’1 then, the result returns all values as the where condition will always return true.
PREVENTION: Use parameterized queries, avoid string interpolation in SQL, validate and sanitize inputs.
b. Command Injection Attack: Allows an attacker to execute arbitrary system commands on the server. Like:
system("ping #{params[:host]}")
if the user puts params[:host] = “127.0.0.1; rm -rf /” then, it could delete all server files.
PREVENTION: Avoid using system commands with untrusted input, use libraries like Open3
that separate input from commands.
c. NoSQL Injection Attack: Targets NoSQL databases (e.g., MongoDB) by injecting malicious queries. Attacker can use the following command to bypass authentication.
User.where({"$where" => "this.name == 'admin' || true"})
PREVENTION: Use ORMs like ‘Mongoid’ for parameterized queries.
Authentication and Authorization attacks prevention for your system
2. Authentication and Authorization Attacks
These attacks target the mechanisms that verify and authorize users. It has 3 sub-types:
a. Brute Force Attacks
b. Session Hijacking
c. Privilege Escalation
a. Brute Force Attacks: Attacker attempts to guess passwords using repeated attempts. For example, attacker tries all combinations of password for an existing user in your application.
PREVENTION: rate-limit login attempts using rack-attack
, lock accounts after several failed attempts, use CAPTCHA for repeated failures.
b. Session Hijacking: Attackers steal session tokens (via XSS or sniffing) to impersonate users. Say a genuine user has signed into your app and the attacker steals the session token from headers to get direct access into the application using the genuine user’s account.
PREVENTION: Use HTTPS and secure HttpOnly cookies, regenerate session IDs after login, invalidate sessions on logout or password change.
c. Privilege Escalation: Attackers gain access to higher privilege levels, often by manipulating roles. Say, the ‘Admin Dashboard’ to your application is supposed to be only visible to admins but an attacker with a compromised normal user’s access tries to call an admin only API and gets the data.
PREVENTION: Enforce role-based access control (e.g., Pundit, CanCanCan), Validate user permissions at the controller and model level.
Cross Site Scripting attacks and their prevention
3. Cross-Site Attacks
These attacks involve manipulating or exploiting web requests and responses. It has 2 sub-types:
a. Cross-Site Scripting (XSS)
b. Cross-Site Request Forgery (CSRF)
a. Cross-Site Scripting (XSS): XSS occurs when an attacker injects malicious scripts into web pages that are viewed by other users. These scripts run in the context of the victim’s browser and can steal cookies or other sensitive information, or even perform actions on behalf of the victim.
Subcategories:
\=> Stored XSS: Malicious script stored in a database and displayed later.
\=> Reflected XSS: Malicious script embedded in a URL and executed immediately.
\=> DOM-based XSS: Script injected into client-side JavaScript.
PREVENTION: Escape and sanitize inputs with sanitize
or escape
, avoid raw
in views, implement a Content Security Policy (CSP).
b. Cross-Site Request Forgery (CSRF): CSRF tricks a logged-in user into performing an unintended action on a trusted site. The attacker leverages the user’s authenticated session to execute unwanted actions like changing account settings or transferring funds.
PREVENTION: Enable CSRF protection with protect_from_forgery
, use csrf_meta_tags
to include the CSRF token in the page's <head>
, validate the Origin
header in API requests.
Data Exposure and Object Access Attacks
4. Data Exposure and Object Access Attacks
These attacks exploit access control and sensitive data handling. It has 3 sub-types:
a. Insecure Direct Object References (IDOR)
b. Mass Assignment Vulnerabilities
c. Data Exposure
a. Insecure Direct Object References (IDOR): Attackers manipulate object references (e.g., IDs) to access unauthorized data. A URL like /users/1234
may allow changing the ID to /users/1235
to access another user’s data.
PREVENTION: Validate object ownership usingcurrent_user.id == User.find(params[:id]).id
, use non-predictable identifiers (e.g., UUIDs).
b. Mass Assignment Vulnerabilities: Attackers exploit bulk updates to modify sensitive attributes. Say, attacker updates the role of a pre-existing compromised user ot admin while updating some other user detail.
PREVENTION: Use strong parameters like params.require(:user).permit(:name, :email)
, use attr_protected
for versions below Rails 3.2.
c. Data Exposure: Sensitive data, such as passwords or API keys, is leaked.
PREVENTION: Encrypt sensitive data with Rails’ encryption APIs and Mask sensitive data in logs.
Distributed Denial of Service (DDoS) Attacks and prevention
5. Distributed Denial of Service (DDoS) Attacks
These attacks aim to disrupt service availability using various techniques. It has 2 sub-types:
a. HTTP Flooding
b. Resource Exhaustion
a. HTTP Flooding: Attackers overload servers with a high volume of requests. Do you recall the time when the BookMyShow site went down as soon as the booking went live for the ColdPlay concert? Yes.. an attack similar to that.
PREVENTION: Use rate-limiting middleware like rack-attack
, cache responses using Rails caching mechanisms.
b. Resource Exhaustion: Attackers deplete server resources by exploiting high-cost operations. A very recent example of this is the CVE-2022–20426 vulnerability in the Android Operating System. Discovered in 2022, this vulnerability would crash the android phone when a user would select a phone account as it would create multiple copies and deplete the memory of the device.
PREVENTION: Limit file upload sizes using config.active_storage
, validate inputs to avoid excessive resource usage.
File Upload and Deserialization Attacks
6. File Upload and Deserialization Attacks
Web applications often handle user-generated content, such as file uploads, or deserialize data for performance and flexibility. However, these processes can introduce significant security vulnerabilities if not handled properly. To understand this better, let’s understand it’s 2 sub-types:
a. Malicious File Uploads
b. Insecure Deserialization
a. Malicious File Uploads: Attacker uploads files containing malicious scripts or malware to corrupt the system. Let’s say a file was uploaded into the system that contained a malware which would damage the entire system.
PREVENTION: Validate file types and sizes using gems like carrierwave
or shrine
, store uploaded files in a safe location, not the root directory.
b. Insecure Deserialization: In this type of attack, unsafe deserialization is exploited to execute malicious payloads. In 2017, a critical vulnerability in Apache Struts 2, a popular open-source web application framework, allowed attackers to perform remote code execution by exploiting insecure deserialization. This vulnerability was notably responsible for the massive Equifax data breach, which exposed the personal information of 147 million people.
PREVENTION: Avoid deserializing untrusted input and Use JSON or YAML parsers that do not evaluate code.
Missed Configuration and Security Misconfigurations being misused
7. Configuration and Security Misconfigurations
As the name suggests, these attacks happen when some configuration is missing or some mis-configuration occurs in the application.
For example:
a. Lack of headers like X-Content-Type-Options
and CSP allows attacks like XSS.
b. Sensitive debugging details (e.g., stack traces) are left in console exposing these details to users.
PREVENTION: Disable detailed error messages in production using config.consider_all_requests_local = false
, Use custom error pages for production.
8. Business Logic Attacks
Here, the attacker Exploits weaknesses or edge cases that were not handled properly in application logic.
For example: A discount coupon can be reused indefinitely due to missing validations.
PREVENTION: Test edge cases in business logic and validate workflows and enforce state transitions in models.
General Best Practices:
Update Rails and Dependencies Regularly: Stay protected against known vulnerabilities.
Perform Security Audits: Use tools like Brakeman for static analysis.
Use security gems: Use gems like
rack-attack
,rack-cors
,secure-headers
andbrakeman
to secure your apps.Educate Your Team: Awareness of common vulnerabilities ensures better prevention.
To read more about application security, refer rails-guides, rack-attack, rack-cors, secure-headers and brakeman for information specific to rails and XSS for cross-site scripting attacks.
Summary:
Staying up-to-date and checking your applications for different security threats is very important. We never know how the slightest negligence can rob away years of work and cause legal implications. Hence,
Stay aware, stay vigilant!
You can follow me on:
LinkedIn: https://www.linkedin.com/in/samvita-karkal-354628168/
X / Twitter: https://x.com/karkalsamvita
Peerlist: https://peerlist.io/samvitak
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.