How to Measure Page Load Times with Selenium?

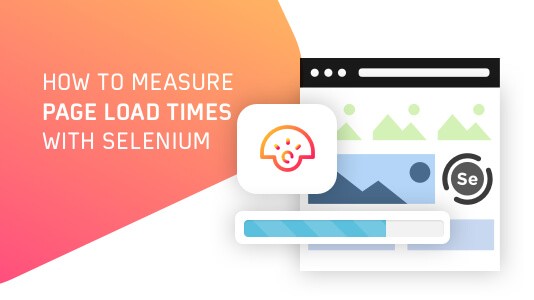
Introduction
In today's fast-paced digital world, page load time is more than just a performance metric it's a critical factor that impacts user experience, website traffic, and ultimately the success of an online business. Websites that load slowly are more likely to lose visitors, leading to decreased conversion rates, poor SEO rankings, and lower customer satisfaction. For these reasons, web developers, QA engineers, and automation testers need to ensure that their web applications perform optimally, especially in terms of loading speed.
Selenium, one of the most popular frameworks for web automation testing, is often used to test the functionality of web applications. But did you know that you can also use Selenium to measure and optimize page load times? In this blog post, we'll explore how to measure page load times with Selenium, with practical examples and code snippets to help you understand how to implement this in your testing workflow.
If you're looking to enhance your Selenium skills or even pursue a Selenium certification for beginners, this guide will provide valuable, hands-on insights. We will cover everything from what Selenium is, why measuring page load time is important, and how to set up the necessary tools to run your tests effectively.
What is Selenium and Why Measure Page Load Times?
Before diving into the details of measuring page load times, it's essential to understand what Selenium is and why page load time matters.
Selenium is an open-source tool for automating web browsers. It supports a range of browsers and programming languages, making it incredibly versatile for testing web applications. It’s commonly used for tasks such as automating repetitive actions, functional testing, and regression testing.
Page load time refers to how long it takes for a web page to fully load and display in a browser. This time includes loading HTML, CSS, JavaScript, images, fonts, and any other content that the browser needs to render the page correctly. According to a report by Google, 53% of mobile users will abandon a site that takes longer than 3 seconds to load. Slow page load times can have severe consequences for both user experience and SEO performance, making it a crucial metric to monitor during testing.
Setting Up Selenium for Page Load Time Testing
To begin measuring page load times with Selenium, you’ll need to set up your testing environment. Here’s a step-by-step guide to help you get started:
1. Install Selenium WebDriver
Before we can run any tests, we need to install Selenium. Selenium WebDriver allows you to control a browser and interact with web elements programmatically. The installation process varies depending on the language you're using (Python, Java, etc.). For this tutorial, let’s use Python.
To install Selenium for Python, use pip:
bash
pip install selenium
2. Download WebDriver for Your Browser
Selenium needs a driver to communicate with the browser of your choice. You can download drivers for different browsers from the following links:
Google Chrome: ChromeDriver
Mozilla Firefox: GeckoDriver
Microsoft Edge: EdgeDriver
Make sure to download the appropriate version of the driver based on your browser version.
3. Set Up Your IDE
Ensure you have a suitable IDE (Integrated Development Environment) for writing Python code. PyCharm, VS Code, or any text editor should work fine. If you're using Jupyter Notebook, it can be especially useful for quick testing.
Understanding Page Load Time
Page load time is generally divided into three key metrics:
DNS Lookup: Time taken to resolve the domain name to an IP address.
Initial Connection: Time taken to establish the connection to the server.
Content Download: Time taken to download the content of the page, including HTML, CSS, images, and JavaScript files.
In Selenium, you can measure these aspects by using the browser’s internal performance metrics. The page load time will give you an overall understanding of how efficiently a page loads.
Using Selenium to Measure Page Load Time
To measure page load time, we can use JavaScript execution within the Selenium WebDriver. By querying the browser's performance timing API, we can collect accurate data about the load time.
1. Code Example to Measure Page Load Time
Here’s a simple Python code snippet using Selenium to measure page load time:
python
from selenium import webdriver
import time
# Set up the WebDriver (for Chrome in this case)
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
# Start the timer
start_time = time.time()
# Open the webpage
driver.get('https://www.example.com')
# Calculate the page load time
load_time = time.time() - start_time
# Print the page load time
print(f"Page load time: {load_time} seconds")
# Close the browser
driver.quit()
2. Explanation of Code
WebDriver Setup: First, we initialize the WebDriver and point it to the Chrome browser.
Timing the Load: Using Python’s time.time(), we start a timer before calling driver.get(), which loads the webpage.
Calculating Load Time: Once the page is loaded, we stop the timer and calculate the difference between the start and end times.
Output: The script prints the page load time in seconds.
This code measures the time it takes for the page to load. However, it does not give detailed breakdowns of different phases of loading (like DNS lookup, connection time, or content download). To get more granular information, you’ll need to leverage browser performance APIs.
Leveraging Browser Performance APIs in Selenium
For more detailed page load timing, you can use the browser's performance timing API, which provides various performance metrics such as DNS resolution time, connection time, and load time for resources.
1. Accessing Performance Timing API in Selenium
You can use JavaScript execution in Selenium automation testing to extract these detailed metrics. Here’s an example:
python
from selenium import webdriver
import json
# Set up the WebDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
# Open the webpage
driver.get('https://www.example.com')
# Execute JavaScript to get the performance timings
performance_data = driver.execute_script("""
return JSON.stringify(window.performance.timing);
""")
# Parse the JSON data
performance_data = json.loads(performance_data)
# Calculate page load time
page_load_time = (performance_data['loadEventEnd'] - performance_data['navigationStart']) / 1000
print(f"Page load time: {page_load_time} seconds")
# Close the browser
driver.quit()
2. Explanation of the Code
JavaScript Execution: The script uses driver.execute_script() to run JavaScript code in the browser, extracting the performance timing data in JSON format.
JSON Parsing: The raw JSON data is parsed to access various timing metrics.
Page Load Time: We calculate the total page load time by subtracting navigationStart from loadEventEnd, giving the total time for the page to load.
This method provides a detailed breakdown of load times and can help identify performance bottlenecks in your web application.
Additional Techniques for Measuring Page Load Time
While Selenium is excellent for basic page load time measurement, there are several additional techniques and tools you can incorporate into your testing workflow:
Network Throttling: Simulate slower network connections (e.g., 3G or 2G) to test how your site performs under real-world conditions.
Browser DevTools Protocol: For more granular control, you can use the Chrome DevTools Protocol, which allows you to access low-level browser data, including resource loading time.
Headless Browsers: Run Selenium tests with headless browsers (without a GUI) for faster execution of page load tests.
Why Measure Page Load Times in Selenium?
Measuring page load times with Selenium can bring several benefits to your testing and development process:
Performance Optimization: Understanding where bottlenecks occur allows you to optimize loading times for a smoother user experience.
SEO Improvement: Search engines like Google use page load times as a ranking factor. Faster pages tend to rank better, improving organic traffic.
Quality Assurance: Page load time is an essential part of web application performance testing, ensuring that your application meets both user and business expectations.
Conclusion
Measuring page load times is a crucial step in ensuring that your web application performs optimally. By integrating Selenium into your testing process, you can automate the measurement of page load times and identify areas for improvement. Whether you're just starting with Selenium or looking to earn your Selenium certification for beginners, mastering performance testing with Selenium is a valuable skill that will help you optimize websites and enhance user experience.
If you're interested in hands-on learning, H2K Infosys offers comprehensive Online Selenium training to equip you with the skills necessary for a successful career in automation testing.
Key Takeaways
Selenium can be used to measure page load times, providing valuable insights into your web application’s performance.
You can utilize browser performance APIs for more detailed load timing metrics.
Understanding page load times helps with optimizing user experience, improving SEO, and ensuring high-quality applications.
If you're ready to take your testing skills to the next level, enroll in H2K Infosys’ Selenium course today and become proficient in measuring and improving web application performance.
Subscribe to my newsletter
Read articles from Stella directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Stella
Stella
I am a passionate blogger focused on writing in-depth articles about Selenium automation testing. My blogs aim to guide learners through the intricacies of IT Courses, offering insights into industry best practices, course certifications. Whether you're just starting out or looking to advance your skills, my content is designed to support your journey toward becoming a certified expert.