Implementing Different Types of Inheritance in Hibernate
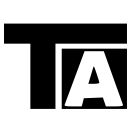
5 min read
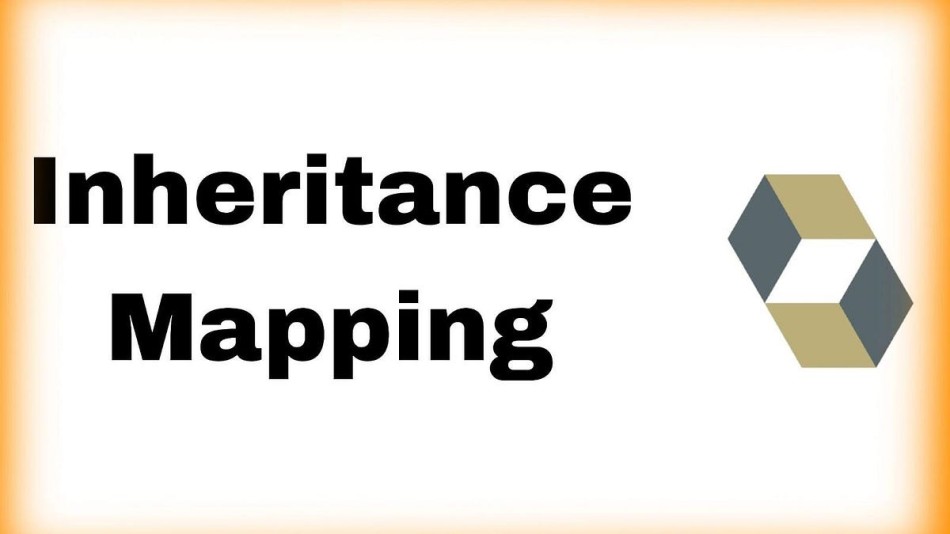
1. What is Inheritance in Hibernate?
Before diving into the implementation, let’s briefly discuss inheritance in Hibernate. Inheritance is the mechanism where one entity can inherit fields and behaviors from another entity. In the context of Hibernate, this means mapping Java class hierarchies to database tables.
1.1 Single Table Inheritance Strategy
The Single Table strategy is the simplest of all inheritance strategies. All entities in the inheritance hierarchy are mapped to a single table, which includes a discriminator column to distinguish between different entity types. This approach is efficient as it uses a single table, but it can result in sparse tables with many null values.
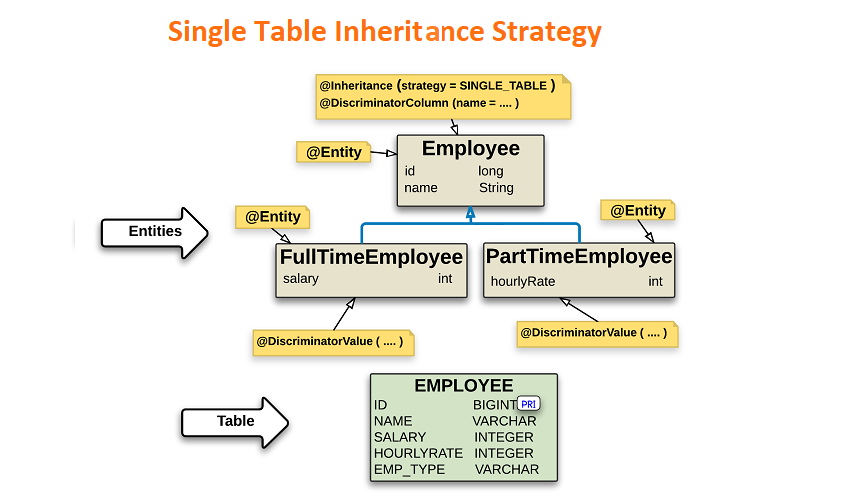
Code Example:
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "entity_type", discriminatorType = DiscriminatorType.STRING)
public class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
}
@Entity
@DiscriminatorValue("Car")
public class Car extends Vehicle {
private int numberOfDoors;
}
@Entity
@DiscriminatorValue("Bike")
public class Bike extends Vehicle {
private boolean hasPedals;
}
Advantages
- Performance: Only one table is queried, making reads and writes faster.
- Simplicity: One table means fewer joins and easier queries.
Disadvantages
- Wasted space: A lot of null fields may be present for different types of entities.
- Limited flexibility: Complex hierarchies can become difficult to manage in a single table.
1.2 Joined Table Inheritance Strategy
The Joined Table strategy maps each class in the hierarchy to its own table. It uses foreign keys to represent relationships between tables. This approach avoids null fields but requires more joins, which can affect performance.
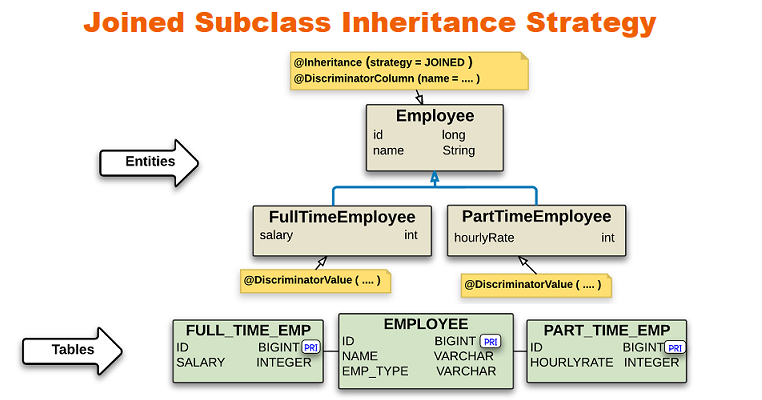
Code Example:
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
}
@Entity
public class Car extends Vehicle {
private int numberOfDoors;
}
@Entity
public class Bike extends Vehicle {
private boolean hasPedals;
}
Advantages
- Efficient storage: No null values as each subclass has its own table.
- Flexibility: Allows for complex hierarchies without overloading a single table.
Disadvantages
- Performance hit: Multiple joins are needed for retrieving a single entity.
- Complex queries: The structure can make query writing more complex.
1.3 Table Per Class Inheritance Strategy
The Table Per Class strategy creates a separate table for each class in the inheritance hierarchy. Each table has all the fields of the parent class and its own fields. This strategy is useful for entities that are mostly unrelated but still share a common parent.
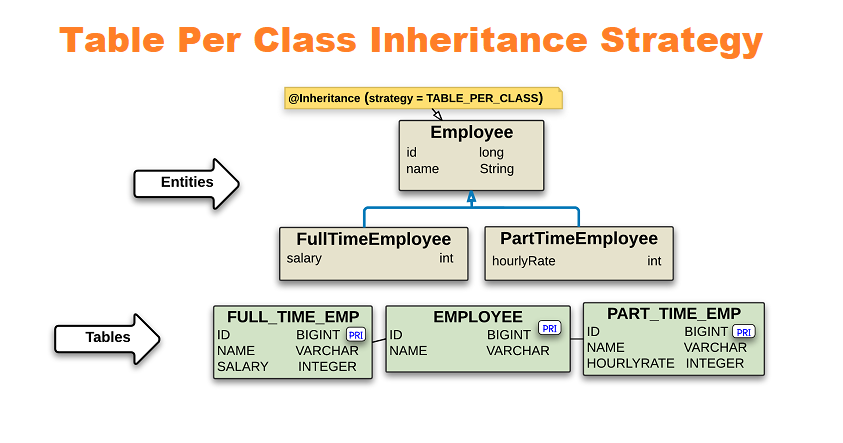
Code Example:
@Entity
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
public class Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String manufacturer;
}
@Entity
public class Car extends Vehicle {
private int numberOfDoors;
}
@Entity
public class Bike extends Vehicle {
private boolean hasPedals;
}
Advantages
- No joins: Since each class is stored in its own table, no joins are required.
- Simpler queries: Each entity can be queried directly without needing to join with parent tables.
Disadvantages
- Data duplication: Fields in the parent class are duplicated across tables.
- Inefficient storage: Redundant data can lead to increased storage requirements.
1.4 Mapped Superclass Inheritance Strategy
The Mapped Superclass strategy doesn’t represent a relationship in the database. Instead, it serves as a way to share attributes between entities without creating a table for the parent class. Only the subclasses have corresponding tables in the database.
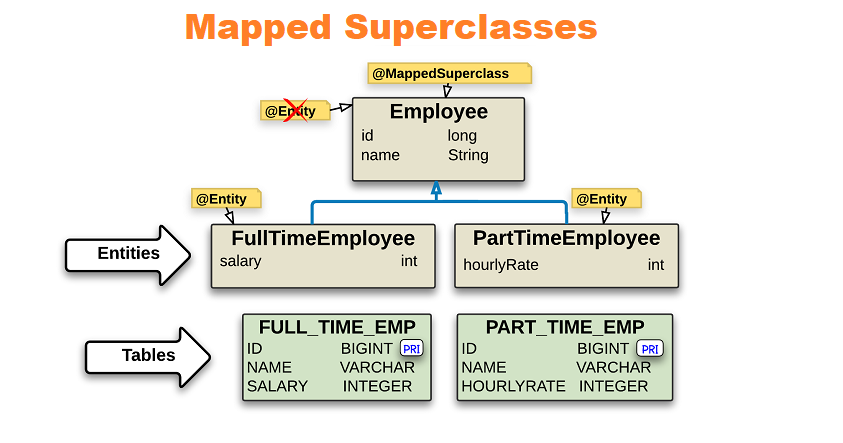
Code Example:
@MappedSuperclass
public class Vehicle {
private String manufacturer;
}
@Entity
public class Car extends Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private int numberOfDoors;
}
@Entity
public class Bike extends Vehicle {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private boolean hasPedals;
}
Advantages
- No additional table: The parent class doesn’t create an extra table, simplifying the schema.
- Reusability: Shared attributes across multiple entities.
Disadvantages
- No polymorphic queries: Since the parent class doesn’t exist as a table, querying all entities that inherit from the parent class isn’t possible.
2. Which Inheritance Strategy Should You Choose?
Choosing the right inheritance strategy depends on the specific needs of your application. Let’s explore the factors that should guide your decision:
2.1 Performance Requirements
If performance is a critical factor, the Single Table strategy might be the best choice due to its efficiency in querying and storage. However, it may not be ideal for complex hierarchies.
2.2 Data Complexity
If your entity hierarchy is complex and involves many fields that may not apply to all subclasses, the Joined Table strategy offers the flexibility needed, at the cost of additional join operations.
2.3 Storage Efficiency
For applications where storage optimization is essential, the Table Per Class and Joined Table strategies will ensure that unnecessary fields are not stored in the database.
2.4 Flexibility in Queries
The Mapped Superclass strategy is perfect for situations where shared fields are needed without requiring polymorphic queries.
3. Conclusion
Each inheritance strategy in Hibernate serves a unique purpose and comes with its pros and cons. The decision to use one over the other depends on the specific requirements of your project, such as performance, data complexity, and storage efficiency. Experiment with these strategies, and you’ll find the best one for your use case.
Feel free to leave a comment below if you have any questions or need further clarification!
Read more at : Implementing Different Types of Inheritance in Hibernate
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
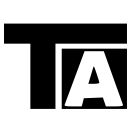
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.