String Morphing Demo in P5JS
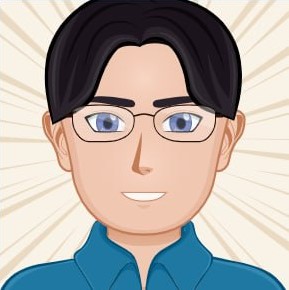
Ý tưởng cơ bản
Mỗi ký tự được tạo thành bởi các điểm, một string được hợp thành bởi các ký tự.
Việc biến ký tự này thành ký tự kia thực chất là dịch chuyển các điểm của ký tự cái này sang vị trí của ký tự kia, và không quan tâm đến thứ tự và vị trí.
Vấn đề 1 - Làm sao lấy thông tin về các điểm cấu tạo nên ký tự?
Trong source code, function getTextPoints(str, x, y, fontSize, N) làm việc này:
Tạo ra một vùng nhớ, một bản vẽ chữ nhật đủ chỗ để vẽ chuỗi str với background màu đen
Vẽ chuỗi str lên đó với màu trắng
Như vậy, nhìn trên bản vẽ ấy, ta chỉ cần lọc tất cả các điểm màu trắng ra là xong
// Collect positions of white pixels (text)
for (let py = 0; py < pg.height; py++) {
for (let px = 0; px < pg.width; px++) {
// Get pixel index
// multiply by 4 because each pixel has 4 values (RGBA)
// pg.width is the width of the graphics buffer
let index = (px + py * pg.width) * 4;
if (pg.pixels[index] > 128) {
// Threshold for white pixels
// Map to canvas coordinates, adjusting for baseline
filledPixels.push({ x: px + x, y: py + y - 50 });
}
}
}
Vấn đề 2 - Xử lý số lượng điểm chênh lệch khi lấy mẫu
Hàm getTextPoints(str, x, y, fontSize, N) có thông số N là số lượng điểm cần lấy từ các ký tự trong chuỗi str.
Nếu tổng số điểm trong chuỗi không đủ => tạo ra các bản clone cho đủ số N
Nếu tổng số điểm dư => lấy ngẫu nhiên cho đủ số N
// Sample N points
if (filledPixels.length <= N) {
// If there are fewer points than requested,
// add random points to fill the gap
// those random points are just the randomly cloned points from the text
while (filledPixels.length < N) {
let randomIndex = floor(random(filledPixels.length));
filledPixels.push({ x: filledPixels[randomIndex].x, y: filledPixels[randomIndex].y });
}
return filledPixels;
} else {
// Shuffle and take first N points
let shuffled = filledPixels.sort(() => 0.5 - Math.random());
return shuffled.slice(0, N);
}
Vấn đề 3 - Biến hình (Morphing)
Việc này được xử lý trong function morph(srcParticles, destParticles). Như đã mô tả ở trên, biến hình thực chất là việc dời toàn bộ N điểm của tập hợp các điểm nguồn srcParticles sang đích destParticles. Mình đã tạo ra một thực thể - class Particle(current, end, color, noiseRange) để mô tả từng điểm với các thông tin:
Tọa độ hiện tại, tọa độ đích, màu sắc, độ nhiễu. Độ nhiễu biểu hiện cho sự rung động của particle ấy.
Particle.draw(): vẽ thực thể này ra màn hình
Particle.update(animationProgress): tính toán vị trí hiện tại của particle - cốt lõi của sư biến hình :) Mình đã dùng một hàm nội suy tuyến tính lerp (linear interpolation) của thư viện p5js để tính vị trí hiện tại, sau đó thêm noise để tao rung động.
update (animationProgress) {
// update the particle's position
// lerp() is a p5.js function that interpolates between two values
this.current.x = lerp(this.current.x, this.end.x, animationProgress);
this.current.y = lerp(this.current.y, this.end.y, animationProgress);
// add some noise to the particle's position
this.current.x += random(-this.noiseRange, this.noiseRange);
this.current.y += random(-this.noiseRange, this.noiseRange);
}
Hàm nội suy tuyến tính - lerp(s,d,t)
Hàm này nhận 2 vector \(\mathbf s\) - source, \(\mathbf d\) - destination và một biến \(0 \le t \le 1\) và trả về \(\mathbf c_t\) là vector vị trí ứng với \(t\).
$$\mathbf c_t = \mathbf s + t \times (\mathbf d - \mathbf s)$$
Ví dụ: Với \(\mathbf s = (1,1), \mathbf d=(2,3), t=0.5\) thì \(\mathbf c_t = (1,1) + 0.5((2-1,3-1)) = (1,1) + 0.5(1,2) = (1.5,2)\)
Tất cả chỉ có vậy thôi, enjoy nhé các bạn!
Subscribe to my newsletter
Read articles from Legos Light directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
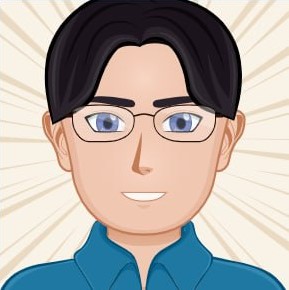