JavaScript DOM Sorcery: Unlocking the Magic Behind Every Modern Website

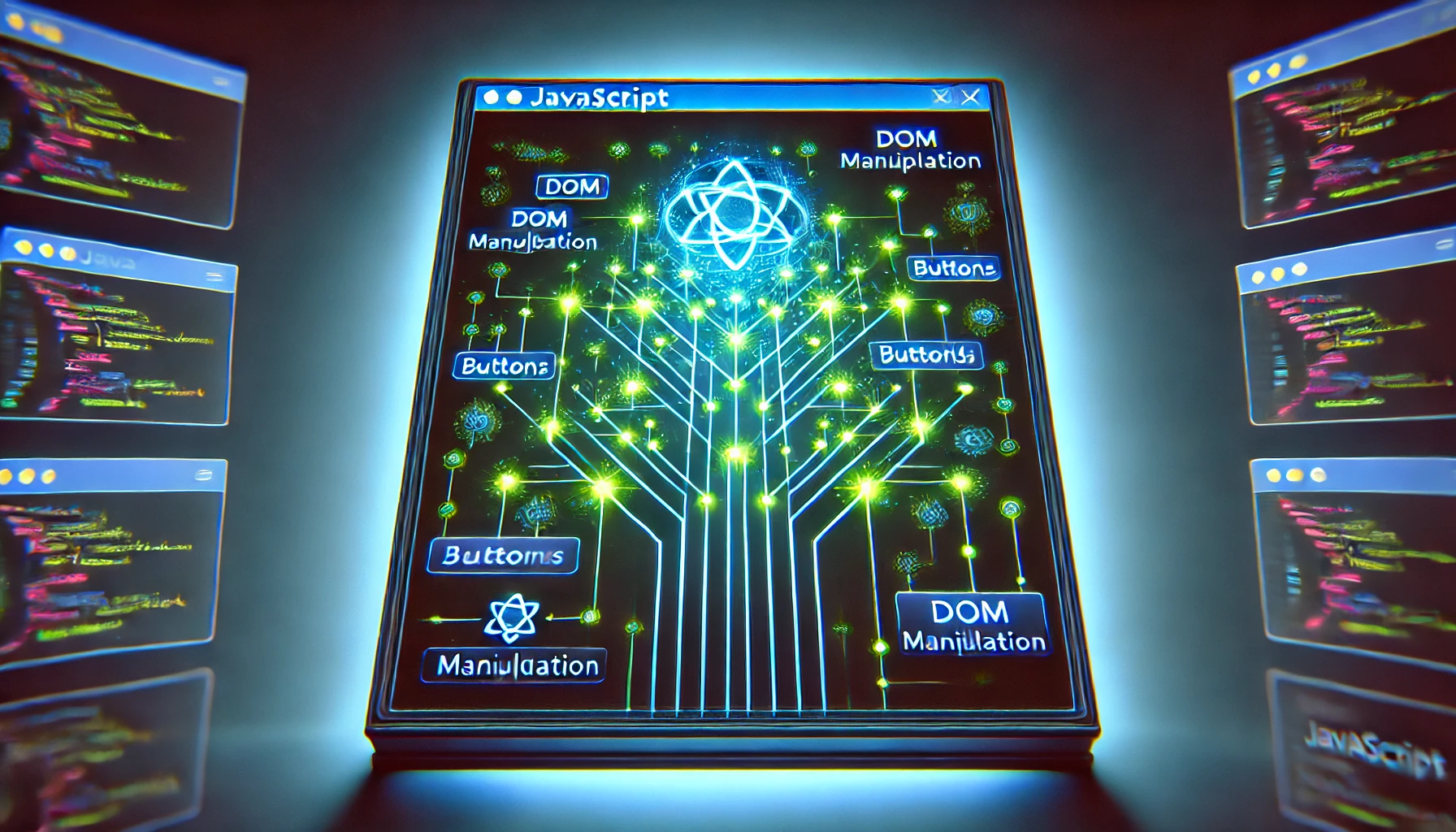
Ever wondered how a button click changes the entire layout of your favorite website? Or how typing into a search box instantly shows matching results? The secret isn't pixie dust—it's the powerful relationship between JavaScript and the Document Object Model (DOM). Let's pull back the curtain on this fascinating duo that powers virtually every interactive website you've ever visited.
What Exactly Is This "DOM" Thing Anyway?
Think of the DOM as a family tree for your webpage. When a browser loads HTML, it doesn't just display text and images—it creates a structured representation where every element becomes an object in a hierarchical tree.
Interesting tidbit: The concept of the DOM wasn't initially created for JavaScript. It emerged as a language-neutral way for programs to interact with HTML, XML, and XHTML documents. JavaScript just happens to be exceptionally good at manipulating it!
But why does this matter? Because the DOM is your gateway to making web pages come alive. Without it, websites would be static documents—digital brochures rather than the interactive experiences we've come to expect.
Accessing DOM Elements: Your JavaScript Superpower
Before you can change anything on a webpage, you need to find the elements you want to modify. JavaScript gives us several methods to do this:
// Get a single element by its ID
const header = document.getElementById('main-header');
// Get elements by their class name (returns a collection)
const paragraphs = document.getElementsByClassName('content-paragraph');
// Get elements by their tag name
const allButtons = document.getElementsByTagName('button');
// Use CSS selectors (modern and powerful)
const firstImportantItem = document.querySelector('.important');
const allImportantItems = document.querySelectorAll('.important');
Think of these methods as your detective tools. They help you locate exactly the elements you need, no matter how complex the page structure gets.
Modifying the DOM: Where the Real Magic Happens
Once you've found your elements, the fun begins. Let's see some common ways to modify the DOM:
Changing Content
// Simple text changes
document.getElementById('greeting').textContent = 'Hello, JavaScript Wizard!';
// Adding HTML content
document.querySelector('.profile-card').innerHTML = `
<h2>User Profile</h2>
<img src="avatar.jpg" alt="Profile picture">
<p>Welcome back, Sarah!</p>
`;
Manipulating Attributes
// Change an image source
document.getElementById('hero-image').src = 'new-image.jpg';
// Toggle a button's disabled state
const submitButton = document.querySelector('button[type="submit"]');
submitButton.disabled = !submitButton.disabled;
// Add or remove a class
document.getElementById('notification').classList.add('visible');
setTimeout(() => {
document.getElementById('notification').classList.remove('visible');
}, 3000); // Remove after 3 seconds
Fun fact: The ability to dynamically modify class names revolutionized web development by allowing developers to trigger CSS animations and transitions through JavaScript!
Transforming Styles On-the-Fly
Ever been amazed by websites that change colors, layouts, or animations as you interact with them? It's all thanks to JavaScript's ability to manipulate CSS properties:
// Change specific styles
const spotlight = document.getElementById('spotlight');
spotlight.style.backgroundColor = '#ffcc00';
spotlight.style.transform = 'scale(1.2)';
spotlight.style.boxShadow = '0 0 20px rgba(255, 204, 0, 0.7)';
// Responsive adjustments based on user actions
function adjustForScreenSize() {
const mainContent = document.querySelector('.content');
if (window.innerWidth < 768) {
mainContent.style.flexDirection = 'column';
mainContent.style.padding = '10px';
} else {
mainContent.style.flexDirection = 'row';
mainContent.style.padding = '20px 40px';
}
}
// Listen for screen resizes
window.addEventListener('resize', adjustForScreenSize);
Creating New Elements From Thin Air
Sometimes you need to add completely new elements to your page. JavaScript makes this a breeze:
// Create a new notification element
function createNotification(message, type = 'info') {
// Create the elements
const notification = document.createElement('div');
notification.className = `notification ${type}`;
const messageText = document.createElement('p');
messageText.textContent = message;
const closeButton = document.createElement('button');
closeButton.textContent = '×';
closeButton.className = 'close-btn';
// Add event listener to close button
closeButton.addEventListener('click', function() {
notification.classList.add('fade-out');
setTimeout(() => notification.remove(), 300);
});
// Build our notification
notification.appendChild(messageText);
notification.appendChild(closeButton);
// Add to the DOM
document.body.appendChild(notification);
// Auto-remove after 5 seconds
setTimeout(() => {
if (notification.parentNode) {
notification.classList.add('fade-out');
setTimeout(() => notification.remove(), 300);
}
}, 5000);
}
// Use it
createNotification('Your changes have been saved!', 'success');
Would you believe that every dynamic notification, modal, tooltip, or popup you've ever seen on a website was likely created using a pattern similar to this? The web is essentially built on these fundamental techniques!
Event Listeners: Making Your Page Respond
A responsive webpage needs to react to user actions. Enter event listeners:
// Simple button click
document.getElementById('submit-form').addEventListener('click', function(event) {
event.preventDefault(); // Stop form submission
validateAndSubmit();
});
// Mouse hover effects
const productCards = document.querySelectorAll('.product-card');
productCards.forEach(card => {
card.addEventListener('mouseenter', function() {
this.classList.add('hovering');
this.querySelector('.product-details').style.opacity = '1';
});
card.addEventListener('mouseleave', function() {
this.classList.remove('hovering');
this.querySelector('.product-details').style.opacity = '0';
});
});
// Keyboard interaction
document.addEventListener('keydown', function(event) {
if (event.key === 'Escape') {
closeAllModals();
}
});
The Flow of DOM Events
When you click a button, multiple events fire in a specific sequence. Understanding this flow is crucial for advanced DOM manipulation:
Did you know? The event bubbling phase that allows events to propagate upward through the DOM tree was a contentious feature during early browser development. Some browser makers wanted events to only trigger on the exact element clicked, but the bubbling approach won out—and thank goodness it did! Without it, modern frameworks like React would be much harder to implement.
Modern DOM Manipulation: Less Code, More Power
The JavaScript ecosystem has evolved dramatically. Modern libraries and frameworks abstract away much of the verbosity:
// Vanilla JavaScript
const items = document.querySelectorAll('.item');
items.forEach(item => {
item.addEventListener('click', function() {
this.classList.toggle('selected');
updateTotalCount();
});
});
// VS a modern framework (React example)
function Item({ name, price }) {
const [selected, setSelected] = useState(false);
return (
<div
className={`item ${selected ? 'selected' : ''}`}
onClick={() => setSelected(!selected)}
>
<h3>{name}</h3>
<p>${price}</p>
</div>
);
}
The Future: What's Coming for DOM Manipulation?
The JavaScript ecosystem continues to evolve at breakneck speed. We're seeing exciting developments like:
Shadow DOM and Web Components providing better encapsulation
CSS Houdini giving JavaScript direct access to the CSS engine
The Intersection Observer API enabling more efficient scroll-based effects
Server Components reducing the need for client-side DOM manipulation entirely
Wrapping Up: Your DOM Journey Has Just Begun
JavaScript's interaction with the DOM is what transforms static web pages into dynamic applications. With these techniques, there's virtually no limit to what you can build in a browser.
The next time you click a button and see a dropdown menu appear, or watch a form validate in real-time, or marvel at a smooth animation, you'll know what's happening behind the scenes. It's not magic—it's JavaScript and the DOM working together to create the modern web experience.
What aspect of DOM manipulation are you most excited to try in your next project? The fundamentals you've learned here will serve as the foundation for everything from simple interactive websites to complex single-page applications.
Subscribe to my newsletter
Read articles from Jatin Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
