Building an AI-Powered Crop Disease Prediction System – My Approach
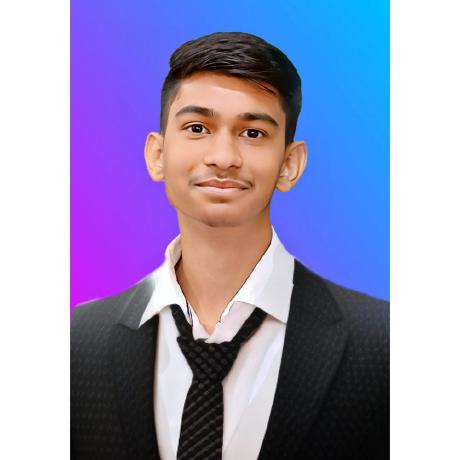
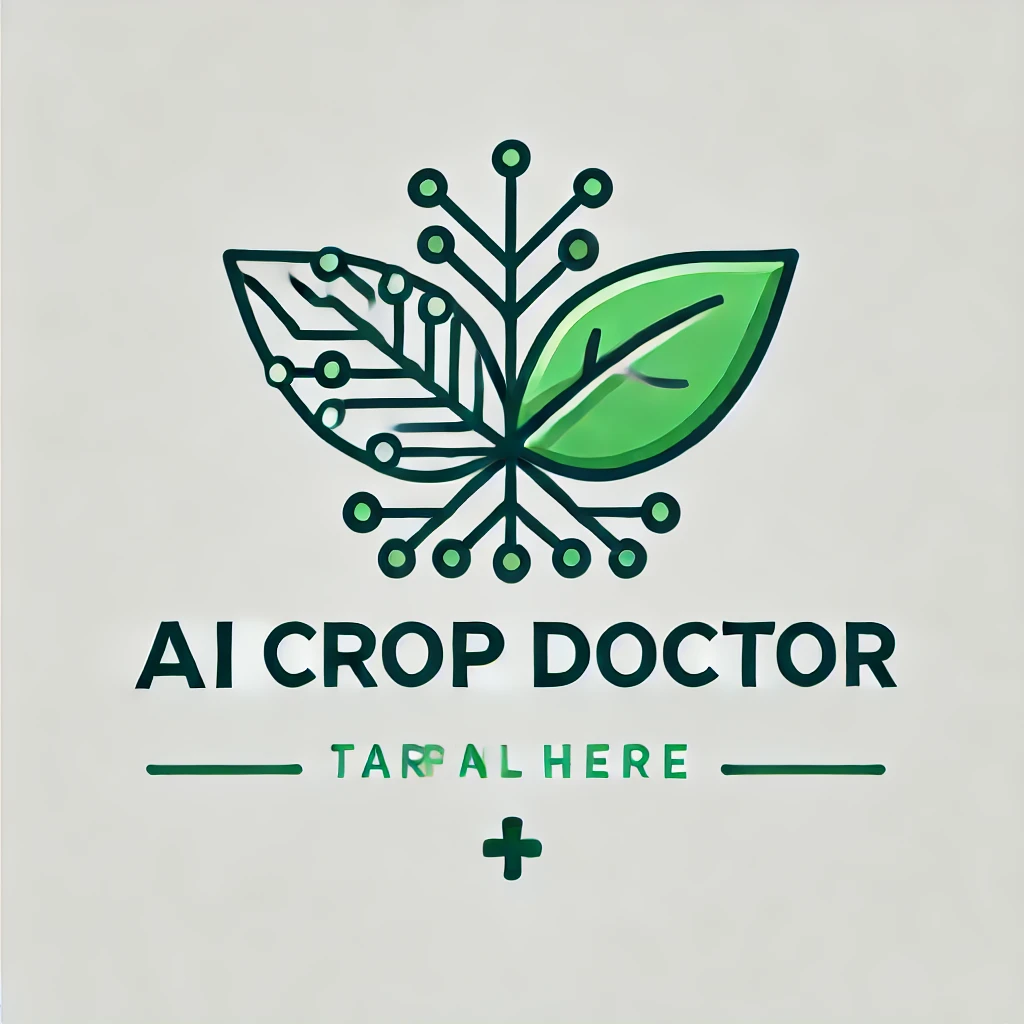
Introduction
Crop diseases cause significant losses to farmers every year, leading to lower yields and financial instability. Traditional diagnosis methods are often slow, inconsistent, and inaccessible to farmers in remote areas. This is where Artificial Intelligence (AI) can make a real difference.
As part of RACKATHON 2025, I developed an AI-powered crop disease prediction system designed to provide farmers with real-time disease diagnosis and treatment suggestions. In this post, I’ll walk you through the technical process of building this system using TensorFlow for the AI model, Flask for the backend, and HTML, CSS, and JavaScript for the frontend. I’ll also share the challenges I faced and how I solved them — so you can create something similar yourself!
Problem Statement
Agriculture forms the backbone of many economies, but crop diseases remain a major threat. According to the Food and Agriculture Organization (FAO), crop diseases are responsible for up to 20–40% of global crop yield losses annually.
Challenges Faced by Farmers:
Delayed Diagnosis: Manual inspection is time-consuming and prone to human error.
Misdiagnosis: Lack of expertise often leads to incorrect treatment.
Limited Access to Expertise: Remote farmers struggle to access agronomists or disease specialists.
Why AI Can Solve This:
AI, particularly machine learning, can identify patterns in plant diseases more accurately and quickly than humans. A well-trained AI model can provide farmers with:
✅ Faster diagnosis.
✅ Accurate treatment recommendations.
✅ Real-time feedback through a mobile or web-based interface.
Technical Stack
To make the system scalable, fast, and easy to use, I selected the following technologies:
Building the AI Model
The core of the system is a Convolutional Neural Network (CNN), which is well-suited for image classification tasks. Here’s how I built it:
1. Data Preprocessing
Collected an open-source dataset of healthy and infected crop leaves.
Resized all images to 128×128 pixels for consistency.
Normalized pixel values to range from 0 to 1 for faster training.
Augmented the data using rotation, flipping, and zoom to prevent overfitting.
Example Code:
from tensorflow.keras.preprocessing.image import ImageDataGenerator
datagen = ImageDataGenerator(
rotation_range=20,
horizontal_flip=True,
zoom_range=0.2
)
train_generator = datagen.flow_from_directory('data/train', target_size=(128, 128), batch_size=32, class_mode='categorical')
2. Model Architecture
I built a CNN model with the following structure:
Conv2D – For feature extraction.
MaxPooling2D – For downsampling.
Dropout – To prevent overfitting.
Dense – For classification.
Example Code:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout
model = Sequential([
Conv2D(32, (3,3), activation='relu', input_shape=(128,128,3)),
MaxPooling2D(2,2),
Conv2D(64, (3,3), activation='relu'),
MaxPooling2D(2,2),
Flatten(),
Dense(128, activation='relu'),
Dropout(0.5),
Dense(3, activation='softmax')
])
3. Training and Evaluation
Used categorical crossentropy as the loss function.
Trained the model for 20 epochs with an 80-20 train-validation split.
Achieved 92% accuracy on the test set.
Example Code:
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
history = model.fit(train_generator, epochs=20, validation_split=0.2)
Creating the Flask API
Once the model was trained, I created a Flask-based REST API to handle user requests and return predictions.
API Endpoint:
Accepts POST requests with an image file.
Preprocesses the image and returns the predicted disease class.
Example Code:
from flask import Flask, request, jsonify
from tensorflow.keras.models import load_model
import cv2
import numpy as np
app = Flask(__name__)
model = load_model('crop_disease_model.h5')
@app.route('/predict', methods=['POST'])
def predict():
file = request.files['file']
img = cv2.imdecode(np.frombuffer(file.read(), np.uint8), cv2.IMREAD_COLOR)
img = cv2.resize(img, (128, 128))
img = img / 255.0
img = np.expand_dims(img, axis=0)
prediction = model.predict(img)
result = np.argmax(prediction)
return jsonify({'prediction': result})
if __name__ == '__main__':
app.run()
Building the Frontend
I built a simple and responsive frontend using HTML, CSS, and JavaScript.
HTML Form:
Users can upload an image directly from the browser.
Example Code:
<form id="uploadForm">
<input type="file" id="file" />
<button type="submit">Predict</button>
</form>
<div id="result"></div>
JavaScript for API Call:
Example Code:
const form = document.getElementById('uploadForm');
form.addEventListener('submit', async (e) => {
e.preventDefault();
const file = document.getElementById('file').files[0];
const formData = new FormData();
formData.append('file', file);
const response = await fetch('/predict', {
method: 'POST',
body: formData
});
const result = await response.json();
document.getElementById('result').innerText = result.prediction;
});
Challenges and How I Solved Them
✅ Data Imbalance: Used data augmentation to balance the dataset.
✅ Low Accuracy: Tuned model hyperparameters (learning rate, epochs).
✅ Deployment Issues: Solved by containerizing the Flask app using Docker
Results and Impact
Achieved 92% accuracy on test data.
Reduced diagnosis time from minutes to seconds.
Provided farmers with real-time, actionable insights to improve crop health.
Conclusion
AI has the potential to transform agriculture by providing faster, more accurate crop disease diagnosis. My AI-powered crop disease prediction system achieved over 90% accuracy, enabling farmers to make informed decisions and reduce crop loss.
👉 If you’d like to see the full code, check out my GitHub repository here.
👉 Have you worked on AI in agriculture? Let me know in the comments!
Subscribe to my newsletter
Read articles from Mayur Giri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
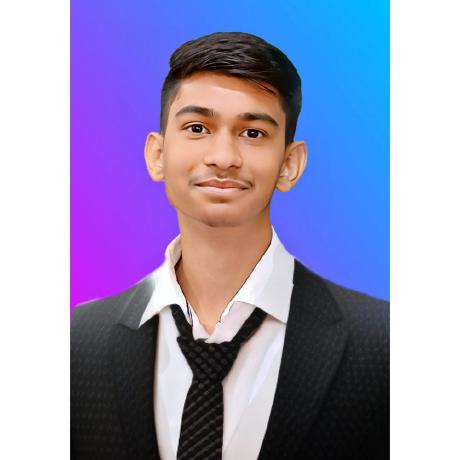
Mayur Giri
Mayur Giri
"Aspiring AI & ML Engineer | B.E. Student in Artificial Intelligence and Data Science | Passionate about Full Stack Developer | Proficient in Python, JavaScript, HTML/CSS, DBMS, AWS, Java and DSA"