Mining Your First Bitcoin Block: A Hands-On Guide

Table of contents
- Introduction
- Key Concepts and Terminology
- Understanding the Mining Process
- 1. Collecting Transactions from the Mempool
- 2. Selecting Transactions for the Block
- 3. Creating the Coinbase Transaction
- 4. Constructing the Merkle Tree
- 5. Mining the Block (Proof of Work)
- 6. Writing the Mined Block to a File
- Conclusion & Summary
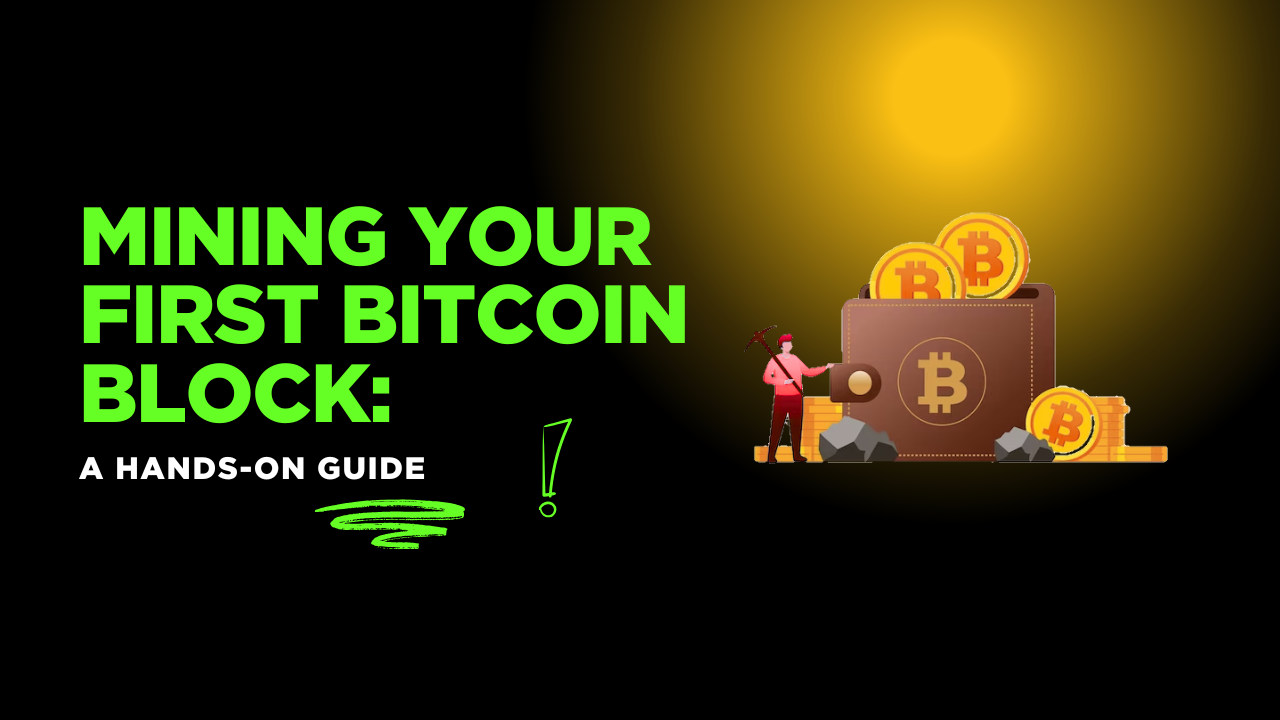
Introduction
Have you ever wondered what happens behind the scenes when a new Bitcoin block is mined? We hear about miners racing to solve complex puzzles, but what does that actually involve? Let’s break it down and build a Bitcoin block from scratch!
In this blog, we’ll walk through the entire mining process—selecting transactions, creating the coinbase transaction, calculating the Merkle root, and assembling the block header. But that’s not all! To officially mine the block, we’ll need to perform proof-of-work, repeatedly hashing the block until we find a valid hash that meets the required difficulty target.
By the end, you won’t just understand Bitcoin mining—you’ll have simulated it yourself. Ready to dig in? Let’s get started!
Key Concepts and Terminology
Before we dive into the mining process, let’s get familiar with some important terms that will come up throughout this blog.
1. Mempool
The mempool (short for "memory pool") is a waiting area for unconfirmed Bitcoin transactions. When a transaction is created, it doesn’t go into a block immediately—it first sits in the mempool, waiting for miners to pick it up and include it in the next block.
2. Coinbase Transaction
No, this isn’t related to the Coinbase exchange! The coinbase transaction is a special transaction that miners create as the first transaction in a block. It rewards the miner with newly minted Bitcoin and collects transaction fees from the included transactions.
3. Merkle Root
A Merkle root is like a fingerprint for all the transactions in a block. It’s a single hash that represents all the transactions in the block, ensuring data integrity. It’s derived using a Merkle tree, where transactions are hashed and combined in pairs until only one final hash remains—the Merkle root.
4. Block Header
The block header is a summary of the block’s key information. It contains:
The previous block’s hash (linking this block to the previous one).
The Merkle root of all transactions in the block.
A timestamp of when the block was created.
The difficulty target (which sets how hard mining is).
A random number called a nonce (used to find a valid block hash).
5. Difficulty Target & Proof of Work
Mining isn’t just about bundling transactions—it’s a race to find a special hash. The block’s hash must be lower than a specific value called the difficulty target. Miners achieve this by adjusting the nonce and repeatedly hashing the block header until they find a valid hash. This process is called proof-of-work and ensures that blocks are not created too quickly.
Understanding the Mining Process
Bitcoin mining consists of:
Collecting Transactions – Miners gather unconfirmed transactions from the mempool.
Creating a Coinbase Transaction – This is the first transaction in a block, which rewards the miner.
Constructing the Merkle Tree – A cryptographic tree that summarizes all transactions.
Building the Block Header – Contains metadata, including the previous block’s hash and Merkle root.
Proof-of-Work (PoW) – Miners find a valid nonce such that the block hash meets the difficulty target.
Appending the Block to the Blockchain – Once mined, the block is added to the chain.
1. Collecting Transactions from the Mempool
Every Bitcoin node maintains a mempool, a temporary storage for unconfirmed transactions. The miner selects transactions based on fees and weight constraints.
Code Snippet: Loading Transactions
mempool_folder = "./mempool"
mempool_files = os.listdir(mempool_folder)
transactions = []
for filename in mempool_files:
if filename == "mempool.json": # Skip the index file
continue
with open(os.path.join(mempool_folder, filename), "r") as f:
tx = json.load(f)
if isinstance(tx, dict) and "txid" in tx and "fee" in tx and "weight" in tx and "hex" in tx:
transactions.append((tx["txid"], tx))
Explanation
The script reads transaction JSON files from the
mempool
folder.Each transaction contains:
txid
: Unique identifierfee
: Transaction fee (higher fees get priority)weight
: Determines how much space it occupies in the blockhex
: Raw transaction data
2. Selecting Transactions for the Block
A Bitcoin block has a maximum weight of 4,000,000 weight units. Transactions are selected based on fee priority while ensuring they fit within this limit.
Code Snippet: Selecting Transactions
transactions.sort(key=lambda x: x[1]["fee"], reverse=True)
selected_txids = []
selected_wtxids = []
total_weight = COINBASE_WEIGHT
for txid, tx in transactions:
if total_weight + tx["weight"] <= 4000000:
selected_txids.append(txid)
tx_bytes = bytes.fromhex(tx["hex"])
wtxid = double_sha256(tx_bytes)[::-1].hex()
selected_wtxids.append(wtxid)
total_weight += tx["weight"]
Explanation
Transactions are sorted by fee (higher fees first).
Transactions are included until the block reaches 4,000,000 weight units.
Witness transaction IDs (
wtxids
) are computed for SegWit compatibility.
3. Creating the Coinbase Transaction
The coinbase transaction is the first transaction in a block. It rewards the miner and includes a witness commitment for SegWit blocks.
Code Snippet: Creating the Coinbase Transaction
def create_coinbase(height, witness_commit):
height_bytes = encode_varint(height)
script_sig = height_bytes + b'\0' * (2 - len(height_bytes))
coinbase_tx = {
"version": 2,
"marker": 0,
"flag": 1,
"vin": [{
"txid": "0" * 64,
"vout": 0xffffffff,
"scriptSig": script_sig.hex(),
"sequence": 0xffffffff,
"witness": [WITNESS_RESERVED_VALUE.hex()]
}],
"vout": [
{
"value": 625000000,
"scriptPubKey": "76a914000000000000000000000000000000000000000088ac"
},
{
"value": 0,
"scriptPubKey": "6a24aa21a9ed" + witness_commit
}
]
}
return coinbase_tx
Explanation
Block height is included in the scriptSig (required by BIP-34).
Output 1: Rewards the miner (12.5 BTC).
Output 2: Stores the witness commitment for SegWit.
4. Constructing the Merkle Tree
A Merkle Tree summarizes all transactions in a block, ensuring integrity and quick verification.
Code Snippet: Computing the Merkle Root
def merkle_root(txids):
if not txids:
return None
level = [bytes.fromhex(txid)[::-1].hex() for txid in txids]
while len(level) > 1:
next_level = []
for i in range(0, len(level), 2):
if i + 1 == len(level):
next_hash = double_sha256(bytes.fromhex(level[i] + level[i])).hex()
else:
next_hash = double_sha256(bytes.fromhex(level[i] + level[i+1])).hex()
next_level.append(next_hash)
level = next_level
return level[0]
Explanation
Transactions are paired and hashed recursively until one root hash remains.
The Merkle root is included in the block header.
5. Mining the Block (Proof of Work)
Bitcoin mining involves finding a nonce such that the block hash meets a difficulty target.
Code Snippet: Mining Process
def mine_block(version, prev_block, merkle_root_hash, timestamp, bits):
nonce = 0
while True:
header = struct.pack("<I32s32sIII",
version,
bytes.fromhex(prev_block)[::-1],
bytes.fromhex(merkle_root_hash),
timestamp,
bits,
nonce
)
block_hash = double_sha256(header)
if block_hash[::-1] < DIFFICULTY_TARGET:
return header.hex(), nonce
nonce += 1
Explanation
The miner iterates nonce values until the double SHA-256 hash meets the difficulty target.
The final block header includes the found nonce.
6. Writing the Mined Block to a File
Once the block is mined, the script writes the block header and included transactions to out.txt
.
Code Snippet: Writing Output
with open("out.txt", "w") as f:
f.write(block_header + "\n")
f.write(binascii.hexlify(coinbase_serialized).decode() + "\n")
f.write("\n".join(selected_txids) + "\n")
Explanation
The block header is recorded first.
The coinbase transaction follows.
Finally, all included transaction IDs are listed.
That is all! You successfully Mined a Block !
Conclusion & Summary
Bitcoin mining is often perceived as an abstract and highly technical process, but at its core, it follows a structured approach involving transaction selection, cryptographic hashing, and proof-of-work computation. Through this blog, we have broken down the mining process step by step, from collecting transactions from the mempool to successfully mining a block and writing it to a file.
Key Takeaways
Transaction Selection – Miners prioritize transactions with the highest fees while staying within the 4MB weight limit.
Coinbase Transaction – This is the first transaction in the block, rewarding the miner and including the witness commitment.
Merkle Tree Construction – A cryptographic structure that ensures all transactions are securely linked and verifiable.
Block Header & Proof of Work – Miners repeatedly hash block headers, adjusting the nonce until they find a valid hash that meets the difficulty target.
Recording the Block – Once mined, the block header, coinbase transaction, and selected transactions are written to an output file, simulating how Bitcoin nodes add blocks to the blockchain.
Final Thoughts
Bitcoin mining is what keeps the network secure and decentralized. The proof-of-work mechanism ensures that transactions are verified and immutable while incentivizing miners through rewards. Although real-world mining requires specialized hardware and energy-intensive computations, this programmatic approach gives us a hands-on understanding of what happens under the hood.
By exploring these concepts in code, we gain a deeper appreciation for blockchain technology and how it maintains trust without central authority. Whether you're a developer, blockchain enthusiast, or just curious about how Bitcoin works, understanding mining at this level provides a solid foundation for further exploration in cryptocurrency and decentralized technologies.
Subscribe to my newsletter
Read articles from Shashank RM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shashank RM
Shashank RM
Sharing my learnings on Full Stack, Web3 and DevOps with the Community.