Understanding Debouncing and Throttling in JavaScript

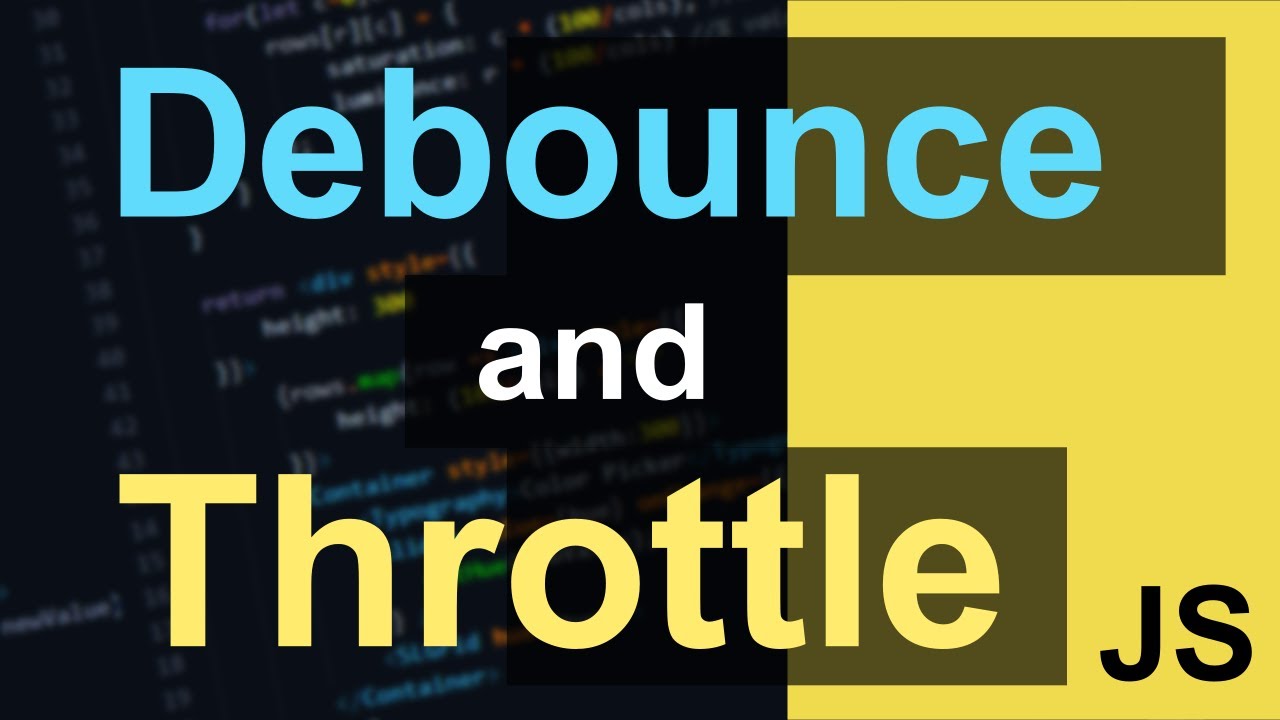
Web performance is a key aspect, and all companies want their websites to load faster, stay performant, and provide a smooth user experience. Javascript applications often need to handle high-frequency events like scrolling, resizing, and user input efficiently to maintain smooth performance. Two common techniques for controlling the execution frequency of functions are debouncing and throttling. While both methods help optimize event handling, they serve distinct purposes and are used in different scenarios. With this article, we know how debouncing and throttling work and their implementation in JavaScript.
What is debouncing?
Debouncing is one of the techniques used to improve performance by limiting the frequency of a particular function called, where the function is triggered frequently, such as input events like typing in an input field or scrolling. This ensures that the function is only called once after a specified period of inactivity.
Use Cases:
Search input suggestions: Only invoke the search API after the user has finished or stopped typing.
Window resizing: Executing the resizing logic after the user has finished or stopped resizing the window.
Button clicks: Prevent double clicks on a button. Implementation We can implement Debouncing by creating a Higher-Order function that will take the original function and a delay amount as input and return a debounce version.
function debounce(fn, delay) {
console.log(arguments); // if i call this funtion with arguments like debounce('angshuman',10); its resturns [Arguments] { '0': 'angshuman', '1': 10 }
let myId; // store the variable
return function (...args) {
// passing the argument of copy value as args
clearTimeout(myId); // clear the previous timout reference of myId
myId = setTimeout(() => {
// store the timeout funtion as referenc with myId
fn.apply(this, args); // apply methods call the function with the given this value and arguments provides as an array
}, delay); // execution the function with delay
};
}
function greet(name){
console.log(name);
}
const callFunc=debounce(()=>greet("angshuman"), 1000);
callFunc() // when its fist call with arguments the myId is undefined so line no 8 is not executable then line no 10 calling with the delay
callFunc() // then second call with this arguments myId hold the reference of the previous call then line 8 excecute and clear the previous reference and the line 10 excute with fn
callFunc() // angshuman // there is no problem the funtion callFunc is calling multiple time its call only once this is call debouncing
What is throttling??
Throttling is a technique that limits the rate at which a function can be called. It ensures that a function is executed at most once in a specified time interval, regardless of how many times its's invoked. This is particularly useful for performance-intensive operations or when you want to control the rate of API calls.
Example: If you have a function that fetches some data from an API every time the user scrolls the page, you might want to throttle it so that it only makes one request every second instead of making hundreds of requests as the user scrolls. This way, you can avoid overloading the server or the browser with unnecessary requests and reduce the bandwidth consumption.
Use Cases:
Scrolling events: Execute scroll-related logic (such as infinite scroll and lazy loading) periodically while the user scrolls.
Mouse movement tracking: Track mouse position at regular intervals for performance-sensitive tasks.
API rate limiting: limit the number of API calls over a period of time.
// main goal of throttling is if you give me multiple request i don't need i get only 1 request at a time
function throttling(fn, delay) {
let myId = null; // variable to keep tarck of the timer
// return throttled version
return (...args) => {
if (myId === null) {
// if there is no timer currently running
fn(...args); // execute the function
myId = setTimeout(() => {
// set a timer to clear the myId after the specified delay
myId = null; // clear the myId to allow the main function to be executed again
}, delay);
}
};
}
throttling()// run the function
throttling() // run the function with specified delay
When to Use Which?
Use debouncing when you want to execute a function only after the user stops performing an action. Use throttling when you want to ensure a function runs at most once per time period, even if the event occurs multiple times. Real-World Example: Scroll Event Imagine a webpage where you load more content when the user scrolls down.
With Debouncing: New content will load only after the user stops scrolling for a certain time.
With Throttling: New content will load at most once every second, even if the user keeps scrolling continuously.
Conclusion:
Both debouncing and throttling are powerful techniques for optimizing performance and improving user experience. They prevent unnecessary function calls, making applications smoother and more efficient.
Use debouncing when user inactivity should trigger the function. Use throttling when a function should execute at regular intervals, even during continuous activity.
Understanding when to use debounce vs. throttle can significantly improve performance, especially in event-driven applications like search, scrolling, and real-time tracking.
Subscribe to my newsletter
Read articles from Angshuman Bhowmick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
