setTimeout, setInterval

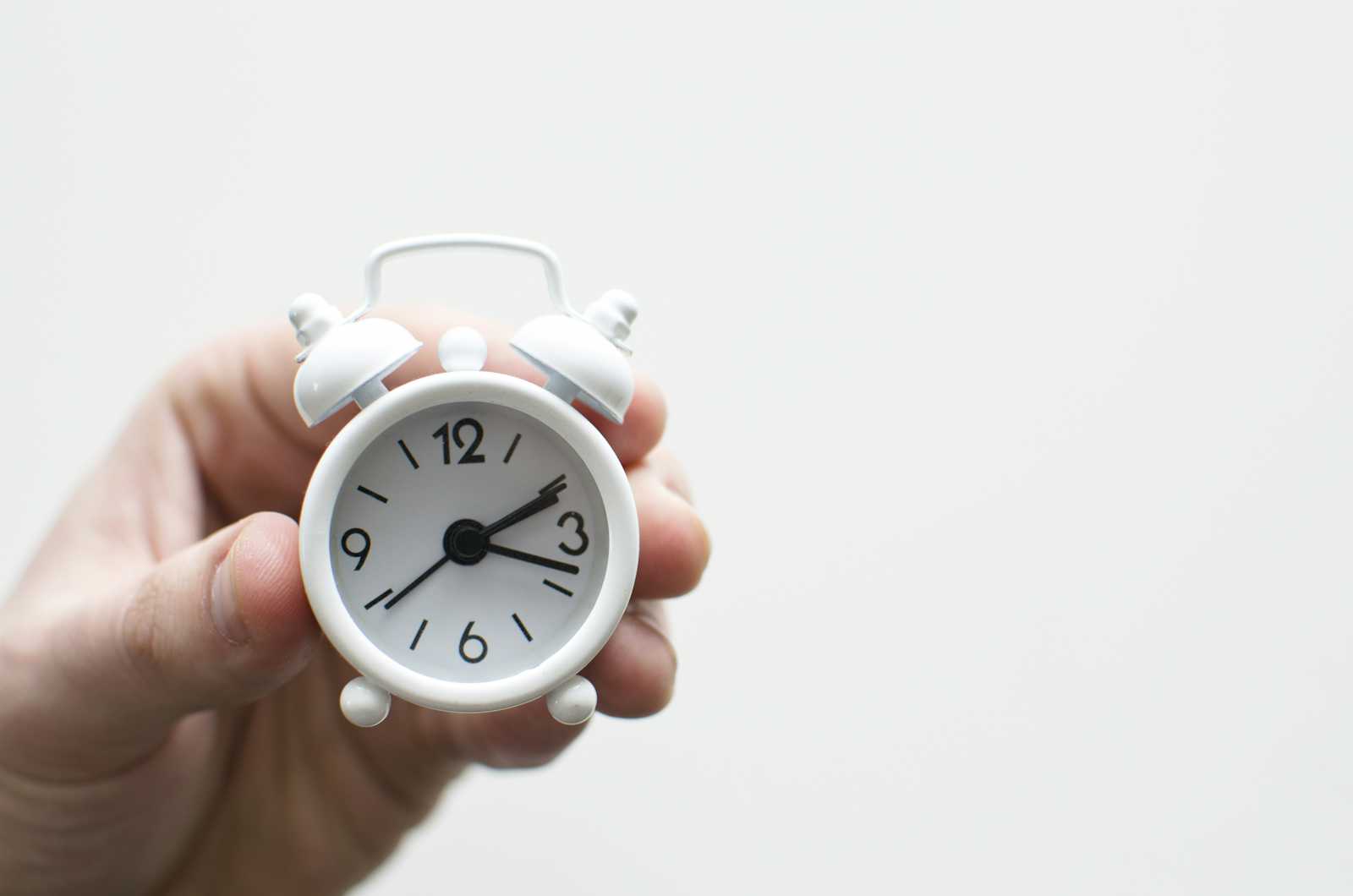
Here are notes on setTimeout
, setInterval
, and related methods in JavaScript:
1. setTimeout()
Definition:
setTimeout()
is a function that executes a piece of code after a specified delay.
Syntax:
let timeoutID = setTimeout(callback, delay, param1, param2, ...);
callback
: Function to execute after the delay.delay
: Time in milliseconds (1000 ms = 1 second).param1, param2, ...
: Optional parameters to pass to the callback function.timeoutID
: Returns a unique ID for clearing the timeout.
Example:
setTimeout(() => {
console.log("Executed after 2 seconds");
}, 2000);
2. clearTimeout()
Definition:
clearTimeout()
is used to cancel a timeout set by setTimeout()
.
Syntax:
clearTimeout(timeoutID);
timeoutID
: The ID returned bysetTimeout()
.
Example:
let timer = setTimeout(() => {
console.log("This will not execute");
}, 3000);
clearTimeout(timer); // Cancels the timeout before execution
3. setInterval()
Definition:
setInterval()
repeatedly executes a function after a specified interval.
Syntax:
let intervalID = setInterval(callback, delay, param1, param2, ...);
callback
: Function to execute at each interval.delay
: Time in milliseconds between executions.intervalID
: Returns a unique ID for clearing the interval.
Example:
let count = 0;
let interval = setInterval(() => {
count++;
console.log("Repeating every 1 second:", count);
}, 1000);
4. clearInterval()
Definition:
clearInterval()
is used to stop an interval set by setInterval()
.
Syntax:
clearInterval(intervalID);
intervalID
: The ID returned bysetInterval()
.
Example:
let counter = 0;
let interval = setInterval(() => {
counter++;
console.log("Counting:", counter);
if (counter === 5) {
clearInterval(interval); // Stops after 5 counts
console.log("Interval Stopped!");
}
}, 1000);
5. setImmediate()
(Node.js only)
Executes a function as soon as the current event loop cycle finishes.
Works similarly to
setTimeout(fn, 0)
, butsetImmediate()
executes before timers.
Example:
setImmediate(() => {
console.log("Executed immediately after I/O tasks");
});
6. process.nextTick()
(Node.js only)
Executes a function before any I/O tasks or timers.
Higher priority than
setImmediate()
.
Example:
process.nextTick(() => {
console.log("Executed before setImmediate");
});
Differences Between setTimeout()
and setInterval()
Feature | setTimeout() | setInterval() |
Execution | Runs once after a delay | Runs repeatedly after a delay |
Return Value | Timeout ID | Interval ID |
Stopping | clearTimeout(timeoutID) | clearInterval(intervalID) |
Best Practices:
Always store the ID returned by
setTimeout()
orsetInterval()
for later clearing.Use
clearTimeout()
andclearInterval()
to prevent memory leaks.Avoid using
setTimeout()
inside a loop; instead, use recursion if needed.Prefer
setImmediate()
andprocess.nextTick()
in Node.js for event-driven tasks.
Subscribe to my newsletter
Read articles from Dikshant Koriwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dikshant Koriwar
Dikshant Koriwar
Hi, I'm Dikshant – a passionate developer with a knack for transforming ideas into robust, scalable applications. I thrive on crafting clean, efficient code and solving challenging problems with innovative solutions. Whether I'm diving into the latest frameworks, optimizing performance, or contributing to open source, I'm constantly pushing the boundaries of what's possible with technology. On Hashnode, I share my journey, insights, and the occasional coding hack—all fueled by curiosity and a love for continuous learning. When I'm not immersed in code, you'll likely find me exploring new tech trends, tinkering with side projects, or simply enjoying a great cup of coffee. Let's connect, collaborate, and build something amazing—one line of code at a time!