Memory Management in JavaScript: How It Works & Why It Matters
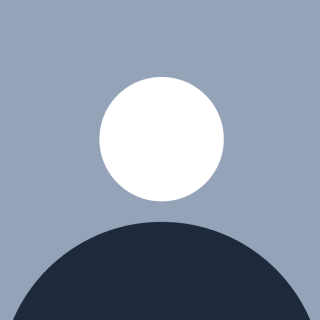
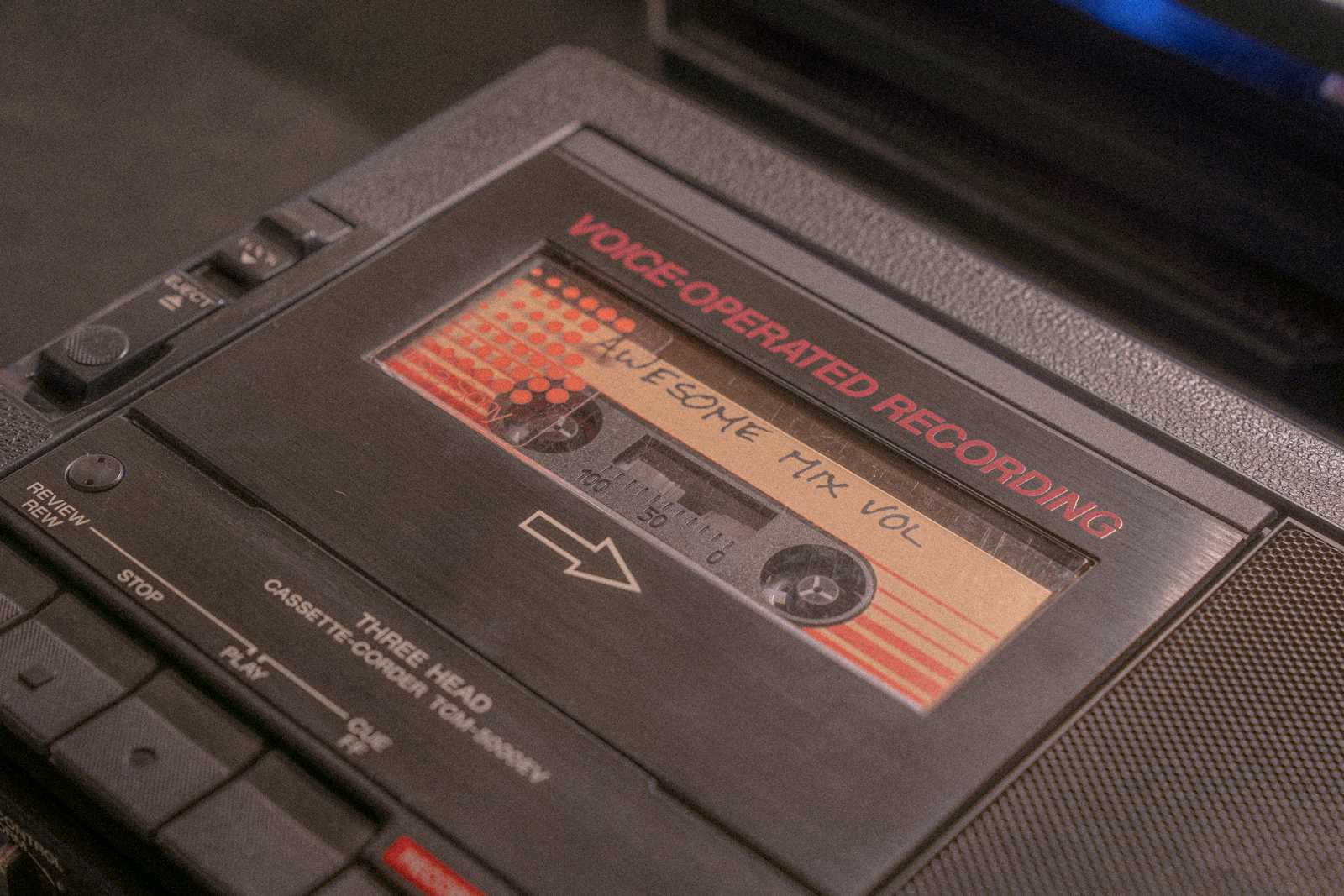
Memory management is crucial in JavaScript since it directly affects performance and efficiency. But unlike low-level languages where developers manually allocate and free memory, JavaScript handles this process automatically through garbage collection.
How Memory Works in JavaScript
1. Memory Allocation: When we declare variables, objects, or functions, JavaScript allocates memory for them.
2. Memory Usage: The allocated memory is used as long as there are references to it.
3. Garbage Collection: When a piece of memory is no longer needed (i.e., no references to it exist), the garbage collector frees it.
Types of Memory in JavaScript
• Stack Memory (for primitive values & function calls)
• Heap Memory (for objects, arrays, closures, etc.)
Common Memory Leaks to Watch Out For
1. Global Variables: Variables declared without let, const, or var remain in memory indefinitely.
2. Uncleared Timers & Intervals: setInterval running without being cleared can cause memory buildup.
3. Detached DOM Elements: If an element is removed from the DOM but still referenced in JavaScript, it stays in memory.
4. Closures Holding Unnecessary References: Functions capturing unnecessary variables in their scope can prevent memory from being freed.
How to Optimize Memory Usage
✅ Use const & let to avoid accidental global variables
✅ Manually clear timers with clearInterval() & clearTimeout()
✅ Remove event listeners when they are no longer needed
✅ Set references to null when objects are no longer needed
Understanding memory management helps prevent performance issues and makes JavaScript apps run smoother. 🚀
Subscribe to my newsletter
Read articles from Enofua Etue Divine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Enofua Etue Divine
Enofua Etue Divine
I'm a tech enthusiast . I'm a web developer and a student at Altschool Africa currently learning Frontend engineering ...