How to Set Up Room Database in Android

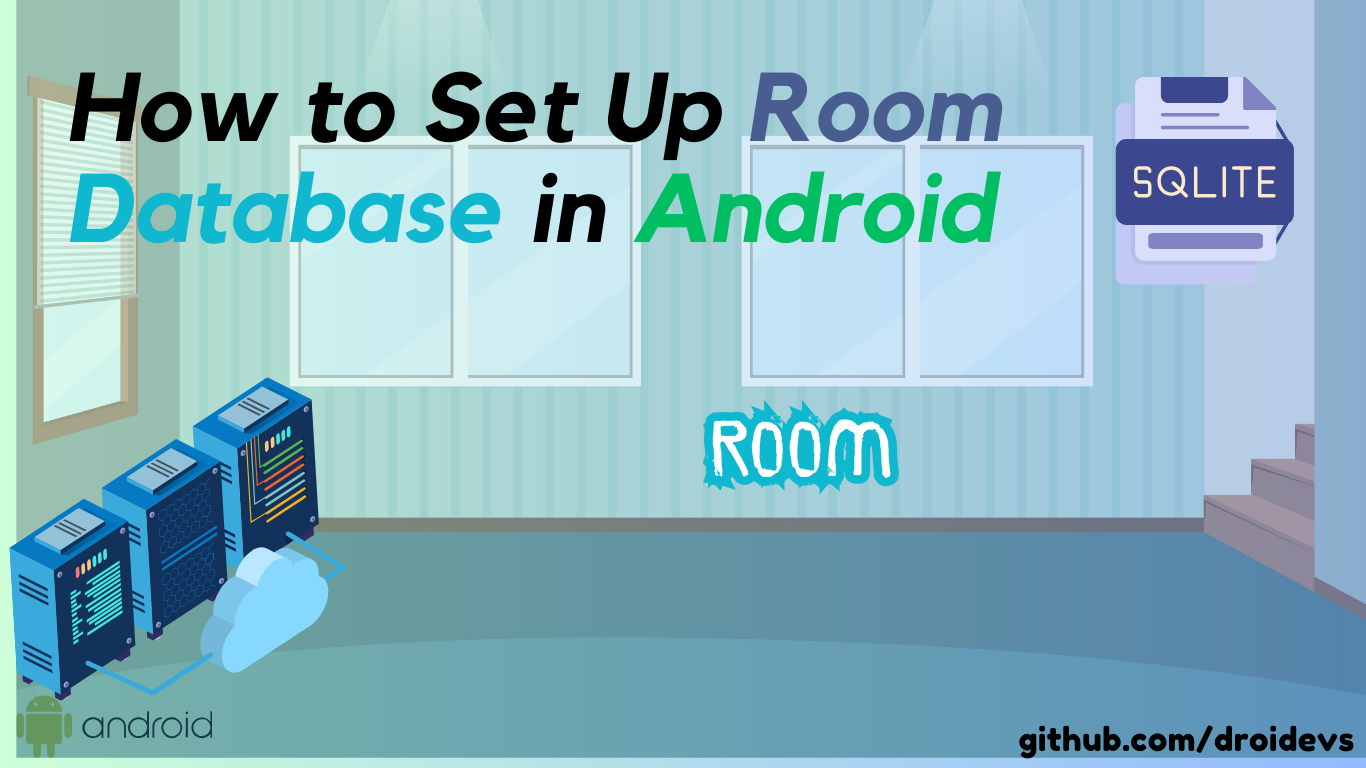
Introduction
Room is an abstraction layer over SQLite, making it easier to work with databases in Android. It's part of Android Jetpack and provides a robust framework for handling data persistence. In this article, we’ll walk through the process of setting up the Room Database in an Android project, focusing on a simple example that can be applied to real-world scenarios, like saving and managing user data in an app. This tutorial is aimed at beginners and intermediate developers who want to implement Room in their Android applications.
Step 1: Add Dependencies
First, we need to add the necessary dependencies to the build.gradle
files.
- Open the
build.gradle
(Project) file and ensure you have Google's Maven repository included:
allprojects {
repositories {
google()
mavenCentral()
}
}
- Next, open the
build.gradle
(App) file and add the Room dependencies inside thedependencies
block:
dependencies {
def room_version = "2.5.0" // Check for the latest version
implementation "androidx.room:room-runtime:$room_version"
annotationProcessor "androidx.room:room-compiler:$room_version" // For Java
kapt "androidx.room:room-compiler:$room_version" // For Kotlin
}
For Kotlin projects, ensure you also apply the Kotlin KAPT plugin at the top of your build.gradle
file:
apply plugin: 'kotlin-kapt'
Step 2: Create the Entity Class
Room uses annotated POJOs (Plain Old Java Objects) as database entities. These entities represent tables in the SQLite database.
Let’s create a simple User
entity to store user information.
Create a new Kotlin class, for example, User.kt
:
import androidx.room.Entity
import androidx.room.PrimaryKey
@Entity(tableName = "user_table")
data class User(
@PrimaryKey(autoGenerate = true) val id: Int = 0,
val name: String,
val age: Int
)
Here:
The
@Entity
annotation marks this class as a Room entity.The
@PrimaryKey
annotation marks the primary key of the table (in this case,id
).The
autoGenerate = true
option automatically generates unique values for theid
field when new rows are inserted into the database.
Step 3: Create the DAO (Data Access Object)
Room uses DAOs to interact with the database. DAOs define methods for database operations like insert, update, delete, and query.
Create a new interface called UserDao.kt
:
import androidx.room.Dao
import androidx.room.Insert
import androidx.room.Query
@Dao
interface UserDao {
@Insert
suspend fun insert(user: User)
@Query("SELECT * FROM user_table")
suspend fun getAllUsers(): List<User>
@Query("DELETE FROM user_table")
suspend fun deleteAll()
}
Here:
The
@Dao
annotation marks this interface as a DAO.The
@Insert
annotation defines a method to insert a user into the database.The
@Query
annotation defines custom SQL queries. In this case, one query to get all users and another to delete all users.
Step 4: Create the Room Database
Next, you need to create the database class that will provide the DAO.
Create a new abstract class called UserDatabase.kt
:
import android.content.Context
import androidx.room.Database
import androidx.room.Room
import androidx.room.RoomDatabase
@Database(entities = [User::class], version = 1)
abstract class UserDatabase : RoomDatabase() {
abstract fun userDao(): UserDao
companion object {
@Volatile
private var INSTANCE: UserDatabase? = null
fun getDatabase(context: Context): UserDatabase {
return INSTANCE ?: synchronized(this) {
val instance = Room.databaseBuilder(
context.applicationContext,
UserDatabase::class.java,
"user_database"
).build()
INSTANCE = instance
instance
}
}
}
}
Here:
The
@Database
annotation defines the database and the entities it holds (in this case,User
).The
getDatabase()
method creates a singleton instance of the database, ensuring that only one instance of the database is used in the app.
Step 5: Use Room in Your Activity or ViewModel
Now that everything is set up, you can start using Room to insert, query, and delete data from the database.
- Insert Data
In your activity or ViewModel, you can access the UserDao
to insert a new user:
class MainActivity : AppCompatActivity() {
private lateinit var userDatabase: UserDatabase
private lateinit var userDao: UserDao
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
userDatabase = UserDatabase.getDatabase(applicationContext)
userDao = userDatabase.userDao()
// Insert a new user
GlobalScope.launch(Dispatchers.IO) {
val user = User(name = "John Doe", age = 25)
userDao.insert(user)
}
}
}
- Query Data
You can query the data and update the UI as needed:
GlobalScope.launch(Dispatchers.IO) {
val users = userDao.getAllUsers()
withContext(Dispatchers.Main) {
// Update UI with users
Log.d("User List", users.toString())
}
}
Step 6: Handle Data in a ViewModel (Optional)
If you're following the MVVM architecture, you should place your data handling logic in a ViewModel
. Here’s an example of how to integrate Room with a ViewModel:
class UserViewModel(application: Application) : AndroidViewModel(application) {
private val userDao: UserDao = UserDatabase.getDatabase(application).userDao()
fun insertUser(user: User) {
viewModelScope.launch(Dispatchers.IO) {
userDao.insert(user)
}
}
fun getAllUsers(): LiveData<List<User>> {
val usersLiveData = MutableLiveData<List<User>>()
viewModelScope.launch(Dispatchers.IO) {
usersLiveData.postValue(userDao.getAllUsers())
}
return usersLiveData
}
}
Then, in your Activity
or Fragment
, you can observe the LiveData
:
userViewModel.getAllUsers().observe(this, Observer { users ->
// Update your UI with the list of users
})
Conclusion
By following these steps, you've successfully set up a Room Database in your Android project. You can now store and retrieve data efficiently in your app. Room handles all the complexities of working with SQLite, allowing you to focus on building great Android applications.
For more advanced topics, such as migrations or using Flow
for reactive programming with Room, check out the official documentation from Google. Happy coding!
Subscribe to my newsletter
Read articles from Mouad Oumous directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
