Day 16: JavaScript Error Handling - Try-Catch & Custom Error Messages ⬇️

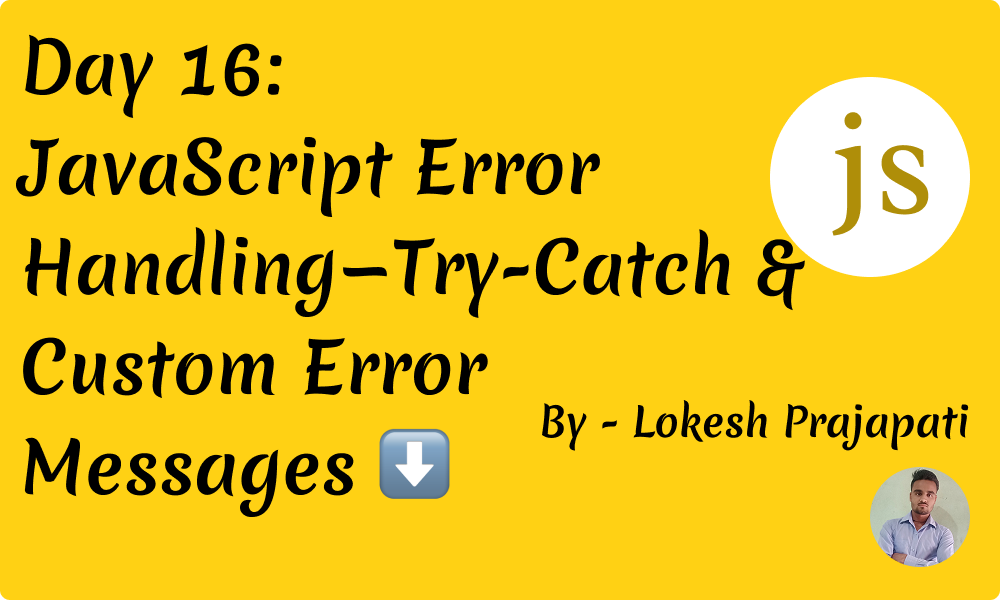
Errors are inevitable in programming. Whether you’re building a simple website or a complex application, understanding how to handle errors effectively can save you time and frustration. In JavaScript, the try-catch
block and custom error messages are powerful tools to manage unexpected issues and keep your code running smoothly. 🚨
In this post, we’ll break down:
What is Error Handling?
Understanding
try
,catch
, andfinally
Creating Custom Error Messages
Real-Life Examples for Better Understanding
Let’s dive in! 🚀
1. What is Error Handling? 🤔
Error handling is the process of catching and managing unexpected conditions in your code. Without proper error handling, your application can crash, break user experience, or display cryptic error messages.
JavaScript provides the try-catch
mechanism to gracefully handle errors and ensure your app remains stable.
2. Understanding try
, catch
, and finally
🔍
The try-catch
block in JavaScript helps handle errors that occur during code execution.
Syntax:
try {
// Code that may throw an error
} catch (error) {
// Handle the error here
} finally {
// (Optional) Code that runs regardless of success or failure
}
How Each Block Works:
✅ try
block: Contains code that may throw an error. ✅ catch
block: Captures the error and lets you handle it gracefully. ✅ finally
block: (Optional) Executes after try
or catch
, whether an error occurs or not. Useful for cleanup tasks. 🧹
Example:
try {
let result = 10 / 0; // Dividing by zero (Not an error in JS, but logic issue)
console.log(result); // Infinity
let data = JSON.parse('{name: "John"}'); // Invalid JSON
} catch (error) {
console.error("Oops! Something went wrong:", error.message);
} finally {
console.log("Execution completed! ✅");
}
Output:
Oops! Something went wrong: Unexpected token n in JSON at position 1
Execution completed! ✅
The
catch
block prevents the app from crashing by capturing the JSON parsing error. 🔥
3. Creating Custom Error Messages ✨
In some cases, JavaScript’s default error messages might not be enough. Creating custom error messages can improve clarity and help developers debug effectively.
Custom Error Example:
function validateAge(age) {
if (age < 18) {
throw new Error("Age must be 18 or older to proceed.");
} else {
console.log("Access granted! ✅");
}
}
try {
validateAge(15); // Throws custom error
} catch (error) {
console.error("Error:", error.message); // Custom error message
}
Output:
Error: Age must be 18 or older to proceed.
Custom error messages make it easier to identify the root cause of the issue. 🚨
4. Real-Life Examples 🌍
Example 1: Handling API Errors with fetch()
async function fetchData() {
try {
let response = await fetch("https://api.example.com/data");
if (!response.ok) {
throw new Error(`HTTP Error! Status: ${response.status}`);
}
let data = await response.json();
console.log("Data received:", data);
} catch (error) {
console.error("Error fetching data:", error.message);
} finally {
console.log("API request completed! 🌐");
}
}
fetchData();
✅ This code gracefully handles network errors, making the application more robust.
Example 2: Form Validation with Custom Error Handling
function submitForm(data) {
try {
if (!data.name) {
throw new Error("Name is required!");
}
if (!data.email.includes("@")) {
throw new Error("Invalid email format!");
}
console.log("Form submitted successfully! 🚀");
} catch (error) {
console.error("Form Submission Error:", error.message);
}
}
submitForm({ name: "", email: "userexample.com" });
Output:
Form Submission Error: Name is required!
5. Key Takeaways 📝
✅ Use try-catch
to manage runtime errors gracefully.
✅ Add custom error messages for better clarity.
✅ Include a finally
block for cleanup tasks.
✅ Handle API errors, form validation issues, and logical errors using these techniques.
By mastering JavaScript’s error handling techniques, you’ll create more reliable and professional applications. 🚀
Conclusion 🏁
Errors are part of every developer’s journey. Knowing how to handle them effectively with try-catch
and custom error messages can greatly improve your coding skills. Start implementing these techniques today, and watch your JavaScript applications become stronger and more resilient. 💪
Have any questions or insights? Drop them in the comments! 😊
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
🚀 JavaScript | React | Shopify Developer | Tech Blogger Hi, I’m Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. I’m currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Let’s learn and grow together. 💡🚀 #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode