Secure Your Apps: Disable Screenshots in Jetpack Compose

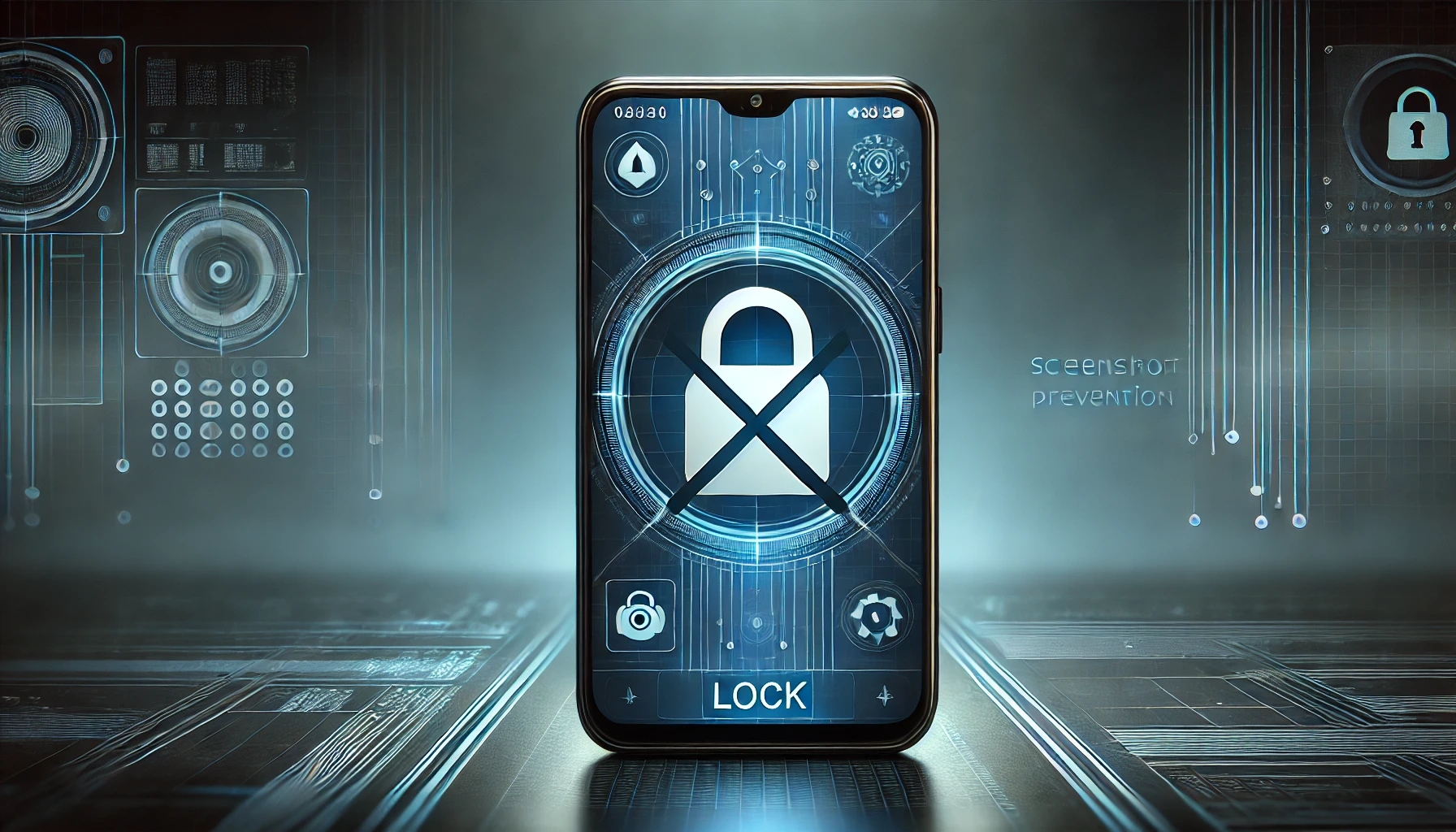
In mobile applications, preventing screenshots is a crucial security measure for protecting sensitive data, such as personal user information, financial details, or confidential documents. Android provides a way to block screenshots and screen recording using platform-specific flags. In this article, we'll explore how to implement screenshot prevention in a Jetpack Compose-based Android app.
Why Prevent Screenshots?
Certain applications require additional security measures to prevent unauthorized access or sharing of sensitive information. Common use cases include:
Banking and financial apps
Secure messaging apps
Exam and educational apps with restricted content
Medical or health-related applications
How to Prevent Screenshots in Jetpack Compose
Android provides a built-in way to disable screenshots using the FLAG_SECURE
window flag. Since Jetpack Compose relies on an Activity
or ComposeView
, we can apply this flag at the activity level.
Step 1: Apply FLAG_SECURE to the Activity
The easiest way to prevent screenshots in a Jetpack Compose app is by adding FLAG_SECURE
to the Window
in the activity’s onCreate
method:
import android.os.Bundle
import android.view.WindowManager
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import com.example.app.ui.theme.MyAppTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// Prevent screenshots and screen recording
window.setFlags(
WindowManager.LayoutParams.FLAG_SECURE,
WindowManager.LayoutParams.FLAG_SECURE
)
setContent {
MyAppTheme {
MyAppScreen()
}
}
}
}
This ensures that the entire activity is protected from screenshots and screen recording.
Step 2: Apply FLAG_SECURE to Specific Screens
If you don’t want to prevent screenshots across the entire app but only on specific screens, you can use LocalView
to access the window and set the flag within a composable.
import android.view.WindowManager
import androidx.compose.runtime.DisposableEffect
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.platform.LocalView
@Composable
fun SecureScreen(content: @Composable () -> Unit) {
val context = LocalContext.current
val window = (context as? ComponentActivity)?.window
val view = LocalView.current
DisposableEffect(view) {
window?.setFlags(
WindowManager.LayoutParams.FLAG_SECURE,
WindowManager.LayoutParams.FLAG_SECURE
)
onDispose {
window?.clearFlags(WindowManager.LayoutParams.FLAG_SECURE)
}
}
content()
}
You can now wrap specific screens with SecureScreen { YourComposableContent() }
to selectively apply the restriction.
Limitations and Considerations
While FLAG_SECURE
is effective at preventing screenshots and screen recording, it has some limitations:
Does not prevent hardware capture: Some devices with external capture cards or rooted systems can still bypass this restriction.
Does not block screenshots in multi-window mode: On some devices, screenshots may still be possible in multi-window mode.
Affects user experience: Blocking screenshots may be frustrating for users who want to take notes or share content for personal use.
Security vs. Usability: Always evaluate whether preventing screenshots is necessary for your use case.
Conclusion
Preventing screenshots in a Jetpack Compose app is simple using the FLAG_SECURE
window flag. You can apply it globally to the entire activity or selectively to specific screens based on your app’s security requirements. However, always balance security with user experience to ensure that your app remains accessible and user-friendly.
Would you implement screenshot prevention in your app? Let me know your thoughts!
Subscribe to my newsletter
Read articles from Chiranjeevi Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
