Integer Enums vs. String Enums in Rails: Which One Should You Use?

Table of contents
- 1. Understanding Integer Enums
- 2. Understanding String Enums
- 3. Real-World Example: String Enums in a Rails App
- 4. Querying with Enums in Rails
- 5. Preventing Issues with Integer Enums
- 6. Making the Right Choice: Integer or String Enums?
- Conclusion
- FAQ: Integer vs. String Enums in Rails
- 1. Can I use both integer and string enums in the same Rails model?
- 2. How can I get a list of all enum values?
- 3. How do I set a default value for an enum?
- 4. How do I query records based on an enum value?
- 5. Can I change the enum values later without breaking existing records?
- 6. How do I prevent invalid values from being saved to an enum column?
- 7. How do I update an enum value dynamically?
- 8. How do I use enum values in a dropdown in a Rails form?
- 9. How can I display a human-readable name for enum values?
- 10. When should I use an index for an enum column?
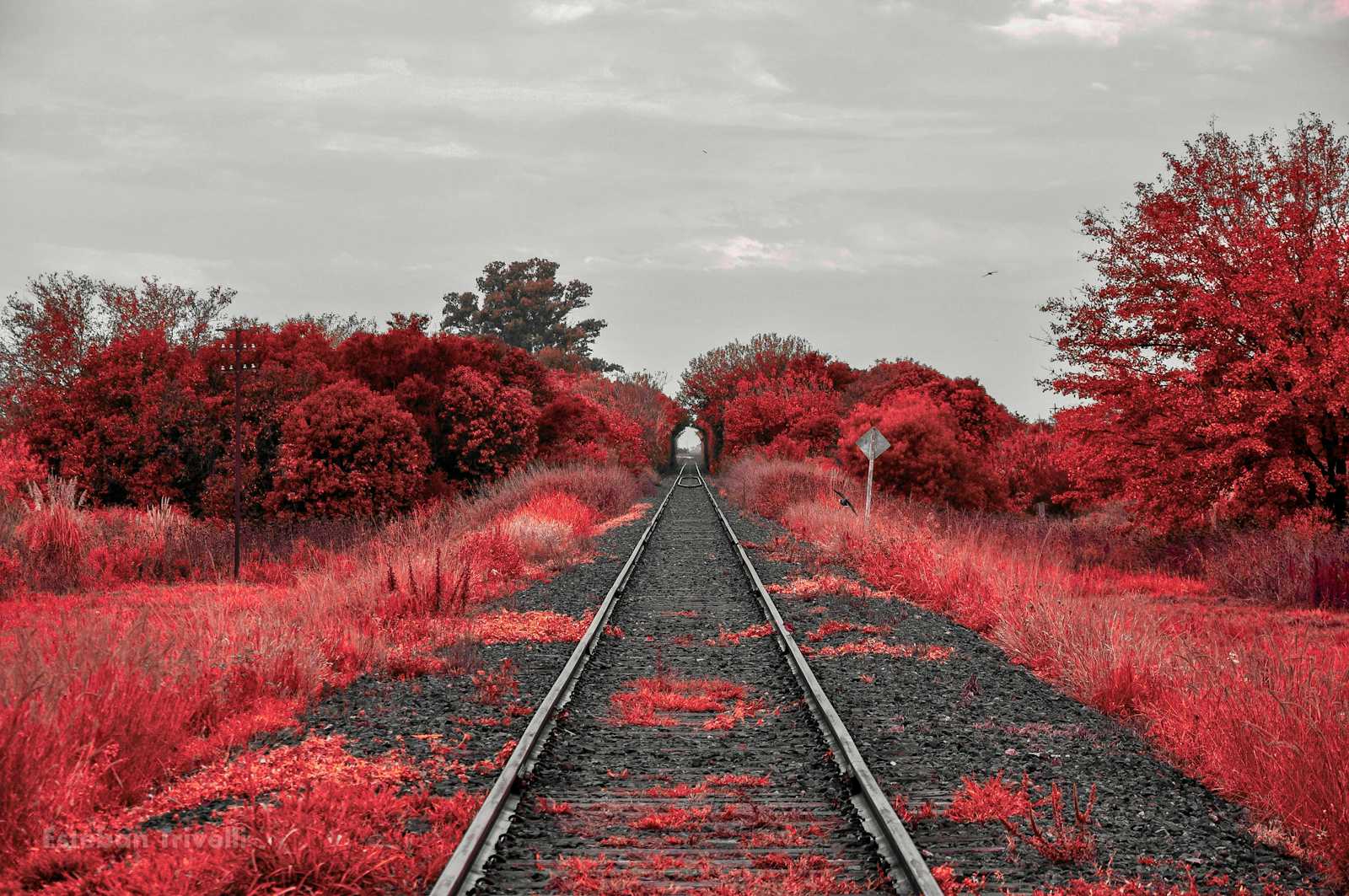
Ruby on Rails provides a powerful enum
feature that allows developers to store structured categorical data efficiently. When defining enums, a critical decision arises—should you use integer enums or string enums?
This article explores their differences, pros and cons, and real-world use cases. We’ll also analyze a practical Rails application using string enums, explaining why they were chosen and how they improve maintainability.
1. Understanding Integer Enums
Integer enums store an integer in the database but expose readable keys in your application.
Defining Integer Enums in Rails
Let’s say we have a Book
model where we track different editions:
class Book < ApplicationRecord
enum edition: { beta: 0, first: 1, second: 2, third: 3 }
end
Here’s how it works:
beta
is stored as0
,first
as1
,second
as2
, and so on.Rails provides helper methods:
book = Book.new(edition: :first)
book.edition # => "first"
book.first? # => true
Advantages of Integer Enums
✅ Efficient Storage – Integers take less space than strings.
✅ Faster Comparisons – Queries using indexed integers are slightly faster.
✅ Strict Constraints – Ensures that only valid enum values are stored.
Disadvantages of Integer Enums
❌ Loss of Readability – The database stores numbers (0
, 1
, 2
), making debugging harder.
❌ Risk of Data Corruption – Changing the order of enum values can break existing records.
2. Understanding String Enums
String enums store human-readable strings in the database instead of integers.
Defining String Enums in Rails
A better alternative is to use string enums, as done in a real Rails app:
class Book < ApplicationRecord
enum edition: %w[beta first second third fourth].index_by(&:itself), suffix: true, default: :beta
end
This setup ensures:
The database stores actual string values (
"beta"
,"first"
, etc.).The
suffix: true
option generates helper methods likebeta_edition?
.
book = Book.new(edition: "first")
book.edition # => "first"
book.first_edition? # => true
Advantages of String Enums
✅ Database Readability – Queries and logs clearly show "first"
, "second"
, etc.
✅ Future-Proof – Unlike integers, adding or reordering values won’t break records.
✅ Easier Debugging – Clearer data representation in the database and logs.
Disadvantages of String Enums
❌ Slightly More Storage – Strings take more space than integers.
❌ Potentially Slower Queries – Comparing strings is slower than comparing integers.
3. Real-World Example: String Enums in a Rails App
Let’s analyze the books
table schema from a real Rails app that uses string enums for theme
and edition
:
Schema
create_table "books", force: :cascade do |t|
t.string "title", null: false
t.string "edition", default: "beta", null: false
t.string "theme", default: "blue", null: false
t.index ["edition"], name: "index_books_on_edition"
end
Model Definition
class Book < ApplicationRecord
enum theme: %w[black blue green magenta orange violet white].index_by(&:itself), suffix: true, default: :blue
enum edition: %w[beta first second third fourth].index_by(&:itself), suffix: true, default: :beta
end
Why Use String Enums in This Case?
✅ Readability – Instead of numbers,
"beta"
and"blue"
are stored in the database.✅ Indexes Improve Performance – The
edition
field has an index for optimized queries.✅ Safer Future Changes – Adding a new edition (
"fifth"
) won’t affect old records.
4. Querying with Enums in Rails
Regardless of whether you use integer or string enums, querying works the same way.
# Find books with "second" edition
Book.where(edition: "second")
# Find books with "green" theme
Book.where(theme: "green")
For integer enums, the query looks like this:
SELECT * FROM books WHERE edition = 1;
For string enums, the query is:
SELECT * FROM books WHERE edition = 'second';
String queries are slightly slower, but the performance difference is negligible for most apps.
5. Preventing Issues with Integer Enums
A major risk of using integer enums is accidental reordering, which can lead to data misinterpretation.
The Problem: Accidental Reordering in Array Syntax
When using an array-based enum definition, the position of each value determines its corresponding integer in the database. If you reorder the array later, existing records can be misinterpreted.
Example of a potential issue:
class Book < ApplicationRecord
enum edition: [:beta, :first, :second]
end
beta
is stored as0
,first
as1
, andsecond
as2
in the database.If you later decide to reorder the array:
class Book < ApplicationRecord
enum edition: [:first, :beta, :second]
end
Now:
first
becomes0
,beta
becomes1
, andsecond
remains2
.
Problem:
All records that previously had beta (0)
will now incorrectly be interpreted as first (0)
, corrupting data integrity.
The Solution: Explicit Integer Mapping
To prevent this issue, always explicitly map integer values by using the hash syntax instead of an array. This ensures that values remain stable even if you change the order of keys in your code.
class Book < ApplicationRecord
enum edition: { beta: 0, first: 1, second: 2, third: 3 }
end
Now, even if you change the order of keys in the model, the database values will remain unchanged because each enum key is explicitly mapped to an integer.
class Book < ApplicationRecord
enum edition: { third: 3, beta: 0, first: 1, second: 2 }
end
Here, third
is still 3
, beta
is 0
, first
is 1
, and second
is 2
, preserving data integrity.
Best Practices for Using Integer Enums Safely
✅ Always use hash syntax ({ key: integer }
) instead of array syntax ([:key1, :key2]
).
✅ Never change existing mappings for deployed applications.
✅ If you must reorder or rename an enum value, write a data migration to update existing records.
✅ Index frequently queried enum columns to improve database performance.
By following these practices, you can avoid accidental data corruption and ensure stability in your Rails application.
6. Making the Right Choice: Integer or String Enums?
Factor | Integer Enums ✅ | String Enums ✅ |
Storage Efficiency | ✅ (More efficient) | ❌ (Uses more space) |
Database Readability | ❌ (Stores numbers) | ✅ (Stores strings) |
Performance | ✅ (Faster queries) | ❌ (Slightly slower) |
Risk of Data Corruption | ❌ (Changing order breaks data) | ✅ (Safe from order changes) |
Ease of Debugging | ❌ (Harder to read logs) | ✅ (Easier to understand) |
Future-Proofing | ❌ (Risky) | ✅ (More maintainable) |
Best Practices
Use integer enums if performance and storage efficiency are your top priority.
Use string enums for better readability and long-term stability, especially if you plan to change enum values.
If using integer enums, always explicitly map values to prevent accidental shifts.
Conclusion
In many Rails applications, string enums are a safer, more maintainable choice—especially when values are likely to change. This is why the Book
model from the real-world example uses string enums for both edition
and theme
.
However, integer enums are better suited for performance-critical applications where storage and indexing matter. By understanding their strengths and weaknesses, you can choose the best option for your Rails app. 🚀
FAQ: Integer vs. String Enums in Rails
1. Can I use both integer and string enums in the same Rails model?
Yes! You can define some enums as integers and others as strings within the same model.
class Book < ApplicationRecord
enum edition: { beta: 0, first: 1, second: 2 }
enum theme: %w[black blue green].index_by(&:itself)
end
Here, edition
is an integer enum, while theme
is a string enum.
2. How can I get a list of all enum values?
Use .keys
to get the enum options as an array.
Book.editions.keys # => ["beta", "first", "second"]
Book.themes.keys # => ["black", "blue", "green"]
For integer enums, Rails automatically converts keys to strings.
3. How do I set a default value for an enum?
Use the default:
option when defining the enum.
class Book < ApplicationRecord
enum edition: %w[beta first second].index_by(&:itself), default: :beta
end
book = Book.new
book.edition # => "beta" (default value)
4. How do I query records based on an enum value?
Use where
to find records with a specific enum value.
Book.where(edition: "first") # For string enums
Book.where(edition: 1) # For integer enums
5. Can I change the enum values later without breaking existing records?
For integer enums: You cannot safely change the order without breaking old records. Always explicitly map values:
enum edition: { beta: 0, first: 1, second: 2 }
For string enums: You can rename or add new values safely because the database stores readable values.
6. How do I prevent invalid values from being saved to an enum column?
Rails automatically validates enums, so you cannot assign an invalid value.
book = Book.new(edition: "unknown")
book.valid? # => false (Rails will reject it)
If you try to save an invalid value, Rails will raise an error.
7. How do I update an enum value dynamically?
Simply assign a new value to the enum column.
book = Book.first # has edition = first
book.edition = "second"
book.save!
For integer enums, you can also use the numeric value:
book.edition = 2 # "second"
book.save!
Or if you are using suffix
as such enum edition: %w[beta first second third fourth].index_by(&:itself), suffix: true, default: :beta
then you can simply do:
book.second_edition!
8. How do I use enum values in a dropdown in a Rails form?
For integer enums:
<%= form.select :edition, Book.editions.keys.map { |key| [key.humanize, key] } %>
For string enums:
<%= form.select :theme, Book.themes.keys.map { |key| [key.humanize, key] } %>
This ensures human-readable dropdown options.
9. How can I display a human-readable name for enum values?
Use .humanize
to format the enum key.
Book.editions.keys.map(&:humanize)
# => ["Beta", "First", "Second"]
For custom translations, use I18n.t
in your locale file:
en:
activerecord:
attributes:
book:
editions:
beta: "Beta Edition"
first: "First Edition"
Then, display it in views:
<%= t("activerecord.attributes.book.editions.#{book.edition}") %>
10. When should I use an index for an enum column?
Indexing an enum column improves query performance, especially for filtering large datasets.
t.index ["edition"], name: "index_books_on_edition"
✅ Use an index if you frequently query by the enum value (where(edition: "first")
).
❌ Skip the index if you rarely query by this column.
Subscribe to my newsletter
Read articles from Chetan Mittal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mittal
Chetan Mittal
I stumbled upon Ruby on Rails beta version in 2005 and has been using it since then. I have also trained multiple Rails developers all over the globe. Currently, providing consulting and advising companies on how to upgrade, secure, optimize, monitor, modernize, and scale their Rails apps.