What Exactly Are PWAs?

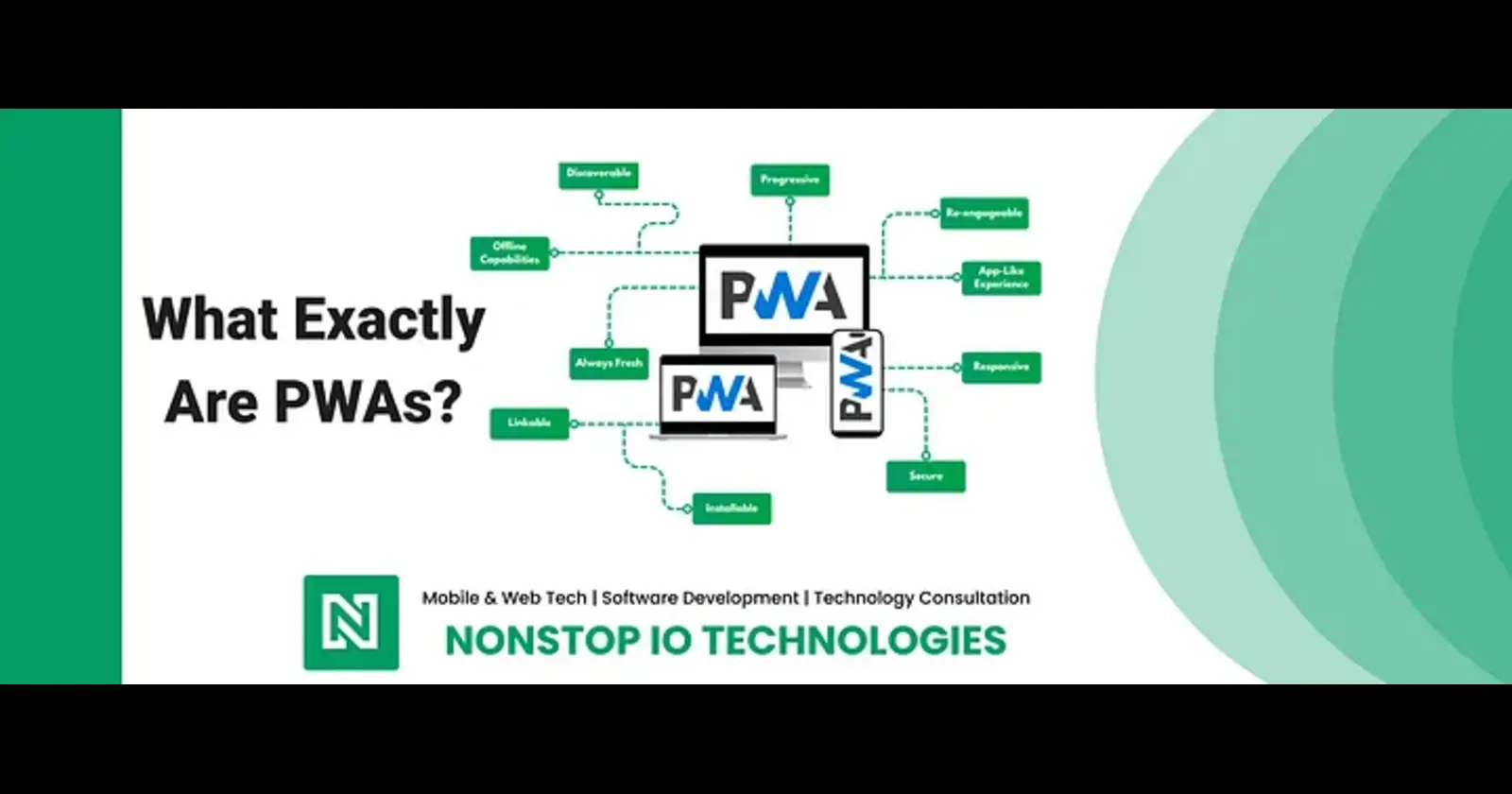
Progressive Web Apps (PWAs) are a set of best practices to make a web application function similarly to a native mobile app. The term “progressive” emphasizes that these applications are progressively enhanced, meaning they work for every user, regardless of their browser choice, because they are built with progressive enhancement as a core principle. PWAs leverage modern web capabilities to deliver an app-like experience to users. They aim to provide a reliable, fast, and engaging user experience, regardless of the network condition.
Key characteristics of PWAs include:
Progressive: They are built to work on any browser and enhance their functionality based on the capabilities of the user’s device.
Responsive: They adapt to different screen sizes and orientations, making them usable on desktops, tablets, and smartphones.
Offline Capabilities: Through service workers, PWAs can work offline or in low-network conditions.
App-Like Experience: They offer a seamless, app-like user experience with smooth interactions and navigation.
Always Fresh: Service workers help keep the content up-to-date, ensuring that users always see the latest version of the app.
Secure: They are served over HTTPS to protect data integrity and privacy.
Discoverable: Thanks to standard web technologies, PWAs can be indexed by search engines and are easily discoverable.
Re-engageable: They support features like push notifications to keep users engaged.
Installable: Users can add PWAs to their home screens, providing quick access without needing an app store.
Linkable: They can be shared easily via URLs without requiring complex installation processes.
Image -Key characteristics of PWA include
How to Implement a PWA in React
Implementing a PWA in a React application involves several steps:
1. Set Up a New React Project
You can create a new React project using Create React App (CRA), which provides an option to set up a PWA out of the box.
npx create-react-app my-pwa --template cra-template-pwa
This command sets up a new React project with PWA support.
2. Configure the Service Worker
The service worker is a script that runs in the background and helps your app work offline. Create React App sets up a basic service worker for you, but you can customize it as needed.
// src/service-worker.js
const CACHE_NAME = "my-pwa-cache-v1";
const urlsToCache = ["/", "/index.html", "/static/js/bundle.js", "/static/js/0.chunk.js", "/static/js/main.chunk.js"];
self.addEventListener("install", event => {
event.waitUntil(
caches.open(CACHE_NAME).then(cache => {
return cache.addAll(urlsToCache);
})
);
});
self.addEventListener("fetch", event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
3. Configure the Web App Manifest: The manifest file provides metadata about your web app, like its name, description, icons, and how it should behave when installed on a device.
{
"short_name": "MyPWA",
"name": "My Progressive Web App",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
],
"start_url": "/",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
Include this manifest file in your public
directory and link to it in your HTML.
<!-- public/index.html -->
<link rel="manifest" href="/manifest.json">
4. Register the Service Worker in Your React App
By default, the Create React App registers the service worker in production mode only. You can register it in your src/index.js
file.
// src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import * as serviceWorker from './serviceWorker';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// If you want your app to work offline and load faster, you can change
// unregister() to register() below. Note this comes with some pitfalls.
// Learn more about service workers: https://bit.ly/CRA-PWA
serviceWorker.register();
5. Add Icons and a Splash Screen
To make your PWA look more like a native app, add icons and a splash screen. Icons are referenced in the manifest file, and you can add a splash screen by configuring the manifest and CSS.
/* src/App.css */
@media screen and (min-width: 320px) and (min-height: 480px) {
/* iPhone 5 */
.splash-screen {
background-image: url('/logo192.png');
}
}
6. Test Your PWA
Finally, test your PWA to ensure it meets all requirements. You can use tools like Lighthouse (built into Chrome DevTools) to audit your PWA and see what areas need improvement.
Real-Life Examples of Effective PWA Implementation
Several prominent companies have successfully adopted Progressive Web Apps (PWAs) to enhance user experience and achieve significant business benefits. Here are a few notable examples:
1. Pinterest
Challenge: Pinterest faced challenges with slow load times and high bounce rates on mobile web.
Solution: Pinterest implemented a PWA to address these issues. The PWA was designed to be fast, responsive, and engaging, with offline capabilities and push notifications.
Impact:
Performance: The PWA significantly improved load times, reducing the time to interact from 23 seconds to 5.6 seconds.
User Engagement: Pinterest saw a 40% increase in time spent on the site.
Revenue: User-generated ad revenue increased by 44%, demonstrating the effectiveness of the PWA in driving user engagement and monetization.
2. Twitter Lite
Challenge: Twitter needed a solution to offer a fast and engaging experience for users in regions with limited network conditions.
Solution: Twitter launched Twitter Lite, a PWA designed to be lightweight and perform well even on slow or unreliable networks.
Impact:
Data Usage: Twitter Lite reduced data usage by 70%, making it accessible to users with limited data plans.
Engagement: There was a 20% increase in tweets sent and a 15% increase in time spent on the site.
Performance: The app improved performance, leading to faster load times and a smoother user experience.
3. Flipkart
Challenge: Flipkart, one of India’s largest e-commerce platforms, aimed to enhance the mobile shopping experience for users with slow internet connections.
Solution: Flipkart developed a PWA that provided a fast, app-like experience with offline capabilities and push notifications.
Impact:
Performance: The PWA reduced the average load time by 50%.
Conversions: Flipkart saw a 70% increase in conversions for users who visited the site via the PWA.
Engagement: The app experienced a significant rise in user engagement and retention.
4. MakeMyTrip
Challenge: MakeMyTrip, an Indian travel company, wanted to improve the mobile experience for users booking travel in areas with varying network conditions.
Solution: They launched a PWA to provide a fast and reliable booking experience with offline access and push notifications.
Impact:
Performance: The PWA improved the speed of the site, leading to faster booking processes.
User Engagement: MakeMyTrip saw a substantial increase in user engagement and interaction.
Conversion Rates: There was a noticeable increase in conversion rates due to the improved user experience.
5. Trivago
Challenge: Trivago sought to enhance the mobile search experience for hotel bookings, especially for users on slow or unreliable networks.
Solution: Trivago implemented a PWA to provide a fast, responsive search experience with offline capabilities and push notifications.
Impact:
Performance: The PWA improved load times and responsiveness, leading to a better user experience.
Engagement: Users spent more time searching and interacting with the app.
Conversion: The improved experience contributed to higher conversion rates for hotel bookings.
Conclusion
These real-life examples highlight the effectiveness of PWAs in addressing various challenges faced by businesses. By adopting PWAs, companies can enhance performance, improve user engagement, and achieve significant business outcomes. Whether it’s reducing load times, increasing user interaction, or boosting conversion rates, PWAs offer a robust solution for creating a superior user experience across different devices and network conditions.
Happy Coding 🚀
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.