Fullstack Boiler Code

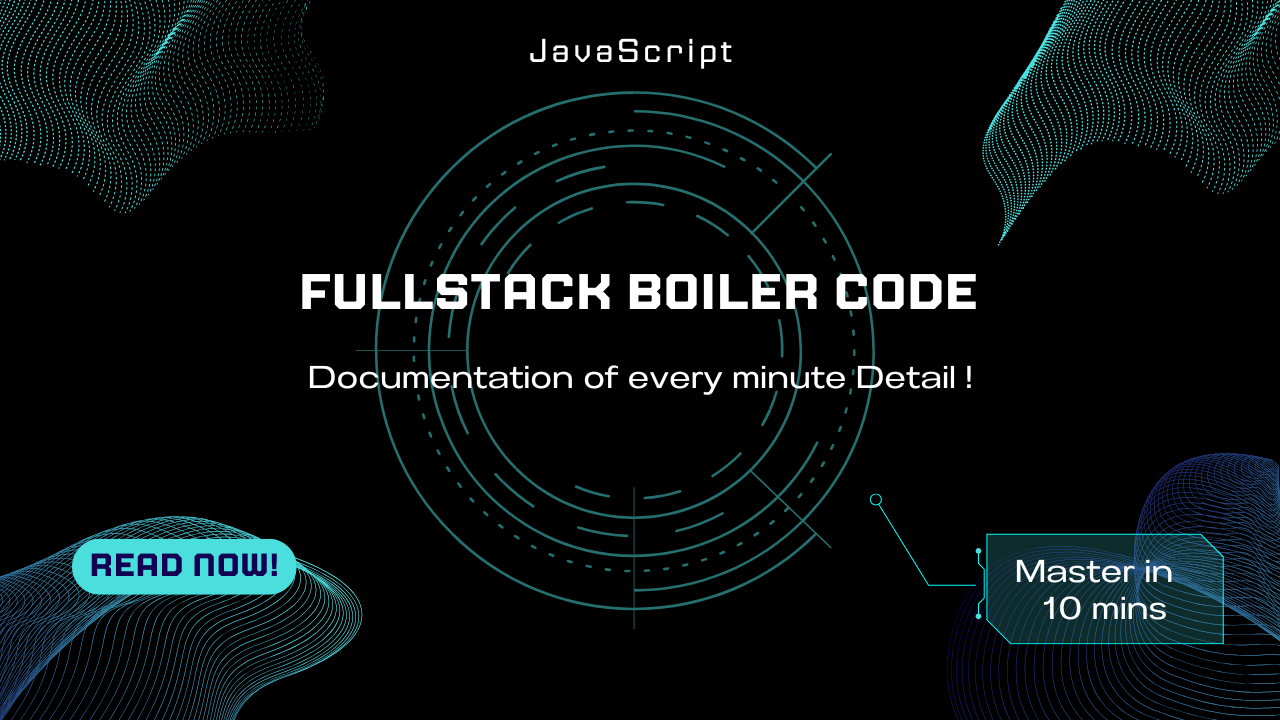
Introduction
Building a full-stack application can be overwhelming, especially when dealing with complex code structures and integrations. In this blog, we break down a full-stack boilerplate with meticulous documentation, covering every minute detail. Whether you're a beginner or an experienced developer, this guide will help you understand the architecture, streamline development, and save valuable time. Let’s dive deep into the essentials of a well-structured full-stack codebase! 🚀
We can run through (node,bun......)
(->) means code...
(S)steps…
--[ Terminal ]--
(S1) npm init
-[Initializes the package - (npm- Node Package Manager)]
(S2) press enter * 7 times
(S3) package.json file must be created
(S4) create new file -> index.js
-[To bring 3rd party libraries in our code we have 2 methods/syntax
-> 1(require) , 2(import)] -[if you are using (require) syntax then no problem you can use it directly...]
-'*'[but if are using (import) syntax then... go-to -> package.json -> write below "main"
-> "type": "commonjs" or "module"
("commonjs" means (require) syntax) and "module" means import syntax]
(S5) package.json -> write below "main" -> "type": "module",
-[if you want to know Summary("Kundli") of any project then go-to ".env" file or ".env.sample" (Environment variables) in this file we can know all the critical infrastructure eg.which database, mailing service is used..etc]
-[where to find? -> check 'Render' site]
-[to keep all the sensitive data safe we use .env]
(S6) Express JS - it makes easier to code
--[ Terminal ]--
-> npm install express
-[we get all the code in the form of 'node_modeules']
(S7) To know which packages we have installed...
-> package.json and the check in "dependencies"
-[to know about 'Versioning check out https://semver.org]
(S8) Copy sample code from 'https://expressjs.com/' in 'index.js'
->
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => { res.send('Hello World!') })
app.listen(port, () => { console.log(`Example app listening on port ${port}`) })
(S9) We are using 'import' syntax so remove (line 1)const express = require('express')
and write -> import express from "express")
-[const app = express() //powers of express are transfered in 'app'(initialize)]
-[common ports -> 3002,4000,5000,3000,5173,8080,8000] -[don't use ports -> 80,443,23]
-[.get & .send...to ren .get request type 127.0.0.1:3000(port no.)]
-[for speed run
-> app.get() '(first define route and what & when to do..(kya karna ahi aur kab karna hai))]
-> 'syntax'
-> app.get("/Keyur" , () => {})
-> app.get("/Keyur" , (request, response) => {res.send("Keyur!"); })
-> routes always starts with "/" (eg-> .app.get("/Keyur" , () => {}) )
(S10)-> npm i -D nodemon
-[monitors node and relodes it]
-[there are 2 types of dependencies 1(project) & 2(developer) dependencies]
(S11) Every time we do use node to run the code hence we use 'scripts'...
-[we can make changes in -> "scripts": {"start": "nodemon index.js"},]
-[now we can write -> npm run start -(now nodemon monitors node and reloads it)]
-[npm run (script name)]
(S12) -[we do not write 'port' in 'index.js' ]
-> go-to '.env' -(in '.env' always write in ALL CAPITAL) -> PORT = 3000
-[to do this we have to import it in 'index.js', but we directly cannot import '.env']
-[hence we use package - "dotenv"] checkout dotenv documentation
-> npm i dotenv
-> now import in 'index.js'
-> import dotenv from "dotenv"
-> dotenv.config()
-> console.log(process.env);
-[according to 'dotenv' documentation]
-[we can also write console.log(process.env.PORT); ]
(method)
-> now in (line19) -> app.listen(port, () => {})
->[instead of 'port' write 'process.env.PORT'
in (line19)]
-> app.listen(process.env.PORT, () => {})
...-(like this)
-> then delete "console.log(process.env.PORT);"
-[we can use above method but we should use the best practice given below(S13)]
(S13) (Best Practice)
-> delete "console.log(process.env.PORT);"
-> keep 'app.listen(port, () => {})'
as it is
-> we can use it variably..so in 'const port' make changes like
-> const port = process.env.PORT || 3000
-[above line means you will find port in 'process.env' if you don't find then 3000 is there!]
- requests [client -> frontend -> backend]
-[to avoid client directly talking to backend, we use term called CORS]
-[CORS - Cross-origin Resource Sharing] errors are solved in backend
(S14)
-> npm i cors
-> import cors from "cors";
-[one way to to use this is write in 'index.js']
->
app.use( cors({ origin:"
https://localhost:3000
",
credentials: true,
methods:['GET','POST','DELETE','OPTIONS'],
allowedHeaders:['Content-Type', 'Authorization'] }) );
// origin means from where incoming request should be allowed
(S15) -[if you want to send json format data]
-> app.use(express.json())
in 'index.js'
-[if something like '%20'(space)(url encoding) is present in url and you don’t want it then we use ->]
-> app.use(express.urlencoded({extended:true}))
(S16) -[backend(logical data) -> to save this data we use Database(mongodb)]
-[we use mongoose package to talk with database (mongodb)]
-[it is also called ORM(Object Relational Mapper) or ODM(Object Data Modeling) ...depends on database]
-> npm i mongoose
-[check mongoose documentation]
-> create a new folder "utils" (to keep all the utilities like mail,DB etc... )
-> create a new file "db.js" in "utils" folder
in "db.js"
->import mongoose from "mongoose";
->mongoose.connect(url)
-[goto MongoDB atlas]
-[after database we need -> ip_whitelisting and -> username-password]
(S17) -> add mongodb url in -> '.env' file
-> MONGO_URL=
-> BASE_URL=
...// and then make changes in in 'index.js'
(S18) -> mongoose.connect(process.env.MONGO_URL);
...// make changes in in 'db.js'
-> import dotenv from "dotenv"
-> dotenv.config()
-> //export a function that connects a DB
→const db = () => { mongoose .connect(process.env.MONGO_URL) .then(() => { console.log("connected to mongoDb"); })
→ .catch((err) => {console.log("Error connecting to mongoDb"); }); }
-> export default db;
(S19)
-> connect db in 'index.js'
-> import db from "./utils/db.js";
...//sometimes we have use '.js' at the end of the file
-> db();
...//connect to db
----[NOW YOU CAN START YOUR OWN PROJECT]----
--[Practice PROJECT]--
-> create a folder named "model" -> create a file named "User.model.js"
->import mongoose from "mongoose";
...// in "User.model.js"
->const userSchema = new mongoose.Schema()
->mongoose.model("User", userSchema)
->export default User
...// default boiler code...
-[to separate controller and routes]- ..// best practice
-> create a new folder -> controller -> create a new file -> user.controller.js
-> const registerUser = async (req, res) => { res.send("registered"); };
export {registerUser}
...// syntax
-> create a new folder -> routes -> create a new file -> user.routes.js
->import express from 'express'
->const router = express.Router()
->export default router
...// syntax
->import userRoutes from "./routes/user.routes.js"
…//import all routes in 'index.js'
->//user routes
->app.use("/api/v1/users", userRoutes)
...// anything that comes after '/api/v1/users' will be transferred to userRoutes
With a well-documented boilerplate, you can streamline your development process and focus on building great features rather than reinventing the wheel. I hope this breakdown helps you navigate full-stack development more efficiently. Feel free to share your thoughts or ask any questions—happy coding! 🚀
Subscribe to my newsletter
Read articles from Keyur Shelke directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
