JavaScript Q&A: alert, prompt, and confirm #5

Table of contents
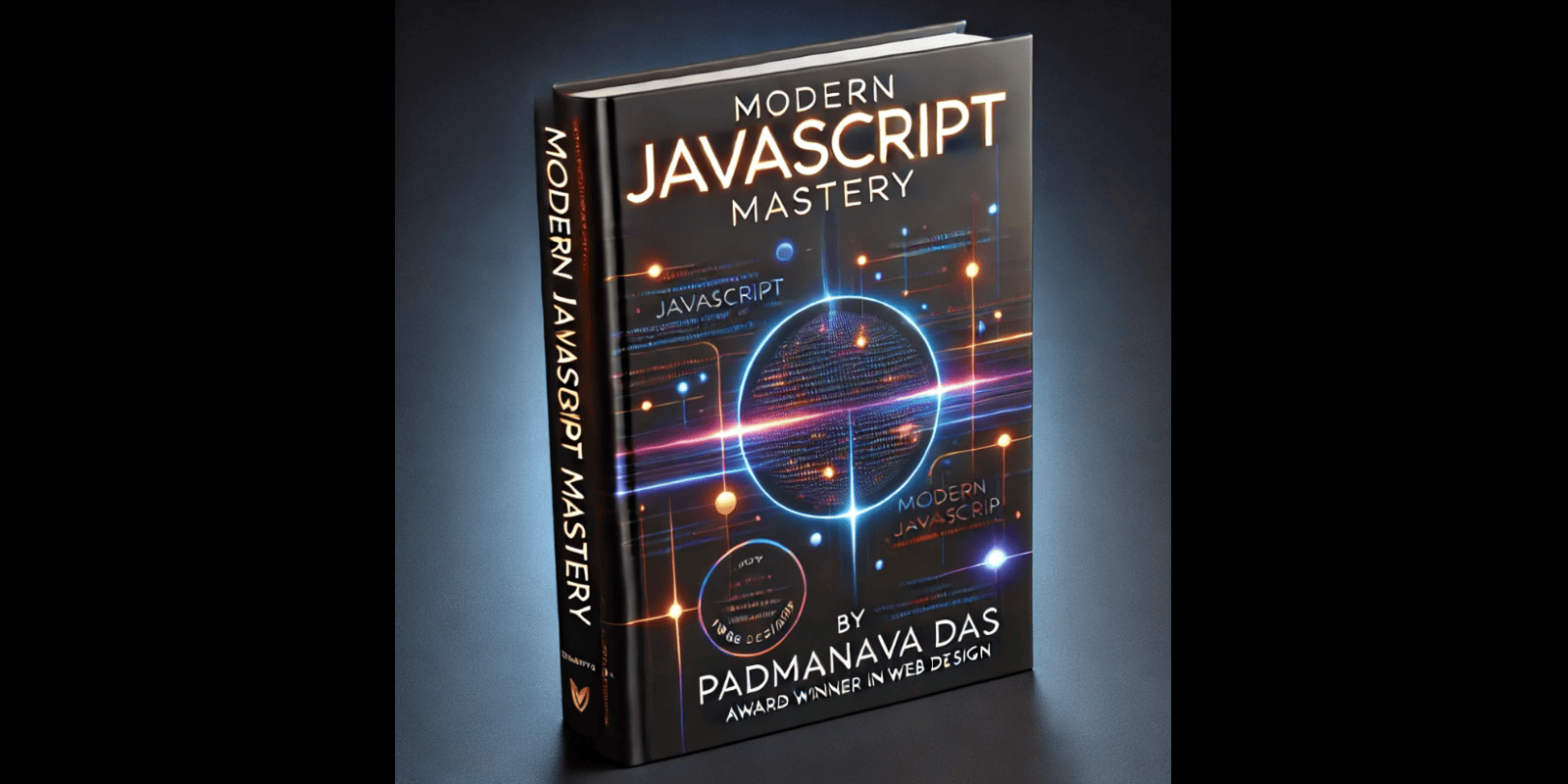
Hello Friends!
Kamon acho!
JavaScript Interaction Q&A: alert
, prompt
, and confirm
Below is a collection of questions and answers designed to deepen your understanding of JavaScript’s interaction functions, along with code snippets for clarity.
1. What is a modal window, and why is it called that?
Ans:
A modal window is a popup that forces the user to interact with it before continuing to use the rest of the page. For example:
alert("This modal window blocks further interaction until dismissed.");
It’s “modal” because it creates a mode where the underlying page is inactive.
2. How does the alert
function work in JavaScript?
Ans:
The alert
function displays a message in a modal dialog and halts script execution until the user clicks “OK.”
alert("This is an alert message!");
// Code here will execute only after the alert is closed.
3. What are the two arguments accepted by the prompt
function?
Ans:
The prompt
function accepts:
A message (string) to display.
An optional default value for the input field.
let response = prompt("What is your favorite color?", "Blue");
console.log("User's favorite color is:", response);
4. What happens when a user presses "Cancel" in a prompt
dialog?
Ans:
If the user clicks "Cancel," the prompt
returns null
:
let input = prompt("Enter a value:", "Default");
if (input === null) {
console.log("User canceled the prompt.");
}
5. Why is it recommended to always provide a default value in prompt
when using Internet Explorer?
Ans:
Without a default value, Internet Explorer might display "undefined"
as the default text. Providing a default value (even an empty string) avoids this issue:
let testInput = prompt("Type something:", ""); // Prevents 'undefined' from appearing
6. What does the confirm
function return when the user presses "OK" and when they press "Cancel"?
Ans:
The confirm
function returns:
true
if the user clicks “OK.”false
if the user clicks “Cancel.”
let userDecision = confirm("Do you agree?");
console.log("User's decision:", userDecision); // true for OK, false for Cancel
7. What are the two main limitations of alert
, prompt
, and confirm
dialogs?
Ans:
They have two key limitations:
Fixed Location: The browser controls where these dialogs appear.
alert("I always appear centered by default!");
No Custom Styling: Their appearance cannot be altered with CSS.
// No CSS can change the style of these dialogs. alert("I have a default design that cannot be styled.");
8. How does a confirm
dialog differ from an alert
dialog?
Ans:
An
alert
shows a message and has only an "OK" button.A
confirm
provides "OK" and "Cancel" buttons, returning a boolean value.
// Alert example:
alert("This is just an alert.");
// Confirm example:
let decision = confirm("Do you want to proceed?");
console.log("Decision:", decision);
9. What will be the output of the following code if the user enters "John" in the prompt?
Ans:
let answer = prompt("Enter your name:", "Guest");
alert(`Welcome, ${answer}!`);
If the user types "John" and clicks OK, the output will be:
// Output in the alert dialog:
"Welcome, John!"
Thank you!
Subscribe to my newsletter
Read articles from Padmanava Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Padmanava Das
Padmanava Das
From Republic of Bharat