Convert PowerPoint to HTML in Python – Fast & Easy Guide

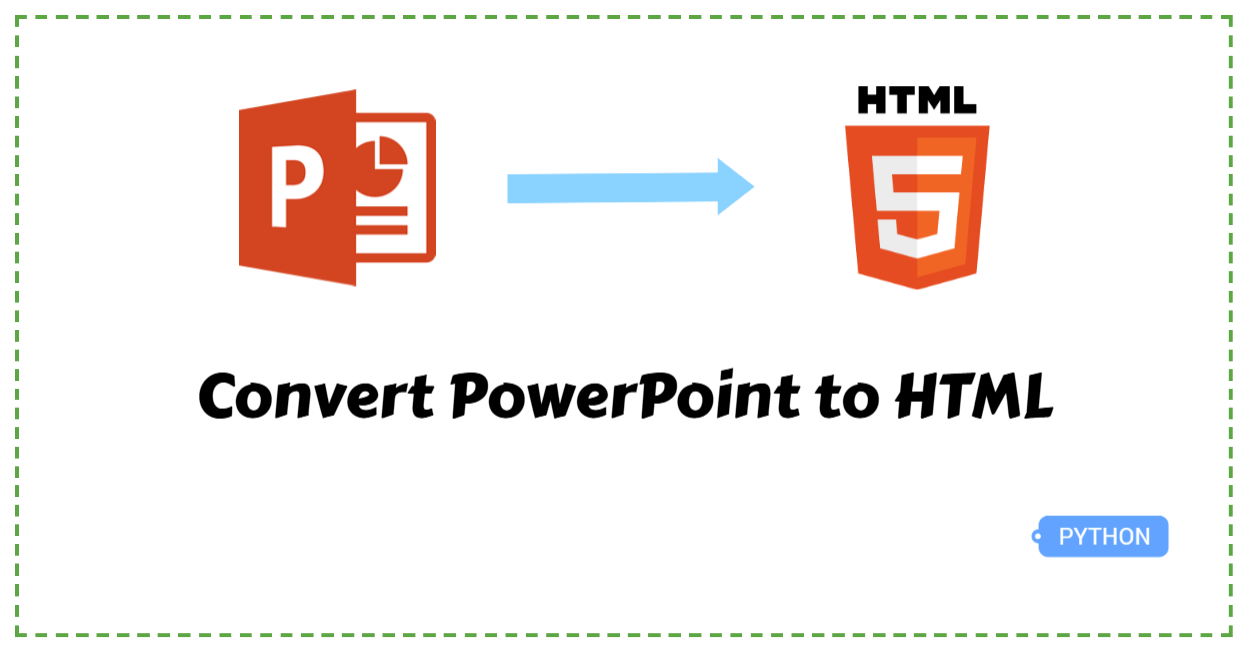
PowerPoint is a powerful tool for creating presentations, but sharing them on the web can be challenging. Not all users have PowerPoint installed, and embedding a presentation in a website often leads to compatibility issues. Exporting PowerPoint to HTML solves these problems by making slides easily viewable in any browser without additional software. HTML can seamlessly display text, images, and animations, ensuring a smooth presentation experience online. In this article, we’ll explore how to use Python to convert PowerPoint to HTML, helping you streamline the process and improve accessibility.
Python Libraries to Convert PowerPoint to HTML
There are several Python libraries that can help convert PowerPoint to HTML, making the process more efficient. Some popular open-source options include python-pptx, LibreOffice, and Unoconv. However, each of these solutions comes with certain limitations:
python-pptx does not support direct conversion. It can only extract elements such as text and images, requiring users to manually generate the HTML structure.
LibreOffice is not a standalone Python library; it must be installed on the system to function.
Unoconv relies on LibreOffice, meaning it inherits the same dependency requirements.
For a more efficient and independent solution, I recommend Spire.Presentation, a professional Python library that does not require third-party dependencies or even MS Office installation. Its intuitive API and extensive features make it an excellent choice for exporting PowerPoint to HTML.
In this article, we will demonstrate how to use Spire.Presentation for this task. You can install it easily with the following pip command: pip install Spire.Presentation
Python Convert PowerPoint Presentations to HTML in 3 Steps
When sharing a PowerPoint presentation online, converting it to HTML ensures better accessibility and compatibility across devices. With Spire.Presentation, the process is simplified into just three steps: create a presentation, load the source file, and save it as an HTML document using the Presentation.SaveToFile() method. Let’s go through each step in detail.
Steps to convert a PowerPoint presentation to HTML:
Create an object of the Presentation class.
Load a source PowerPoint file using the Presentation.LoadFromFile() method.
Save the PowerPoint file as HTML through the Presentation.SaveToFile() method.
Here is an example of how to convert an entire PowerPoint presentation to HTML:
from spire.presentation.common import *
from spire.presentation import *
# Create a Presentation instance
ppt = Presentation()
# Load a PowerPoint document
ppt.LoadFromFile("/pre1.pptx")
# Save the document to HTML format
ppt.SaveToFile("/PretoHTML.html", FileFormat.Html)
ppt.Dispose()
Convert PowerPoint Slides to HTML in Python
Sometimes, you may only need to share or display a specific slide from a PowerPoint presentation instead of converting the entire file. With Spire.Presentation, you can achieve this easily using the ISlide.SaveToFile() method. This allows you to convert a slide into an HTML format after accessing the specified slide. Below, we’ll walk through the steps and provide code examples.
Steps to convert a PowerPoint slide to HTML:
Create a Presentation instance.
Read a PowerPoint presentation from files using the Presentation.LoadFromFile() method.
Get a specified slide through the Presentation.Slides[] property.
Save the slide as HTML with the ISlide.SaveToFile() method.
Below is the Python example of how to convert the second slide of a PowerPoint file to HTML:
from spire.presentation.common import *
from spire.presentation import *
# Create a Presentation instance
ppt = Presentation()
# Load a PowerPoint document
ppt.LoadFromFile("/pre1.pptx")
# Get the second slide
slide = ppt.Slides[1]
# Save the slide to HTML format
slide.SaveToFile("/SlidetoHTML.html", FileFormat.Html)
ppt.Dispose()
To Wrap Up
This guide walks you through converting PowerPoint to HTML in Python, covering both full presentation conversion and exporting specific slides. To ensure clarity, it includes step-by-step instructions and code examples. By the end of this article, you'll be able to seamlessly convert PowerPoint to HTML with ease!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
