A Guide to API Requests with Fetch in JavaScript

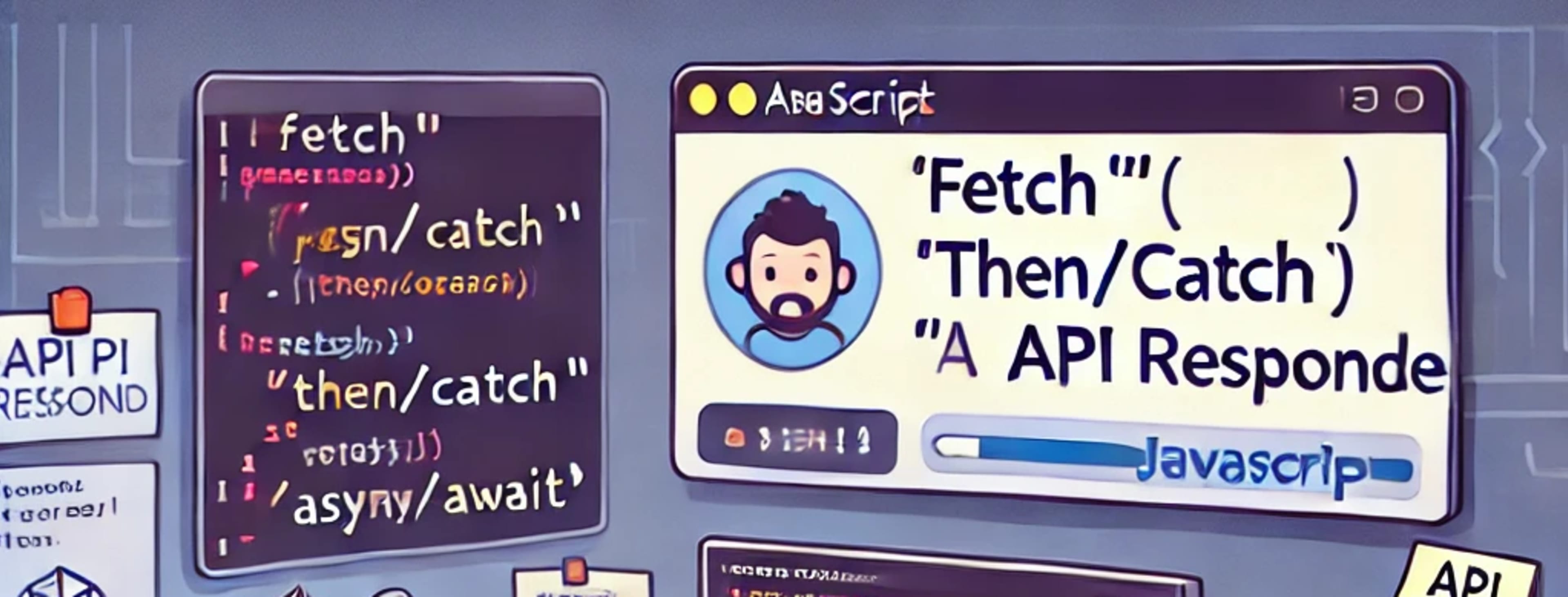
As a developer, when dealing with real-world web or app development projects, we need to call multiple API endpoints from the front end to the backend. This can be done in multiple ways, like by using fetch(URL, options)
, axios
and other methods.
Real World example:
Suppose we have a web app that generates random jokes. What we can do is, at the click of the button generate
we can call the backend API, which generates a random joke and shows it to the user.
Click the respective tab HTML, CSS, JS to see the code.
In this article, we are going to cover the fetch()
method from Javascript global objects to make an API call and handle the response, as well as do a little DOM manipulation.
API calls can take time to give back a response, maybe because the backend needs to read/write/update the data from the database(I/O operations in DB are time taking process), which can be hosted at a distance from the backend, or it needs to run some business logic in the server. So, to handle this kind of situation, we need to handle the API calling asynchronously by either using async
, await
or then
, catch
methods with fetch as it returns the promises and resolve with Response if success otherwise, if rejected then with Error object.
fetch() using then
, catch
methods.
Syntax
fetch(URL, options)
fetch(URL, options).then(res => #handle response).catch(error => #handle error)
It is the method/syntax that is used to make an API call were URL
is the API endpoint on which we have to send the request and options
are the objects that define the extra parameters/metadata for the API request, like body, request method, header, authorization code, params, etc.
List of some key-value pairs required during development.
Method
It is used to define request type, that what type of request we are making to the specific endpoint.
Available methods:
a. GET → Read the data
b. POST → Create new resources
c. PUT → Update the resource with complete new data
d. DELETE → Delete specific or group of resources
e. PATCH → Update the part of the data
we will cover all the methods in the upcomming articles.
Body
It is used to add extra information for the API endpoint. It can be used with any method.
For example, we can use this with the POST method for signup to add the username and password. The backend can access the username and add validation rules, such as "user already exists," "password follows specific rules.".
The value of the body should be a string, If you want to add the objects, then you have to convert it to string using
JSON.stringify(‘your object’)
syntax.Some extra types which body accepts.
we will cover the body options in lot more detail in upcoming articles.
Headers
It sends the client/sender information to the server, like if the request method is POST, then we can set
Content-Type: ‘application/json’
In the header options, we can set any type of data in the Content-Type, which is acceptable by the header options so that the server upfront knows which type of data it will receive.
Like this, more options are available which we will discus in more detail on some other article. Our main focus in this article is to handle our first API request.
Real-world example of fetch using the concepts we just learned.
generateBtn.addEventListener('click', () => {
fetch(API_GENERATE_JOKE, options)
.then(res => res.json())
.then(joke => {
jokeSetup.innerText = joke.setup
jokePunchLine.innerText = joke.punchline
console.log(joke)
})
If you go through the code in the codepen, you will find that we have a bunch of JS code where we are accessing the setup and punchline span element and adding the click event listener to the Generate button. As we click on the button, the callback function runs, where we are calling the generate joke API with the GET method.
The fetch()
returns the promise, and in promise we can add multiple layers of then method and manipulate the data according to our need. We are passing the arrow function as the parameter to then() method, which accepts the Response from the fetch. In the first then() method, we convert the Response object into JSON so that we can read our data from the response object, which combines lots of information.
Now, in the second then() method, we get the response and print it in the console from the callback function.
fetch() using async
and await
Another way to handle API calls is by using async
and await
, which makes the code more readable and easier to manage compared to chaining multiple .then()
methods. The async
keyword is used to define an asynchronous function, and within it, the await
keyword is used to pause the execution until the promise resolves.
Syntax
async function fetchData() {
try {
let response = await fetch(URL, options);
let data = await response.json();
console.log(data);
} catch (error) {
console.error("Error fetching data:", error);
}
}
Explanation
Defining an async function
We declare an asynchronous function usingasync function fetchData()
. This allows us to use theawait
keyword inside it.Fetching data with
await
The
fetch(URL, options)
method returns a promise.Instead of using
.then()
, we useawait
to pause execution until the request completes.
Handling the response
We use
await response.json()
to convert the response into a JavaScript object.Since
response.json()
also returns a promise, we useawait
again.
Error handling with
try...catch
If an error occurs during the fetch (e.g., network failure), it will be caught in the
catch
block.The
console.error()
method logs the error details.
Real-world example using async
and await
Let's modify our joke generator example to use async
and await
:
generateBtn.addEventListener('click', async () => {
try {
let response = await fetch(API_GENERATE_JOKE, options);
let joke = await response.json();
jokeSetup.innerText = joke.setup;
jokePunchLine.innerText = joke.punchline;
console.log(joke);
} catch (error) {
console.error("Error fetching joke:", error);
}
});
Key Differences from .then()
Approach
More readable and structured
Theasync/await
version looks more like synchronous code, making it easier to understand.Better error handling
Instead of using.catch()
, we usetry...catch
, which provides a cleaner way to handle errors.
Subscribe to my newsletter
Read articles from Barun Tiwary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Barun Tiwary
Barun Tiwary
Hii buddy, wanna learn, explore tech world. Come together to explore the world of Technology