Using Channels & Dataflow in .NET for High Performance, Real Time Applications

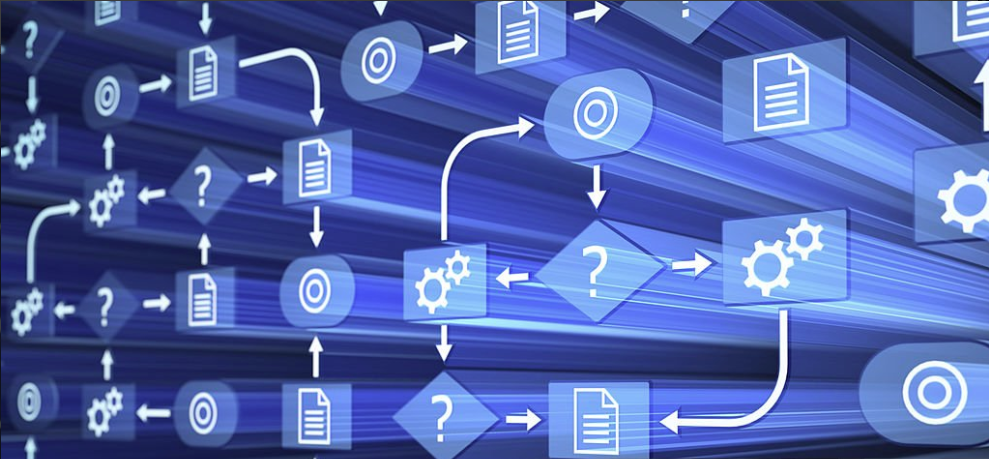
Building real time applications in .NET often requires handling multiple concurrent operations efficiently. Whether processing incoming data streams, managing background tasks, or coordinating workflows, performance is a key concern. Traditional threading models or basic Task.Run
approaches may not always scale well under heavy loads. This is where System.Threading.Channels
and System.Threading.Tasks.Dataflow
become invaluable tools.
Channels for High Throughput Workloads
Channels provide an asynchronous, thread safe queueing mechanism that helps decouple producers from consumers. They offer fine grained control over data flow, ensuring that messages are processed efficiently without unnecessary contention.
Take an application that receives telemetry data from thousands of sensors. Using a Channel<int>
, sensor readings can be enqueued and processed asynchronously without overwhelming system resources:
var channel = Channel.CreateUnbounded<int>();
async Task Producer(ChannelWriter<int> writer)
{
for (int i = 0; i < 1000; i++)
{
await writer.WriteAsync(i);
}
writer.Complete();
}
async Task Consumer(ChannelReader<int> reader)
{
await foreach (var value in reader.ReadAllAsync())
{
Console.WriteLine($"Processed: {value}");
}
}
var producerTask = Producer(channel.Writer);
var consumerTask = Consumer(channel.Reader);
await Task.WhenAll(producerTask, consumerTask);
This approach ensures that the producer does not block the consumer, making it ideal for high throughput scenarios. If back pressure needs to be applied, a bounded channel can be used instead, limiting the number of unprocessed messages in memory.
Dataflow for Complex Pipelines
For workflows requiring multiple stages of processing, the System.Threading.Tasks.Dataflow
library provides a structured approach. A pipeline can be constructed using blocks that accept, process, and distribute data between various stages.
A common example is processing a stream of user actions, transforming them, and sending the results to storage. Using TransformBlock
and ActionBlock
, this can be achieved as follows:
var transformBlock = new TransformBlock<string, string>(action =>
{
return $"Processed: {action}";
}, new ExecutionDataflowBlockOptions { MaxDegreeOfParallelism = 4 });
var actionBlock = new ActionBlock<string>(result =>
{
Console.WriteLine(result);
});
transformBlock.LinkTo(actionBlock, new DataflowLinkOptions { PropagateCompletion = true });
transformBlock.Post("User clicked button");
transformBlock.Post("User scrolled page");
transformBlock.Complete();
await actionBlock.Completion;
This setup ensures that multiple transformations occur in parallel while maintaining an ordered flow of execution. The MaxDegreeOfParallelism
setting allows adjustment of concurrency levels to match system capabilities.
Choosing the Right Approach
Channels work well when managing independent producers and consumers with minimal dependencies. They offer a straightforward way to implement event driven architectures where different components operate at different speeds. On the other hand, Dataflow provides a structured way to build complex processing pipelines, particularly useful when tasks depend on the results of previous stages.
Real time applications require careful consideration of memory usage, task scheduling, and workload distribution. By using these tools, we can create solid, scalable solutions that handle high frequency data streams efficiently. Whether processing messages from a queue, handling real time user interactions, or building an event driven system, integrating Channels or Dataflow into a .NET application can significantly enhance performance and maintainability.
Subscribe to my newsletter
Read articles from Patrick Kearns directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
