๐ Understanding Closures in JavaScript ๐ฅ

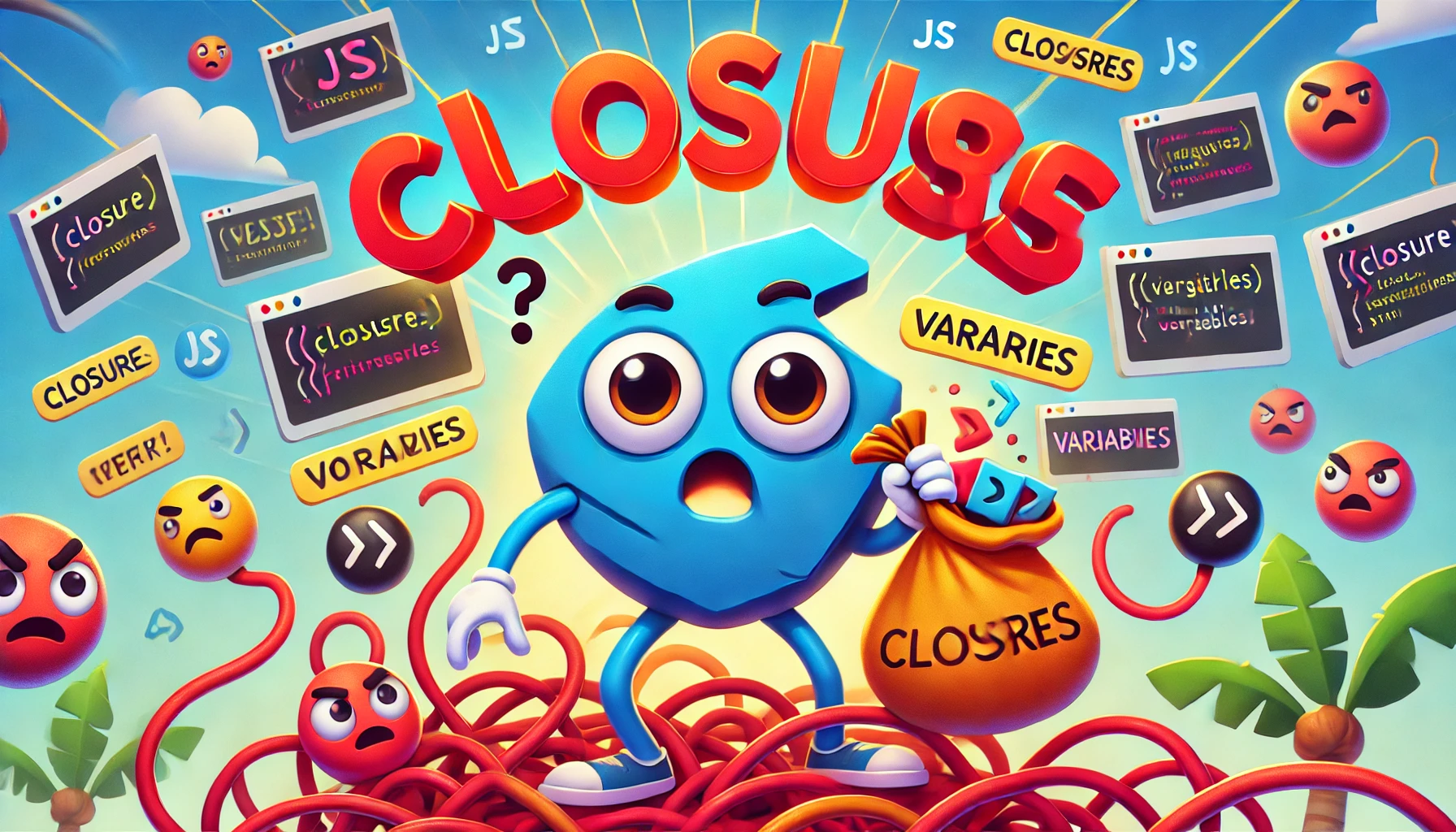
Closures are an important concept in JavaScript that help functions remember the variables from their parent function, even after the parent function has finished running. ๐ง โจ
๐ค What is a Closure?
A closure happens when a function is created inside another function and keeps access to the outer function's variables. ๐
๐ Example:
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log(`Outer: ${outerVariable}, Inner: ${innerVariable}`);
};
}
const newFunction = outerFunction("Hello");
newFunction("World");
๐ฅ๏ธ Output:
Outer: Hello, Inner: World
โ
Even though outerFunction
has finished running, innerFunction
still remembers outerVariable
.
๐ฏ Why Use Closures?
1๏ธโฃ Data Privacy ๐
Closures help keep variables private:
function counter() {
let count = 0;
return function () {
count++;
console.log(count);
};
}
const myCounter = counter();
myCounter(); // 1๏ธโฃ
myCounter(); // 2๏ธโฃ
๐น count
cannot be accessed directly from outside.
2๏ธโฃ Remembering Values ๐ง
Closures help functions remember values:
function multiply(x) {
return function(y) {
return x * y;
};
}
const double = multiply(2);
console.log(double(5)); // ๐
โ
The function double
remembers x = 2
.
โ ๏ธ A Common Mistake ๐จ
Using var
inside a loop can cause unexpected results:
for (var i = 0; i < 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
โณ Output (after 1 second):
3
3
3
โ Why? Because var
is function-scoped and all callbacks refer to the same i
.
โ
Fix: Use let
instead of var
to create a new scope for each loop:
for (let i = 0; i < 3; i++) {
setTimeout(function() {
console.log(i);
}, 1000);
}
โ Correct Output:
0
1
2
๐ Conclusion
Closures are powerful! ๐ก They help with: โ Keeping variables private ๐ โ Remembering values ๐ง โ Handling loops correctly ๐
Mastering closures will make you a better JavaScript developer! ๐
Subscribe to my newsletter
Read articles from Anjali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
