A Discussion on the forEach Loop in JavaScript

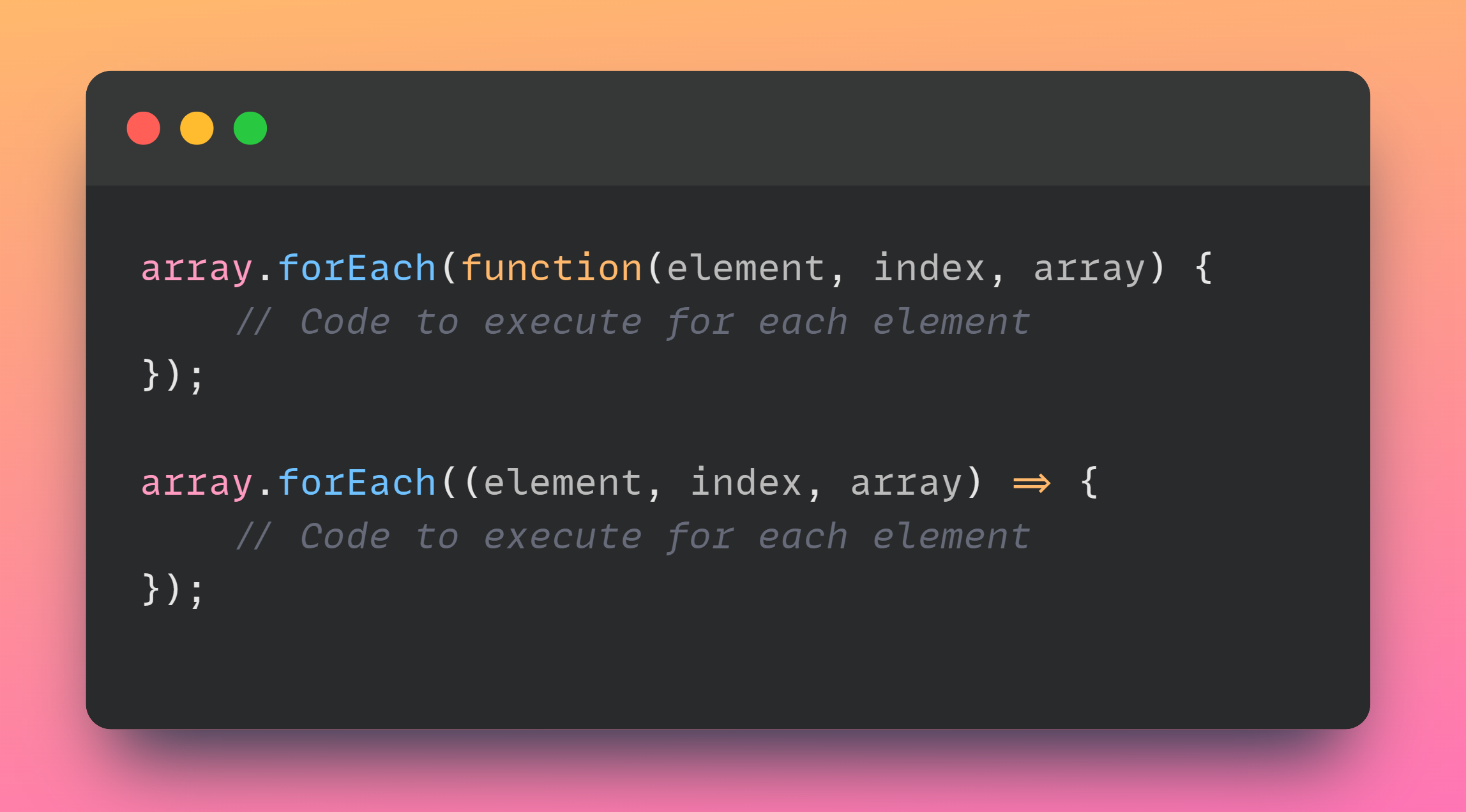
Hey, I’m writing this to share something that might just make your JavaScript journey a little smoother—the forEach
loop. If you’ve ever found yourself writing traditional for
loops and wondering if there’s a cleaner way, you’re not alone. I’ve been there too.
forEach
is one of those handy methods that let you iterate over data effortlessly, making your code more readable and expressive. In this post, I’ll walk through how forEach
works and show its application across different structures—arrays, objects, and even nested data. Let’s get started!
Why forEach
?
Unlike traditional for
or while
loops, forEach
is a higher-order function that directly interacts with each element of an array without requiring explicit index handling. This makes it a cleaner and more intuitive choice for iteration.
Basic Syntax & Parameters
At its core, forEach
takes a callback function and executes it for every element in the array. Here’s the syntax
Or, using an arrow function:
Breaking Down the Parameters:
element
→ The current item in the array or the current element being processed . (Required)index
→ The position of the element in the array. (Optional)array
→ The entire array being iterated. (Optional)
Now that we understand the basics, let’s explore how forEach
can be applied across different data structures in JavaScript! 🚀
1. Using forEach
with Simple Arrays
Let’s start with the most common use case—iterating over an array of values.
OUTPUT:
Number: 10
Number: 20
Number: 30
Number: 40
Here, forEach
executes the callback for each element in the numbers
array.
2. Using forEach
with Arrays of Objects
Often, we work with arrays of objects, such as a list of products, users, or transactions. Let’s see how forEach
helps in such cases:
here in the code snippet, as you can see there is a tasks array of objects in which different tasks are mentioned along with a priority number and whether it is completed or not by having boolean key completed. Our Job is to find all the tasks which are not completed and sort them according to priority.
To solve this problem we have created a empty notCompleted
list or array to store the desired task. In the for each loop element represents each individual object inside the tasks array as the loop iterates through it.
We check if
element.completed
isfalse
, meaning task is not yet completed.If the condition is met, we push that task into the
notCompleted
array.After the loop completes,
notCompleted
contains all the tasks that are still pending.
At this stage, the tasks are filtered but not sorted. To sort them based on priority , we can use the inbuilt sort method like this,
Now, notCompleted
will list the tasks in order of priority, with the most urgent ones appearing first. 🚀
Before exploring forEach loop more on different types of nested data items, let’s take a look some of the properties
3. Some Properties of forEach loop
The forEach()
method does not return anything (undefined
). It simply executes a callback function on each element of an array but does not create a new array or modify the original one (unless explicitly done inside the callback).
1️⃣ Iterates Over Each Element → It executes a callback function for each array element.
2️⃣ Does Not Modify Original Array → Unless explicitly modified inside the callback.
3️⃣ Does Not Return Anything → Always returns undefined
, unlike map()
or filter()
.
4️⃣ Cannot Be Chained → Since it returns undefined
, chaining another method like .map()
after forEach()
won't work.
5️⃣ Does Not Support break
or return
→ Unlike for
or for...of
loops, you cannot break or return early inside forEach()
.
6️⃣ Executes Synchronously → Runs immediately and does not wait for asynchronous operations inside the callback.
Final Thoughts: When to Use forEach
?
forEach
is a great choice when:
✔ You need to execute a function for each element of an array.
✔ You don’t need to return a modified array (use map
instead for that).
✔ You want clean, readable iteration without managing indexes manually.
However, keep in mind:
❌ forEach
cannot be stopped or broken—use a for...of
loop or some()
if you need early termination.
❌ It does not return a value, so don’t expect transformations like map()
.
By understanding its use across different data structures, you can write cleaner and more efficient JavaScript code. So, next time you find yourself reaching for a for
loop, ask yourself—can forEach
do the job better? 🚀
Subscribe to my newsletter
Read articles from Santwan Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Santwan Pathak
Santwan Pathak
"A beginner in tech with big aspirations. Passionate about web development, AI, and creating impactful solutions. Always learning, always growing."