JavaScript Functions & Scope: A Deep Dive ๐

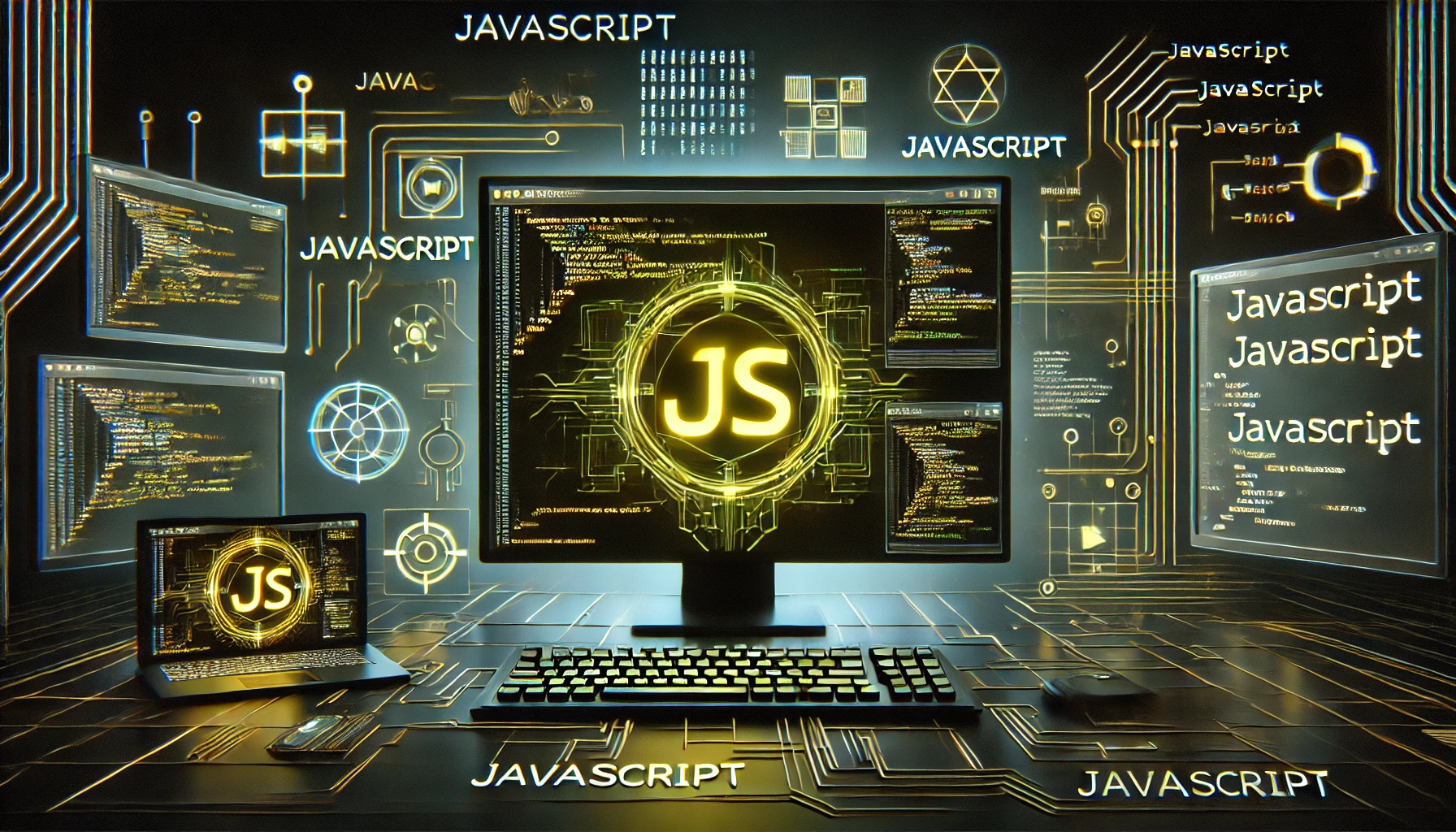
Introduction
Functions are one of the most powerful features of JavaScript. They allow us to write reusable, modular, and maintainable code. Along with functions, understanding scope is crucial for writing efficient and bug-free programs. In this blog, we will explore different types of functions and how JavaScript handles scope.
1๏ธโฃ What is a Function in JavaScript?
A function is a block of reusable code that performs a specific task. Functions help in organizing and structuring the code efficiently.
Function Syntax:
function greet(name) {
return Hello, ${name}!
;
}
console.log(greet("Vivek")); // Output: Hello, Vivek!
2๏ธโฃ Function Declaration vs Function Expression
Function Declaration
A function declaration defines a named function that can be called before its declaration due to hoisting.
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // Output: 8
Function Expression
In a function expression, the function is assigned to a variable and cannot be called before it is defined.
const subtract = function(a, b) {
return a - b;
};
console.log(subtract(8, 3)); // Output: 5
3๏ธโฃ Arrow Functions (ES6)
Arrow functions provide a concise way to write functions and automatically bind this
.
const multiply = (a, b) => a * b;
console.log(multiply(4, 3)); // Output: 12
4๏ธโฃ Understanding JavaScript Scope
Scope determines the accessibility of variables in JavaScript. There are three main types:
๐น Global Scope
Variables declared outside any function are accessible everywhere.
let globalVar = "I'm Global";
function showGlobal() {
console.log(globalVar);
}
showGlobal(); // Output: I'm Global
๐น Local Scope (Function Scope)
Variables declared inside a function are accessible only within that function.
function localScopeExample() {
let localVar = "I'm Local"; console.log(localVar);
}
localScopeExample(); // Output: I'm Local
// console.log(localVar); // Error: localVar is not defined
๐น Block Scope (ES6: let
and const
)
Variables declared with let
and const
inside a block {}
cannot be accessed outside.
{
let blockScoped = "Inside Block";
console.log(blockScoped); // Output: Inside Block
}
// console.log(blockScoped); // Error: blockScoped is not defined
5๏ธโฃ Hoisting in Functions
Function declarations are hoisted, meaning they can be called before their definition.
console.log(hoistedFunction()); // Works fine!
function hoistedFunction() {
return "Hoisting Works!";
}
However, function expressions are not hoisted.
console.log(notHoisted()); // Error
const notHoisted = function() {
return "This will not work!";
};
Conclusion
Understanding functions and scope is essential for mastering JavaScript. Functions help organize code, while scope ensures variables are used efficiently. We also learned about hoisting, which impacts how functions behave.
๐ฏ What's Next? In the next blog, we will explore JavaScript Closures in detail. Stay tuned! ๐
๐ Read More on: vivekwebdev.hashnode.dev ๐
Subscribe to my newsletter
Read articles from Vivek varshney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vivek varshney
Vivek varshney
Full-Stack Web Developer | Blogging About Tech, React.js, Angular, Node.js & Web Development. Turning Ideas into Code & Helping Businesses Grow!