OOP encapsulation doubt and find differences in method override
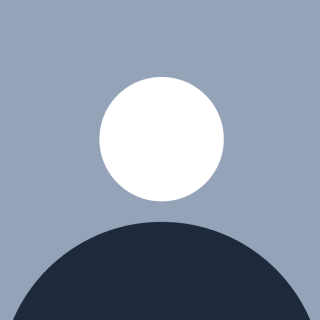
Table of contents
- Code Example for Task Management
- 1️⃣ How can we change #isCompleted without a getter/setter?
- 2️⃣ Does task.completeTask() directly go to the Task class method?
- 3️⃣ How can we name the same method (completeTask****) in two classes without polymorphism?
- 4️⃣ Why didn’t we extend the Task class in TaskManager?
- 5️⃣ Why does task.completeTask() work, but this.tasks.completeTask() gives an error?
//Concept Covered
//encapsulation(Using private properties)
//Classess(Moduler and reusable code)
class Task{
#isCompleted //private variable (Encapsulation)
constructor(title,description){
this.title=title;
this.description=description;
this.#isCompleted=false;
}
#markAsCompleted(){
this.#isCompleted=true;
console.log(`${this.title} is marked as completed`);
}
completeTask(){
this.#markAsCompleted();
}
getTaskInfo(){
return{
title:this.title,
description:this.description,
completed:this.#isCompleted
}
}
}
class TaskManager{
constructor(){
this.tasks=[];
}
addTask(title,description){
const newTask= new Task(title,description);
console.log('new taskis ',newTask);
this.tasks.push(newTask);
}
deleteTask(title){
this.tasks=this.tasks.filter(task =>task.title!==title);
}
completeTask(title){
const task=this.tasks.find(task=>task.title === title);
if(task){
task.completeTask();
}else{
console.log(`Task with title "${title}" not found.`);
}
}
showTask(){
console.log(this.tasks.map(task=>task.getTaskInfo()));
}
}
const manager= new TaskManager();
manager.addTask("Learn Oop","Practice JS OOP concepts");
manager.addTask("Build a Project", "Apply concepts by coding");
manager.showTask();
manager.deleteTask("Build a Project");
manager.completeTask("Learn Oop");
manager.showTask();
console.log(manager);
Code Example for Task Management
1️⃣ How can we change #isCompleted
without a getter/setter?
The reason we can change #isCompleted
inside completeTask()
is because it is a method within the same class (Task
).
👉 Private fields (#isCompleted
) can only be accessed within the class they are defined in.
Since completeTask()
is inside Task
, it has access to the private variable and can modify it.
❌ You cannot access #isCompleted
outside of the Task
class, meaning manager.tasks[0].#isCompleted = true;
would cause an error.
2️⃣ Does task.completeTask()
directly go to the Task
class method?
Yes! Here's why:
When we call
manager.completeTask("Learn Oop")
, it:Finds the
Task
object inthis.tasks
(which storesTask
instances).Calls
task.completeTask()
, which belongs to theTask
class.Inside
completeTask()
, the private variable#isCompleted
is set totrue
.
🔹 Key point:
Every object in this.tasks
is an instance of the Task
class, so calling task.completeTask()
on it executes the method fromTask
.
3️⃣ How can we name the same method (completeTask
****) in two classes without polymorphism?
There's no issue with having the same method name in different classes.
Polymorphism is when different classes have the same method name but different behaviors.
Here,
Task.completeTask()
marks a task as completed, andTaskManager.completeTask(title)
finds a task and callsTask.completeTask()
.
So, there's no method overriding happening here—just separate methods with the same name in different classes.
4️⃣ Why didn’t we extend the Task
class in TaskManager
?
Extending (class TaskManager extends Task
) would mean TaskManager inherits properties and methods of Task, but this is not needed.
TaskManager is not a type of Task—it is a manager that handles multiple
Task
objects.Composition is better than inheritance here:
- Instead of
TaskManager
being aTask
, it contains an array ofTask
objects.
- Instead of
🔹 When to use inheritance vs. composition?
Inheritance (extends) | Composition (has-a relationship) |
Use when one class is a specialized version of another (Car extends Vehicle ). | Use when one class is composed of multiple instances of another (TaskManager has many Task objects ). |
👉 Here, TaskManager
has Task
objects, so we use composition.
5️⃣ Why does task.completeTask()
work, but this.tasks.completeTask()
gives an error?
this.tasks
is an array ofTask
objects.Arrays don't have a
completeTask()
method—onlyTask
objects do.
👉 Example:
this.tasks[0].completeTask(); // ✅ Works! Because tasks[0] is a Task object.
this.tasks.completeTask(); // ❌ Error! Because `this.tasks` is an array.
💡 Fix: To complete all tasks in the array, you can use a loop:
this.tasks.forEach(task => task.completeTask());
Summary:
1️⃣ Private fields (#isCompleted
) can only be accessed within the same class, which is why completeTask()
can modify it.
2️⃣ task.completeTask()
directly calls the method inside Task
, which updates the private variable.
3️⃣ Method names can be the same in different classes because they are not overriding each other.
4️⃣ We use composition (TaskManager contains Tasks) instead of inheritance, since TaskManager
is not a type of Task
.
5️⃣ this.tasks
is an array, so calling this.tasks.completeTask()
throws an error—we need to call completeTask()
on an individual Task
object.
Subscribe to my newsletter
Read articles from Ashraful Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Ashraful Islam
Ashraful Islam
Full-stack developer proficient in building web applications with React, Redux, Redux-Thunk,RTK,RTK Query and Tailwind CSS for the frontend, and using Express.js, Node.js, MongoDB, and SQL for backend development. Experienced in C for general coding tasks and has some knowledge of Python, particularly for machine learning model training. Committed to efficiency and ongoing growth in software development.