JavaScript Data Types Explained: A Beginner's Guide with Examples

Table of contents
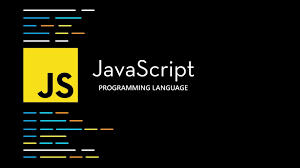
Hi! Today is another day to dive into learning JavaScript.
Today, we're focusing on JavaScript data types, a fundamental concept every developer needs to understand.
Now, before we start, I want you to grasp a simple analogy that aims to help you understand data types in JavaScript.
Imagine you have a bunch of items scattered on the floor. Each item is different, serving its unique purpose. To keep things organized, you decide to group or label them based on their qualities. In JavaScript, data types work the same way. They help us categorize and identify values so we can use them efficiently in our code.
By understanding JavaScript data types, you’ll be able to work with variables more effectively and write cleaner, error-free programs.
Types of Data Types in JavaScript with Examples
The first type of data type in JavaScript is the String. Strings are used to represent text and are enclosed in quotes, which can be either single or double quotes.
The next JavaScript data type is the Number. Numbers in JavaScript represent both whole numbers and decimal values, which we can also call floating point numbers.
The next data type is called the Boolean. Booleans are represented by either true or false; that is, these are the two possible values they have. They are commonly used in conditional statements to check if a condition is met or not.
The next data type is called the Null. Null represents an intentional absence of any value. That means the variable exists, being one of the data types, but there is nothing there.
The next data type is Undefined. Undefined in JavaScript means that there is no proper definition to the variable; that is, you have successfully defined a variable but haven’t assigned a value to it.
The next data type is the Symbol. Symbol is a unique data type used to create data types that are guaranteed to be unique.
The next data type is the BigInt. BigInt represents a large set of numbers that goes beyond the limit of regular numbers.
So, let us use an example to see how a particular data type works. We will be using the Boolean.
The above code checks whether a number is even or odd.
We start by defining a function called
checkNum
and passing in a parameter,num
.Since we are checking whether something is true or false, we use a conditional statement, that is, an if statement.
The condition inside the
if
statement checks ifnum
is divisible by 2 using the modulus operator (%
).The expression
num % 2 === 0
means:num % 2
returns the remainder whennum
is divided by2
.If the remainder is
0
(=== 0
), that meansnum
is even, so we returntrue
.Otherwise, we return
false
, meaning the number is odd.
Finally, we call the function and pass in
4
and9
as arguments to check their results:checkNum(4)
returnstrue
because 4 is even.checkNum(9)
returnsfalse
because 9 is odd.
I believe that with this simple explanation, you should understand what data types in JavaScript mean and have a basic understanding of how to use them.
To learn more about data types, pls visit the MDN documentation, and to get access to the codes, visit the GitHub repo.
Subscribe to my newsletter
Read articles from USIWO SONIA directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

USIWO SONIA
USIWO SONIA
I am a Frontend Developer and Writer from Nigeria