Deploying Applications on Google Cloud Run: Maximizing Cost Efficiency with Serverless Containers
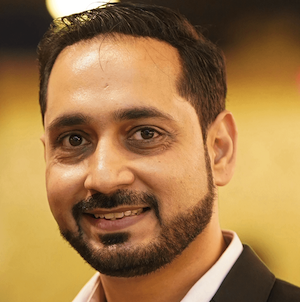
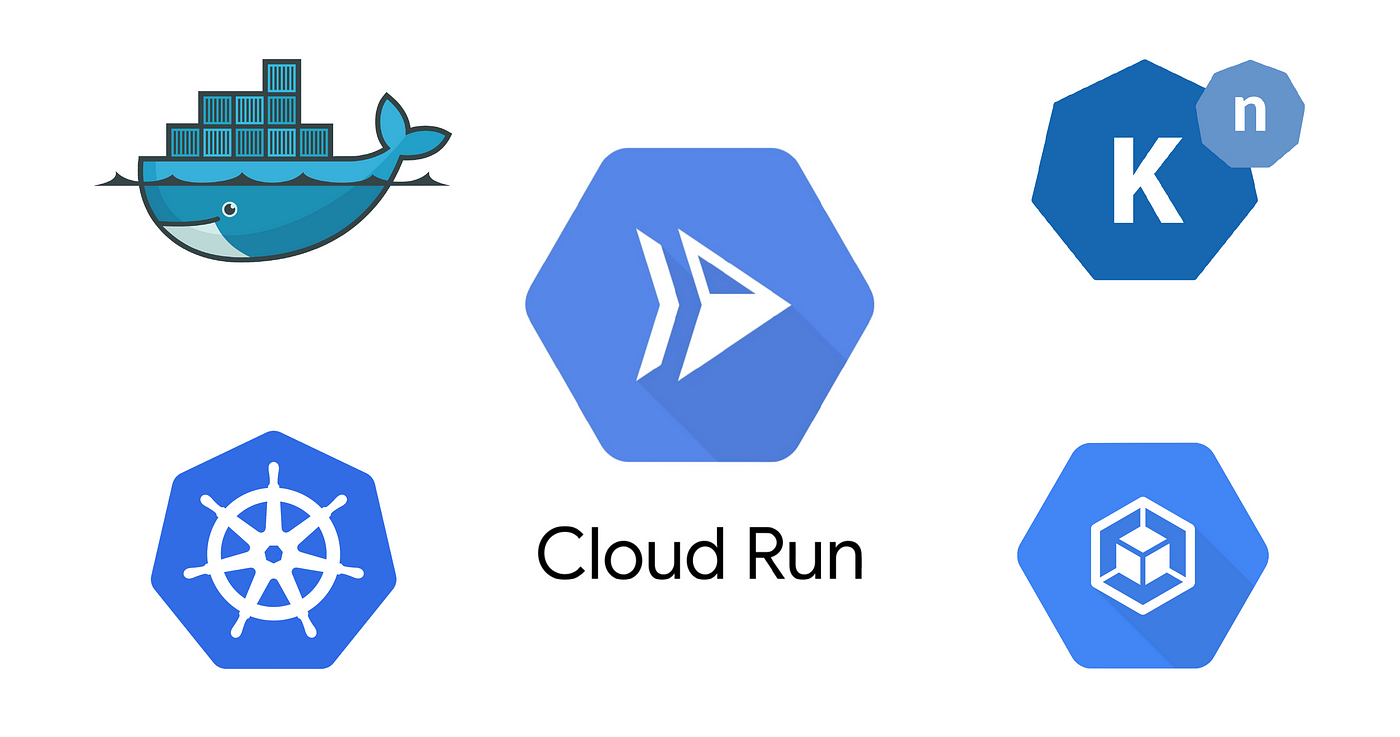
Google Cloud Run is a fully managed compute platform that enables you to run stateless containers without worrying about the underlying infrastructure. It abstracts away server management, automatically scales your applications based on demand, and only charges you for the resources you use.
It is essential to understand how it differs from Serverless technologies and even the traditional way of deploying on Kubernetes.
Serverless vs. Cloud Run: While both are serverless in nature, Cloud Run specifically focuses on running containerized applications. Unlike traditional serverless platforms (e.g., AWS Lambda, Google Cloud Functions), which are limited to specific runtimes and code formats, Cloud Run allows you to deploy any containerized application, giving you more flexibility.
Traditional Kubernetes vs. Cloud Run: Kubernetes (e.g., GKE) requires you to manage clusters, nodes, and scaling policies. Cloud Run, on the other hand, is fully managed, eliminating the need for cluster management. It automatically scales to zero when idle and scales up instantly during traffic spikes, making it more cost-effective and easier to use for lightweight, event-driven workloads.
Before we start with the deployment of our first application on Google Cloud Run, I want to show case the pricing plan and review that up to 2M requests are already free for the month. Even after that, it is only $0.04 / million request. Review detailed pricing here.
Now that we understand the basics of Google Cloud Run and how cheap is it to host our applications on Cloud Run, lets try creating a “Hello World“ application.
Pre-requisites:
Google Cloud Account
Billing Enabled
Google Cloud Run API Enabled
Gcloud CLI installed on local machine (you can also use cloud-shell)
Agenda:
During this elmental exploration journey of Deployment on Google Cloud Run we will go through the following concepts:
Enable Cloud Run API using CLI
Create a simple Node.js Application
Create a Dockerfile to containerize the application
Create and Push the Image to Google Artifact Repository
Deploy the Application
Cleanup by deleting service and images.
Cloud Run API setup
If you are already logged in with your gcloud cli, then list your account to verify the right account
gcloud auth list
You should see an output like:
Credentialed accounts:
- myaccount@mydomain.com (active)
Now list the projects in your account to verify the right project is selected as current project or if required then create a project:
gcloud config list project
This will give an output like below:
[core]
project = nodejs-gcp-66776654433
For more information on gcloud CLI, review the cli documentation here.
Now that we have selected the right account and project, lets start by enabling the Google Cloud Run API. You can do it from the API and Services Section of the Google Cloud Console also by lets do it gcloud cli, run the following command:
gcloud services enable run.googleapis.com
It can take a moment to enable the API, once done, then let us set the right compute region and location environment variable to use later for docker commands.
export LOCATION="us-east-1
export GOOGLE_CLOUD_PROJECT="nodejs-gcp-66776654433"
gcloud config set compute/region $LOCATION
Here, the region will be your preferred region.
Write a Nodejs Hello World Application
To write an express-based nodejs application, you will need two files, a package.json
to list all the dependencies and an index.js
file with the application logic.
Create a
package.json
file and write the following content into it. (You can also use npm init, if you have npm installed on your machine)vi package.json
{ "name": "helloworld", "description": "Simple hello world sample in Node", "version": "1.0.0", "main": "index.js", "scripts": { "start": "node index.js" }, "author": "Google LLC", "license": "Apache-2.0", "dependencies": { "express": "^4.17.1" } }
Create
index.js
file and write the following content into it:const express = require('express'); const app = express(); const port = process.env.PORT || 8080; app.get('/', (req, res) => { const name = process.env.NAME || 'World'; res.send(`Hello ${name}!`); }); app.listen(port, () => { console.log(`helloworld: listening on port ${port}`); });
You may try to run it locally by first downloading the package dependencies using
npm install
and then running the commandnode index.js
Containerize the Application using Dockerfile
The Docker deamon uses a Dockerfile to create a docker image, which can be used later to run its containers. Create a file named Dockerfile
in the same folder with the following content:
# Use the official lightweight Node.js 12 image.
# https://hub.docker.com/_/node
FROM node:12-slim
# Create and change to the app directory.
WORKDIR /usr/src/app
# Copy application dependency manifests to the container image.
# A wildcard is used to ensure copying both package.json AND package-lock.json (when available).
# Copying this first prevents re-running npm install on every code change.
COPY package*.json ./
# Install production dependencies.
# If you add a package-lock.json, speed your build by switching to 'npm ci'.
# RUN npm ci --only=production
RUN npm install --only=production
# Copy local code to the container image.
COPY . ./
# Run the web service on container startup.
CMD [ "npm", "start" ]
Now you need to build your docker image using the either the local Docker daemon, or if you are running these commands on your Google Cloud Shell then you can also use gcloud
cli to build it on cloud. here are both the procedures:
Build Docker Image using Local Docker Daemon (Docker Desktop in most cases)
docker build . -t gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld:1.0.0 docker push gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld:1.0.0
Remember, we created the
GOOGLE_CLOUD_PROJECT
environment variable already. In you cloud shell, you will not need to create it as it is available by default.The first command will build the image, where as the second command will push it to your GCR (Google Container Registry)
Build and push Docker image using
gcloud
cli by running the following commandgcloud builds submit --tag gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld
The above command will build the Image in cloud and even push it to the respective repository as well.
You may list the images by using the following commands
gcloud container images list docker images
You may use the first command to list the images on GCR, where as second command will list the images on your local machine.
Lets test the application by running the container locally
docker run -d -p 8080:8080 gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld
The above command will pull the Image from the GCR if not already available locally. In the second Step it will run a container using the pulled image. To test the application, do
curl localhost:8080
you should seehello world
message as a response from the container.
Deploy the Image as a Cloud Run Service
Deploying any Docker Image as a docker container on Google Cloud Run is fairly simple and straight forward, use the following command:
gcloud run deploy --image gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld
--allow-unauthenticated --region=$LOCATION
We used the —allow-unauthenticated
flag as we want to keep the application public. We used the same $LOCATION
environment variable we created earlier.
On Success, you will get a service URL and other details as follows:
Service [helloworld] revision [helloworld-00001-xit] has been deployed
and is serving 100 percent of traffic.
Service URL: https://helloworld-abc1234-uc.a.run.app
Congratulations! you have successfully deployed your first Cloud Run Application. You can also deploy applications directly from public repositories like docker hub and other GCR.io registries.
To verify your deployment, use the Service URL to open it in your browser. You can also verify and manage the deployed services by navigating to Cloud Run in your Google Cloud Console as follows:
Cleanup
Run the following command to delete the docker image from the GCR registry:
gcloud container images delete
gcr.io/$GOOGLE_CLOUD_PROJECT/helloworld
Also, run the following commands to delete the local docker image:
docker rmi hellowworld:1.0.0
docker image prune -f
delete running Cloud Run service by using the cli as follows:
gcloud run services delete helloworld --region=$LOCATION
Conclusion:
Deploying a container on Google Cloud Run is simple, straightforward, and cost-effective, making it an excellent choice for a wide range of applications. By leveraging its serverless architecture, you can focus on building and scaling your applications without worrying about infrastructure management. Here are some key use cases where Google Cloud Run shines, offering both convenience and cost efficiency:
Deploying a Static Website Using NGINX:
Hosting a static website is a perfect fit for Cloud Run. With its ability to scale to zero, you only pay when users access your site. This is ideal for portfolios, documentation sites, or small business websites that don’t require constant uptime.Deploying a Front-End Application (React, Angular, or Vue):
Cloud Run seamlessly handles single-page applications (SPAs) built with modern frameworks. Its automatic scaling ensures your app remains responsive during traffic spikes, while the pay-as-you-go model keeps costs low during periods of low activity.Deploying an API:
Whether it’s a RESTful API or a GraphQL endpoint, Cloud Run is an excellent platform for backend services. Its ability to handle concurrent requests and scale instantly makes it ideal for APIs with fluctuating traffic, such as those used in mobile apps or microservices architectures.Deploying Machine Learning Models (e.g., Deepseek LLM):
Cloud Run is a great choice for deploying machine learning models or AI-powered applications. For instance, you can containerize a large language model (LLM) like Deepseek and deploy it as a scalable, cost-effective service. Since Cloud Run scales to zero, you avoid paying for idle resources when the model isn’t in use.Event-Driven Applications:
Cloud Run integrates seamlessly with event-driven architectures. For example, you can deploy a service that processes data from Pub/Sub, triggers workflows in response to Cloud Storage events, or handles webhooks from third-party services. This makes it ideal for batch processing, data pipelines, or automation tasks.Microservices and Lightweight Workloads:
If you’re building a microservices-based application, Cloud Run allows you to deploy each service independently. Its lightweight nature and fast cold-start times make it perfect for small, focused services that don’t require the overhead of a full Kubernetes cluster.Prototyping and Development:
For developers and startups, Cloud Run is a cost-effective way to prototype and test new ideas. You can quickly deploy and iterate on your applications without worrying about infrastructure costs or complexity.
In summary, Google Cloud Run offers a versatile, cost-efficient platform for deploying a wide variety of applications. Its serverless nature, automatic scaling, and pay-as-you-go pricing make it an attractive option for everything from static websites and APIs to advanced AI models like Deepseek LLM. By choosing Cloud Run, you not only simplify deployment but also optimize costs, ensuring you only pay for what you use. Whether you’re a startup, a developer, or an enterprise, Cloud Run empowers you to focus on innovation while leaving the infrastructure management to Google Cloud.
Subscribe to my newsletter
Read articles from Arslan Ali Ansari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
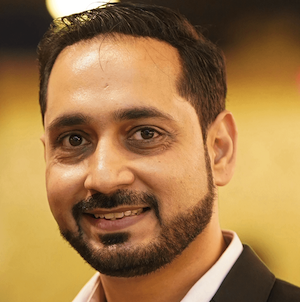
Arslan Ali Ansari
Arslan Ali Ansari
A technology evangelist and entrepreneur passionate about software development using cloud-native technologies. Currently leading a cross-Atlantic team of enthusiasts developing and extending the core of Kubernetes at kaiops.io