Technical Deep Dive: Defending Your Backend from Steganography Attacks

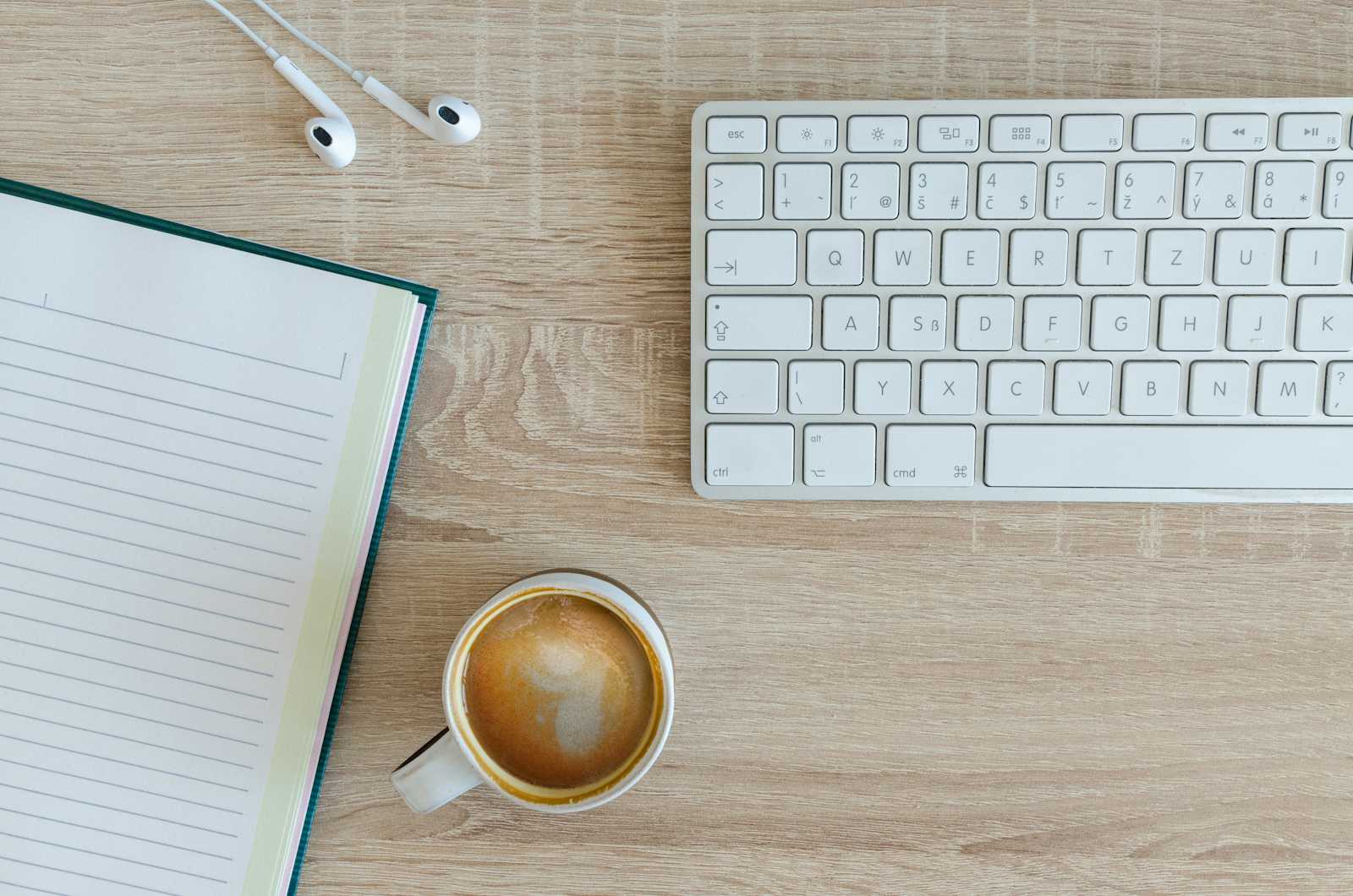
At Olotu square recent Hackday event, I witnessed a live demonstration where an image file was manipulated to hide malicious code. This scenario—where attackers exploit file upload vulnerabilities through steganography—prompted me to explore a highly technical, layered defense strategy. In this post, I'll detail a rigorous approach for backend developers to mitigate such threats, complete with practical Node.js code samples and Docker sandboxing techniques.
1. Rigorously Validate File Uploads
Relying solely on file extensions is not secure. Instead, inspect the file’s binary data (magic numbers) to confirm its true format. Using the file-type
library, you can enforce a whitelist of allowed MIME types.
const fileType = require('file-type');
const fs = require('fs');
async function validateFile(filePath) {
const stream = fs.createReadStream(filePath);
const type = await fileType.fromStream(stream);
// Enforce allowed MIME types
if (!type || (type.mime !== 'image/jpeg' && type.mime !== 'image/png')) {
throw new Error('Invalid file type');
}
console.log(`Validated file: ${type.mime}`);
return type;
}
// Usage example:
validateFile('uploads/sample.jpg')
.then(() => console.log('File is safe to process.'))
.catch(err => console.error('Validation error:', err.message));
This code ensures that only files with the correct magic numbers for JPEG or PNG files proceed further in the processing pipeline.
2. Re-encode and Strip Metadata
Even valid files might harbor hidden data. Re-encoding images effectively normalizes the content and strips out non-essential metadata. For a more performance-focused approach, consider using the Sharp library:
const sharp = require('sharp');
async function reencodeImage(inputPath, outputPath) {
try {
await sharp(inputPath)
// Resize or simply re-encode to remove extra embedded data
.toFormat('jpeg', { quality: 90 })
.toFile(outputPath);
console.log('Image re-encoded and metadata removed.');
} catch (error) {
console.error('Error re-encoding image:', error);
}
}
// Usage example:
reencodeImage('uploads/sample.png', 'processed/sample.jpg');
This method decodes the image and outputs a new JPEG file, effectively eliminating any hidden payloads present in the original metadata.
3. Implement Sandboxing for File Processing
Isolating file processing tasks minimizes risk. Containerizing these operations using Docker can significantly mitigate damage if malicious content is processed.
Dockerfile for a Node.js File Processor:
FROM node:14
WORKDIR /app
COPY package.json .
RUN npm install
COPY . .
# Set a non-root user for added security
RUN useradd -ms /bin/bash appuser
USER appuser
CMD ["node", "processUploads.js"]
By running file processing inside a container, you isolate any potentially harmful operations, reducing the risk to your primary infrastructure.
4. Integrate Steganalysis (Optional Advanced Step)
For environments requiring high security, you can integrate steganalysis tools like StegExpose. While many such tools are command-line based, you can invoke them from Node.js using the child_process
module.
const { exec } = require('child_process');
function runStegExpose(filePath) {
// Adjust the command and parameters as needed based on your steganalysis tool installation
exec(`stegexpose ${filePath}`, (error, stdout, stderr) => {
if (error) {
console.error(`StegExpose error: ${error.message}`);
return;
}
if (stderr) {
console.error(`StegExpose stderr: ${stderr}`);
return;
}
console.log(`StegExpose output: ${stdout}`);
});
}
// Usage example:
runStegExpose('processed/sample.jpg');
This approach adds an extra layer of scrutiny, flagging files with statistical anomalies that could indicate hidden data.
5. Monitor and Log All File Upload Activities
Detailed logging provides an audit trail and can help detect anomalies early. The following sample uses the Winston logging library:
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'logs/uploads.log' })
]
});
function logUpload(fileName, fileSize) {
logger.info({ message: 'File uploaded', fileName, fileSize });
}
// Example: Log an upload event
logUpload('sample.jpg', 204800);
Monitoring file sizes, validation failures, and re-encoding results helps create a comprehensive picture of file activity and potential threats.
Conclusion
Steganography attacks hide in plain sight by embedding malicious payloads within otherwise legitimate files. By implementing strict validation using magic numbers, re-encoding files to strip metadata, sandboxing file processing with Docker, optionally integrating steganalysis tools, and maintaining robust logging, you build a resilient backend defense.
These technical measures not only fortify your file upload pipelines but also prepare your systems to handle evolving threats. Stay vigilant, continuously update your processes, and consider each layer as a critical part of a comprehensive security strategy.
Feel free to share your thoughts or additional techniques for hardening backend systems against these sophisticated attacks.
Subscribe to my newsletter
Read articles from Samuel Nwankwo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Samuel Nwankwo
Samuel Nwankwo
I am a software developer based in Nigeria with some years of experience in the industry. I am an expert in several programming languages, including PHP, JavaScript, and Java for Android development. When I am not coding, I enjoys playing video games and watching sci-fi movies. I am also an avid reader and enjoys learning about new technologies and programming concepts.