Difference Between Composition and Inheritance
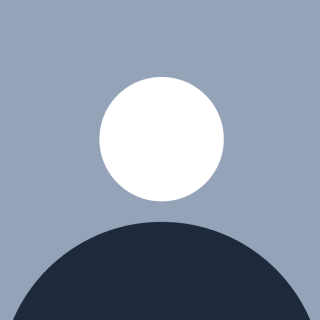
Inheritance: Accessing Parent Class Methods and Variables
If a class does not have direct access to parent class’s properties or methods, then we can use inheritance for access the parent class.
Example 1: Using extends
for Inheritance
class Parent{
constructor(){
this.parentProperty="I am from parent Property";
}
parentMethod() {
console.log("This is a parent method");
}
}
class Child extends Parent{
constructor(){
super(); // Calls Parent constructor
}
childMethod(){
console.log(this.parentProperty);
this.parentMethod()
}
}
const child= new Child();
child.childMethod();
I am from Parent class
This is a parent method
Here, Child
automatically gets access to Parent
methods and properties because it extends it
Example 2: Using Composition (this.parent = new Parent()
)
If a class does NOT inherit from another class, but we still need access to it, we can manually store an instance.
class Parent {
constructor() {
this.name = "Parent";
}
greet() {
console.log("Hello from Parent");
}
}
class Child {
constructor() {
this.parent = new Parent(); // ✅ Giving access to Parent manually
}
useParentMethod() {
this.parent.greet(); // ✅ Accessing Parent method using composition
}
}
const child = new Child();
child.useParentMethod(); // Output: Hello from Parent
Here, Child
does not inherit Parent
, but it still accesses its methods using this.parent
.
✅ If a class instance has access to another class, it can use its methods.
✅ Using extends
allows a class to inherit another class's methods and properties.
✅ If a class does not inherit but still needs access, we can create an instance (this.parent = new Parent()
).
Subscribe to my newsletter
Read articles from Ashraful Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Ashraful Islam
Ashraful Islam
Full-stack developer proficient in building web applications with React, Redux, Redux-Thunk,RTK,RTK Query and Tailwind CSS for the frontend, and using Express.js, Node.js, MongoDB, and SQL for backend development. Experienced in C for general coding tasks and has some knowledge of Python, particularly for machine learning model training. Committed to efficiency and ongoing growth in software development.