Day 17: JavaScript ES6+ Featuresโ-โDestructuring, Spread Operator & Moreย ๐

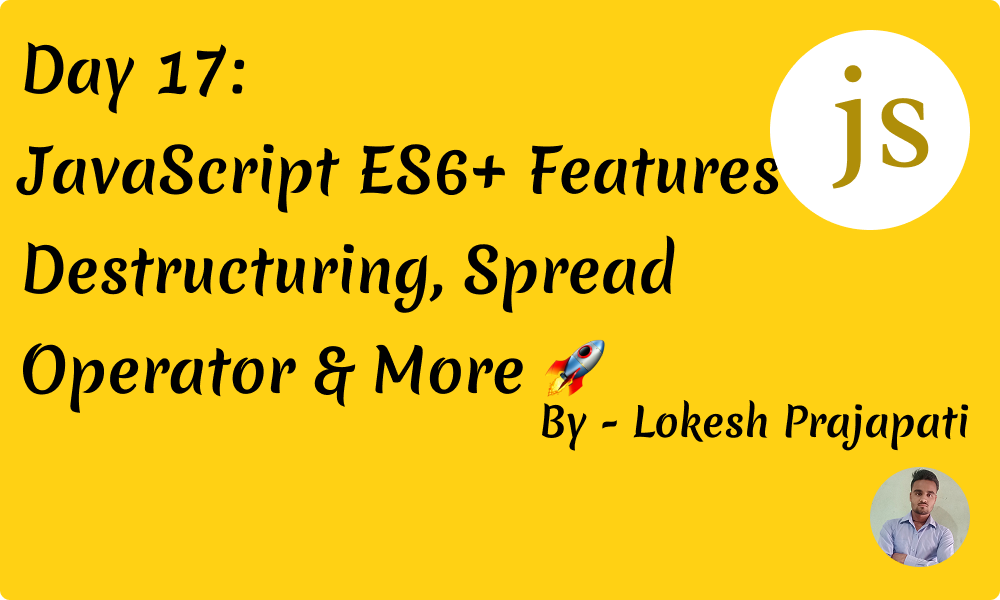
JavaScript ES6 (ECMAScript 2015) introduced several powerful features that improved code readability, efficiency, and scalability. Mastering these features will help you write cleaner and more modern JavaScript code.
In this post, weโll explore:
Destructuring
Spread Operator
Arrow Functions
Template Literals
Default Parameters
Letโs dive in! ๐ฅ
1. Destructuring Assignment ๐ฆ
Destructuring allows you to extract values from arrays or objects and assign them to variables in a clean and concise way.
Array Destructuring
const fruits = ["apple", "banana", "cherry"];
const [first, second, third] = fruits;
console.log(first); // Output: apple
console.log(second); // Output: banana
Object Destructuring
const person = { name: "John", age: 30 };
const { name, age } = person;
console.log(name); // Output: John
console.log(age); // Output: 30
โ Tip: Destructuring is perfect for fetching API responses and handling data effectively.
2. Spread Operator (โฆ) ๐
The spread operator allows you to expand iterable elements like arrays and objects.
Example 1: Merging Arrays
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, ...arr2];
console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
Example 2: Copying Objects
const obj1 = { a: 1, b: 2 };
const obj2 = { ...obj1, c: 3 };
console.log(obj2); // Output: { a: 1, b: 2, c: 3 }
โ Tip: Spread operator simplifies tasks like copying data, merging arrays, and passing function arguments.
3. Arrow Functions โก๏ธ
Arrow functions offer a concise syntax for writing functions in JavaScript.
Example:
const add = (a, b) => a + b;
console.log(add(5, 3)); // Output: 8
Benefits of Arrow Functions:
โ
Shorter syntax
โ
Automatically binds this
to the parent scope
4. Template Literals (`) ๐
Template literals allow you to create dynamic strings using backticks (`
) and the ${}
syntax for variable interpolation.
Example:
const name = "Alice";
const greeting = `Hello, ${name}! Welcome to ES6.`;
console.log(greeting); // Output: Hello, Alice! Welcome to ES6.
โ Tip: Template literals are great for constructing multiline strings and embedding expressions.
5. Default Parameters โ๏ธ
Default parameters provide default values for function arguments to avoid undefined
values.
Example:
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
greet("John"); // Output: Hello, John!
greet(); // Output: Hello, Guest!
โ Tip: Default parameters improve code stability by handling missing arguments.
6. Enhanced Object Literals ๐
ES6 introduced improved syntax for defining objects.
Example:
const name = "John";
const age = 30;
const user = { name, age, greet() { console.log("Hello!"); } };
console.log(user);
Output:
{ name: "John", age: 30, greet: [Function: greet] }
โ Tip: Enhanced object literals simplify object creation and method definitions.
Conclusion ๐
JavaScript ES6+ features make coding easier, cleaner, and more efficient. Mastering concepts like destructuring, the spread operator, and template literals can greatly improve your development skills. ๐
Which ES6+ feature is your favorite? Let me know in the comments! ๐
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
๐ JavaScript | React | Shopify Developer | Tech Blogger Hi, Iโm Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. Iโm currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Letโs learn and grow together. ๐ก๐ #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode