How to Build Real-Time Chat Applications with Laravel and Pusher
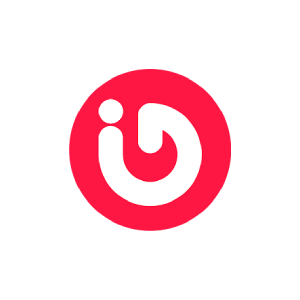
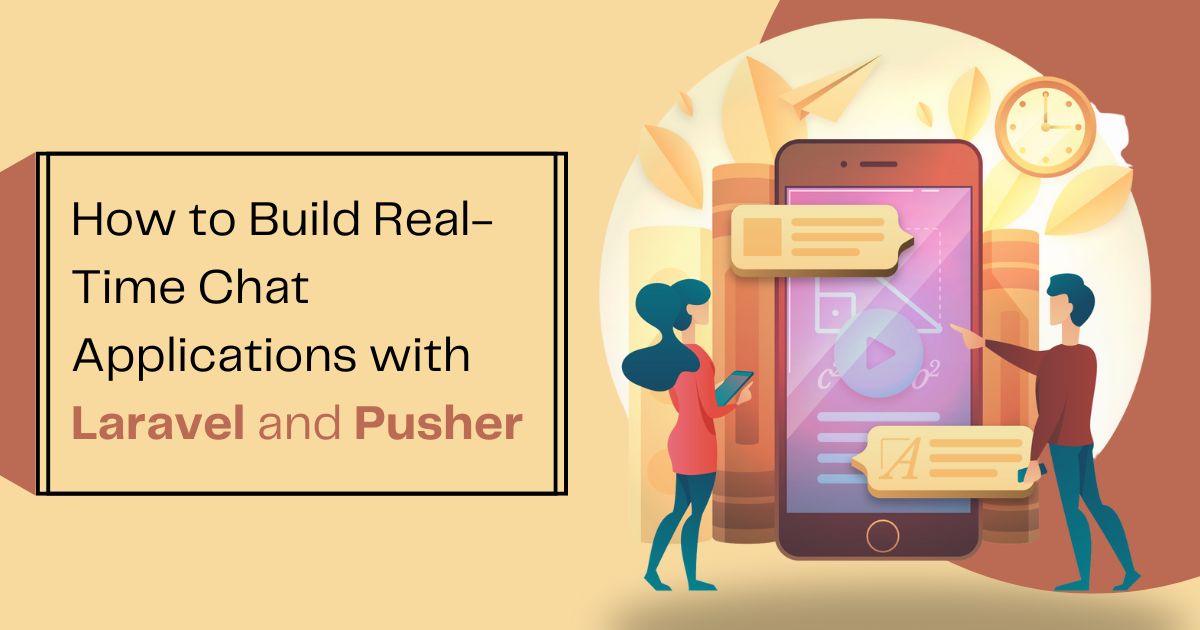
Web developers commonly undertake real-time chat application development as an important project. The rise of instant communication functionality in web services creates a growing demand that developers can approach through Laravel Development and Pusher-based systems, which serve as enjoyable educational experiences. This beginner guide explains the entire process of developing a real-time chat application that uses Laravel and Pusher.
What You Will Learn in This Guide:
How to set up a Laravel project
How to install and configure Pusher
How to create the basic structure of a chat application
How to send and receive real-time messages
How to integrate Pusher with Laravel for broadcasting events
How to test and deploy your application
What is Laravel?
We will begin this guide by briefly examining Laravel before starting the chat application
development. Laravel is a PHP web application framework that simplifies web app development by supplying various built-in functionalities. The framework has an elegant syntax and built-in features that handle requests and authentication alongside other functionalities. Earth Ads Studio can develop apps rapidly because of its extensive development tools, which makes it a leading PHP framework.
What is Pusher?
Pusher's web application service delivers real-time messaging and instant notifications to online applications. You can use Pusher to transmit live data to your clients in real-time, thus making it the perfect choice for developing live chat applications. WebSockets enable your application to deliver instant updates to clients through Pusher automatically.
Setting Up the Laravel Project
Let's begin by setting up the Laravel project on your machine.
Step 1: Install Laravel
Install Composer on your machine first to proceed. Laravel installation requires a dependency manager PHP application named Composer.
To install Laravel, open your terminal and run:
bash
Copy
composer create-project --prefer-dist laravel/laravel real-time-chat
This command will create a new Laravel project named "real-time-chat". Once the installation is complete, navigate into your project directory:
bash
Copy
cd real-time-chat
Step 2: Set Up Your Database
Laravel supports multiple database systems like MySQL, PostgreSQL, and SQLite. You can configure your database by editing the .env file located at the root of your project. Here's an example configuration for MySQL:
plaintext
Copy
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=chat_app
DB_USERNAME=root
DB_PASSWORD=
Create the database on your MySQL server by running:
bash
Copy
mysql -u root -p
CREATE DATABASE chat_app;
Installing Pusher
To add real-time functionality to our chat app, we will integrate Pusher. This involves installing Pusher's PHP SDK and JavaScript library.
Step 1: Install Pusher PHP SDK
Use Composer to install the Pusher SDK:
bash
Copy
composer requires pusher/pusher-php-server
Step 2: Create a Pusher Account
Go to the Pusher website and sign up for an account. After logging in, create a new app under the dashboard. You will be given the credentials for the app, such as:
App ID
App Key
App Secret
App Cluster
Please keep this information safe; you'll need it to configure Pusher.
Step 3: Configure Pusher in Laravel
Now, you need to add your Pusher credentials to the .env file:
plaintext
Copy
BROADCAST_DRIVER=pusher
PUSHER_APP_ID=your-app-id
PUSHER_APP_KEY=your-app-key
PUSHER_APP_SECRET=your-app-secret
PUSHER_APP_CLUSTER=your-app-cluster
Then, in the config/broadcasting.php file, set the connections. Pusher array to use the credentials from the .env file:
php
Copy
'pusher' => [
'driver' => 'pusher',
'key' => env('PUSHER_APP_KEY'),
'secret' => env('PUSHER_APP_SECRET'),
'app_id' => env('PUSHER_APP_ID'),
'cluster' => env('PUSHER_APP_CLUSTER'),
'encrypted' => true,
],
Creating the Chat Application
Let's create the structure of our real-time chat application.
Step 1: Database Schema for Chat
To store chat messages, create a migration and model for the Message:
bash
Copy
php artisan make:model Message -m
In the generated migration file, define the schema for storing chat messages:
php
Copy
public function up()
{
Schema::create('messages', function (Blueprint $table) {
$table->id();
$table->string('username');
$table->text('message');
$table->timestamps();
});
}
Run the migration:
bash
Copy
php artisan migrate
Step 2: Create a Controller for Handling Chat
Now, create a controller to handle chat functionality:
bash
Copy
php artisan make: controller ChatController
In the ChatController, add the following methods:
php
Copy
public function index()
{
return view('chat.index');
}
public function sendMessage(Request $request)
{
$message = new Message();
$message->username = $request->username;
$message->message = $request->message;
$message->save();
broadcast(new NewMessage($message)); // Broadcasting event
return response()->json($message);
}
Step 3: Create a Pusher Event
Next, create an event that will be broadcast to clients when a new message is sent:
bash
Copy
php artisan make:event NewMessage
In the NewMessage event, broadcast the Message:
php
Copy
public function __construct(Message $message)
{
$this->message = $message;
}
public function broadcastOn()
{
return new Channel('chat');
}
Step 4: Frontend Setup for Real-Time Updates
Now, we need to display the messages in real time. First, install Laravel Echo and Pusher JS:
bash
Copy
npm install --save laravel-echo pusher-js
Next, configure Echo in resources/js/bootstrap.js:
js
Copy
import Echo from 'laravel-echo';
import Pusher from 'pusher-js';
window.Pusher = Pusher;
window.Echo = new Echo({
broadcaster: 'pusher',
key: 'your-app-key',
cluster: 'your-app-cluster',
forceTLS: true
});
Create a simple chat interface in resources/views/chat/index.blade.php:
html
Copy
<div id="chat-container">
<div id="messages"></div>
<input type="text" id="username" placeholder="Your name" />
<textarea id="message" placeholder="Type a message"></textarea>
<button onclick="sendMessage()">Send</button>
</div>
<script>
Echo.channel('chat')
.listen('NewMessage', (event) => {
const messagesDiv = document.getElementById('messages');
messagesDiv.innerHTML += <p><strong>${event.message.username}</strong>: ${event.message.message}</p>;
});
function sendMessage() {
const username = document.getElementById('username').value;
const message = document.getElementById('message').value;
axios.post('/send-message', { username, message })
.then(response => {
console.log('Message sent');
})
.catch(error => {
console.log(error);
});
}
</script>
Step 5: Testing the Application
Now that everything is set up, you can test your application by running:
bash
Copy
php artisan serve
Visit http://127.0.0.1:8000/chat in your browser. Open multiple tabs or windows to see the real-time chat in action.
FAQs
Q1: What is Laravel Echo, and how does it work?
Through its JavaScript library, Laravel Echo provides users with efficient methods to subscribe to channels while listening to live events. Through WebSockets, your server enables push message delivery to clients without requiring them to refresh their pages.
Q2: Can I use Pusher for other types of real-time notifications?
Pusher enables numerous real-time notifications, from live scores to real-time updates and alerts. Laravel Echo operates outside of the constraints that impact chat applications.
Q3: Do I need to pay for Pusher?
Users can choose between no-cost and paid plan options when using Pusher. The free plan covers the typical needs of small projects, but you should select a paid plan when working with increased traffic levels alongside additional features.
Q4: Is this chat application secure?
An application ready for production needs authentication and authorization features, which should be added before proceeding to build. Laravel provides built-in authentication tools that are suitable for deployment.
Q5: How can I deploy this application?
The deployment of your Laravel application works on both shared hosting platforms and cloud services, which include Heroku, AWS, and DigitalOcean. Configuring your deployment requires proper settings for your production environment's .env and broadcasting services.
Conclusion
For developers seeking experience with real-time application development, web developers should start building a chat system that integrates Laravel and Pusher. This tutorial enabled you to establish a Laravel setup alongside Pusher installation and develop a basic chat application that delivers live message updates.
Subscribe to my newsletter
Read articles from iGex Solutions directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
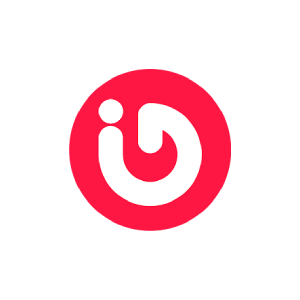
iGex Solutions
iGex Solutions
iGex Solutions here for you.We are delivering Web Development, Web Design, Mobile App, Laravel Development, Wordpress Website, PHP & Laravel Development & SEO Services in USA or Europe. Elevate your online presence, drive innovation, and achieve digital excellence with our expert solutions tailored to meet the evolving needs of businesses in these dynamic markets.