Effective Error Handling in Flutter with Bloc and Repository Patterns

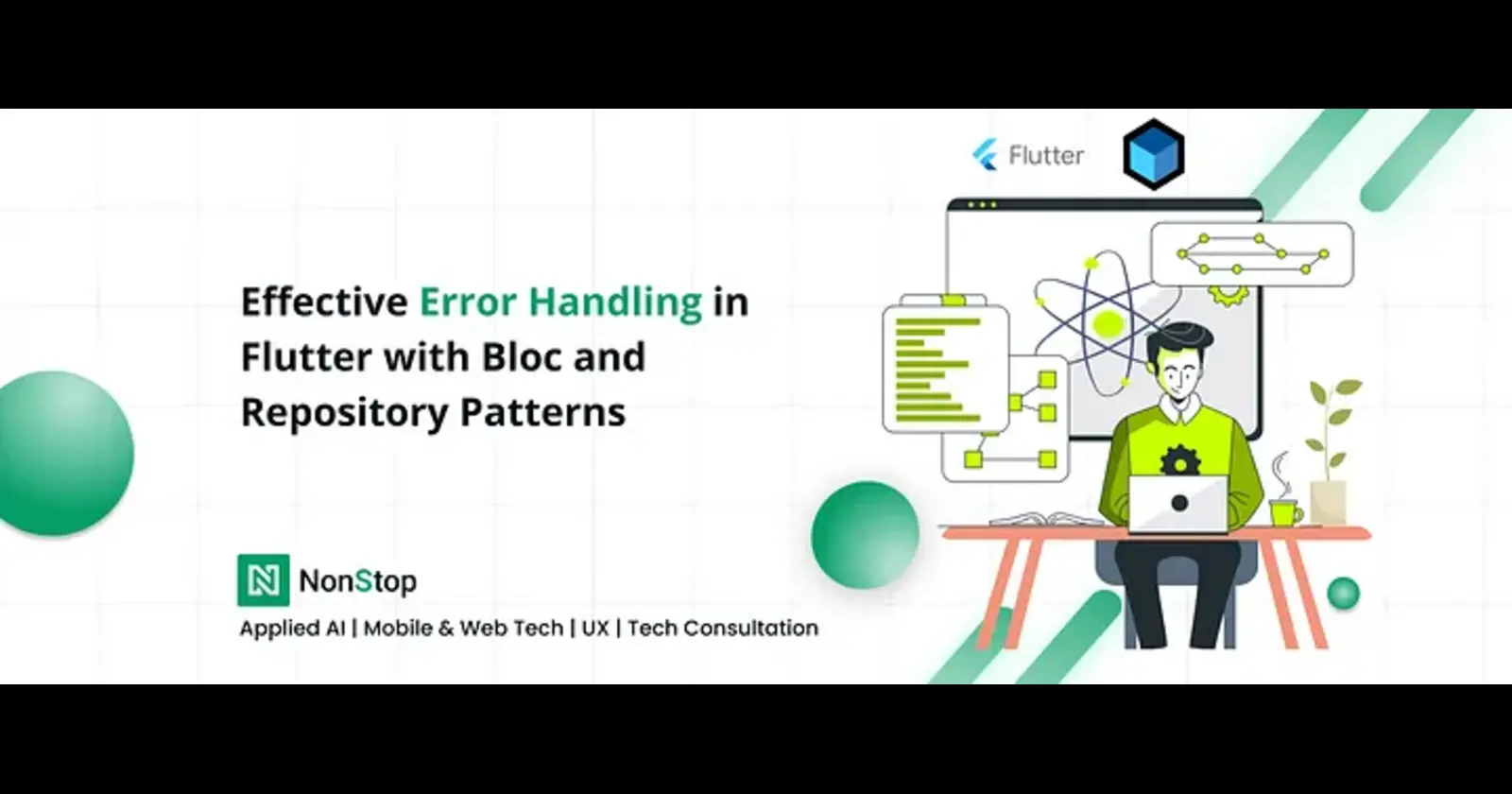
Error handling is essential for providing a seamless user experience and maintaining app stability. The Bloc and Repository patterns manage errors across the app by separating data and business logic layers, enabling centralized error management. This approach standardizes error handling, making it easier to maintain and read.
In this blog, we’ll explore how to implement error handling in Flutter using a repository that catches specific exceptions and rethrows them as standardized exceptions, and a Bloc that only needs to handle a single type of error. This design helps keep the Bloc layer focused on business logic and state management without needing to manage multiple types of low-level exceptions.
Repository Layer: Catching Specific Exceptions
In the repository layer, we catch specific exceptions (like FailedException
) that arise from network calls or other data access issues and rethrow them as a custom, standardized RepoException
. This hides the complexity of underlying errors from the Bloc, making error handling simpler and more consistent.
Let’s look at an example of a UserRepositoryRepo
and its implementation UserRepositoryImpl
:
// Custom exception classes
class FailedException implements Exception {
final String message;
FailedException(this.message);
@override
String toString() => 'FailedException: $message';
}
class RepoException implements Exception {
final String message;
RepoException(this.message);
@override
String toString() => 'RepoException: $message';
}
// Repository interface
abstract class UserRepositoryRepo {
Future<User> fetchUser(int userId);
}
// Repository implementation
class UserRepositoryImpl extends UserRepositoryRepo {
final ApiClient apiClient;
UserRepositoryImpl(this.apiClient);
@override
Future<User> fetchUser(int userId) async {
try {
final response = await apiClient.getUser(userId);
if (response.isSuccess) {
return User.fromJson(response.data);
} else {
throw FailedException(response.message); // Specific exception
}
} on FailedException catch (e) {
throw RepoException(e.message); // Convert to RepoException
} catch (e) {
throw RepoException('An unexpected error occurred.'); // Generic fallback
}
}
}
Explanation:
UserRepositoryImpl
:
Handles the actual data-fetching logic.
Catches
FailedException
and rethrows it as aRepoException
for the Bloc layer.Converts any unexpected errors into a generic
RepoException
, ensuring the Bloc layer only needs to handle one exception type.
This setup ensures that the Bloc doesn’t need to handle multiple exception types, simplifying its error management.
Bloc Layer: Standardized Error Handling with RepoException
In the Bloc, we handle only the RepoException
. This simplifies error handling, allowing the Bloc to focus on business logic and state management without worrying about specific exceptions from the repository.
Here’s an example of how this works in UserBloc
:
// Bloc for managing user-related events and states
class UserBloc extends Bloc<UserEvent, UserState> {
final UserRepositoryRepo userRepository;
UserBloc(this.userRepository) : super(UserInitial()) {
on<FetchUserEvent>(_onFetchUser);
}
Future<void> _onFetchUser(
FetchUserEvent event,
Emitter<UserState> emit,
) async {
try {
emit(UserLoading());
final user = await userRepository.fetchUser(event.userId);
emit(UserLoaded(user));
} on RepoException catch (error) {
emit(UserError(error.message)); // Emit error state for UI feedback
}
}
}
Explanation:
UserBloc:
Catches only
RepoException
, keeping error handling simple and streamlined.Emits a
UserError
state if an error occurs, allowing the UI to display a user-friendly error message.By handling only one exception type, the Bloc layer remains decoupled from specific exceptions in the repository, making it easier to maintain and extend.
Handling Errors in the UI
In the UI layer, we can easily display error messages based on the Bloc’s state. Here’s a basic example of how the UI can react to different states:
class UserScreen extends StatelessWidget {
final int userId;
UserScreen(this.userId);
@override
Widget build(BuildContext context) {
return BlocProvider(
create: (_) => UserBloc(UserRepositoryImpl(ApiClient()))..add(FetchUserEvent(userId)),
child: Scaffold(
appBar: AppBar(title: Text('User Details')),
body: BlocConsumer<UserBloc, UserState>(
listener: (context, state) {
if (state is UserError) {
// Show an error message as a SnackBar or as UI feedback
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text(state.message)),
);
}
},
builder: (context, state) {
if (state is UserLoading) {
return Center(child: CircularProgressIndicator());
} else if (state is UserLoaded) {
return UserDetailWidget(user: state.user);
} else if (state is UserError) {
// Display the error message on the screen
return Center(child: Text(state.message, style: TextStyle(color: Colors.red, fontSize: 16)));
} else {
return Center(child: Text('No data available'));
}
},
),
),
);
}
}
Explanation:
BlocConsumer:
Listens to Bloc state changes. When
UserError
is emitted, it shows aSnackBar
with the error message, giving immediate feedback.Displays a
CircularProgressIndicator
when inUserLoading
state, user data inUserLoaded
, or a fallback message if an error occurs.
Error Handling in UI:
When
UserError
is emitted, theSnackBar
shows a quick notification to the user.If the screen is in
UserError
state, the error message will also be displayed prominently in red on the screen, providing a clear indication of the error. Here we can customize any other error like ‘Failed to load user data’
Benefits of This Approach
Centralized Error Management: By handling specific exceptions in the repository and rethrowing them as a single
RepoException
, we reduce complexity in Bloc, focusing only on higher-level error handling.Consistent UI Feedback: With error states standardized, we can provide consistent feedback to users without scattering error logic across various layers.
Improved Maintainability: The Bloc layer remains decoupled from specific exceptions in the repository, allowing for easier updates and code readability.
Summary
Using a standardized approach to error handling in Flutter with the Bloc and Repository patterns simplifies error management and improves app stability. By:
Catching and converting specific exceptions to a standard
RepoException
in the repository,Handling only
RepoException
in Bloc for streamlined state management, andProviding user-friendly feedback in the UI,
we achieve a clean, maintainable error-handling structure. This approach enhances user experience by providing clear feedback and robust app behavior, especially when errors occur.
This method keeps your error-handling code organized, ensuring that errors are managed consistently across your Flutter app, and establishes a solid foundation for building scalable, user-friendly applications.
Hope you enjoyed this article!
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.