Polyfills in JavaScript: Bridging the Gap Between Old and New

Table of contents
- TL;DR:
- Introduction:
- What Are Polyfills?
- Why Do We Need Polyfills?
- Key Concepts for Writing Polyfills:
- Example: Writing a Polyfill for Array.prototype.map
- Flow Explanation with an Example
- Improving the Polyfill:
- Best Practices for Writing Polyfills
- Conclusion
- Join the Discussion:
- Engage with Us:
- Share Your Feedback:
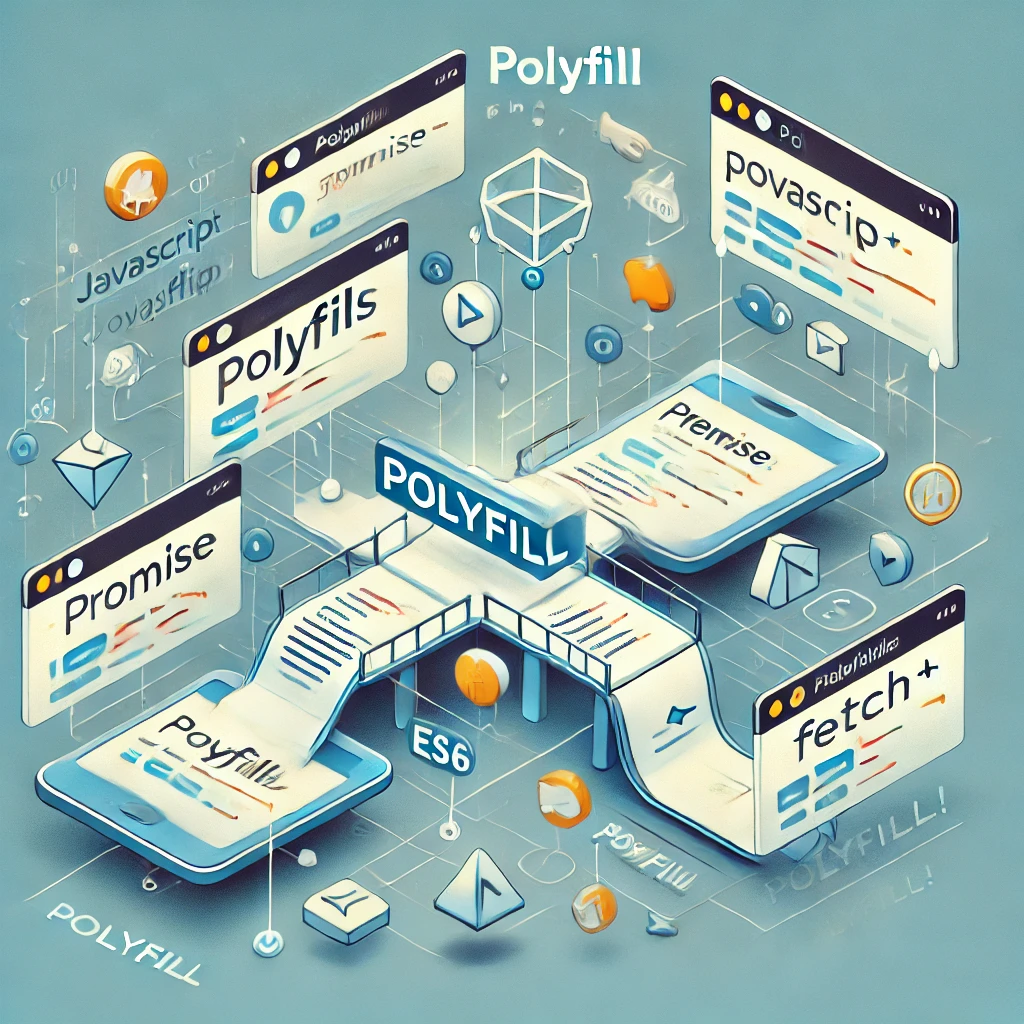
TL;DR:
Polyfills are code snippets that add modern JavaScript features to older environments, making sure compatibility of the code. They’re needed because browsers update at different paces, and developers want to use the latest features without breaking older systems. Writing polyfills involves understanding prototypes, this
context, and feature detection. For example, a polyfill for Array.prototype.map
can be created by looping through the array, applying a callback, and returning a new array. Always use feature detection, avoid polluting prototypes recklessly, and handle edge cases for polyfills.
Introduction:
JavaScript is a language, that is constantly evolving with new features and syntax. However, not all users use the latest browsers, and developers often face compatibility issues. This is where polyfills come into picture. In this blog, we’ll explore what polyfills are, why they’re essential, the core concepts behind writing them, and how to create a polyfill for the Array.prototype.map
method.
What Are Polyfills?
A polyfill is a piece of code that emulates a modern JavaScript feature in older environments that lack support for the new features. Think of it as a translator: if a browser doesn’t understand a new JavaScript method like Array.prototype.map
, a polyfill steps in and says, “Don’t worry—I’ll teach you how to do this!”
For example, the map
method was introduced in ECMAScript 5 (ES5). Older browsers like Internet Explorer 8 don’t support it. A polyfill allow to use map
even in those browsers by replicating its functionality.
Why Do We Need Polyfills?
1. Browser Fragmentation:
Browsers update at different speeds. While Chrome and Firefox auto-update, many users like to stay with outdated browsers (e.g., IE, older mobile browsers). Polyfills let developers use modern syntax without excluding these users.
2. Forward Compatibility:
Developers want to write code using the latest standards (ES6+), but not all users have access to environments that support these features. Polyfills act as a bridge.
3. Unified Codebase:
Instead of maintaining separate codebases for different browsers, polyfills let you write code once and ensure it works everywhere.
Key Concepts for Writing Polyfills:
1. Prototypes:
Most JavaScript methods (like map
, filter
, etc.) are added to an object’s prototype. For example, Array.prototype.map
means all arrays inherit this method. When writing a polyfill, we generaly extend the prototype of built-in objects.
// Adding a method to Array.prototype
Array.prototype.myMap = function(callback) { /* ... */ };
2. The this
Keyword
Inside a polyfill, this
refers to the object calling the method. For instance, in arr.map
()
, this
inside map
points to arr
. Properly handling this
is critical for polyfills to work.
3. Feature Detection:
Always check if a feature exists before polyfilling it. This avoids overwriting native implementations, which are faster and more reliable.
if (!Array.prototype.map) {
// Add the polyfill
}
4. Handling Edge Cases:
Native methods handle edge cases like sparse arrays, non-integer indices, and invalid callbacks. A good polyfill should replicate this behavior.
Example: Writing a Polyfill for Array.prototype.map
Let’s create a simplified polyfill for Array.prototype.map
to understand the process.
Step 1: Check for Existing Implementation
if (!Array.prototype.myMap) {
Array.prototype.myMap = function(callback) {
// Polyfill logic here
};
}
Step 2: Initialize a New Array
const newArray = [];
Step 3: Loop Through the Array
Use a for
loop to iterate over each element. The this
keyword refers to the array instance calling myMap
.
for (let i = 0; i < this.length; i++) {
// Apply the callback to each element
const transformedElement = callback(this[i], i, this);
newArray.push(transformedElement);
}
Step 4: Return the New Array
return newArray;
Full Polyfill Code:
if (!Array.prototype.myMap) {
Array.prototype.myMap = function(callback) {
const newArray = [];
for (let i = 0; i < this.length; i++) {
newArray.push(callback(this[i], i, this));
}
return newArray;
};
}
Flow Explanation with an Example
Let’s test our polyfill with an example:
const numbers = [1, 2, 3];
const doubled = numbers.myMap((num) => num * 2);
console.log(doubled); // Output: [2, 4, 6]
Step-by-Step Execution
Check for
myMap
: Since we’re using our polyfill,myMap
is called.Loop Through
numbers
: Thefor
loop runs fromi = 0
toi = 2
.Apply Callback: For each element,
callback(1, 0, [1,2,3])
,callback(2, 1, [1,2,3])
, etc., are executed.Build
newArray
: The results (2
,4
,6
) are pushed intonewArray
.Return Result:
[2, 4, 6]
is returned.
Improving the Polyfill:
The above example is simplified. A production-ready polyfill would handle:
thisArg
Parameter: Built-Inmap
accepts a second argument to set thethis
value inside the callback.Result Array: As built-in
map
function iterates through each and every element, it is clear that result array must be of same size as the original array.Loop:
map
method not only works on array but also on array-like-objects(objects that have length property) and internally iterates the array till length property is met and updates the result array with new values at appropriate keys not indexes.Sparse Arrays: Skipping empty slots (e.g.,
[1, , 3]
).Type Checks: Making sure the callback is a function.
For more details check: ECMA 2025 documentation
Here’s an enhanced version:
if (!Array.prototype.map) {
Array.prototype.map = function(callback, thisArg) {
if (typeof callback !== 'function') {
throw new TypeError(callback + ' is not a function');
}
const result = new Array(this.length);
let flag = 0;
while(flag<this.length){
if(this.hasOwnProperty(flag)) { //handling sparse array
const value = this[flag];
const newValue = callback.call(thisArg,value,flag,this);
result[flag] = newValue;
}
flag++;
}
return result;
};
}
Best Practices for Writing Polyfills
Feature Detection First:
Avoid overriding existing methods.Use IIFEs to Avoid Pollution:
Wrap polyfills in Immediately Invoked Function Expressions to limit scope:(function() { if (!Array.prototype.map) { /* ... */ } })();
Handle Edge Cases:
Check for valid callbacks, sparse arrays, and correctthis
binding.Performance:
Built-In methods are faster. Use polyfills sparingly and optimize loops.Security:
Modifying prototypes can lead to conflicts. UseObject.defineProperty
to make methods non-enumerable:Object.defineProperty(Array.prototype, 'myMap', { value: function(callback) { /* ... */ }, enumerable: false // Won’t show up in for...in loops });
Conclusion
Polyfills allow developers to use modern JavaScript while maintaining backward compatibility. By understanding prototypes, this
, and feature detection, robust polyfills can be written that replicate native behavior. Remember to test thoroughly, handle edge cases, and prioritize performance.
Join the Discussion:
We appreciate your thoughts and experiences with Polyfills! Kindly use the comment section to ask any questions, share your views, or discuss how you can apply this in real life.
Engage with Us:
👍 Did you find this article helpful? Give it a like!
💭 Share your favourite tech jokes in the comments.
🔔 Subscribe for more tech content that's educational and occasionally funny.
Share Your Feedback:
Your feedback helps us create better content. Drop a comment below about:
Your experience with Polyfills.
Suggestions for future technical articles.
Subscribe to my newsletter
Read articles from Abhijeet Sinha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
