Set Background Color for Paragraphs & Text in Word with Python [Tutorial]

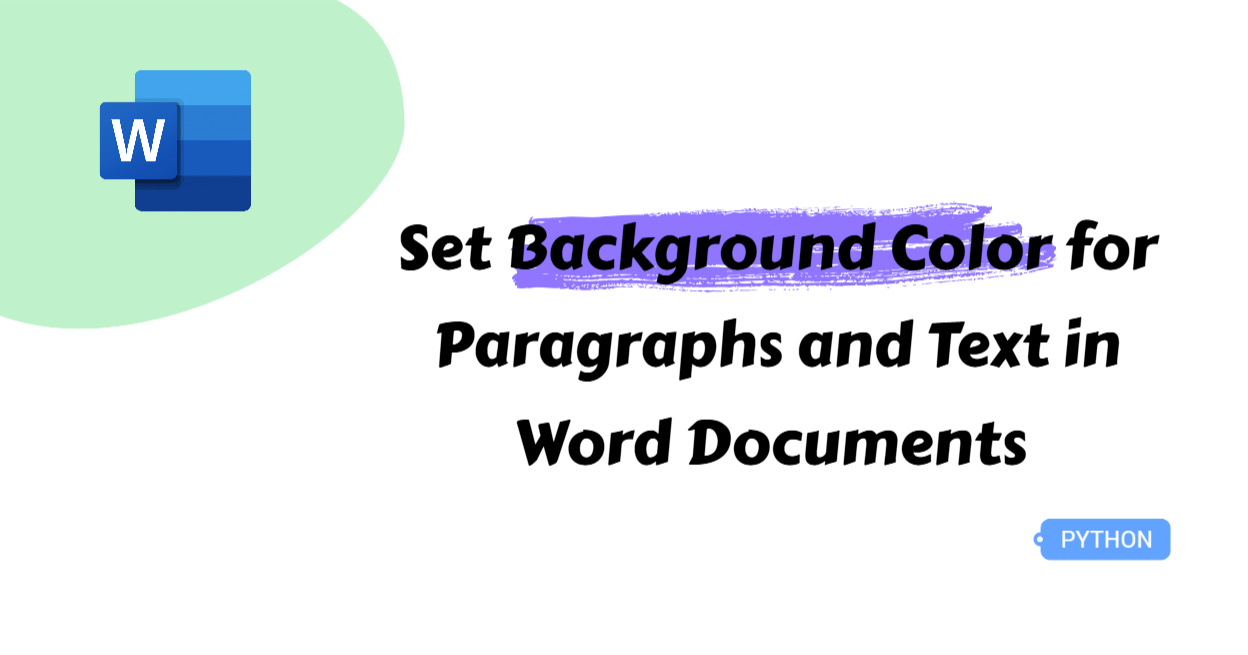
Word documents often contain extensive text, making it essential to highlight key information for better readability. Setting a background color for important content helps readers quickly identify crucial points. While Word allows manual formatting, automating this task with Python is far more efficient, especially for large documents. In this article, we’ll explore how to use Python to set background colors for paragraphs and text in Word, with clear step-by-step instructions and code examples to streamline the process.
Python Libraries to Set Background Color in Word Files
To simplify this process, using a Python library for Word document manipulation is essential. However, not all libraries support both paragraph and text background coloring. The commonly used python-docx allows setting background colors for text but lacks functionality for entire paragraphs.
Fortunately, enterprise libraries like Spire.Doc and Aspose.Words offer comprehensive solutions. These libraries not only support background color settings for both text and paragraphs but also provide advanced features such as adding background images, document formatting, and other processing tasks.
Among them, Spire.Doc stands out for its easy-to-use API, making it simple to implement these features. In this article, we’ll demonstrate how to set background colors for specific paragraphs and text using Spire.Doc. You can install it via pip: pip install Spire.Doc
.
How to Set Background Color for Paragraphs in Word with Python
Now that the preparation is complete, let’s dive into the main topic—how to customize the background color of a paragraph using Python. Highlighting key paragraphs with a background color helps draw attention to important information, enhancing readability. With Spire.Doc, you can easily achieve this using the Paragraph.Format.BackColor property. Let’s take a look at how it works!
Steps to set background color for paragraphs in Word documents:
Create a Document class.
Load a Word document from files through the Document.LoadFromFile() method.
Get a section using the Document.Sections[] property.
Get a paragraph with the Section.Paragraphs.get_Item() method.
Set a background color for the paragraph through the Paragraph.Format.BackColor property.
Save the updated Word file using the Document.SaveToFile() method.
Here is an example showing how to customize the background color for the 5th paragraph in the first section of a Word file:
from spire.doc import *
from spire.doc.common import *
# Create an instance of Document class and load a Word document
doc = Document()
doc.LoadFromFile("/AI-Generated Art.docx")
# Get the first section
section = doc.Sections.get_Item(0)
# Get the fifth paragraph
paragraph = section.Paragraphs.get_Item(4)
# Set background color for the paragraph
paragraph.Format.BackColor = Color.get_AliceBlue()
# Save the document
doc.SaveToFile("/ParagraphBackground.docx")
doc.Close()
Python to Set Background Color for Text in Word Documents
If the important information in a Word document is just a keyword or a phrase rather than a full paragraph, you’ll need to set the background color for specific text. This involves loading the source document, identifying the target words, phrases, or sentences, and then applying the background color using the TextRange.CharacterFormat.TextBackgroundColor property. Let’s go through the detailed steps to accomplish this.
Steps to set background color for text in a Word file:
Create an object of the Document class.
Read a Word file through the Document.LoadFromFile() method.
Find all the occurrences of a specific text using the Document.FindAllString() method.
Loop through all occurrences and get each of them as a text range with the TextSelection.GetAsOneRange(True) method.
Set a background color for the current text range through the TextRange.CharacterFormat.TextBackgroundColor property.
Save the modified Word document.
Here is an example of setting the background color of the word “AI” in a Word document:
from spire.doc import *
from spire.doc.common import *
# Create an instance of Document class and load a Word document
doc = Document()
doc.LoadFromFile("/AI-Generated Art.docx")
# Find text in the Word document
findResults = doc.FindAllString("AI", False, True)
# Loop through the finding results to set background color for all occurrences
for text in findResults:
# Get an occurrence as a text range
textRange = text.GetAsOneRange(True)
# Set the background color of the text range
textRange.CharacterFormat.TextBackgroundColor = Color.get_DarkGreen()
# Set the background color of a sepecified occurrence
# Get an occurrence as one text range
# textRange = findResults[1].GetAsOneRange()
# Set the background color of the text range
# textRange.CharacterFormat.BackgroundColor = Color.get_DarkCyan()
# Save the document
doc.SaveToFile("/TextBackground.docx")
doc.Close()
The Bottom Line
This guide demonstrates how to set background colors for paragraphs and text in Word using Python. With step-by-step instructions and code examples, you'll be able to customize background colors effortlessly and enhance document readability with ease!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
