How to Enhance Your Front End Projects with SEO
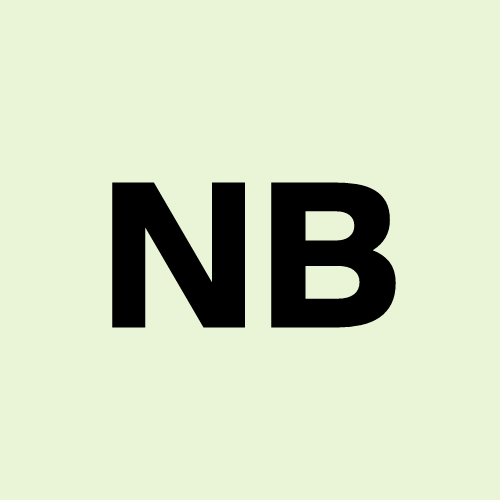
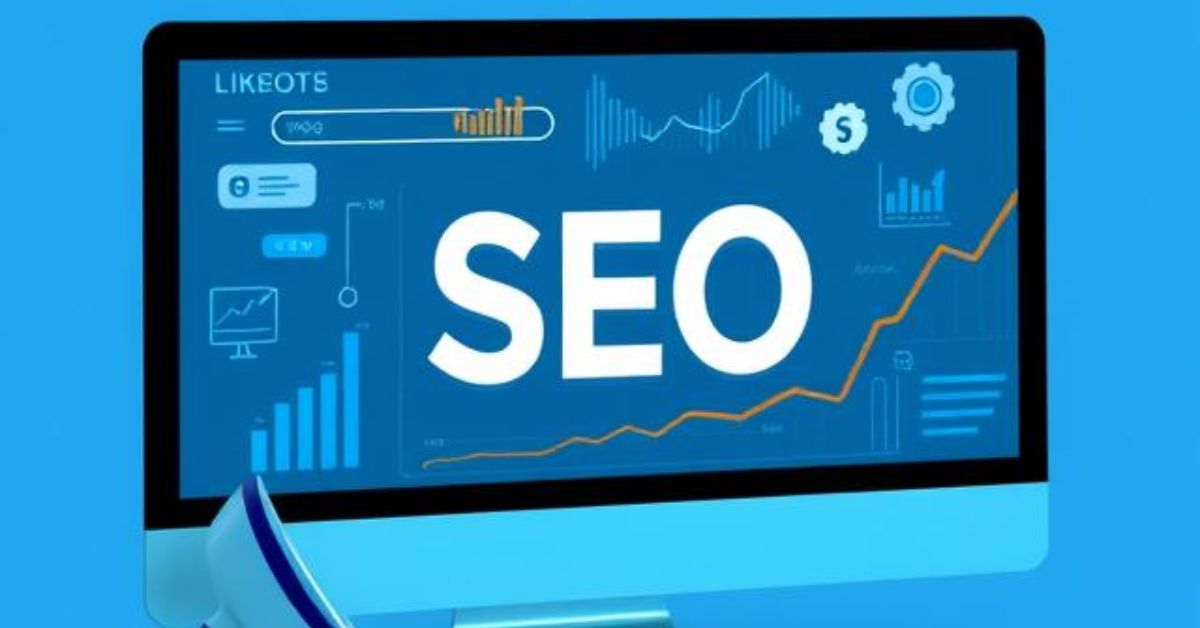
In today's digital landscape, creating visually appealing and functional front-end projects is only half the battle. Without proper search engine optimization (SEO), even the most impressive websites can remain hidden in the depths of search results. Let's explore how developers can enhance their front-end projects with effective SEO strategies that drive organic traffic and improve visibility.
Understanding the SEO-Frontend Connection
Frontend developers often focus on user experience, responsiveness, and aesthetics. However, incorporating SEO principles into the development process can significantly impact a website's performance in search engines. The good news is that many SEO improvements align perfectly with best practices for frontend development.
Core Web Vitals: Performance Meets SEO
Google's Core Web Vitals have become crucial ranking factors, directly connecting frontend performance with search visibility. These metrics include:
Largest Contentful Paint (LCP)
LCP measures loading performance—how quickly the largest content element becomes visible. To improve LCP:
Image Optimization for Better LCP
// Optimize images with modern formats and lazy loading
const optimizeImages = () => {
const images = document.querySelectorAll('img:not([loading])');
images.forEach(img => {
// Add lazy loading to images below the fold
if (!isInViewport(img)) {
img.setAttribute('loading', 'lazy');
}
// Add srcset for responsive images
if (!img.hasAttribute('srcset') && img.src) {
const src = img.src;
const fileExtension = src.split('.').pop();
if (['jpg', 'jpeg', 'png'].includes(fileExtension.toLowerCase())) {
const basePath = src.substring(0, src.lastIndexOf('.'));
img.setAttribute('srcset', `
${basePath}-small.${fileExtension} 400w,
${basePath}-medium.${fileExtension} 800w,
${basePath}.${fileExtension} 1200w
`);
img.setAttribute('sizes', '(max-width: 600px) 400px, (max-width: 1200px) 800px, 1200px');
}
}
});
};
// Helper function to check if element is in viewport
const isInViewport = (element) => {
const rect = element.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= window.innerHeight &&
rect.right <= window.innerWidth
);
};
// Run optimization when DOM is fully loaded
document.addEventListener('DOMContentLoaded', optimizeImages);
First Input Delay (FID) and Interaction to Next Paint (INP)
These metrics measure interactivity—how quickly your site responds to user interactions. Minimize JavaScript execution time by:
Splitting code into smaller chunks
Deferring non-critical JavaScript
Using web workers for complex operations
Cumulative Layout Shift (CLS)
CLS measures visual stability—how much elements move around as the page loads. To improve CLS:
Always include dimensions for images and videos
Reserve space for dynamic content
Avoid inserting content above existing content
Semantic HTML: The Foundation of SEO
Using semantic HTML elements helps search engines understand your content structure, which improves crawling and indexing efficiency.
Semantic HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Comprehensive guide to frontend development best practices for improved SEO performance">
<title>Frontend SEO Guide: Optimize Your Web Projects for Search Engines</title>
<link rel="canonical" href="https://yourwebsite.com/frontend-seo-guide">
</head>
<body>
<header>
<nav aria-label="Main Navigation">
<ul>
<li><a href="/">Home</a></li>
<li><a href="/services">Services</a></li>
<li><a href="/blog">Blog</a></li>
<li><a href="/contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<article>
<h1>Frontend SEO Guide: Optimize Your Web Projects</h1>
<section>
<h2>Understanding Core Web Vitals</h2>
<p>Core Web Vitals are essential metrics that measure user experience...</p>
</section>
<section>
<h2>Semantic HTML Best Practices</h2>
<p>Using the right HTML elements improves how search engines interpret your content...</p>
</section>
<aside>
<h3>Related Resources</h3>
<ul>
<li><a href="/blog/core-web-vitals">Deep Dive into Core Web Vitals</a></li>
<li><a href="/blog/structured-data">Structured Data Implementation</a></li>
</ul>
</aside>
</article>
</main>
<footer>
<section>
<h2>About Us</h2>
<p>We specialize in creating SEO-friendly frontend solutions.</p>
</section>
<p>© 2025 Your Company</p>
</footer>
</body>
</html>
Mobile-First Indexing
Google primarily uses the mobile version of your content for indexing and ranking. Ensure your frontend is responsive and provides an excellent mobile experience:
Implement responsive design patterns
Use viewport meta tags correctly
Test your site across multiple devices and screen sizes
Structured Data: Making Content Machine-Readable
Implementing structured data helps search engines understand your content context, potentially leading to rich snippets in search results.
Implementing JSON-LD Structured Data
// Add JSON-LD structured data to the page
document.addEventListener('DOMContentLoaded', () => {
// For a blog article
const articleStructuredData = {
"@context": "https://schema.org",
"@type": "BlogPosting",
"headline": document.querySelector('h1').textContent,
"datePublished": document.querySelector('meta[property="article:published_time"]')?.content || new Date().toISOString(),
"dateModified": document.querySelector('meta[property="article:modified_time"]')?.content || new Date().toISOString(),
"author": {
"@type": "Person",
"name": document.querySelector('.author-name')?.textContent || "Author Name"
},
"image": document.querySelector('meta[property="og:image"]')?.content || "",
"publisher": {
"@type": "Organization",
"name": "Your Company Name",
"logo": {
"@type": "ImageObject",
"url": "https://yourwebsite.com/logo.png"
}
},
"description": document.querySelector('meta[name="description"]')?.content || "",
"mainEntityOfPage": {
"@type": "WebPage",
"@id": window.location.href
}
};
// Create script element for JSON-LD
const script = document.createElement('script');
script.type = 'application/ld+json';
script.textContent = JSON.stringify(articleStructuredData);
// Append to head
document.head.appendChild(script);
});
Technical SEO Considerations for Frontend Developers
URL Structure
Create clean, descriptive URLs that reflect your content hierarchy. Avoid query parameters when possible, and use hyphens to separate words.
Meta Tags
Include essential meta tags in your HTML head section:
Essential SEO Meta Tags
<head>
<!-- Basic meta tags -->
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your Page Title - Brand Name</title>
<meta name="description" content="A concise, compelling description of your page content (150-160 characters)">
<!-- Canonical URL to prevent duplicate content issues -->
<link rel="canonical" href="https://yourwebsite.com/page-url">
<!-- Open Graph tags for social sharing -->
<meta property="og:title" content="Your Page Title">
<meta property="og:description" content="Your page description optimized for social sharing">
<meta property="og:image" content="https://yourwebsite.com/images/og-image.jpg">
<meta property="og:url" content="https://yourwebsite.com/page-url">
<meta property="og:type" content="website">
<!-- Twitter Card tags -->
<meta name="twitter:card" content="summary_large_image">
<meta name="twitter:title" content="Your Page Title">
<meta name="twitter:description" content="Your page description optimized for Twitter">
<meta name="twitter:image" content="https://yourwebsite.com/images/twitter-image.jpg">
</head>
Handling JavaScript-Heavy Applications
Single-page applications (SPAs) and JavaScript frameworks present unique SEO challenges. Consider these solutions:
Server-side rendering (SSR)
Static site generation (SSG)
Dynamic rendering for search engines
Implementing proper routing with History API
Content Optimization
While your design and technical implementation are important, content remains king in SEO. Frontend developers can contribute by:
Creating a clear content hierarchy using proper heading structure (H1-H6)
Ensuring text is accessible and not embedded in images
Using descriptive alt attributes for images
Balancing keyword usage naturally throughout the content
Measuring SEO Impact
Implement analytics and monitoring to track how your frontend optimizations affect SEO performance:
Set up Google Search Console and Google Analytics
Monitor Core Web Vitals through PageSpeed Insights and Lighthouse
Track rankings for target keywords
Analyze user behavior signals (bounce rate, time on page, etc.)
Working with SEO Professionals
While developers can implement many SEO improvements themselves, collaborating with an SEO agency or specialist can provide valuable insights tailored to your specific business goals and target audience. These professionals can help identify opportunities you might miss and create a comprehensive strategy that complements your frontend development work.
Conclusion
Enhancing your frontend projects with SEO isn't just about satisfying search engine algorithms—it's about creating better user experiences. By implementing the strategies outlined in this guide, you'll develop frontend projects that are not only visually appealing and functional but also discoverable by your target audience.
Remember that SEO is an ongoing process. Stay updated with the latest search engine guidelines, continuously monitor your performance metrics, and be prepared to adapt your frontend development practices as new standards emerge.
Subscribe to my newsletter
Read articles from Narinder Bhandari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
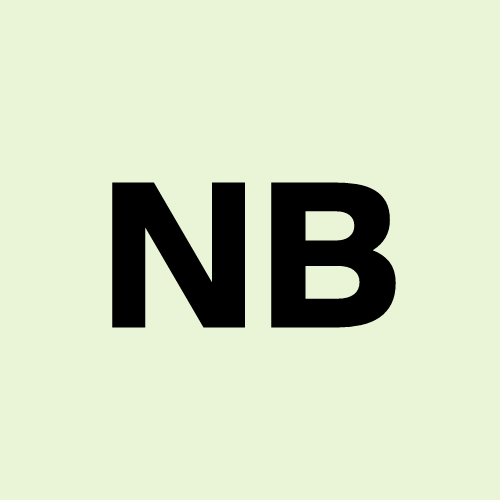