Mastering PowerShell Profiles
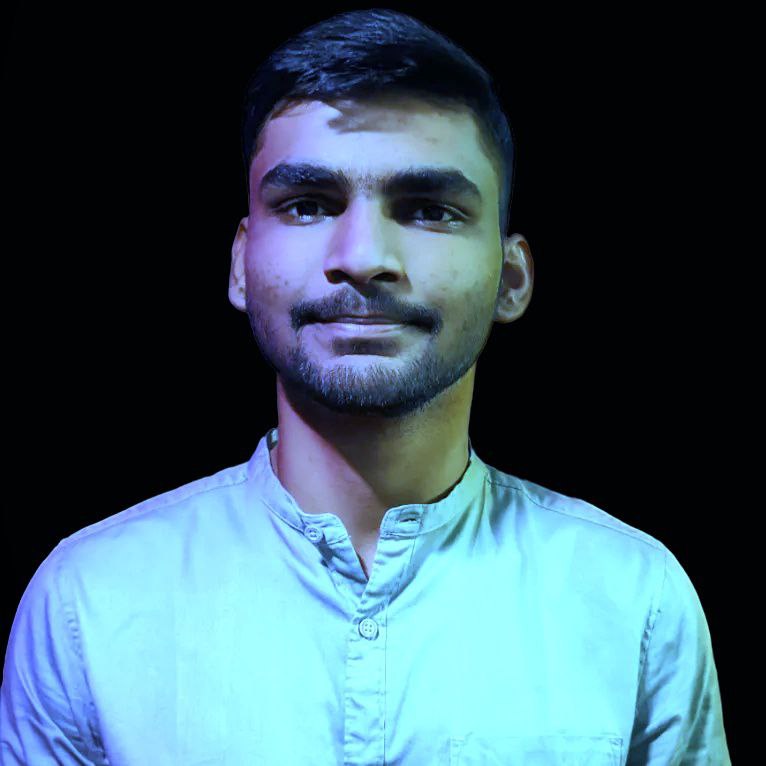
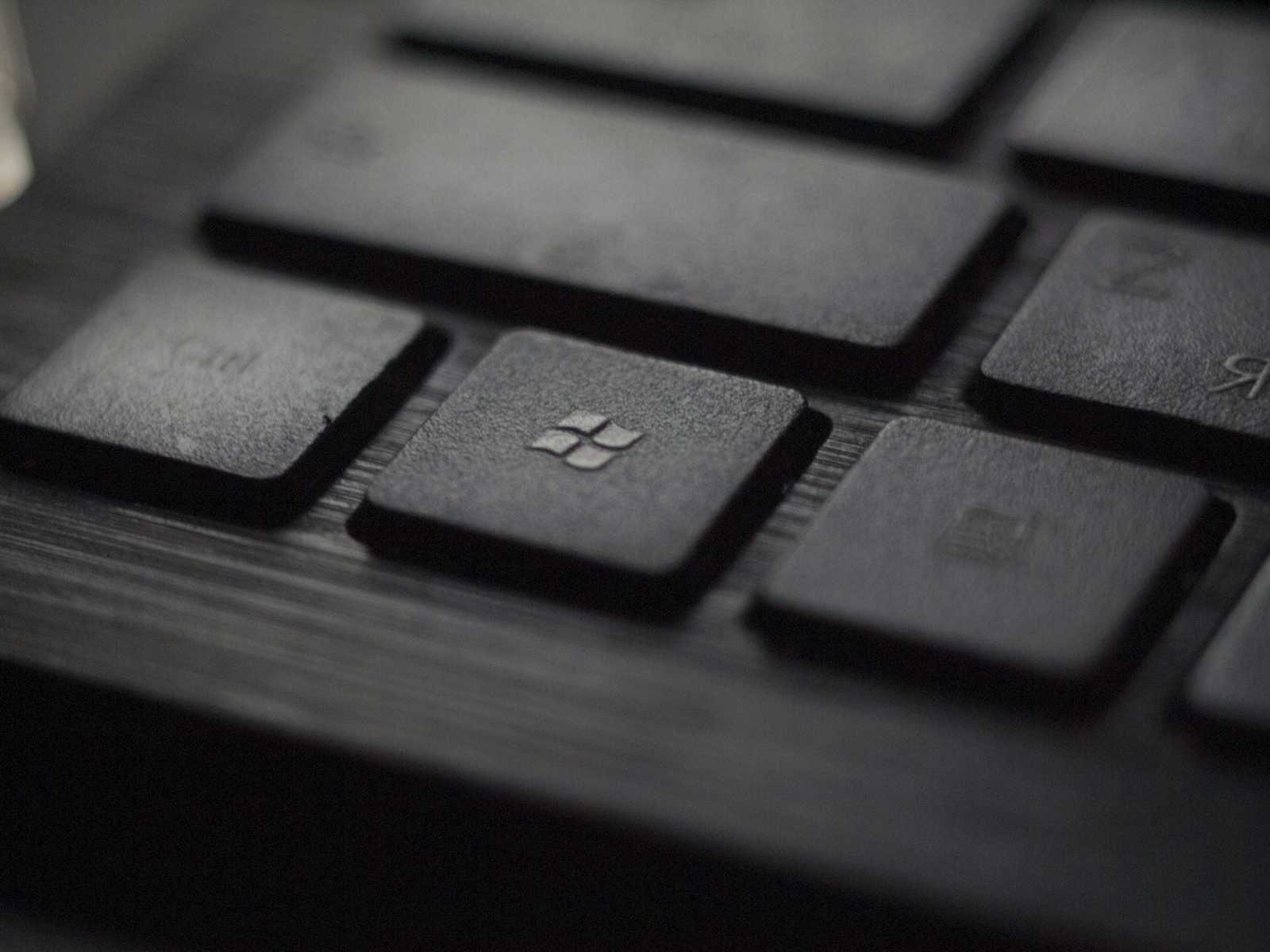
Introduction
PowerShell isn’t just a command line tool—It’s the Swiss Army knife of windows automation. Whether you’re managing files, deploying apps, or wrangling servers. But did you know you can make it even better? PowerShell Profiles; your secret weapon for aliases, shortcuts and automation spells.
Think of it like an automation script that runs everytime you launch PowerShell. Customize it, and it’ll unlock:
Productivity boosts (type less, do more!)
Personalized workflows (goodbye, repetitive tasks!)
A terminal that feels like home (rainbow prompts, anyone?)
Let’s dive in!
What is PowerShell profile?
A PowerShell profile is a script file (.ps1
) that runs automatically when you start a PowerShell session. It’s where you stash your favorite aliases, functions, and settings—like a “Welcome to My World” banner for your terminal.
There are four types of profiles, depending on scope:
All users, All hosts
This profile applies to all users and all PowerShell hosts on the system. It is located at
$PsHome\Profile.ps1
.All users, Current host
This profile applies to all users but only to the specific PowerShell host (e.g., console or ISE). It is located at
$PsHome\Microsoft.PowerShell_profile.ps1
.Current user, All hosts
This profile applies to the current user for all PowerShell hosts. It is located at
$Home\Documents\Profile.ps1
(or$Home\My Documents\Profile.ps1
in older versions).Current user, Current host
This profile applies to the current user and the specific PowerShell host. For the console, it is located at
$Home\Documents\WindowsPowerShell\Microsoft.PowerShell_profile.ps1
. For the ISE, it is located at$Home\Documents\WindowsPowerShell\Microsoft.PowerShellISE_profile.ps1
.
The $profile
variable in PowerShell contains the paths to these profiles. When PowerShell starts, it executes these profiles in a specific order, if they exist.
Check if you already have a profile:
Test-Path $PROFILE
If it returns False
, time to create one!
Creating a PowerShell profile
No profile? No problem! Run this.
New-Item -Path $PROFILE -ItemType File -Force
This will create the ps1
file for the profile on the location where it should be.
Now, edit it with Notepad (for the brave) or VS Code (for the fancy).
notepad $PROFILE # Classic Vibes
code $PROFILE # Modern Flair
Customizing your PowerShell Profile
Here’s where the fun begins!
Aliases: Shortcuts for Lazy Typists
Turn
Get-ChildItem
intoll
(because life’s short):
Set-Alias ll Get-ChildItem
Now ll
lists your files.
Auto-Load modules:
Hate typing
Import-Module Az
everytime? Add it to your profile:
Import-Module Az # Azure admins, rejoice
Custom functions
Create a function to nuke temp files:
function Clear-TempFiles {
Write-Host "Blasting junk into oblivion..." -ForegroundColor Red
Remove-Item -Path $env:TEMP\* -Recurse -Force
}
Run Clear-TempFiles
anytime.
Fancy Prompts
Make your prompt glow like a neon sign:
function prompt {
Write-Host "[$(Get-Date -Format 'HH:mm:ss')]" -NoNewline -ForegroundColor Cyan
Write-Host " PS $env:USERNAME@" -NoNewline -ForegroundColor Magenta
Write-Host "$env:COMPUTERNAME >" -ForegroundColor Yellow
return " "
}
Managing and Securing your profile
Secure credential storage
Never hardcode passwords! Use PowerShell’s
SecretManagement
module or encrypted files:
# Save credentials securely (uses Windows Data Protection API)
$credential = Get-Credential
$credential | Export-CliXml -Path "$env:USERPROFILE\secure_cred.xml"
# Load credentials later
$storedCred = Import-CliXml -Path "$env:USERPROFILE\secure_cred.xml"
Azure Key Vault Integration
For cloud users, fetch secrets dynamically from Azure Key Vault:
# Ensure Az module is imported first
function Get-MySecret {
param ($SecretName)
$secret = Get-AzKeyVaultSecret -VaultName "MyVault" -Name $SecretName -AsPlainText
return $secret
}
# Usage:
$apiKey = Get-MySecret -SecretName "ProdAPIKey"
Environment Variables for Sensitive Data
Store tokens in environment variables (safer than plain text in scripts):
# Set via PowerShell (temporary)
$env:GITHUB_TOKEN = "ghp_abc123"
# Or permanently in Windows:
[System.Environment]::SetEnvironmentVariable('GITHUB_TOKEN', 'ghp_abc123', 'User')
Advanced Use Cases for Power Users
Automated SSH connections with logging
Log SSH sessions and automate connections:
function Connect-DevServer {
$session = New-SSHSession -ComputerName "dev.example.com" -Credential $storedCred
Start-SSHStream -SessionId $session.SessionId -ShellStream "DevSession"
# Log the session
$timestamp = Get-Date -Format "yyyyMMdd-HHmmss"
Start-Transcript -Path "$env:USERPROFILE\ssh_logs\$timestamp.txt"
}
Custom Command Logging
Track every command you run in a searchable JSON file:
$global:CommandHistoryPath = "$env:USERPROFILE\ps_history.json"
function Log-Command {
param ($command)
$logEntry = @{
Timestamp = Get-Date -Format "o"
Command = $command
User = $env:USERNAME
Host = $env:COMPUTERNAME
} | ConvertTo-Json
Add-Content -Path $global:CommandHistoryPath -Value $logEntry
}
# Override the default Add-History to log
$originalAddHistory = Get-Command Add-History
function Add-History {
param([string[]]$Lines)
$Lines | ForEach-Object { Log-Command $_ }
& $originalAddHistory @PSBoundParameters
}
Dynamic Environment Switcher
Auto-load AWS/Azure/GCP profiles based on your current project folder:
function Set-CloudEnv {
param ($Environment)
switch ($Environment) {
"AWS-Prod" {
$env:AWS_ACCESS_KEY_ID = (Get-MySecret -SecretName "AWSProdKey")
$env:AWS_SECRET_ACCESS_KEY = (Get-MySecret -SecretName "AWSProdSecret")
Write-Host "AWS Production environment loaded!" -ForegroundColor Yellow
}
"Azure-Dev" {
Connect-AzAccount -Credential $storedCred
Set-AzContext -SubscriptionId "12345-abcde"
Write-Host "Azure Dev environment loaded!" -ForegroundColor Cyan
}
}
}
Some security tips
Rotate credentials regularly: Automate token refreshes with Azure AD or AWS IAM roles.
Audit your profile: Use
Unblock-File $PROFILE
if scripts are blocked by Windows.Use JEA (Just Enough Administration): Restrict PowerShell capabilities for non-admin users.
Experiment with these snippets, and remember: with great power comes great automation! 🔥
Subscribe to my newsletter
Read articles from Pranav Tripathi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
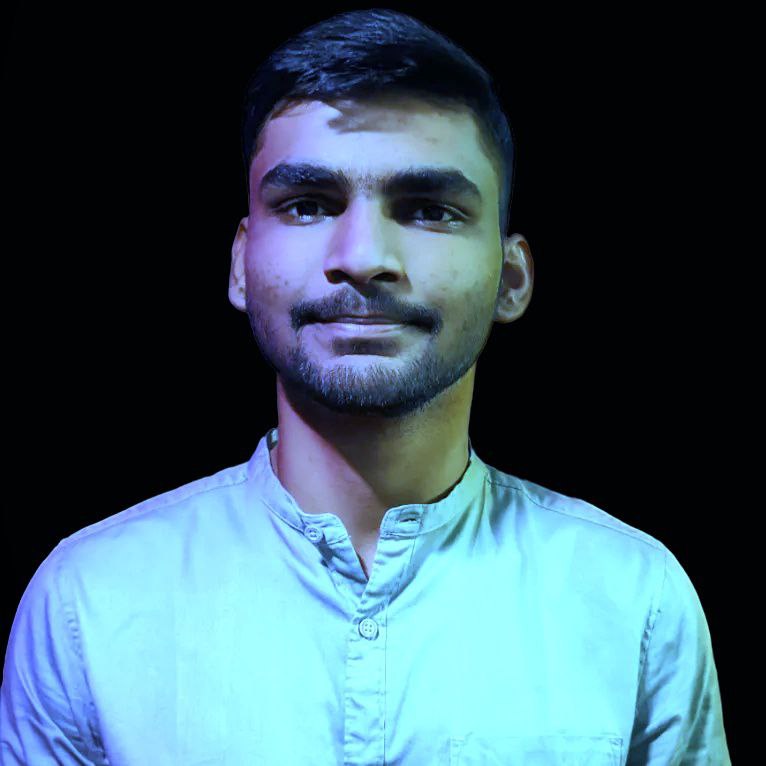
Pranav Tripathi
Pranav Tripathi
I'm a dedicated full-stack developer specializing in building dynamic and scalable applications. I have a strong focus on delivering efficient and user-friendly solutions, and I thrive on solving complex problems through technology.