Why Your Website is Dead Without Event Handling (And How to Fix It)

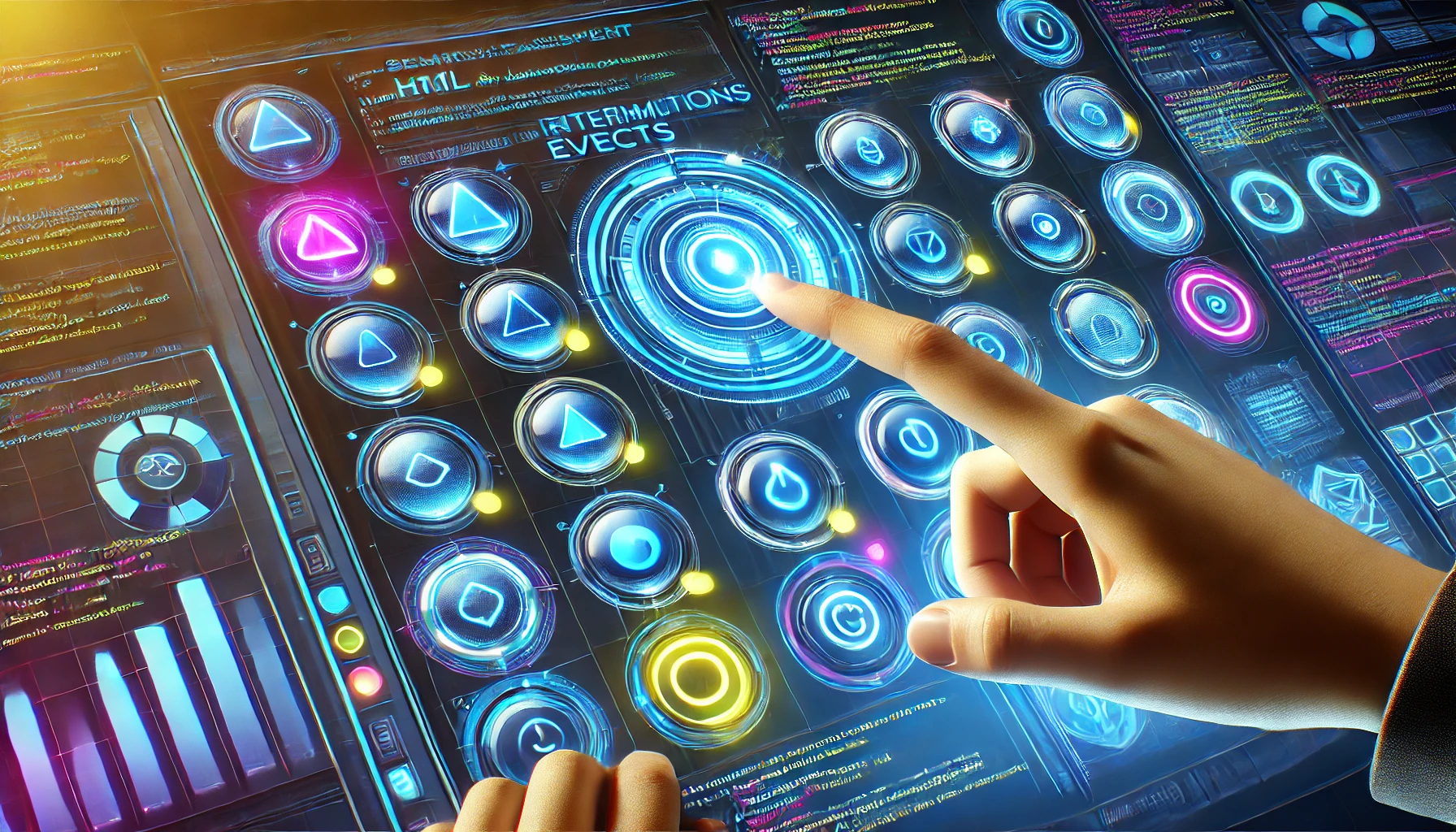
You've built a beautiful website with perfect HTML and CSS. It looks stunning. But when users visit, they click buttons and... nothing happens. Your masterpiece feels lifeless. That's because you're missing the secret ingredient that brings websites to life: event handling.
Have you ever wondered what makes applications like Gmail, Twitter, or even simple forms respond instantly to your actions? It's not magic—it's JavaScript events working behind the scenes.
What Are Events Anyway?
Events are simply things that happen in the browser—like a user clicking a button, scrolling down a page, or typing in a text field. But without proper event handling, your website is just sitting there, unable to respond to these user actions.
Think of events as messengers telling your code: "Hey! Something just happened! Do you want to respond to it?"
The Anatomy of JavaScript Events
Before diving into examples, let's understand the structure of events:
Event target: The HTML element where the event originated
Event type: What kind of action occurred (click, keypress, load, etc.)
Event handler: The function that executes in response to the event
Event object: Contains information about the event
Did you know the DOM (Document Object Model) registers over 200 different types of events? Most developers only use about a dozen regularly, but knowing they exist gives you tremendous power to create interactive experiences.
Making Elements Listen: Event Listeners
The most common way to handle events is by adding event listeners to DOM elements. This tells the browser: "Watch this element, and when a specific event happens, run this function."
Here's a simple example:
// Select the button
const button = document.querySelector('#myButton');
// Add an event listener for clicks
button.addEventListener('click', function() {
// This code runs when the button is clicked
document.body.style.backgroundColor = 'skyblue';
alert('You clicked the button!');
});
That's it! Just three parts:
The target element (
button
)The event type (
'click'
)The handler function (what happens when the event occurs)
Fun fact: Before addEventListener
became standard, developers had to use inline event handlers like <button onclick="doSomething()">
. While you might still see this in legacy code, the modern approach provides better separation of concerns.
The Event Object: Your Information Goldmine
When an event happens, JavaScript automatically creates an event object containing valuable information. This object is passed to your event handler function:
const input = document.querySelector('input');
input.addEventListener('keyup', function(event) {
// 'event' contains information about what just happened
console.log(`You pressed: ${event.key}`);
console.log(`Key code: ${event.keyCode}`);
// We can also see which element triggered the event
console.log(`Target element: ${event.target.tagName}`);
});
Ever wondered how autocomplete suggestions appear as you type? They use these exact event objects to detect keystrokes and respond accordingly.
Event Propagation: The Hidden Waterfall
Here's where things get interesting—and a bit mind-bending. When an event occurs on an element, it doesn't just stay there. It travels through the DOM in a fascinating way.
When you click on a nested element (like a button inside a div inside another div), the event travels in three phases:
Capturing phase: The event travels DOWN from the window to the target element
Target phase: The event reaches the target
Bubbling phase: The event travels UP from the target back to the window
By default, event listeners are triggered during the bubbling phase. But you can change this by setting the third parameter of addEventListener
to true
:
// This will trigger during the capturing phase
element.addEventListener('click', handleClick, true);
// This triggers during bubbling (default behavior)
element.addEventListener('click', handleClick, false);
Did you know this event propagation model was a major point of contention during the browser wars of the late 1990s? Netscape championed event capturing, while Microsoft preferred event bubbling. The W3C wisely decided to include both in the standard!
Stopping Propagation: The Emergency Brake
Sometimes you want to prevent an event from traveling further. For example, when clicking a button inside a clickable container, you might want only the button's click handler to run.
That's where stopPropagation()
comes in:
childElement.addEventListener('click', function(event) {
// This prevents the event from bubbling up
event.stopPropagation();
console.log('Child clicked!');
});
parentElement.addEventListener('click', function() {
// This won't run when child is clicked
console.log('Parent clicked!');
});
Preventing Default Behavior: Taking Control
Many HTML elements have default behaviors. Links navigate to URLs, form submissions reload the page, and checkboxes toggle on and off. Sometimes you want to override these behaviors.
Enter preventDefault()
:
// Select a form
const form = document.querySelector('form');
// Add submit event listener
form.addEventListener('submit', function(event) {
// Prevent the form from submitting normally
event.preventDefault();
// Custom form handling code here
validateForm();
submitViaAjax();
});
This is how single-page applications work their magic—they intercept link clicks and form submissions to handle navigation without page reloads!
Event Delegation: The Performance Hack
Imagine you have a list with 100 items, and each needs a click handler. Adding 100 separate event listeners would be inefficient. Instead, you can use event delegation:
// Instead of 100 event listeners on each <li>
const list = document.querySelector('ul');
// One event listener on the parent <ul>
list.addEventListener('click', function(event) {
// Check if the clicked element is an <li>
if (event.target.tagName === 'LI') {
console.log('List item clicked:', event.target.textContent);
}
});
This works because of event bubbling—the event starts at the list item and bubbles up to the parent list, where your single event handler catches it.
Many JavaScript frameworks use this pattern internally to minimize memory usage and improve performance. Pretty clever, right?
Real-World Example: Interactive Form
Let's bring it all together with a practical example—a form with real-time validation:
document.addEventListener('DOMContentLoaded', function() {
const form = document.getElementById('signupForm');
const emailInput = document.getElementById('email');
const passwordInput = document.getElementById('password');
const submitButton = document.getElementById('submit');
// Validate email format as user types
emailInput.addEventListener('input', function(event) {
const email = event.target.value;
const isValid = /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email);
if (isValid) {
emailInput.classList.remove('invalid');
emailInput.classList.add('valid');
} else {
emailInput.classList.remove('valid');
emailInput.classList.add('invalid');
}
// Update submit button state
updateSubmitButton();
});
// Check password strength
passwordInput.addEventListener('input', function(event) {
const password = event.target.value;
const isStrong = password.length >= 8;
if (isStrong) {
passwordInput.classList.remove('invalid');
passwordInput.classList.add('valid');
} else {
passwordInput.classList.remove('valid');
passwordInput.classList.add('invalid');
}
// Update submit button state
updateSubmitButton();
});
// Form submission
form.addEventListener('submit', function(event) {
event.preventDefault();
console.log('Form submitted!');
// AJAX submission would go here
});
function updateSubmitButton() {
const emailValid = emailInput.classList.contains('valid');
const passwordValid = passwordInput.classList.contains('valid');
submitButton.disabled = !(emailValid && passwordValid);
}
});
Wrapping Up
Event handling is what transforms static web pages into dynamic, interactive applications. Without it, the web would still be a collection of linked documents rather than the interactive platform we use daily.
Remember these key points:
Use
addEventListener
to make elements respond to user actionsThe event object provides crucial context about what happened
Understanding event propagation helps debug tricky issues
Event delegation can significantly improve performance
Preventing default behaviors gives you control over the browser
Now go forth and make your websites come alive with the power of events! Your users (and your portfolio) will thank you.
What's your favorite use of event handling on the web? Share in the comments below!
Subscribe to my newsletter
Read articles from Jatin Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
