A Deep Dive into Web Development š
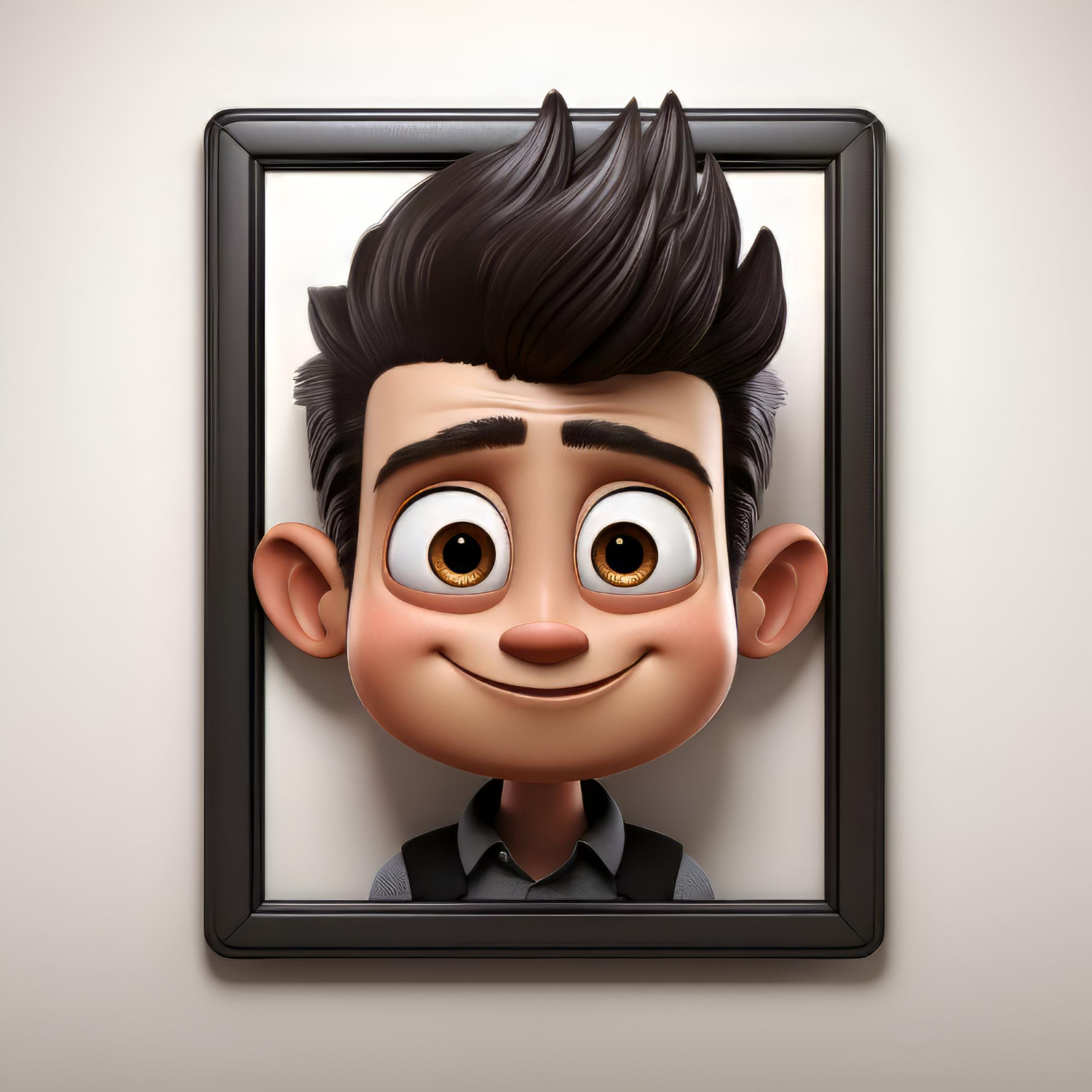
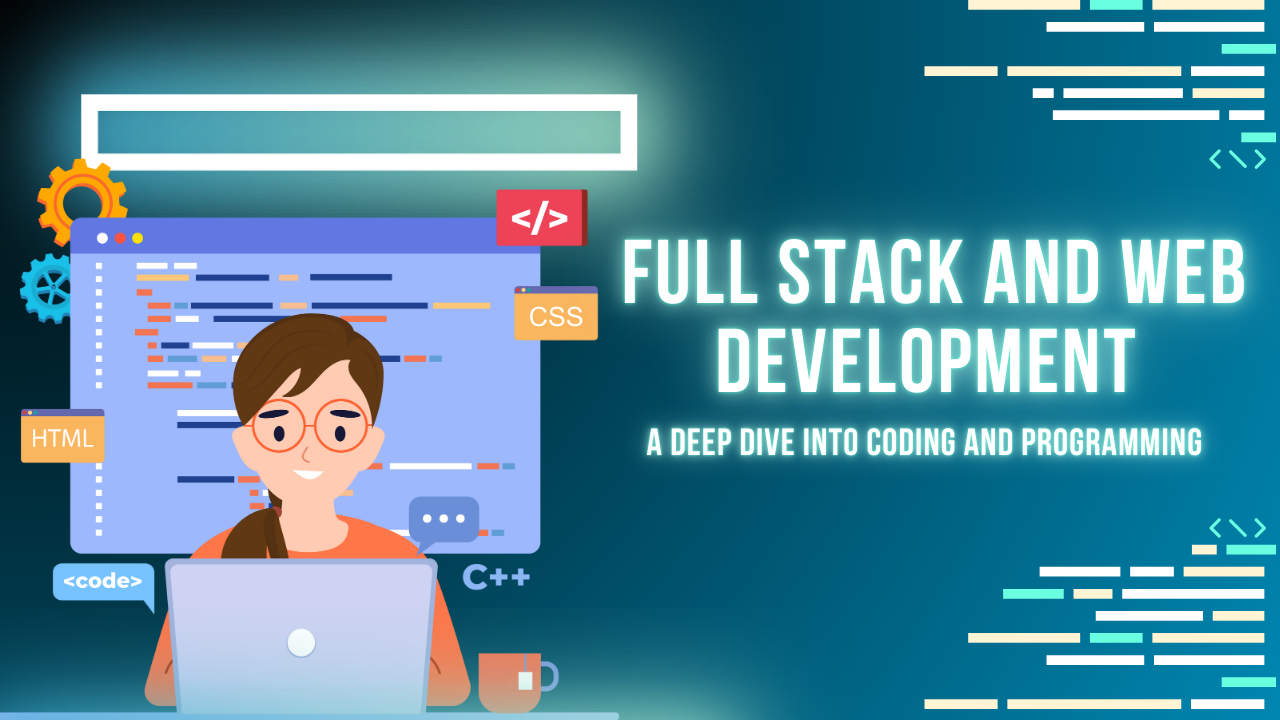
Chapter 1: The Morning Craving ā The Beginning of a Web Request
You wake up on a rainy morning in Bengaluru, craving a hot cup of chai. Instead of making it at home, you grab your phone and visit Chai Wavesā website to order your favorite A Large Chai.
The moment you hit Enter, a fascinating journey begins. The website doesnāt just appear magicallyāitās built on a complex foundation of frontend and backend technologies, each working in perfect harmony to ensure you get your chai, hot, fresh, and masaledaarš.
Your browser (the client) sends a request to the server, saying:
š¬ āHey, I need the homepage of Chai Waves. Show me the menu!ā
How Does This Work?
The request is sent using the HTTP protocol to a remote web server.
The server looks at the request, retrieves the required HTML, CSS, and JavaScript files, and sends them back to your browser.
Your browser then renders the page beautifully, loading the menu, images, and layout exactly as the developers designed it.
ā
Key Concept: The Frontend-
The frontend is everything you see on your screenāthe design, colors, fonts, buttons, and images.
Itās built using three core technologies:
HTML (Hyper Text Markup Language): The āskeletonā of the webpage.
CSS (Cascading Style Sheets): The āskin and clothesā that style everything.
JavaScript: The āmusclesā that make the webpage interactive.
š” Analogy: Think of the chai shopās website as a restaurant menu:
HTML = The list of items (structure).
CSS = The menuās design (colors, fonts).
JavaScript = The waiter who interacts with you (interactivity).
Now that the homepage has loaded, it's time to explore the menu!
Chapter 2: The Art of Browsing ā How Data Loads Dynamically
As you scroll through the chai menu, have you noticed something? The page doesnāt reload! Instead, new chai items appear smoothly. This is asynchronous data loading at play.
ā You decide to check out the specialty chai section. Instead of refreshing the whole page, the system fetches only the needed data from the server.
How Does This Work?
The website uses AJAX (Asynchronous JavaScript and XML) or the Fetch API to request only the needed chai menu details.
Instead of sending a new request for the entire webpage, your browser asks for just the updated menu section.
The data is retrieved in JSON (JavaScript Object Notation) format and is displayed without reloading the page.
ā
Key Concept: Asynchronous Updates-
This makes websites fast and interactive, preventing unnecessary page loads.
š” Analogy: Imagine being at a restaurant where the waiter hands you a new section of the menu without taking away the entire booklet.
š¹ Real-Life Example: Ever noticed how when you type in Google Search, suggestions appear instantly? Thatās asynchronous data fetching in action!
With the menu in place, you decide: Masala Chai with extra ginger. Itās time to order!
Chapter 3: Adding to Cart ā The Role of Databases & Indexing
Clicking "Add to Cart" may seem like a simple action, but behind the scenes, a powerful database system is working to:
Verify if ginger is in stock.
Retrieve price details.
Apply any loyalty discounts (if you have them).
How Does the Database Search Work?
The chai shop's backend uses a Relational Database (SQL) or NoSQL database to store information about:
Chai types, ingredients, and prices.
Customer orders and history.
Available stock in real-time.
Since searching databases can be slow with large amounts of data, indexing speeds up the process.
ā
Key Concept: Indexing for Fast Search-
Instead of scanning the entire database, the system looks at a pre-organized index to find results instantly.
š” Analogy: Think of it as finding a word in a dictionary using the alphabetical index instead of reading the whole book.
š¹ Real-Life Example: Amazonās "One-Click Buy" feature retrieves product details in milliseconds using database indexing!
Your chai is now in the cart, but the real magic happens when you place the order.
Chapter 4: Placing the Order ā API Calls & Backend Processing
So, youāve decided on your Masala Chai with extra ginger, added it to your cart, and now youāre ready to place the order. You click the "Place Order" button, expecting everything to just work. But behind this single click, a complex chain of backend processes kicks into action, ensuring your order is accurately placed, processed, and confirmed.
4.1. The Request Journey ā From Frontend to Backend
As soon as you press "Place Order", your browser sends a request to the chai shopās server. But what exactly happens in this process?
Frontend (Client-Side) Action:
Your browser captures your order details (selected chai, size, add-ons, quantity, and total price).
These details are formatted into a structured request (usually JSON format) and sent to the backend via an API call.
API Request Handling:
The request is received by the web server and passed to the backend API (Application Programming Interface).
APIs are the bridge between the frontend and backend, ensuring that data is securely transmitted and processed.
Backend (Server-Side) Processing:
The backend server reads the order details and processes the request.
It interacts with the database to:
Verify if the selected chai is still available in stock.
Calculate the final price, including taxes and discounts.
Assign a unique order ID to track your order.
Once all checks are complete, the system confirms your order and sends a response back to the frontend.
Response Sent Back to Client:
The frontend receives a response from the server:
{ "order_id": "CFE12345", "status": "Confirmed", "estimated_time": "15 mins" }
The confirmation message, "Your order has been placed successfully!", appears on your screen, and the order tracking page is activated.
ā
Key Concept: REST APIs & Server-Side Processing (Week 6 & 7)
REST APIs act like waiters in a restaurant. Your frontend (customer) tells the waiter (API) what they want, and the waiter delivers this request to the kitchen (backend server). Once the kitchen prepares the food (processes the request), the waiter brings it back to the table (response to the frontend).
4.2. The Role of the Database ā Ensuring Order Accuracy
At this stage, the database plays a crucial role in storing and managing your order details.
š¹ How does the database handle your order?
The order details are inserted into the database under a table named
Orders
.A separate
Inventory
table is checked to ensure the ingredients required for your chai are available.The
Users
table is updated to store your purchase history (for loyalty rewards and order history).
š¹ Why is Database Indexing Important?
Imagine thousands of people ordering chai simultaneously. Searching for each customerās order in a huge database can be slow. Thatās where database indexing comes ināit organizes data efficiently, just like a table of contents in a book, making searches faster.
š¹ Example of an SQL Query Used in This Process:
insert into Orders (user_id, chai_type, size, addons, total_price, status)
values (123, 'Masala Chai', 'Larhe', 'Ginger', 5.99, 'Confirmed');
This SQL query ensures your order is recorded and ready to be processed by the cafƩ.
š” Analogy: Think of a restaurant waiter writing down your order and passing it to the kitchen. If the kitchen runs out of ingredients, they alert the waiter, who then informs you.
Chapter 5: Secure Payment ā Encryption, SQL Injection Prevention & HTTPS
Now that your order is confirmed, itās time to pay for your chai. You enter your credit card details, expecting a smooth checkout process. But behind the scenes, multiple security measures are in place to protect your sensitive financial data.
5.1. The Secure Payment Process ā Step by Step
š How does the system handle your payment securely?
Entering Payment Information:
You type your credit card number, CVV, and expiry date into the payment form.
Your browser encrypts this data before sending it to the payment gateway.
Secure Transmission via HTTPS:
The payment details are sent over an HTTPS connection.
HTTPS (HyperText Transfer Protocol Secure) ensures that all data is encrypted during transmission, preventing hackers from intercepting it.
Payment Gateway Processing:
The request is sent to a third-party payment gateway (e.g., Stripe, PayPal, Razorpay).
The payment gateway verifies:
Whether the credit card is valid.
Whether sufficient funds are available.
If the transaction follows anti-fraud protocols.
Bank Authorization:
The payment gateway forwards the request to your bank.
The bank approves or rejects the transaction based on available balance and security checks.
Response Sent Back to Server:
If successful, the payment gateway sends a "Payment Successful" response.
The order status is updated in the database from
"Pending"
to"Paid"
.You receive a confirmation message: "Your payment has been processed successfully!"
ā
Key Concept: HTTPS Encryption-
HTTPS is like sealing a confidential letter before sending it through the mailāit ensures that no one can read or modify your data while itās being transmitted.
5.2. Preventing SQL Injection ā Stopping Hackers from Stealing Data
šØ What if a hacker tries to manipulate the payment system?
One of the most common hacking techniques is SQL Injection, where an attacker inserts malicious SQL code into input fields to steal or modify data.
š¹ Example of a Malicious SQL Injection Attack:
Imagine a hacker enters the following into the payment form:
' or 1=1; --
If the system is not protected, this could trick the database into giving access to all user payment details!
š¹ How Do Websites Prevent SQL Injection?
Using Parameterized Queries: Instead of raw SQL, we use prepared statements to ensure inputs are treated only as data, not as executable code.
Validating User Inputs: Ensuring no special characters or unexpected symbols are allowed.
š¹ Secure SQL Query Example (Prevention Method):
select * from Users where username = ? and password = ?
Here, the "?" placeholders ensure only valid inputs are processed, making SQL injection impossible.
ā
Key Concept: SQL Injection Prevention & Web Security-
By sanitizing inputs and using prepared statements, the system ensures that only valid transactions are processed, protecting both the business and its customers.
5.3. Final Step ā Payment Confirmation Email
Once payment is complete, the system sends a receipt via email using an automated email API.
š” Analogy: This is like getting a printed receipt at a store after paying for your chai.
ā
Key Concept: Secure Transactions & Data Protection-
The entire payment process follows strict data security protocols to prevent fraud, theft, and unauthorized access.
Chapter 6: Real-Time Tracking ā Web Sockets, REST APIs & Load Balancing
With your payment successfully processed, the chai shopās system is now fully engaged in getting your order ready. But how do you know whatās happening with your order?
You open the order tracking page, and there it isāa real-time status update:
ā
Order Received
š Being Prepared
š² Out for Delivery
ā Delivered
Itās almost like watching a live feed of your chaiās journey from the chaiwale bhaiya to your doorstep. But have you ever wondered how this real-time tracking works?
6.1. REST API vs. WebSockets ā How Order Tracking Works
There are two main ways websites update information:
REST API (Pull-Based Updates)
Every few seconds, your browser asks the server, "Has my order status changed?"
The server responds with the latest status.
This method is inefficient because it constantly asks for updates, even when nothing has changed.
WebSockets (Push-Based Updates ā The Better Way)
Instead of asking repeatedly, your browser opens a direct connection to the server.
Whenever the status changes, the server instantly sends the update to your screen.
This is faster and more efficient than REST APIs.
ā Key Concept: REST APIs vs. WebSockets-
REST APIs ā Like calling a restaurant every 5 minutes to ask, "Is my food ready?"
WebSockets ā Like getting a notification on your phone when your food is ready.
š” Analogy: Imagine you order a pizza delivery:
If you keep calling the store every 5 minutes to check, thatās REST APIs (polling).
If the store texts you automatically when the pizza is out for delivery, thatās WebSockets.
Since order tracking doesnāt require high traffic, WebSockets are used for instant updates!
6.2. Load Balancing ā Handling Thousands of Orders at Once
Now, imagine thousands of customers ordering coffee at the same time. How does the system prevent overloading and crashing?
š¹ This is where Load Balancers come in!
š How Load Balancers Work:
They distribute incoming traffic across multiple servers.
This prevents one server from getting overloaded while others sit idle.
Load balancers redirect traffic dynamically based on server health and traffic levels.
ā
Key Concept: Load Balancing-
Load balancing ensures that millions of users can place orders simultaneously without delays.
š” Analogy: Imagine a crowded Starbucks with multiple baristas.
Instead of all customers going to one counter, a manager directs them to different counters, making the service faster and smoother.
This is exactly how load balancers distribute traffic across multiple web servers.
6.3. Caching ā Why Your Order Summary Loads Instantly
Even though your order status updates dynamically, some parts of the page donāt change oftenālike the menu, pricing, and your order history. Instead of querying the database every time you check your order, the system uses caching.
š How Caching Works:
A copy of frequently accessed data (like your order summary) is stored temporarily.
When you refresh the page, instead of querying the database, the system retrieves the cached copy instantly.
ā Key Concept: Caching for Faster Performance-
Without caching: "Let me check the database every time."
With caching: "I already have this information, no need to check again."
š” Analogy: Imagine a bartender memorizing your regular drink order so you donāt have to repeat it every time.
š¹ Real-Life Example:
When you revisit Amazon, it loads your cart instantlyāeven if you haven't logged in. Thatās because it uses caching to store your cart locally!
Now that you know exactly where your order is, what happens when the chai finally arrives?
Chapter 7: The Final Sip ā The Last Mile of Web Development
At last! The delivery person rings your doorbell. You open the door, take the cup of chai, and breathe in the aroma. Your order is complete.
But waitāthereās still one final step.
7.1. Rating Your Experience ā More Backend Magic
As soon as you take your first sip, a rating pop-up appears on your screen:
ā ā ā ā ā ā "How was your experience?"
What happens when you rate your order?
Frontend Interaction:
As soon as you click a star rating, JavaScript captures your feedback.
The rating is sent to the server using an API request.
Backend Processing:
The rating is stored in the database under a table called
OrderFeedback
.The system calculates an average rating for that specific coffee type.
The coffee shop analyzes feedback to improve their services.
ā
Key Concept: Storing & Analyzing User Feedback-
By collecting feedback, the chai shop can:
Identify popular menu items.
Improve service based on user reviews.
Boost customer satisfaction.
š” Analogy: Imagine being at a restaurant, and the waiter asks, "Did you enjoy your meal?" Your answer helps improve the overall experience for future customers.
7.2. Sending You a Receipt ā Email API Integration
Right after you rate your order, you receive an email confirmation with:
A digital receipt for your purchase.
A promo code for your next visit.
A reminder to join their loyalty program.
š¹ How Does This Work?
The system automatically triggers an API request to an email service provider (e.g., SendGrid, Mailgun, AWS SES).
The email template is generated and customized with your order details.
The email is sent securely to your inbox!
ā
Key Concept: Automated Email APIs-
Modern applications automate emails instead of sending them manually.
š” Analogy: Imagine a restaurant automatically sending you a thank-you text after dining there.
š¹ Real-Life Example: Every time you order from Swiggy, Zomato, or Amazon, you receive an email confirmation instantly.
7.3. The Power of Analytics ā How Your Order Improves Future Service
Even though your order is complete, your data is still valuable. The coffee shop's system:
Analyzes which chai flavors are trending.
Tracks busy hours to optimize staffing.
Uses machine learning to predict inventory needs.
ā
Key Concept: Data-Driven Decision Making-
Every order placed contributes to improving the next customerās experience.
š” Analogy: Just like Netflix analyzes your watch history to recommend shows, coffee shops analyze past orders to optimize their menu.
Final Thoughts ā The Magic Behind a Simple Chai Order
From the moment you clicked "Place Order" to your first sip, you just witnessed:
ā
Frontend (JavaScript, Async Updates, DOM Manipulation)
ā
Backend (APIs, Databases, Server-Side Processing, WebSockets)
ā
Security (HTTPS, SQL Injection Prevention, Authentication)
ā
Performance (Caching, Load Balancing, Indexing)
ā
Scalability (WebSockets, Cloud Infrastructure, Replication)
Conclusion: The Magic Behind a Simple Click
What seemed like a simple chai order was actually a complex orchestration of web technologies working seamlessly in the background. From the moment you typed the chai shopās URL to the second you took your first sip, multiple systemsāincluding frontend rendering, asynchronous updates, APIs, databases, security protocols, and real-time trackingāensured a smooth and efficient experience. This is the beauty of modern web developmentāevery click, every request, and every interaction is powered by thousands of lines of code and well-designed architectures that make our lives easier without us even noticing. So, the next time you order your favorite chai online, take a moment to appreciate the invisible magic that makes it all possible. āš
Subscribe to my newsletter
Read articles from Alok Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
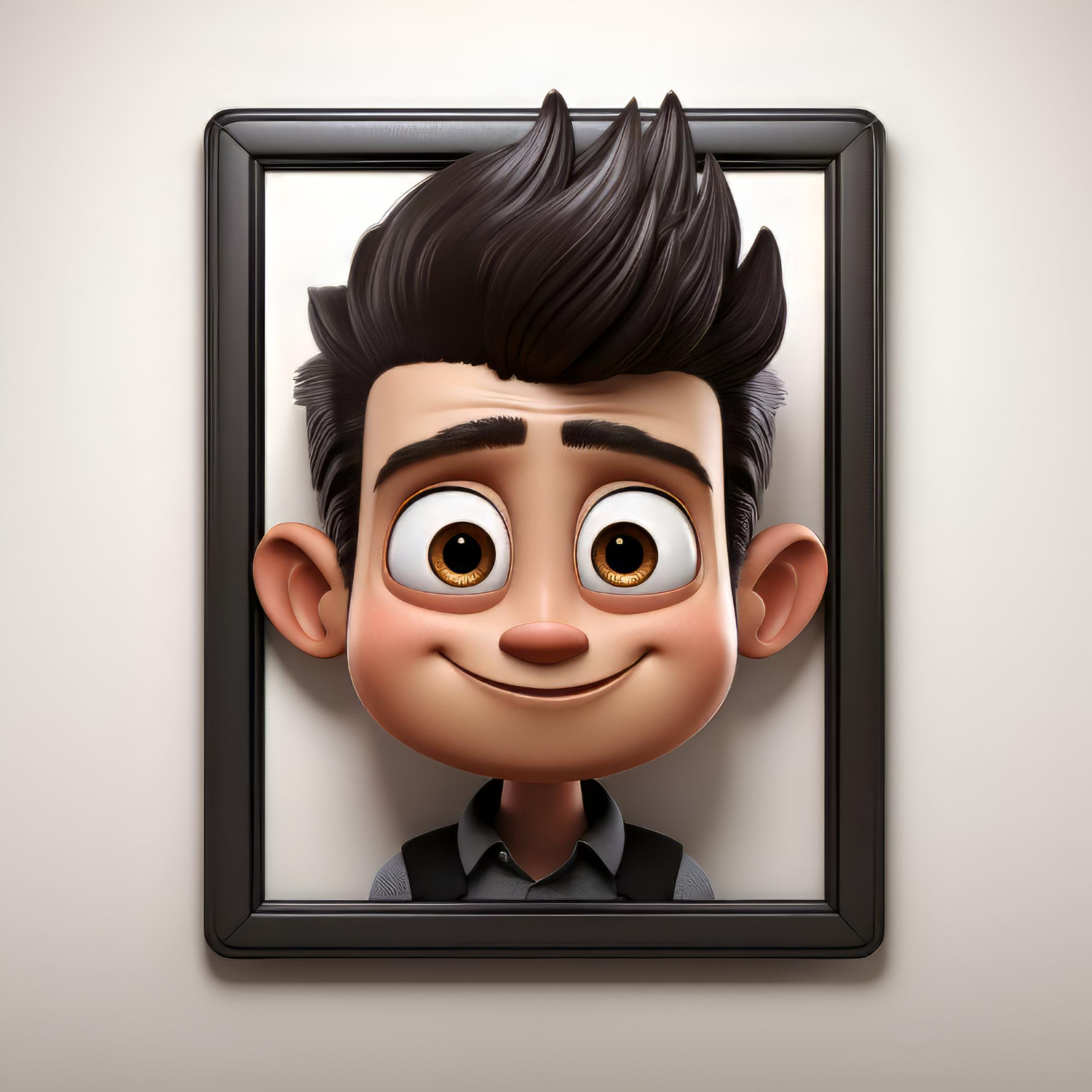
Alok Gupta
Alok Gupta
Iām an aspiring web developer keen on learning new things. Iām dedicated to keeping up to date with the latest stuffs in web development. I always lookout for chances to grow and learn. When Iām not coding, I enjoy reading about Indiaās fascinating history. It helps satisfy my curiosity and teaches me about the diverse India.