Building a Simple Password Strength Checker with JavaScript

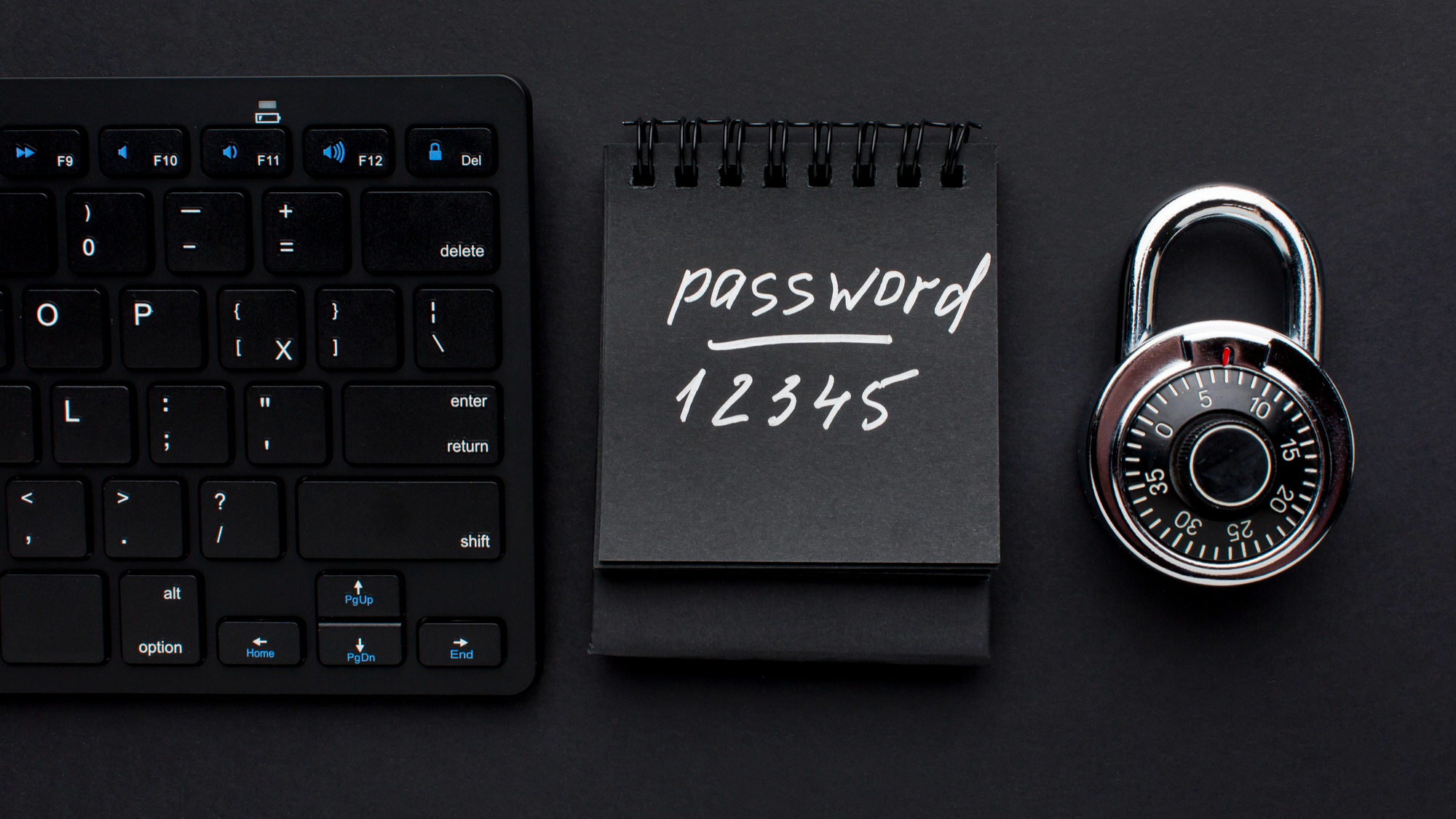
In today's digital world, strong passwords are the first line of defense against unauthorized access to our accounts. As web developers, we have a responsibility to help users create strong passwords by providing real-time feedback. In this blog post, we'll build a comprehensive password strength checker using JavaScript that evaluates passwords based on multiple criteria and provides meaningful feedback to users.
Why Password Strength Matters
Before diving into the code, let's understand why password strength is crucial:
Weak passwords can be cracked in seconds using modern computing power
Data breaches expose millions of passwords yearly
Many users reuse passwords across multiple sites
Stronger passwords exponentially increase the time needed to crack them
A good password strength checker encourages users to create passwords that are:
Long enough
Use a mix of character types
Avoid common patterns or dictionary words
Are unique and memorable
Our Approach
Our password strength checker will:
Evaluate password strength based on multiple criteria
Provide a strength score from 0-100
Give specific feedback on how to improve the password
Update in real-time as the user types
Use visual cues to indicate strength level
Let's get started!
HTML Structure
First, we'll create a simple form with a password input field:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Password Strength Checker</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Password Strength Checker</h1>
<div class="password-container">
<label for="password">Enter a password:</label>
<input type="password" id="password" placeholder="Type your password here">
<button id="toggle-password" type="button">Show</button>
</div>
<div class="strength-meter">
<div class="strength-bar" id="strength-bar"></div>
</div>
<div id="strength-text">Strength: </div>
<ul id="feedback-list"></ul>
</div>
<script src="script.js"></script>
</body>
</html>
CSS Styling
Next, let's add some CSS to make our checker visually appealing:
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
line-height: 1.6;
margin: 0;
padding: 0;
background-color: #f5f5f5;
color: #333;
}
.container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
background-color: white;
border-radius: 8px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
color: #2c3e50;
}
.password-container {
position: relative;
margin-bottom: 20px;
}
label {
display: block;
margin-bottom: 8px;
font-weight: bold;
}
input[type="password"] {
width: 100%;
padding: 12px;
border: 1px solid #ddd;
border-radius: 4px;
font-size: 16px;
box-sizing: border-box;
}
#toggle-password {
position: absolute;
right: 10px;
top: 38px;
background: none;
border: none;
cursor: pointer;
color: #666;
}
.strength-meter {
height: 8px;
background-color: #eee;
border-radius: 4px;
margin-bottom: 20px;
overflow: hidden;
}
.strength-bar {
height: 100%;
width: 0%;
transition: width 0.5s, background-color 0.5s;
}
#strength-text {
margin-bottom: 15px;
font-weight: bold;
}
#feedback-list {
padding-left: 20px;
}
#feedback-list li {
margin-bottom: 5px;
}
.weak {
background-color: #ff4d4d;
}
.medium {
background-color: #ffa64d;
}
.strong {
background-color: #ffff4d;
}
.very-strong {
background-color: #4dff4d;
}
JavaScript Implementation
Now, let's implement the core functionality in JavaScript:
document.addEventListener('DOMContentLoaded', function() {
// Get DOM elements
const passwordInput = document.getElementById('password');
const strengthBar = document.getElementById('strength-bar');
const strengthText = document.getElementById('strength-text');
const feedbackList = document.getElementById('feedback-list');
const toggleButton = document.getElementById('toggle-password');
// Toggle password visibility
toggleButton.addEventListener('click', function() {
if (passwordInput.type === 'password') {
passwordInput.type = 'text';
toggleButton.textContent = 'Hide';
} else {
passwordInput.type = 'password';
toggleButton.textContent = 'Show';
}
});
// Listen for input changes
passwordInput.addEventListener('input', function() {
const password = passwordInput.value;
const result = checkPasswordStrength(password);
// Update the strength bar
strengthBar.style.width = result.score + '%';
// Update the strength class
strengthBar.className = 'strength-bar';
if (result.score < 25) {
strengthBar.classList.add('weak');
} else if (result.score < 50) {
strengthBar.classList.add('medium');
} else if (result.score < 75) {
strengthBar.classList.add('strong');
} else {
strengthBar.classList.add('very-strong');
}
// Update the strength text
strengthText.textContent = 'Strength: ' + result.strength;
// Update the feedback list
feedbackList.innerHTML = '';
result.feedback.forEach(item => {
const li = document.createElement('li');
li.textContent = item;
feedbackList.appendChild(li);
});
});
// Password strength checker function
function checkPasswordStrength(password) {
// Initialize score and feedback array
let score = 0;
const feedback = [];
// If password is empty, return early
if (password.length === 0) {
return {
score: 0,
strength: 'None',
feedback: ['Please enter a password']
};
}
// Check length
if (password.length < 8) {
feedback.push('Password should be at least 8 characters long');
} else {
score += 20;
if (password.length > 12) {
score += 10;
}
}
// Check for lowercase letters
if (!/[a-z]/.test(password)) {
feedback.push('Add lowercase letters');
} else {
score += 10;
}
// Check for uppercase letters
if (!/[A-Z]/.test(password)) {
feedback.push('Add uppercase letters');
} else {
score += 10;
}
// Check for numbers
if (!/[0-9]/.test(password)) {
feedback.push('Add numbers');
} else {
score += 10;
}
// Check for special characters
if (!/[^A-Za-z0-9]/.test(password)) {
feedback.push('Add special characters');
} else {
score += 15;
}
// Check for repeated characters
if (/(.)\1{2,}/.test(password)) {
feedback.push('Avoid using repeated characters');
score -= 10;
}
// Check for sequences
const sequences = ['abcdef', '123456', 'qwerty'];
for (const seq of sequences) {
if (password.toLowerCase().includes(seq)) {
feedback.push('Avoid common sequences');
score -= 10;
break;
}
}
// Check for common passwords (simplified)
const commonPasswords = ['password', 'admin', '123456', 'qwerty', 'welcome'];
if (commonPasswords.includes(password.toLowerCase())) {
feedback.push('Avoid commonly used passwords');
score = Math.max(0, score - 30);
}
// Add variety bonus for using different character types
let varietyCount = 0;
if (/[a-z]/.test(password)) varietyCount++;
if (/[A-Z]/.test(password)) varietyCount++;
if (/[0-9]/.test(password)) varietyCount++;
if (/[^A-Za-z0-9]/.test(password)) varietyCount++;
if (varietyCount >= 3 && password.length >= 8) {
score += 15;
}
// Cap the score at 100
score = Math.min(100, Math.max(0, score));
// Determine strength category
let strength;
if (score < 25) {
strength = 'Very Weak';
} else if (score < 50) {
strength = 'Weak';
} else if (score < 75) {
strength = 'Strong';
} else {
strength = 'Very Strong';
}
// If password is strong and there's no feedback, add a positive message
if (score >= 75 && feedback.length === 0) {
feedback.push('Excellent password!');
}
return {
score,
strength,
feedback
};
}
});
Understanding the Password Strength Algorithm
Let's break down how our password strength algorithm works:
Basic Criteria
Our algorithm evaluates passwords based on several key criteria:
Length: Longer passwords get higher scores
8+ characters: +20 points
12+ characters: additional +10 points
Character variety:
Lowercase letters: +10 points
Uppercase letters: +10 points
Numbers: +10 points
Special characters: +15 points
Penalizations:
Repeated characters: -10 points
Common sequences: -10 points
Common passwords: up to -30 points
Bonus points:
- Using 3+ character types with 8+ length: +15 points
Score Calculation
The final score is calculated by adding and subtracting points based on these criteria, with a minimum of 0 and a maximum of 100. The score is then mapped to a strength category:
0-24: Very Weak
25-49: Weak
50-74: Strong
75-100: Very Strong
Feedback Generation
One of the most useful aspects of our checker is the specific feedback it provides. For each criterion that isn't met, we add a clear, actionable suggestion to help users improve their password.
Enhancing the Checker
Now let's add some advanced features to make our checker even more robust:
Entropy Calculation
Entropy is a measure of how unpredictable a password is. Let's add an entropy estimator:
// Add this to your checkPasswordStrength function
function calculateEntropy(password) {
let charset = 0;
if (/[a-z]/.test(password)) charset += 26;
if (/[A-Z]/.test(password)) charset += 26;
if (/[0-9]/.test(password)) charset += 10;
if (/[^A-Za-z0-9]/.test(password)) charset += 33; // Approx. special chars
// Shannon entropy formula: log2(charset^length)
// Simplified to: length * log2(charset)
return password.length * (Math.log(charset) / Math.log(2));
}
// Inside the function, add this calculation
const entropy = calculateEntropy(password);
// Adjust score based on entropy
if (entropy > 60) score += 10;
Dictionary Word Check
Let's add a simplified check for dictionary words:
// Add this inside the checkPasswordStrength function
const commonWords = ['password', 'welcome', 'admin', 'hello', 'office', 'monkey', 'dragon', 'baseball', 'football', 'letmein', 'master', 'sunshine', 'shadow'];
// Check if password contains a common word
for (const word of commonWords) {
if (password.toLowerCase().includes(word) && word.length > 3) {
feedback.push('Avoid using common words in your password');
score = Math.max(0, score - 15);
break;
}
}
Full Enhanced Version
Here's the complete enhanced JavaScript code:
document.addEventListener('DOMContentLoaded', function() {
// Get DOM elements
const passwordInput = document.getElementById('password');
const strengthBar = document.getElementById('strength-bar');
const strengthText = document.getElementById('strength-text');
const feedbackList = document.getElementById('feedback-list');
const toggleButton = document.getElementById('toggle-password');
// Toggle password visibility
toggleButton.addEventListener('click', function() {
if (passwordInput.type === 'password') {
passwordInput.type = 'text';
toggleButton.textContent = 'Hide';
} else {
passwordInput.type = 'password';
toggleButton.textContent = 'Show';
}
});
// Listen for input changes
passwordInput.addEventListener('input', function() {
const password = passwordInput.value;
const result = checkPasswordStrength(password);
// Update the strength bar
strengthBar.style.width = result.score + '%';
// Update the strength class
strengthBar.className = 'strength-bar';
if (result.score < 25) {
strengthBar.classList.add('weak');
} else if (result.score < 50) {
strengthBar.classList.add('medium');
} else if (result.score < 75) {
strengthBar.classList.add('strong');
} else {
strengthBar.classList.add('very-strong');
}
// Update the strength text
strengthText.textContent = 'Strength: ' + result.strength;
// Update the feedback list
feedbackList.innerHTML = '';
result.feedback.forEach(item => {
const li = document.createElement('li');
li.textContent = item;
feedbackList.appendChild(li);
});
});
// Password strength checker function
function checkPasswordStrength(password) {
// Initialize score and feedback array
let score = 0;
const feedback = [];
// If password is empty, return early
if (password.length === 0) {
return {
score: 0,
strength: 'None',
feedback: ['Please enter a password']
};
}
// Check length
if (password.length < 8) {
feedback.push('Password should be at least 8 characters long');
} else {
score += 20;
if (password.length > 12) {
score += 10;
}
}
// Check for lowercase letters
if (!/[a-z]/.test(password)) {
feedback.push('Add lowercase letters');
} else {
score += 10;
}
// Check for uppercase letters
if (!/[A-Z]/.test(password)) {
feedback.push('Add uppercase letters');
} else {
score += 10;
}
// Check for numbers
if (!/[0-9]/.test(password)) {
feedback.push('Add numbers');
} else {
score += 10;
}
// Check for special characters
if (!/[^A-Za-z0-9]/.test(password)) {
feedback.push('Add special characters');
} else {
score += 15;
}
// Check for repeated characters
if (/(.)\1{2,}/.test(password)) {
feedback.push('Avoid using repeated characters');
score -= 10;
}
// Check for sequences
const sequences = ['abcdef', '123456', 'qwerty', 'password', 'abc123'];
for (const seq of sequences) {
if (password.toLowerCase().includes(seq)) {
feedback.push('Avoid common sequences');
score -= 10;
break;
}
}
// Common passwords check (simplified)
const commonPasswords = ['password', 'admin', '123456', 'qwerty', 'welcome', 'letmein', 'football', 'baseball', 'dragon'];
if (commonPasswords.includes(password.toLowerCase())) {
feedback.push('Avoid commonly used passwords');
score = Math.max(0, score - 30);
}
// Common word check
const commonWords = ['password', 'welcome', 'admin', 'hello', 'office', 'monkey', 'dragon', 'baseball', 'football', 'letmein', 'master', 'sunshine', 'shadow'];
for (const word of commonWords) {
if (password.toLowerCase().includes(word) && word.length > 3) {
feedback.push('Avoid using common words in your password');
score = Math.max(0, score - 15);
break;
}
}
// Calculate entropy
const entropy = calculateEntropy(password);
// Adjust score based on entropy
if (entropy > 60) score += 10;
// Add variety bonus for using different character types
let varietyCount = 0;
if (/[a-z]/.test(password)) varietyCount++;
if (/[A-Z]/.test(password)) varietyCount++;
if (/[0-9]/.test(password)) varietyCount++;
if (/[^A-Za-z0-9]/.test(password)) varietyCount++;
if (varietyCount >= 3 && password.length >= 8) {
score += 15;
}
// Cap the score at 100
score = Math.min(100, Math.max(0, score));
// Determine strength category
let strength;
if (score < 25) {
strength = 'Very Weak';
} else if (score < 50) {
strength = 'Weak';
} else if (score < 75) {
strength = 'Strong';
} else {
strength = 'Very Strong';
}
// If password is strong and there's no feedback, add a positive message
if (score >= 75 && feedback.length === 0) {
feedback.push('Excellent password!');
}
return {
score,
strength,
feedback,
entropy: Math.round(entropy)
};
}
// Function to calculate password entropy
function calculateEntropy(password) {
let charset = 0;
if (/[a-z]/.test(password)) charset += 26;
if (/[A-Z]/.test(password)) charset += 26;
if (/[0-9]/.test(password)) charset += 10;
if (/[^A-Za-z0-9]/.test(password)) charset += 33; // Approx. special chars
// Shannon entropy formula: log2(charset^length)
// Simplified to: length * log2(charset)
return password.length * (Math.log(charset) / Math.log(2));
}
});
Adding Advanced Features
Let's enhance our checker with two more advanced features:
1. Password Generation
Let's add a password generator feature to help users create strong passwords:
// Add this to your HTML
<div class="generator-container">
<button id="generate-password">Generate Strong Password</button>
<span id="generated-password"></span>
</div>
// Add this to your JavaScript
const generateButton = document.getElementById('generate-password');
const generatedPasswordSpan = document.getElementById('generated-password');
generateButton.addEventListener('click', function() {
const password = generateStrongPassword();
generatedPasswordSpan.textContent = password;
passwordInput.value = password;
// Trigger the input event to update the strength meter
const inputEvent = new Event('input');
passwordInput.dispatchEvent(inputEvent);
});
function generateStrongPassword() {
const lowercase = 'abcdefghijklmnopqrstuvwxyz';
const uppercase = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
const numbers = '0123456789';
const specialChars = '!@#$%^&*()-_=+[]{}|;:,.<>?';
const allChars = lowercase + uppercase + numbers + specialChars;
// Generate a password of length 12-16
const length = Math.floor(Math.random() * 5) + 12;
let password = '';
// Ensure at least one of each character type
password += lowercase.charAt(Math.floor(Math.random() * lowercase.length));
password += uppercase.charAt(Math.floor(Math.random() * uppercase.length));
password += numbers.charAt(Math.floor(Math.random() * numbers.length));
password += specialChars.charAt(Math.floor(Math.random() * specialChars.length));
// Fill the rest randomly
for (let i = 4; i < length; i++) {
password += allChars.charAt(Math.floor(Math.random() * allChars.length));
}
// Shuffle the password
return password.split('').sort(() => 0.5 - Math.random()).join('');
}
2. Time to Crack Estimation
Let's add an estimate of how long it would take to crack the password:
// Add this to your checkPasswordStrength function result
// Calculate time to crack (simplified model)
function calculateCrackTime(entropy) {
// Assumes 10 billion guesses per second (modern hardware)
const guessesPerSecond = 10000000000;
// Number of possible combinations = 2^entropy
const possibleCombinations = Math.pow(2, entropy);
// Average time to crack in seconds (assuming 50% of combinations need to be tried)
const secondsToCrack = possibleCombinations / (2 * guessesPerSecond);
return formatTimeToHuman(secondsToCrack);
}
function formatTimeToHuman(seconds) {
if (seconds < 60) {
return 'less than a minute';
} else if (seconds < 3600) {
return Math.floor(seconds / 60) + ' minutes';
} else if (seconds < 86400) {
return Math.floor(seconds / 3600) + ' hours';
} else if (seconds < 31536000) {
return Math.floor(seconds / 86400) + ' days';
} else if (seconds < 3153600000) {
return Math.floor(seconds / 31536000) + ' years';
} else {
const years = Math.floor(seconds / 31536000);
if (years < 1000) {
return years + ' years';
} else if (years < 1000000) {
return Math.floor(years / 1000) + ' thousand years';
} else if (years < 1000000000) {
return Math.floor(years / 1000000) + ' million years';
} else {
return 'billions of years';
}
}
}
// Then add this to the return object
return {
score,
strength,
feedback,
entropy: Math.round(entropy),
crackTime: calculateCrackTime(entropy)
};
// And update the strengthText line to show this:
strengthText.textContent = `Strength: ${result.strength} (Entropy: ${result.entropy} bits) - Time to crack: ${result.crackTime}`;
Final UI Enhancements
To make our password strength checker more user-friendly, let's add a few final touches:
- Add CSS for the generator container:
.generator-container {
margin-top: 20px;
padding: 15px;
background-color: #f9f9f9;
border-radius: 4px;
border: 1px solid #ddd;
}
#generate-password {
background-color: #4CAF50;
color: white;
border: none;
padding: 8px 16px;
border-radius: 4px;
cursor: pointer;
font-size: 14px;
}
#generate-password:hover {
background-color: #45a049;
}
#generated-password {
display: inline-block;
margin-left: 15px;
font-family: monospace;
padding: 5px;
background-color: #eee;
border-radius: 3px;
}
- Add a copy button for the generated password:
<div class="generator-container">
<button id="generate-password">Generate Strong Password</button>
<span id="generated-password"></span>
<button id="copy-password" style="display: none;">Copy</button>
</div>
const copyButton = document.getElementById('copy-password');
generateButton.addEventListener('click', function() {
const password = generateStrongPassword();
generatedPasswordSpan.textContent = password;
passwordInput.value = password;
copyButton.style.display = 'inline-block';
// Trigger the input event to update the strength meter
const inputEvent = new Event('input');
passwordInput.dispatchEvent(inputEvent);
});
copyButton.addEventListener('click', function() {
navigator.clipboard.writeText(generatedPasswordSpan.textContent)
.then(() => {
const originalText = copyButton.textContent;
copyButton.textContent = 'Copied!';
setTimeout(() => {
copyButton.textContent = originalText;
}, 2000);
})
.catch(err => {
console.error('Failed to copy: ', err);
});
});
Best Practices for Password Strength Checking
As we wrap up our implementation, let's review some best practices:
Don't block weak passwords entirely - Instead, encourage stronger ones through feedback
Focus on entropy - The unpredictability of a password is more important than complexity rules
Provide actionable feedback - Tell users exactly how to improve their passwords
Use visual indicators - Color-coded meters help users understand password strength at a glance
Consider user experience - Balance security with usability to prevent frustration
Stay updated - Password security evolves, so keep your criteria current
Conclusion
Building a robust password strength checker is an important step in improving the security of your web applications. Our implementation:
Evaluates passwords using multiple criteria
Provides real-time visual feedback
Offers specific suggestions for improvement
Includes advanced features like entropy calculation and time-to-crack estimation
Helps users generate strong passwords
By implementing this password strength checker, you're not just enforcing security policies—you're educating users about better password practices.
Next Steps
To further enhance this password strength checker, consider:
Implementing a server-side component to check against large databases of breached passwords
Adding localization for multiple languages
Creating analytics to track password strength patterns (anonymously) across your user base
Implementing a version that works with password managers
The complete code for this tutorial is available in the accompanying code examples. Feel free to adapt and extend it for your own projects!
Happy coding, and stay secure!
Subscribe to my newsletter
Read articles from Learn Computer Academy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
