Mastering Angular Encapsulation: A Deep Dive into ViewEncapsulation Modes

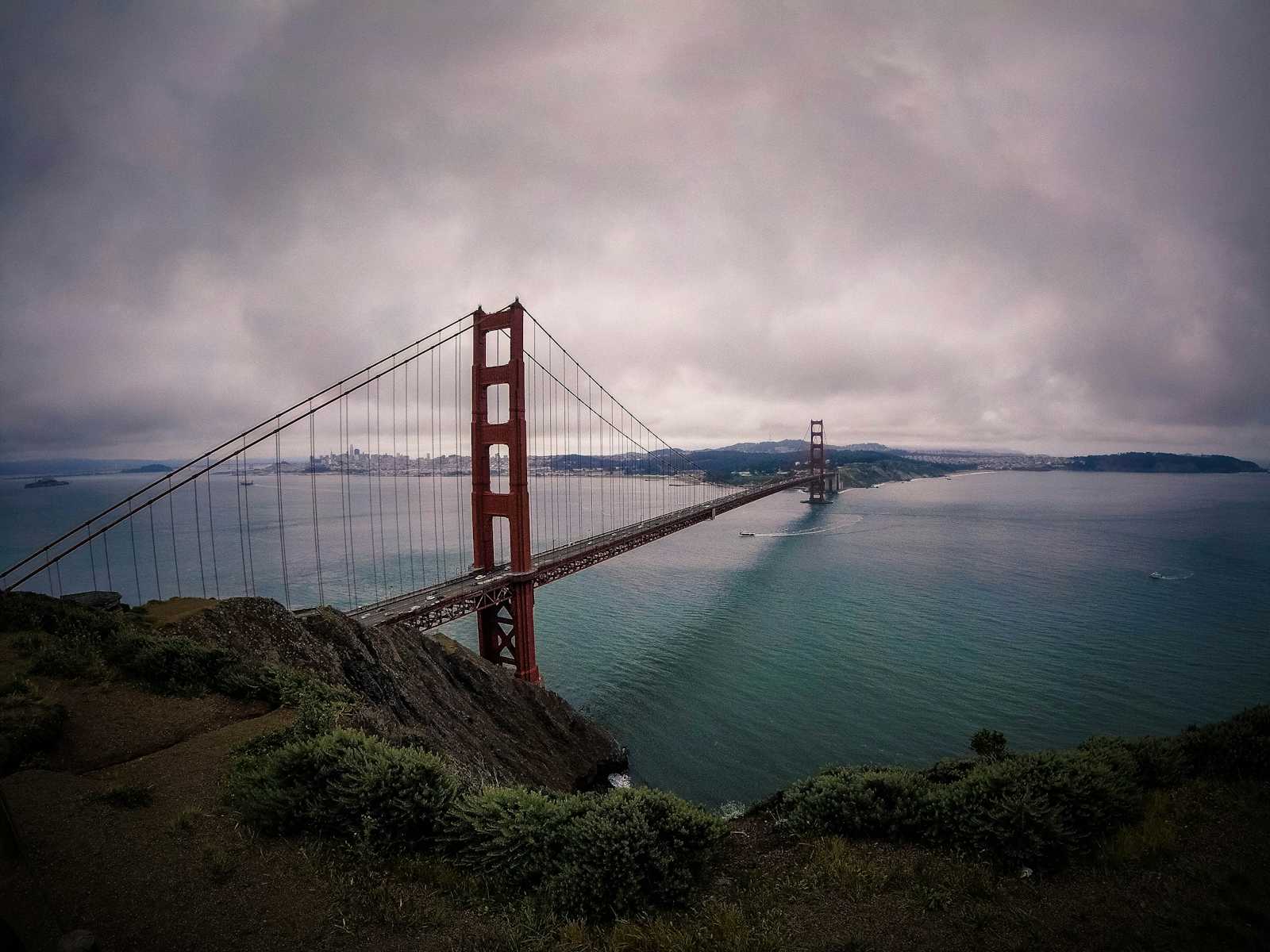
Encapsulation in Angular
Encapsulation in Angular refers to how a component's styles and template are isolated from the rest of the application. It ensures that styles defined within a component do not "leak out" and affect other parts of the application, and vice versa. This isolation is critical for maintaining modularity, scalability, and maintainability in large-scale applications.
Angular provides three primary encapsulation modes, each with its own behavior and use cases. Let’s dive into them with more detail, examples, and practical considerations.
1. ViewEncapsulation.Emulated (Default)
This is the default encapsulation mode in Angular. It emulates the behavior of shadow DOM without relying on the browser's native shadow DOM API.
How It Works
Angular adds unique attributes to the HTML elements within the component's template.
These attributes act as "scopes" for the component's styles.
The styles defined in the component's CSS file are rewritten to include these attributes as selectors, ensuring they apply only to the component's elements.
For example:
@Component({
selector: 'app-example',
template: `<p>Hello World</p>`,
styles: [`p { color: red; }`],
encapsulation: ViewEncapsulation.Emulated // Default
})
export class ExampleComponent {}
The rendered output might look like this:
<p _ngcontent-c123456789="true">Hello World</p>
And the CSS will be transformed to:
p[_ngcontent-c123456789] {
color: red;
}
Benefits
Provides good encapsulation without requiring native shadow DOM support.
Works consistently across all browsers, including older ones.
No need for special styling techniques or browser-specific workarounds.
Drawbacks
Styles can still be overridden if global styles have higher specificity or use
!important
.Encapsulation is not as strong as true shadow DOM, so there may still be edge cases where styles bleed out.
When to Use
- Use this mode for most components in your application. It strikes a balance between encapsulation and compatibility.
2. ViewEncapsulation.ShadowDom
This mode uses the browser's native shadow DOM API to encapsulate the component's view.
How It Works
Angular creates a shadow root for the component's host element.
The component's template and styles are rendered inside this shadow root.
Styles defined within the shadow DOM are completely isolated from the rest of the page, and external styles cannot affect the component unless explicitly allowed (e.g., using CSS custom properties or
::part
).
Example:
@Component({
selector: 'app-shadow-dom',
template: `<p>Hello Shadow DOM</p>`,
styles: [`p { color: green; }`],
encapsulation: ViewEncapsulation.ShadowDom
})
export class ShadowDomComponent {}
The rendered output will look like this:
<app-shadow-dom>
#shadow-root
<p>Hello Shadow DOM</p>
</app-shadow-dom>
Benefits
Provides the strongest form of encapsulation.
Native browser implementation ensures high performance.
Ideal for creating reusable, self-contained components.
Drawbacks
Shadow DOM is not supported by older browsers (e.g., Internet Explorer). However, modern browsers have excellent support.
Styling can become more complex because you need to use shadow DOM-specific techniques like
::part
or::slotted
to style elements from the outside.
When to Use
Use this mode when you need complete encapsulation and are targeting modern browsers.
Ideal for building highly modular, reusable components or web components.
3. ViewEncapsulation.None
This mode disables view encapsulation entirely, meaning styles are added to the global scope.
How It Works
Angular does not apply any encapsulation techniques.
The component's styles are added to the global CSS scope.
Styles defined in the component's CSS can affect other elements on the page, and styles from other parts of the application can affect the component's elements.
Example:
@Component({
selector: 'app-global-styles',
template: `<p>Hello Global</p>`,
styles: [`p { color: purple; }`],
encapsulation: ViewEncapsulation.None
})
export class GlobalStylesComponent {}
The rendered output will look like this:
<p>Hello Global</p>
And the CSS will be added globally:
p {
color: purple;
}
Benefits
Useful for integrating with third-party libraries that manipulate the DOM.
Allows styles to "bleed out" intentionally, which can be helpful in specific scenarios.
Drawbacks
Breaks encapsulation, leading to potential style conflicts.
Makes the application harder to maintain as the codebase grows.
When to Use
- Use this mode sparingly, only when you have a specific reason to disable encapsulation (e.g., integrating with legacy systems or third-party libraries).
How to Set View Encapsulation
You can set the view encapsulation mode for a component using the encapsulation
property in the @Component
decorator:
import { Component, ViewEncapsulation } from '@angular/core';
@Component({
selector: 'my-component',
template: `
<p>This is my component!</p>
`,
styles: [`
p {
color: blue;
}
`],
encapsulation: ViewEncapsulation.Emulated // Or ShadowDom, or None
})
export class MyComponent {}
Choosing the Right Encapsulation Mode
Here’s a decision-making guide to help you choose the appropriate encapsulation mode:
Mode | Use Case | Browser Support | Encapsulation Strength |
Emulated | Most components in your application. Balances encapsulation and compatibility. | All browsers | Medium |
ShadowDom | Reusable, modular components. Targeting modern browsers. | Modern browsers only | Strong |
None | Integrating with third-party libraries or legacy systems. | All browsers | None |
Best Practices
Stick to Emulated for Most Cases: Unless you have a specific reason to use another mode, stick with
ViewEncapsulation.Emulated
. It provides sufficient encapsulation for most use cases while maintaining broad browser compatibility.Use ShadowDom for Web Components: If you're building reusable web components or need the strongest encapsulation, use
ViewEncapsulation.ShadowDom
.Avoid None Unless Necessary: Disabling encapsulation should be avoided unless you have a compelling reason, such as integrating with legacy systems or third-party libraries.
Leverage CSS Custom Properties: When using
ShadowDom
, use CSS custom properties (--custom-property
) to allow controlled customization of styles from outside the component.Test Across Browsers: If you use
ShadowDom
, ensure you test your application across different browsers to confirm compatibility.
Conclusion
Angular's view encapsulation is a powerful feature that helps developers create modular, maintainable, and scalable applications. By understanding the different encapsulation modes—Emulated
, ShadowDom
, and None
—you can make informed decisions about how to structure your components and manage styles effectively.
Each mode has its strengths and weaknesses, and the choice depends on your specific requirements. For most applications, Emulated
is the best choice due to its balance of encapsulation and compatibility. However, for advanced use cases like building reusable web components, ShadowDom
provides the strongest encapsulation. Use None
sparingly and only when absolutely necessary.
By following best practices and leveraging the right encapsulation mode, you can build robust and maintainable Angular applications.
Final words:
The three types of encapsulation in Angular are:
ViewEncapsulation.Emulated (Default)
ViewEncapsulation.ShadowDom
ViewEncapsulation.None
Each mode serves a unique purpose, and the choice depends on your application's needs and browser support requirements.
Subscribe to my newsletter
Read articles from Rohit Bhat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
