How a 500KB Image Can Take 24MB in Memory—And What You Can Do About It

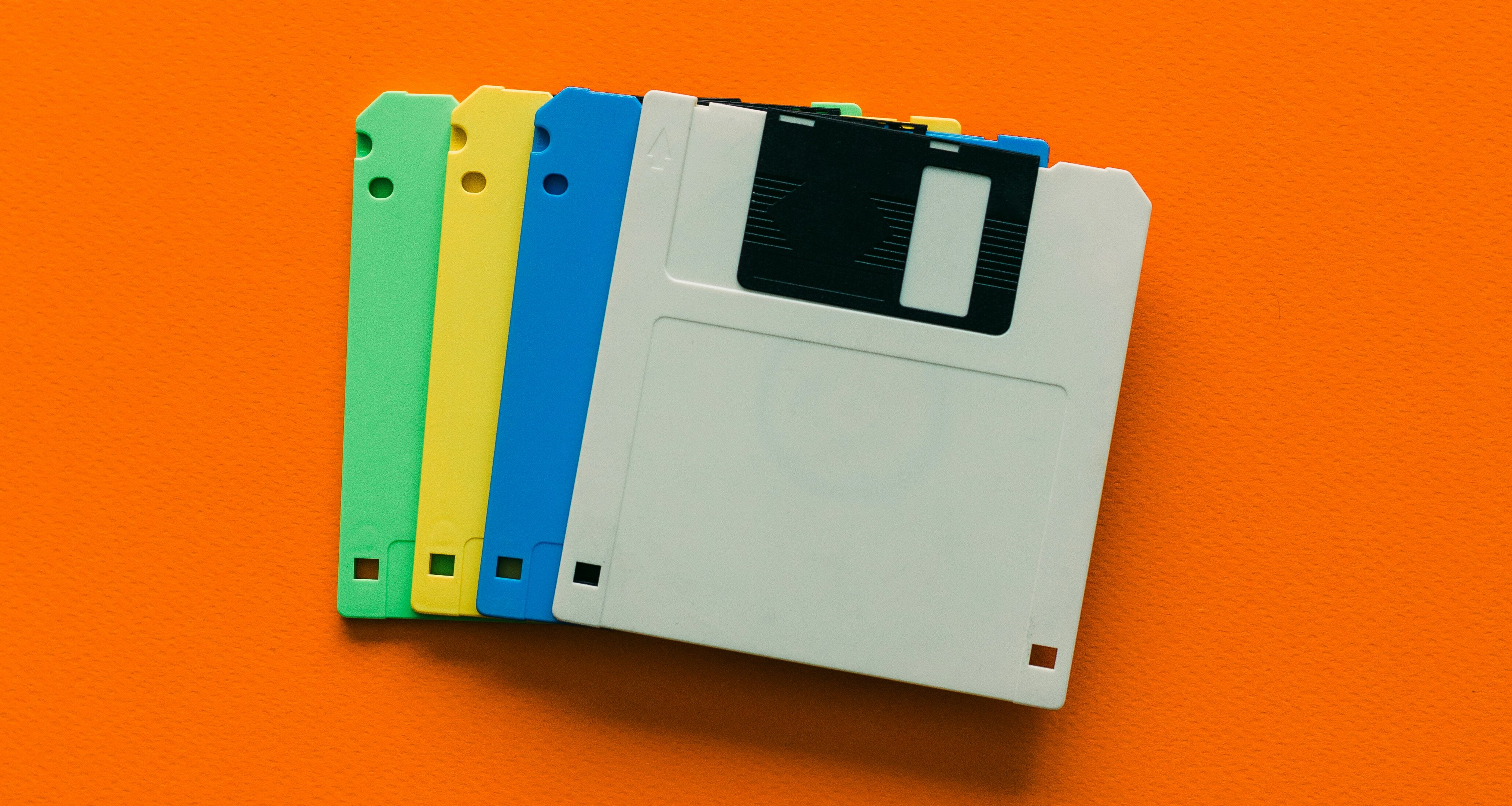
Introduction
When working on a recent client project, I encountered a serious performance issue: the app was consuming excessive memory, leading to slow performance, crashes, and issues with lists rendering large images. Profiling the app revealed that image handling was a major culprit, especially with high-resolution assets stored in the project and fetched from the server. Through debugging, I discovered a shocking crucial detail: an image's in-memory representation can be significantly larger than its file size on disk.
In this article, I'll break down why this happens and share practical strategies on how you can optimize image memory usage in iOS apps.
Why Do Images Take Up So Much Memory?
The key to understanding image memory usage lies in how images are stored on disk versus how they are processed in memory.
Compressed Image (On Disk) vs. Uncompressed Image (In Memory)
Images on disk are often stored in compressed formats like JPEG or PNG, which significantly reduce file size. However, when an image is loaded into memory, it needs to be decompressed into a raw format that the system can render efficiently.
For example, let’s consider a 500KB JPEG image with a resolution of 3000x2000 pixels:
JPEG file size (on disk): 500KB (compressed)
Decompressed size (in memory):
Each pixel typically requires 4 bytes (RGBA: Red, Green, Blue, Alpha)
Total pixels: 3000 x 2000 = 6,000,000 pixels
Memory required: 6,000,000 x 4 bytes = 24MB
If the image has a higher resolution or uses more color data, this can easily double, leading to 48MB or more of memory usage per image!
Now, imagine rendering multiple high-resolution images in a list, the memory usage quickly adds up, causing performance issues, lag, and even crashes on devices with limited RAM.
Debugging the Issue
How I Discovered the Problem
During development, I noticed that:
The app became slower when loading images from the API.
Scrolling lists with images lagged or caused crashes.
Xcode Instruments showed high memory usage and warnings.
To isolate the issue, I tried:
Removing all resource-intensive work (but the issue persisted).
Disabling media loading completely, and suddenly, the app became much faster.
This confirmed that images were the primary cause of excessive memory consumption.
Optimizing Image Memory Usage
To solve this issue, I applied several best practices that significantly reduced memory usage and improved performance.
1. Downsample Large Images Before Displaying
Instead of loading full-resolution images into memory, downsample them to a smaller size that matches the display size. This ensured that images use only as much memory as needed, significantly reducing their footprint while maintaining visual quality.
2. Cache Images to Avoid Re-Loading
Repeatedly decoding and rendering images is expensive. Using an image caching solution prevents redundant memory usage by storing images in memory or disk after they are first loaded, reducing the need for repeated decoding. Using NSCache
in my case
3. Use Efficient Rendering Techniques
Avoid keeping multiple large images in memory at the same time. Instead, I used lazy loading and remove images that are no longer visible. Additionally, I ensured that image views reuse existing memory rather than creating new instances repeatedly.
4. Monitor and Profile Memory Usage
Using Xcode’s Instruments (especially the Memory Profiler and Leaks tool) to analyze how the app handles images. This helped identify excessive memory consumption and potential leaks, allowing me to fine-tune the app performance.
Key Takeaways from This Experience
Here are some key takeaways I learned from debugging, researching, and understanding these issues:
In-memory images are much larger than their file size on disk due to decompression.
Profiling tools like Xcode Instruments is a friend, and can help identify excessive memory usage.
Optimizing image handling with downsampling, caching, and efficient formats significantly improves performance.
By applying these strategies, I was able to reduce memory usage and improve responsiveness in a client’s app. If you're facing similar issues, these techniques could help ensure a smoother experience for users.
Further Reading & References
If you want to dive deeper into optimizing memory usage in iOS, check out these resources:
Medium: Downsampling images for better memory consumption and UICollectionView performance
Short Reddit Discussion: How to optimize memory usage when loading images
What’s Next?
If you found this article helpful, feel free to share your thoughts! Have you faced similar memory issues in your apps? Let’s discuss in the comments! 🚀
For more insights into my work and interests, feel free to connect with me on LinkedIn, Twitter or explore my projects on GitHub.
Subscribe to my newsletter
Read articles from Cédric Bahirwe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Cédric Bahirwe
Cédric Bahirwe
iOS Engineer building world-class iOS apps using SwiftUI, UIKit and Whatnots