Day 18: JavaScript Best Practicesโ-โWriting Clean & Maintainable Codeย ๐

Table of contents
- 1. Use Meaningful and Descriptive Variable Names
- 2. Keep Functions Small and Focused
- 3. Avoid Global Variables
- 4. Use Template Literals for String Concatenation
- 5. Use Default Parameters
- 6. Use the Spread and Rest Operators
- 7. Handle Errors with Try-Catch Blocks
- 8. Use Strict Mode for Safer Code
- 9. Optimize Loops & Use Array Methods
- Conclusion ๐ฏ
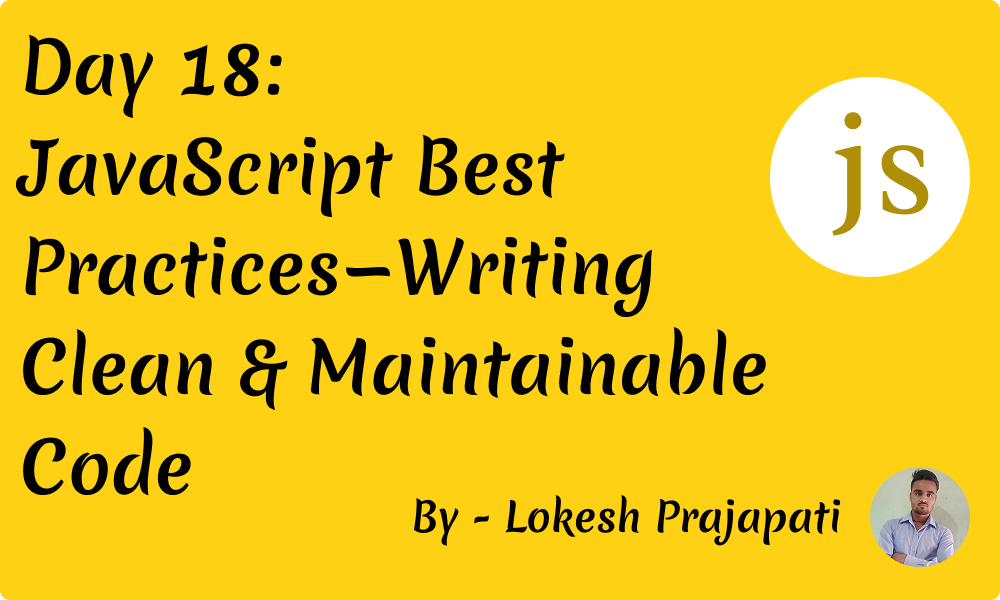
JavaScript is one of the most powerful programming languages, but writing clean, efficient, and maintainable code is key to ensuring long-term success. Whether youโre working solo or with a team, following best practices helps improve readability, maintainability, and performance.
1. Use Meaningful and Descriptive Variable Names
Poorly named variables can make your code hard to understand. Use clear and meaningful names that describe their purpose.
Bad Example:
let x = 10;
let y = 20;
let z = x + y;
console.log(z);
Good Example:
let price = 10;
let tax = 20;
let totalCost = price + tax;
console.log(totalCost);
โ
Tip: Use camelCase for variables (totalCost
), PascalCase for classes (UserProfile
), and UPPER_CASE for constants (MAX_LIMIT
).
2. Keep Functions Small and Focused
A function should perform a single task effectively. If it does more, consider breaking it into smaller functions.
Bad Example:
function processUserData(user) {
console.log("Saving user data...");
database.save(user);
sendEmail(user.email);
logActivity(user.id, "User data processed");
}
Good Example:
function saveUser(user) {
database.save(user);
}
function notifyUser(email) {
sendEmail(email);
}
function logUserActivity(userId) {
logActivity(userId, "User data processed");
}
function processUserData(user) {
saveUser(user);
notifyUser(user.email);
logUserActivity(user.id);
}
โ Tip: Follow the Single Responsibility Principle (SRP) to keep functions clean and reusable.
3. Avoid Global Variables
Global variables can lead to conflicts and unexpected behavior in large applications.
Bad Example:
var count = 0; // Global variable
function increment() {
count++;
}
Good Example:
function counter() {
let count = 0;
return function () {
return ++count;
};
}
const increment = counter();
console.log(increment());
console.log(increment());
โ
Tip: Use let
and const
instead of var
to avoid polluting the global scope.
4. Use Template Literals for String Concatenation
String concatenation with +
can make code look cluttered. Template literals (``
) improve readability.
Bad Example:
const name = "Alice";
const message = "Hello, " + name + "! Welcome to JavaScript.";
Good Example:
const name = "Alice";
const message = `Hello, ${name}! Welcome to JavaScript.`;
โ Tip: Template literals support multi-line strings, making complex text formatting easier.
5. Use Default Parameters
Default parameters prevent function errors when arguments are missing.
Bad Example:
function greet(name) {
if (!name) {
name = "Guest";
}
console.log(`Hello, ${name}!`);
}
Good Example:
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
โ Tip: Always provide sensible default values for function parameters.
6. Use the Spread and Rest Operators
The spread (...
) operator expands arrays, while the rest (...
) operator gathers multiple arguments.
Example: Copying an Array
const numbers = [1, 2, 3];
const newNumbers = [...numbers, 4, 5];
console.log(newNumbers); // Output: [1, 2, 3, 4, 5]
Example: Using Rest Operator in Functions
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4)); // Output: 10
โ Tip: The spread operator helps in merging objects and arrays efficiently.
7. Handle Errors with Try-Catch Blocks
Use try-catch
to prevent crashes from unexpected errors.
Bad Example:
const data = JSON.parse(userInput); // If userInput is invalid JSON, it will throw an error
Good Example:
try {
const data = JSON.parse(userInput);
console.log("Parsed successfully:", data);
} catch (error) {
console.error("Invalid JSON format:", error.message);
}
โ Tip: Always handle potential errors to ensure a smooth user experience.
8. Use Strict Mode for Safer Code
Strict mode prevents common JavaScript pitfalls.
Example:
"use strict";
x = 10; // Throws an error because 'x' is not declared
console.log(x);
โ
Tip: Always enable "use strict"
in scripts to catch mistakes early.
9. Optimize Loops & Use Array Methods
Prefer built-in array methods over for
loops for better readability and performance.
Bad Example:
const numbers = [1, 2, 3, 4, 5];
const squared = [];
for (let i = 0; i < numbers.length; i++) {
squared.push(numbers[i] * numbers[i]);
}
Good Example:
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map(num => num * num);
โ
Tip: Use .map()
, .filter()
, and .reduce()
for cleaner code.
Conclusion ๐ฏ
Writing clean and maintainable JavaScript code is essential for scalability, teamwork, and long-term project success. By following these best practices, you can:
โ Write better structured code ๐๏ธ โ Improve readability and efficiency ๐โก โ Reduce bugs and errors ๐๐ซ
Which JavaScript best practice do you follow the most? Drop your thoughts in the comments! ๐๐
Subscribe to my newsletter
Read articles from Lokesh Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lokesh Prajapati
Lokesh Prajapati
๐ JavaScript | React | Shopify Developer | Tech Blogger Hi, Iโm Lokesh Prajapati, a passionate web developer and content creator. I love simplifying JavaScript, React, and Shopify development through easy-to-understand tutorials and real-world examples. Iโm currently running a JavaScript Basics to Advanced series on Medium & Hashnode, helping developers of all levels enhance their coding skills. My goal is to make programming more accessible and practical for everyone. Follow me for daily coding tips, tricks, and insights! Letโs learn and grow together. ๐ก๐ #JavaScript #React #Shopify #WebDevelopment #Coding #TechBlogger #LearnToCode