Building a .NET Web API

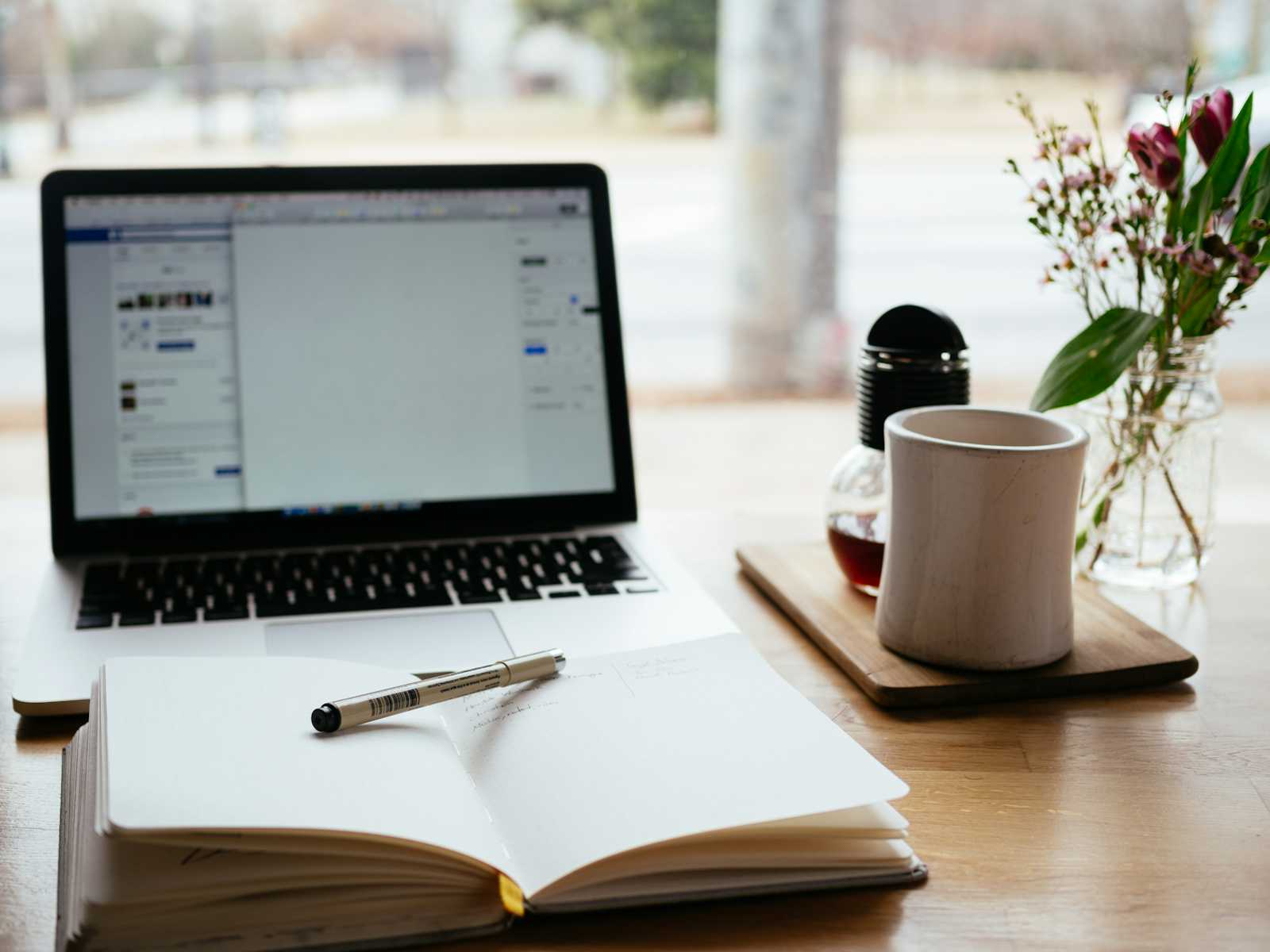
Introduction
In the modern world of software development, APIs (Application Programming Interfaces) have become the backbone of communication between different software systems. One of the most popular and robust frameworks for building APIs is the .NET framework by Microsoft. In this article, we'll dive into the essentials of building a .NET Web API, from setup to deployment.
Prerequisites
Before we get started, ensure you have the following installed:
.NET SDK: You can download it from the official Microsoft .NET website.
Visual Studio: An IDE for coding. You can also use Visual Studio Code or any other editor.
Postman: A tool for testing APIs.
Step 1: Creating a New Project
Open Visual Studio and create a new project. Select "ASP.NET Core Web API" from the project templates. Give your project a name and location, then click "Create".
Step 2: Configuring Your Project
Target Framework: Select the .NET version you want to use.
Authentication: Choose "None" for simplicity (authentication can be added later).
Other Configurations: Keep the default settings and click "Create".
Step 3: Understanding the Project Structure
Once the project is created, you'll see several folders and files:
Controllers: Contains the controllers for handling API requests.
Models: Contains the data models.
Program.cs: The entry point of the application.
Startup.cs: Configures services and the app's request pipeline.
Step 4: Creating a Model
Models represent the data structure. Create a new class in the "Models" folder:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
Step 5: Creating a Controller
Controllers handle the HTTP requests. Create a new controller in the "Controllers" folder:
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
namespace YourNamespace.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class ProductsController : ControllerBase
{
private static List<Product> products = new List<Product>
{
new Product { Id = 1, Name = "Product1", Price = 10.0M },
new Product { Id = 2, Name = "Product2", Price = 20.0M }
};
[HttpGet]
public ActionResult<IEnumerable<Product>> GetProducts()
{
return products;
}
[HttpGet("{id}")]
public ActionResult<Product> GetProduct(int id)
{
var product = products.Find(p => p.Id == id);
if (product == null)
{
return NotFound();
}
return product;
}
[HttpPost]
public ActionResult<Product> PostProduct(Product product)
{
products.Add(product);
return CreatedAtAction(nameof(GetProduct), new { id = product.Id }, product);
}
}
}
Step 6: Running Your API
Run your project by clicking on the "Run" button in Visual Studio. Your API will start, and you can test the endpoints using a tool like Postman.
Conclusion
Building a .NET Web API is straightforward and offers a lot of flexibility and power. With this guide, you've created a basic API that handles CRUD operations. You can expand this by adding authentication, logging, and other features to meet your application's needs.
Subscribe to my newsletter
Read articles from Mobeena Bee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
